


Summarize what you need to pay attention to when coding in Python
1. map, filter, reduce
1) map(func, input_list)
Apply the function to each element on the input list, such as:
input_list = [1, 2 , 3, 4, 5]
def pow_elem(x):
"""
Expand x to the power
:param x:
:return:
"""
return x * x
def multi_x_y(x, y):
return x * y
print map(pow_elem, input_list) # output:[1, 4, 9, 16, 25]
print map(multi_x_y, input_list, input_list) # output:[1, 4, 9, 16, 25]
2) filter(func_or_none, sequence)
Filter out the values in the sequence that satisfy the function return True, and form a new sequence return, such as:
def is_odd(x):
"""
Determine whether x is an odd number
:param x:
:return:
"""
return True if x % 2 > 0 else False
print filter(is_odd, input_list ) # output: [1, 3, 5]
3) reduce(function, sequence)
reduce() function receives parameters similar to map(), a function f and a list, but the behavior Unlike map(), the function f passed to reduce() must receive two parameters. reduce() repeatedly calls function f for each element of the list and returns the final result value. For example: reduce(lambda x, y: x+y, [1, 2, 3, 4, 5]) is equivalent to ((((1+2)+3)+4)+5)
print reduce (lambda x, y: x * y, input_list) # output: 120
2. Ternary operation
The following two ways of writing are equivalent:
"Yes" if 2==2 else " No"
("No", "Yes")[2==2]
That is:
1) condition_is_true if condition else condition_is_false
2) (if_test_is_false, if_test_is_true)[test]## Both #1) and 2) can implement ternary operations, but 2) is rarer and less elegant. At the same time, 2) is not a short-circuit operation, as follows:
5 if True else 5/0 # output: 5
(1/0, 5)[True] # throw exception-> ZeroDivisionError: integer division or modulo by zero
1) In Python, we can define it inside the function function and call it, such as:
def hi(name="patty"):
print("now you are inside the hi() function")
return "now you are in the greet() function"
return "now you are in the welcome() function"
print(welcome())
print("now you are back in the hi() function")
The output result is:
now you are inside the hi() function
now you are in the greet() function
now you are in the welcome() function
now you are back in the hi() function
def hi(name="patty"):
def greet():
return "now you are in the greet() function"
return "now you are in the welcome() function"
print hi() # < ;function greet at 0x109379a28>
print hi()() # now you are in the greet() function
In the above code, the hi() call returns a function object, which can be obtained from the if/else statement It is judged that what is returned is the greet() function. When we call hi()(), we actually call the internal function greet().
def hi():
return "hi patty!"
print("I am doing some boring work before executing hi()")
print(func())
Output result:
I am doing some boring work before executing hi()
hi patty!
So far, we have implemented a simple decorator, which outputs a line before calling the hi() function. In actual applications, it may be some preprocessing operations. In fact, the function of the decorator is to add some common functions before and after your core logic is executed.
def a_new_decorator(a_func):
print("I am doing some boring work before executing a_func() ")
print("I am the function which needs some decoration to remove my foul smell")
#outputs: "I am the function which needs some decoration decoration to remove my foul smell"
#now a_function_requiring_decoration is wrapped by wrapTheFunction()
a_function_requiring_decoration()
# I am doing some boring work before executing a_func()
# I am the function which needs some decoration to remove my foul smell
# I am doing some boring work after executing a_func()
5) Annotation form
@a_new_decorator
def b_function_requiring_decoration():
print("I am the another function which needs some decoration to remove my foul smell")
b_function_requiring_decoration()
# I am doing some boring work before executing a_func()
# I am the another function which needs some decoration to remove my foul smell
# I am doing some boring work after executing a_func()
Here @a_new_decorator is equivalent to a_new_decorator(b_function_requiring_decoration)
6) Get name
For a_function_requiring_decoration in 4), we print print(a_function_requiring_decoration.__name__) and get The result is wrapTheFunction, but actually what we want to get is the a_function_requiring_decoration function name corresponding to a_func. Python provides us with wraps to solve this problem.
from functools import wraps
def a_new_decorator(a_func):
@wraps(a_func)
def wrapTheFunction():
print("I am doing some boring work before executing a_func()" )
a_func()
print("I am doing some boring work after executing a_func()")
return wrapTheFunction
7) Decorator Some application scenarios
User authentication
def requires_auth(f):
@wraps(f)
def decorated(*args, **kwargs):
auth = {"username": "patty", "password": "123456"}
if not check_auth(auth['username'], auth['password']):
authenticate()
return f(*args, * *kwargs)
def check_auth(username, password):
print "Starting check auth..."
return True if (username == 'patty' and password == '123456') else False
def authenticate():
print "Already authenticate"
return decorated
@requires_auth
def welcome():
return " Welcome patty!"
print welcome()
logging
def logit(func):
@wraps(func)
def with_logging(*args, ** kwargs):
print(func.__name__ + " was called")
return func(*args, **kwargs)
return with_logging
@logit
def addition_func(x ):
"""Do some math."""
return x + x
result = addition_func(4)
will print: addition_func was called
8) Decorator with parameters
from functools import wraps
def logit(logfile='out.log'):
def logging_decorator(func):
@wraps(func )
def wrapped_function(*args, **kwargs):
log_string = func.__name__ + " was called"
print(log_string)
Open the logfile and append
with open( logfile, 'a') as opend_file:
#Now we log to the specify logfile
Return wrapped_function
RETURN LOGGING_DECORTOR
## @logit()
pass
myfunc1()
# A file called out.log now exists, with the above string
@logit(logfile='func2.log')
pass
myfunc2()
9) Use the class as Decorator
import os
class Logit(object):
self.log_file = log_file
def __call__(self, func):
with open(self.log_file, 'a') as fout:
log_msg = func.__name__ + " was called"
fout.write(log_msg)
fout.write(os.linesep)
self.notify()
def notify(self):
class EmailLogit(Logit):
'''
A logit implementation for sending emails to admins
when the function is called.
'''
def __init__(self, log_file , email='admin@myproject.com'):
self.email = email
super(EmailLogit, self).__init__(log_file)
def notify(self):
# Send an email to self.email
#Will not be Implemented here
completely Open (Self.log_file, 'A) as f:
F.write ("do something ...")
# ("log1.txt")
def myfunc3():
pass
@EmailLogit("log2.txt")
def myfunc4():
pass
Use Using classes as decorators makes our code look more concise, and it can also achieve personalization and reuse of functions through inheritance.
4. Variable types
Variable types in Python include lists and dictionaries. The elements in these objects are changeable, such as
>>> foo = ['hi ']
>>> foo += ['patty']
['hi', 'patty']
>>> foo[0]='hello'
>>> foo
['hello', 'patty']
>>> fdict = {"name":" patty"}
>>> fdict.update({"age":"23"})
>>> fdict
{'age': '23', 'name ': 'patty'}
>>> fdict
{'age': '25' , 'name': 'patty'}
In the method, if the incoming parameter adopts a variable type and is assigned a default value, please note that the following situations will occur:
>>> def add_to(num, target=[]):
... target.append(num)
... return target
>>> add_to(1)
[1]
>>> add_to(2)
[1, 2]
>>> add_to(3)
[1, 2, 3]
This is because the default parameters are calculated when the method is defined, rather than calculated again each time it is called. Therefore, in order to avoid the above situation, when we expect to calculate with a new empty list every time the method is called, we can write it as follows:
>>> def add_to(num, target= None):
... if target is None:
... target = []
... target.append(num)
... return target
...
>>> add_to(1)
[1]
>>> add_to(2)
[2]
5. Shallow copy and deep copy Copy
In Python, there are differences between object assignment and copy (deep/shallow copy). If you don’t pay attention when using it, unexpected results may occur.
1) The default in Python is shallow copying
>>> foo = ['hi']
>>> id (foo)
4458211232
>>> id(bar)
4458211232
>>> bar.append("patty")
>>> bar
['hi', 'patty']
>>> foo
['hi', 'patty']
Note: id(foo)==id(bar) , indicating that foo and bar refer to the same object. When the append operation is performed on the list through the bar reference, the output of foo is consistent with that of bar because it points to the same memory space.
2) Deep copy
>>> foo
['hi', {'age': 20, 'name': 'patty'}]
>> ;> import copy
>>> slow
['hi', {'age': 20, 'name' : 'patty'}]
>>> slow[0]='hello'
>>> slow
['hello', {'age': 20, 'name ': 'patty'}]
>>> foo
['hi', {'age': 20, 'name': 'patty'}]
Note: Since slow is correct The deep copy of foo actually opens a new space in the memory and copies the content referenced by the foo object to the new memory space. Therefore, when the content referenced by the slow object is updated, the changes are only reflected On the reference of the slow object, the content referenced by the foo object has not changed.
6. Collection
1) defaultdict
For ordinary dict, if you obtain a non-existent key, a KeyError error will be triggered, as follows:
some_dict = {}
some_dict['colours' ]['favourite'] = "yellow"
# Raises KeyError: 'colours'
But through defaultdict, we can avoid this situation, as follows:
import collections
import json
tree = lambda: collections.defaultdict(tree)
some_dict = tree()
some_dict['colours']['favourite'] = "yellow"
print json.dumps(some_dict)
# Works fine, output: {"colours": {"favourite": "yellow"}}
2) OrderedDict
OrderedDict can print out the dictionary in the key order when we define the dictionary and change the value. The values will not change the order of the keys. However, after the keys are deleted and reinserted, the keys will be reordered to the end of the dict.
from collections import OrderedDict
colours = OrderedDict([("Red", 198), ("Green", 170), ("Blue", 160)])
for key, value in colours.items():
print(key, value)
3) Counter
Use Counter to count the number of occurrences of specific items, such as:
from collections import Counter
colours = (
('Yasoob', 'Yellow'),
('Ali', 'Blue'),
('Arham', 'Green'),
( 'Ali', 'Black'),
('Yasoob', 'Red'),
('Ahmed', 'Silver'),
)
favs = Counter(name for name, color in colours)
print(favs)
# Counter({'Yasoob': 2, 'Ali': 2, 'Arham': 1, 'Ahmed': 1})
4) deque
deque is a double-ended queue, which can be inserted and deleted at the head and tail respectively, as follows:
from collections import deque
queue_d = deque()
queue_d.append( 1)
queue_d.append(2)
print queue_d # deque([1, 2])
queue_d.appendleft(3)
print queue_d # deque([3, 1, 2])
queue_d.pop()
print queue_d # deque([3, 1])
queue_d.popleft()
print queue_d # deque([1])
deque can set the maximum length of the queue. When the number of elements exceeds the maximum length, the corresponding number of elements will be deleted from the opposite direction of the current insertion direction, as follows:
queue_c = deque(maxlen=5, iterable=[2, 4, 6])
queue_c.extend([7, 8])
print queue_c # deque([2, 4, 6, 7, 8], maxlen=5)
queue_c.extend([ 10, 12])
print(queue_c) # deque([6, 7, 8, 10, 12], maxlen=5)
queue_c.extendleft([18])
print(queue_c) # deque([18, 6, 7, 8, 10], maxlen=5)
5) nametuple
tuple is an immutable list. The elements in the tuple cannot be reassigned. We can only Access the elements in the tuple through index. The nametuple can be regarded as an immutable dictionary, and the elements in the tuple can be accessed through name. For example:
from collections import namedtuple
Animal = namedtuple('Animal', 'name age type')
perry = Animal(name="perry", age=31, type="cat ")
print(perry)
# Output: Animal(name='perry', age=31, type='cat')
print(perry.name)
# Output: 'perry'
print(perry[0])
# Output: 'perry'
print(perry._asdict())
# Output: OrderedDict ([('name', 'perry'), ('age', 31), ('type', 'cat')])
7、Object introspection
1) dir: List the All methods of the object
2) type: returns the type of the object
3) id: returns the id of the object
1)list
>>> ; squared = [x**2 for x in range(10)]
>>> squared
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
2) dict
{v: k for k, v in some_dict.items()}
3) set
>>> squared = {x**2 for x in range (10)}
>>> squared
set([0, 1, 4, 81, 64, 9, 16, 49, 25, 36])
try:
print('I am sure no exception is going to occur!')
except Exception:
print('exception')
else:
# any code that should only run if no exception occurs in the try,
# but for which exceptions should NOT be caught
print('This would only run if no exception occurs. And an error here '
'would NOT be caught.')
finally:
print('This would be printed in every case.')
# This would only run if no exception occurs.
# This would be printed in every case.
The statements in else will be executed before finally.
a_list = [[1, 2], [3, 4], [5, 6]]
print(list(itertools.chain.from_iterable(a_list)) )
# Output: [1, 2, 3, 4, 5, 6]
print(list(itertools.chain(*a_list)))
# Output: [1, 2, 3, 4, 5, 6]
class A(object):
def __init__(self, a, b, c, d, e, f):
self.__dict__.update({k: v for k, v in locals().items() if k != 'self'})
11. for-else statement
There are two normal ways to end the for statement: one is when specific conditions are met The next break is to jump out of the loop, and the second is to end all conditional loops. The else statement in for-else will only be executed when all conditions have been judged and the for loop ends normally, as follows:
for x in range(1, 10, 2):
if x % 2 == 0:
print "found even of %d"%x
break
else:
print "not foud even"
# output: not foud even
12. Compatible with Python 2+ and Python 3+
1) Use the __future__ module to reference Python 3+ modules in the Python 2+ environment
2) Compatible module import method
try:
import urllib.request as urllib_request # for Python 3
except ImportError:
import urllib2 as urllib_request # for Python 2
Reference:
The above is the detailed content of Summarize what you need to pay attention to when coding in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


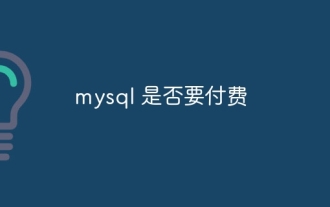
MySQL has a free community version and a paid enterprise version. The community version can be used and modified for free, but the support is limited and is suitable for applications with low stability requirements and strong technical capabilities. The Enterprise Edition provides comprehensive commercial support for applications that require a stable, reliable, high-performance database and willing to pay for support. Factors considered when choosing a version include application criticality, budgeting, and technical skills. There is no perfect option, only the most suitable option, and you need to choose carefully according to the specific situation.
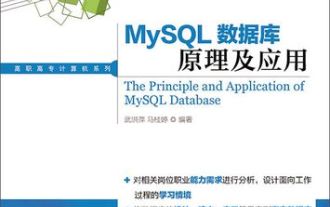
The article introduces the operation of MySQL database. First, you need to install a MySQL client, such as MySQLWorkbench or command line client. 1. Use the mysql-uroot-p command to connect to the server and log in with the root account password; 2. Use CREATEDATABASE to create a database, and USE select a database; 3. Use CREATETABLE to create a table, define fields and data types; 4. Use INSERTINTO to insert data, query data, update data by UPDATE, and delete data by DELETE. Only by mastering these steps, learning to deal with common problems and optimizing database performance can you use MySQL efficiently.
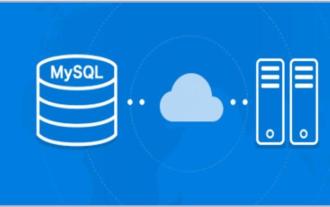
The main reasons for MySQL installation failure are: 1. Permission issues, you need to run as an administrator or use the sudo command; 2. Dependencies are missing, and you need to install relevant development packages; 3. Port conflicts, you need to close the program that occupies port 3306 or modify the configuration file; 4. The installation package is corrupt, you need to download and verify the integrity; 5. The environment variable is incorrectly configured, and the environment variables must be correctly configured according to the operating system. Solve these problems and carefully check each step to successfully install MySQL.
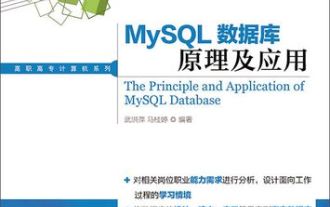
MySQL download file is corrupt, what should I do? Alas, if you download MySQL, you can encounter file corruption. It’s really not easy these days! This article will talk about how to solve this problem so that everyone can avoid detours. After reading it, you can not only repair the damaged MySQL installation package, but also have a deeper understanding of the download and installation process to avoid getting stuck in the future. Let’s first talk about why downloading files is damaged. There are many reasons for this. Network problems are the culprit. Interruption in the download process and instability in the network may lead to file corruption. There is also the problem with the download source itself. The server file itself is broken, and of course it is also broken when you download it. In addition, excessive "passionate" scanning of some antivirus software may also cause file corruption. Diagnostic problem: Determine if the file is really corrupt
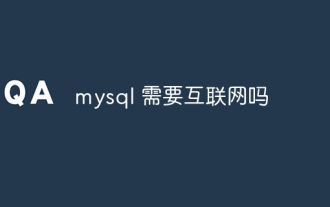
MySQL can run without network connections for basic data storage and management. However, network connection is required for interaction with other systems, remote access, or using advanced features such as replication and clustering. Additionally, security measures (such as firewalls), performance optimization (choose the right network connection), and data backup are critical to connecting to the Internet.
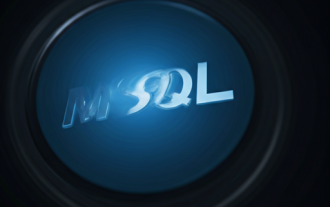
MySQL database performance optimization guide In resource-intensive applications, MySQL database plays a crucial role and is responsible for managing massive transactions. However, as the scale of application expands, database performance bottlenecks often become a constraint. This article will explore a series of effective MySQL performance optimization strategies to ensure that your application remains efficient and responsive under high loads. We will combine actual cases to explain in-depth key technologies such as indexing, query optimization, database design and caching. 1. Database architecture design and optimized database architecture is the cornerstone of MySQL performance optimization. Here are some core principles: Selecting the right data type and selecting the smallest data type that meets the needs can not only save storage space, but also improve data processing speed.
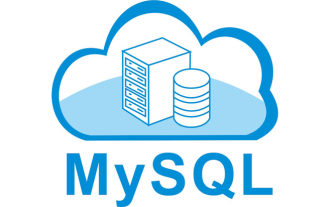
MySQL refused to start? Don’t panic, let’s check it out! Many friends found that the service could not be started after installing MySQL, and they were so anxious! Don’t worry, this article will take you to deal with it calmly and find out the mastermind behind it! After reading it, you can not only solve this problem, but also improve your understanding of MySQL services and your ideas for troubleshooting problems, and become a more powerful database administrator! The MySQL service failed to start, and there are many reasons, ranging from simple configuration errors to complex system problems. Let’s start with the most common aspects. Basic knowledge: A brief description of the service startup process MySQL service startup. Simply put, the operating system loads MySQL-related files and then starts the MySQL daemon. This involves configuration
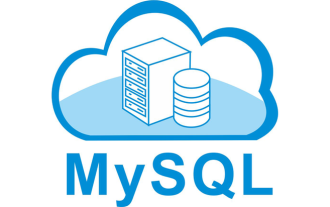
MySQL performance optimization needs to start from three aspects: installation configuration, indexing and query optimization, monitoring and tuning. 1. After installation, you need to adjust the my.cnf file according to the server configuration, such as the innodb_buffer_pool_size parameter, and close query_cache_size; 2. Create a suitable index to avoid excessive indexes, and optimize query statements, such as using the EXPLAIN command to analyze the execution plan; 3. Use MySQL's own monitoring tool (SHOWPROCESSLIST, SHOWSTATUS) to monitor the database health, and regularly back up and organize the database. Only by continuously optimizing these steps can the performance of MySQL database be improved.
