Django introduction to paging examples
The paging function is necessary in every website. For paging, it is actually to calculate the starting position of the data that should be displayed on the page in the database table based on the user's input.
Determine the paging requirements:
1. 每页显示的数据条数 2. 每页显示页号链接数 3. 上一页和下一页 4. 首页和末页
Rendering:
First, use the built-in paging function of django, Write the paging class:
1 from django.core.paginator import Paginator, Page # 导入django分页模块 2 3 4 class PageInfo(object): 5 def __init__(self, current_page, all_count, base_url, per_page=10, show_page=11): 6 """ 7 8 :param current_page: 当前页 9 :param all_count: 总页数10 :param base_url: 模板11 :param per_page: 每页显示数据条数12 :param show_page: 显示链接页个数13 """14 #若url错误,默认显示第一页(错误类型可能为:空页面编号,非整数型页面编号)15 try:16 self.current_page = int(current_page)17 except Exception as e:18 self.current_page = 119 20 #根据数据库信息条数得出总页数 21 a, b = divmod(all_count, per_page)22 if b:23 a += 124 self.all_page = a 25 26 self.base_url = base_url27 self.per_page = per_page28 self.show_page = show_page29 30 #当前页起始数据id31 def start_data(self): 32 return (self.current_page - 1) * self.per_page33 34 #当前页结束数据id35 def end_data(self): 36 return self.current_page * self.per_page37 38 #动态生成前端html39 def pager(self):40 page_list = []41 half = int((self.show_page - 1)/2)42 #如果:总页数 < show_page,默认显示页数范围为: 1~总页数43 if self.all_page < self.show_page:44 start_page = 145 end_page = self.all_page + 146 #如果:总页数 > show_page47 else:48 #如果:current_page - half <= 0,默认显示页数范围为:1~show_page49 if self.current_page <= half:50 start_page = 151 end_page = self.show_page + 152 else:53 #如果:current_page + half >总页数,默认显示页数范围为:总页数 - show_page ~ 总页数54 if self.current_page + half > self.all_page:55 end_page = self.all_page + 156 start_page = end_page - self.show_page57 else:58 start_page = self.current_page - half59 end_page = self.current_page + half + 160 61 #首页62 first_page = "<li><a href='%s?page=%s'>首页</a></li>" %(self.base_url, 1)63 page_list.append(first_page)64 65 #上一页(若当前页等于第一页,则上一页无链接,否则链接为当前页减1)66 if self.current_page <= 1:67 prev_page = "<li><a href='#'>上一页</a></li>"68 else:69 prev_page = "<li><a href='%s?page=%s'>上一页</a></li>" %(self.base_url, self.current_page-1)70 page_list.append(prev_page)71 72 #动态生成中间页数链接73 for i in range(start_page, end_page):74 if i == self.current_page:75 temp = "<li class='active'><a href='%s?page=%s'>%s</a></li>" %(self.base_url, i, i)76 else:77 temp = "<li><a href='%s?page=%s'>%s</a></li>" % (self.base_url, i, i)78 page_list.append(temp)79 80 #下一页(若当前页等于最后页,则下一页无链接,否则链接为当前页加1)81 if self.current_page >= self.all_page:82 next_page = "<li><a href='#'>下一页</a></li>"83 else:84 next_page = "<li><a href='%s?page=%s'>下一页</a></li>" %(self.base_url, self.current_page+1)85 page_list.append(next_page)86 87 #末页(若总页数只有一页,则无末页标签)88 if self.all_page > 1:89 last_page = "<li><a href='%s?page=%s'>末页</a></li>" % (self.base_url, self.all_page)90 page_list.append(last_page)91 92 return ''.join(page_list)
Then, write the method in views (written here in app01):
1 from utils.pagnition import PageInfo # 从文件中导入上步自定义的分页模块2 3 def custom(request):4 all_count = models.UserInfo.objects.all().count() # 获取要显示数据库的总数据条数5 page_info = PageInfo(request.GET.get('page'), all_count, '/custom.html/',) # 生成分页对象6 user_list = models.UserInfo.objects.all()[page_info.start_data():page_info.end_data()] # 利用分页对象获取当前页显示数据7 return render(request, 'custom.html', {'user_list': user_list, 'page_info': page_info}) # 模板渲染
Then, in templates Write the "custom.html" file in the directory:
1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>customers</title> 6 {# 引入bootstrap样式#} 7 <link rel="stylesheet" href="/static/plugins/bootstrap-3.3.7-dist/css/bootstrap.css?1.1.11"> 8 </head> 9 <body>10 <h1>customers</h1>11 {#当前页显示的数据#}12 <ul>13 {% for row in user_list %}14 <li>{{ row.name }}</li>15 {% endfor %}16 </ul>17 18 {#分页#}19 <nav aria-label="Page navigation">20 <ul class="pagination">21 {# 传入page_info.pager#}22 {{ page_info.pager|safe }}23 </ul>24 </nav>25 26 </body>27 </html>
Finally, add the url relationship (urls.py):
1 from django.conf.urls import url2 from django.contrib import admin3 from app01 import views as app01_views4 5 urlpatterns = [6 url(r'^custom.html/$', app01_views.custom),7 ]
At this point, This completes the custom paging module using Django's paging function, which can be applied to different business pages.
Reference materials:
1. The Road to Python [Part 17]: Django [Advanced Chapter]
The above is the detailed content of Django introduction to paging examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
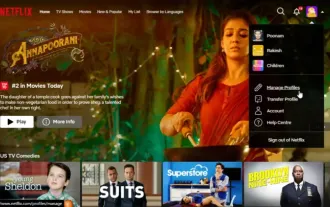
An avatar on Netflix is a visual representation of your streaming identity. Users can go beyond the default avatar to express their personality. Continue reading this article to learn how to set a custom profile picture in the Netflix app. How to quickly set a custom avatar in Netflix In Netflix, there is no built-in feature to set a profile picture. However, you can do this by installing the Netflix extension on your browser. First, install a custom profile picture for the Netflix extension on your browser. You can buy it in the Chrome store. After installing the extension, open Netflix on your browser and log into your account. Navigate to your profile in the upper right corner and click
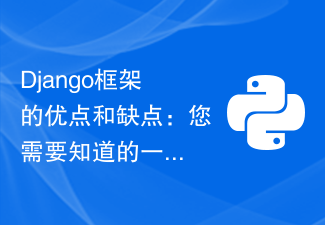
Django is a complete development framework that covers all aspects of the web development life cycle. Currently, this framework is one of the most popular web frameworks worldwide. If you plan to use Django to build your own web applications, then you need to understand the advantages and disadvantages of the Django framework. Here's everything you need to know, including specific code examples. Django advantages: 1. Rapid development-Djang can quickly develop web applications. It provides a rich library and internal
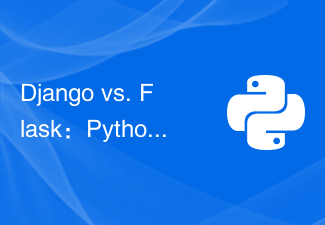
Django and Flask are both leaders in Python Web frameworks, and they both have their own advantages and applicable scenarios. This article will conduct a comparative analysis of these two frameworks and provide specific code examples. Development Introduction Django is a full-featured Web framework, its main purpose is to quickly develop complex Web applications. Django provides many built-in functions, such as ORM (Object Relational Mapping), forms, authentication, management backend, etc. These features allow Django to handle large
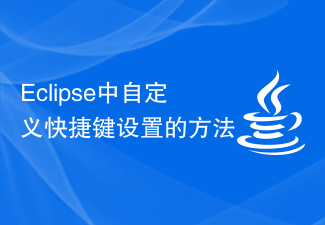
How to customize shortcut key settings in Eclipse? As a developer, mastering shortcut keys is one of the keys to improving efficiency when coding in Eclipse. As a powerful integrated development environment, Eclipse not only provides many default shortcut keys, but also allows users to customize them according to their own preferences. This article will introduce how to customize shortcut key settings in Eclipse and give specific code examples. Open Eclipse First, open Eclipse and enter
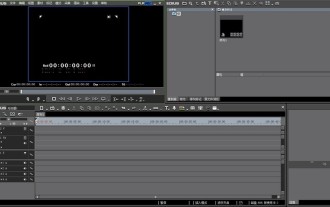
1. The picture below is the default screen layout of edius. The default EDIUS window layout is a horizontal layout. Therefore, in a single-monitor environment, many windows overlap and the preview window is in single-window mode. 2. You can enable [Dual Window Mode] through the [View] menu bar to make the preview window display the playback window and recording window at the same time. 3. You can restore the default screen layout through [View menu bar>Window Layout>General]. In addition, you can also customize the layout that suits you and save it as a commonly used screen layout: drag the window to a layout that suits you, then click [View > Window Layout > Save Current Layout > New], and in the pop-up [Save Current Layout] Layout] enter the layout name in the small window and click OK
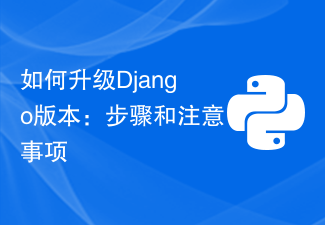
How to upgrade Django version: steps and considerations, specific code examples required Introduction: Django is a powerful Python Web framework that is continuously updated and upgraded to provide better performance and more features. However, for developers using older versions of Django, upgrading Django may face some challenges. This article will introduce the steps and precautions on how to upgrade the Django version, and provide specific code examples. 1. Back up project files before upgrading Djan
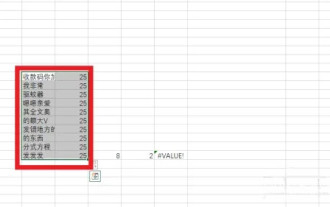
In an excel table, sometimes you may need to insert coordinate axes to see the changing trend of the data more intuitively. Some friends still don’t know how to insert coordinate axes in the table. Next, I will share with you how to customize the coordinate axis scale in Excel. Coordinate axis insertion method: 1. In the excel interface, select the data. 2. In the insertion interface, click to insert a column chart or bar chart. 3. In the expanded interface, select the graphic type. 4. In the right-click interface of the table, click Select Data. 5. In the expanded interface, you can customize it.
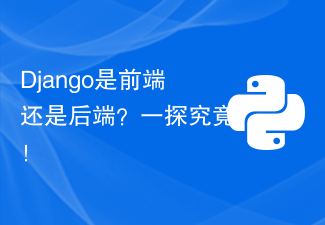
Django is a web application framework written in Python that emphasizes rapid development and clean methods. Although Django is a web framework, to answer the question whether Django is a front-end or a back-end, you need to have a deep understanding of the concepts of front-end and back-end. The front end refers to the interface that users directly interact with, and the back end refers to server-side programs. They interact with data through the HTTP protocol. When the front-end and back-end are separated, the front-end and back-end programs can be developed independently to implement business logic and interactive effects respectively, and data exchange.
