Introduction to TaskExecutor interface and types
一 TaskExecutor interface
Spring’s TaskExecutor interface is equivalent to the Java.util.concurrent.Executor interface. In fact, the main reason for its existence is to abstract the dependence on Java 5 when using thread pools. This interface has only one method execute(Runnable task), which accepts an execution task according to the semantics and configuration of the thread pool.
TaskExecutor was originally created to provide a thread pool abstraction to other Spring components when needed. For example, the ApplicationEventMulticaster component, JMS's AbstractMessageListenerContainer, and the integration of Quartz all use the TaskExecutor abstraction to provide a thread pool. Of course, you can also use this abstraction layer if your beans require thread pool behavior.
二 TaskExecutor type
Some TaskExecutor implementations are predefined in the Spring distribution package. With them, you don't even need to implement it yourself anymore.
SimpleAsyncTaskExecutor Class
This implementation does not reuse any threads, or it starts a new thread every time it is called. However, it still supports setting a limit on the total number of concurrent threads. When the limit on the total number of concurrent threads is exceeded, new calls will be blocked until a position is released. If you need a real pool, read on.
SyncTaskExecutor Class
This implementation will not execute asynchronously. Instead, each call is executed in the thread that originated the call. Its main use is when multi-threading is not required, such as simple test cases.
ConcurrentTaskExecutor class
This implementation is a wrapper for the Java 5 java.util.concurrent.Executor class. There is another alternative, the ThreadPoolTaskExecutor class, which exposes the Executor's configuration parameters as bean properties. It is rarely necessary to use ConcurrentTaskExecutor, but if ThreadPoolTaskExecutor is not enough, ConcurrentTaskExecutor is another alternative.
SimpleThreadPoolTaskExecutor class
This implementation is actually a subclass of Quartz’s SimpleThreadPool class, which listens to Spring’s life cycle callbacks. This is its typical use when you have a thread pool that needs to be shared between Quartz and non-Quartz components.
ThreadPoolTaskExecutor class
It does not support any replacement or downport of the java.util.concurrent package. Both Doug Lea and Dawid Kurzyniec's implementations of java.util.concurrent use different package structures, causing them to not run correctly.
This implementation can only be used in the Java 5 environment, but it is the most commonly used in this environment. The bean properties it exposes can be used to configure a java.util.concurrent.ThreadPoolExecutor and wrap it into a TaskExecutor. If you need a more advanced class, such as ScheduledThreadPoolExecutor, we recommend that you use ConcurrentTaskExecutor instead.
TimerTaskExecutor class
This implementation uses a TimerTask as the implementation behind it. The difference between it and SyncTaskExecutor is that the method call is made in a separate thread, although it is synchronized in that thread.
WorkManagerTaskExecutor Class
CommonJ is a set of specifications jointly developed by BEA and IBM. These specifications are not Java ee standards, but they are common standards for BEA and IBM application server implementations
This implementation uses CommonJ WorkManager as its underlying implementation and is the most important to configure CommonJ WorkManager applications in Spring context the type. Similar to SimpleThreadPoolTaskExecutor, this class implements the WorkManager interface, so it can be used directly as a WorkManager.
Three Simple Examples of TaskExcutor
1 taskExcutor


package com.test;import org.springframework.core.task.TaskExecutor;public class MainExecutor { private TaskExecutor taskExecutor; public MainExecutor (TaskExecutor taskExecutor) { this.taskExecutor = taskExecutor; } public void printMessages() { for(int i = 0; i < 25; i++) { taskExecutor.execute(new MessagePrinterTask("Message" + i)); } } private class MessagePrinterTask implements Runnable { private String message; public MessagePrinterTask(String message) { this.message = message; } public void run() { System.out.println(message); } } }


package com.test;import org.springframework.context.ApplicationContext;import org.springframework.context.support.ClassPathXmlApplicationContext;public class TaskTest {//本地测试,不用部署到tomcatpublic static void main(String[] args) { System.out.println("测试任务调度开始..."); ApplicationContext appContext = new ClassPathXmlApplicationContext("applicationContext.xml"); MainExecutor te = (MainExecutor)appContext.getBean("taskExecutorExample"); te.printMessages(); System.out.println("--------"); } }
3.applicationContext.xml配置


<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "/spring-beans.dtd"><beans> <bean id="taskExecutorExample" class="com.test.MainExecutor"> <constructor-arg ref="taskExecutor" /> </bean> <bean id="taskExecutor" class="org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor"> <property name="corePoolSize" value="5" /> <property name="maxPoolSize" value="10" /> <property name="queueCapacity" value="25" /> </bean></beans>
The above is the detailed content of Introduction to TaskExecutor interface and types. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
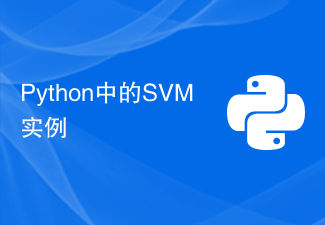
Support Vector Machine (SVM) in Python is a powerful supervised learning algorithm that can be used to solve classification and regression problems. SVM performs well when dealing with high-dimensional data and non-linear problems, and is widely used in data mining, image classification, text classification, bioinformatics and other fields. In this article, we will introduce an example of using SVM for classification in Python. We will use the SVM model from the scikit-learn library
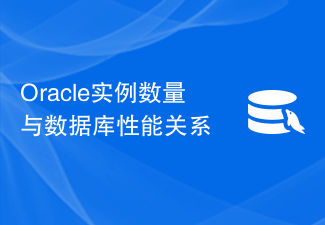
The relationship between the number of Oracle instances and database performance Oracle database is one of the well-known relational database management systems in the industry and is widely used in enterprise-level data storage and management. In Oracle database, instance is a very important concept. Instance refers to the running environment of Oracle database in memory. Each instance has an independent memory structure and background process, which is used to process user requests and manage database operations. The number of instances has an important impact on the performance and stability of Oracle database.
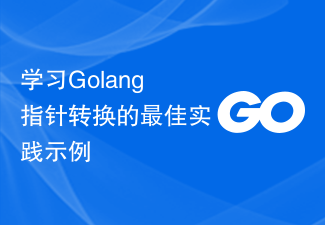
Golang is a powerful and efficient programming language that can be used to develop various applications and services. In Golang, pointers are a very important concept, which can help us operate data more flexibly and efficiently. Pointer conversion refers to the process of pointer operations between different types. This article will use specific examples to learn the best practices of pointer conversion in Golang. 1. Basic concepts In Golang, each variable has an address, and the address is the location of the variable in memory.

As the new generation of front-end frameworks continues to emerge, VUE3 is loved as a fast, flexible, and easy-to-use front-end framework. Next, let's learn the basics of VUE3 and make a simple video player. 1. Install VUE3 First, we need to install VUE3 locally. Open the command line tool and execute the following command: npminstallvue@next Then, create a new HTML file and introduce VUE3: <!doctypehtml>
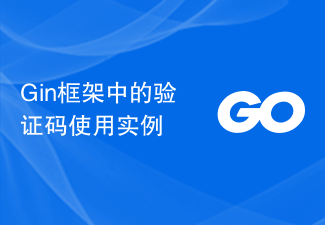
With the popularity of the Internet, verification codes have become a necessary process for login, registration, password retrieval and other operations. In the Gin framework, implementing the verification code function has become extremely simple. This article will introduce how to use a third-party library to implement the verification code function in the Gin framework, and provide sample code for readers' reference. 1. Install dependent libraries Before using the verification code, we need to install a third-party library goCaptcha. To install goCaptcha, you can use the goget command: $goget-ugithub
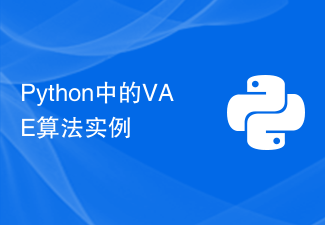
VAE is a generative model, its full name is VariationalAutoencoder, which is translated into Chinese as variational autoencoder. It is an unsupervised learning algorithm that can be used to generate new data, such as images, audio, text, etc. Compared with ordinary autoencoders, VAEs are more flexible and powerful and can generate more complex and realistic data. Python is one of the most widely used programming languages and one of the main tools for deep learning. In Python, there are many excellent machine learning and deep
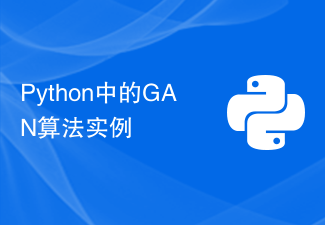
Generative Adversarial Networks (GAN) is a deep learning algorithm that uses two neural networks to compete with each other to generate new data. GAN is widely used for generation tasks in image, audio, text and other fields. In this article, we will use Python to write an example of a GAN algorithm for generating images of handwritten digits. Dataset Preparation We will use the MNIST data set as our training data set. The MNIST data set contains
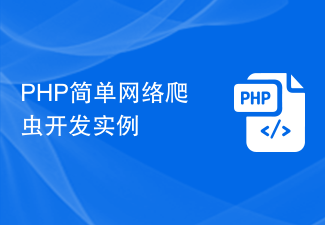
With the rapid development of the Internet, data has become one of the most important resources in today's information age. As a technology that automatically obtains and processes network data, web crawlers are attracting more and more attention and application. This article will introduce how to use PHP to develop a simple web crawler and realize the function of automatically obtaining network data. 1. Overview of Web Crawler Web crawler is a technology that automatically obtains and processes network resources. Its main working process is to simulate browser behavior, automatically access specified URL addresses and extract all information.
