Detailed explanation of obtaining objects through Class class
The way to obtain an object through the Class object is to obtain it through class.newInstance(), and instantiate an object by calling the default construction parameters.
1 /** 2 * Created by hunt on 2017/6/27. * 测试的实体类 4 * @Data 编译后会自动生成set、get、无惨构造、equals、canEqual、hashCode、toString方法 5 */ 6 @Data 7 public class Person { 8 private String name; 9 private int age;10 }
1 /** 2 * Created by hunt on 2017/6/27. 3 */ 4 public class NewInstanceTest { 5 public static void main(String[] args) { 6 Class<Person> personClass = Person.class;//获取Class实例 7 try { 8 Person p = personClass.newInstance();//通过Class获得Person实例 9 p.setAge(28);10 p.setName("hunt");11 System.out.println(p);12 } catch (InstantiationException e) {13 e.printStackTrace();14 } catch (IllegalAccessException e) {15 e.printStackTrace();16 }17 }18 }
Tip: class.newInstance() is instantiated through a parameterless constructor. An object has a parameterless constructor by default. Parametric constructor, if there is a parameterized constructor, the parameterless constructor does not exist, and a java.lang.InstantiationException exception will be thrown when the object is obtained through reflection.
1 /** 2 * Created by hunt on 2017/6/27. 3 * 测试的实体类 4 */ 5 6 public class Person { 7 private String name; 8 private int age; 9 10 public String getName() {11 return name;12 }13 14 public int getAge() {15 return age;16 }17 18 public void setName(String name) {19 this.name = name;20 }21 22 public void setAge(int age) {23 this.age = age;24 }25 26 public Person(String name,int age){}//有参数构造函数27 }
1 /** 2 * Created by hunt on 2017/6/27. 3 */ 4 public class NewInstanceTest { 5 public static void main(String[] args) { 6 Class<Person> personClass = Person.class;//获取Class实例 7 try { 8 Person p = personClass.newInstance();//通过Class获得Person实例 9 p.setAge(28);10 p.setName("hunt");11 System.out.println(p.getAge()+"----"+p.getName());12 } catch (InstantiationException e) {13 e.printStackTrace();14 } catch (IllegalAccessException e) {15 e.printStackTrace();16 } } }
Summary: When creating entity classes in the future, you must bring a no-argument constructor so that you can use reflection in the future. No exception is thrown when instantiating the object.
The above is the detailed content of Detailed explanation of obtaining objects through Class class. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


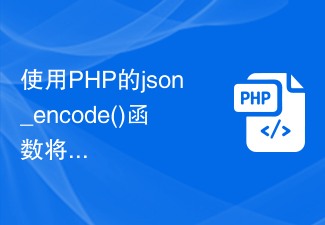
JSON (JavaScriptObjectNotation) is a lightweight data exchange format that has become a common format for data exchange between web applications. PHP's json_encode() function can convert an array or object into a JSON string. This article will introduce how to use PHP's json_encode() function, including syntax, parameters, return values, and specific examples. Syntax The syntax of the json_encode() function is as follows: st
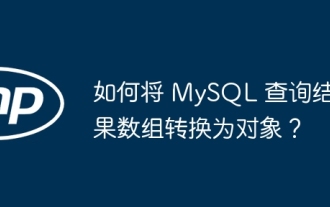
Here's how to convert a MySQL query result array into an object: Create an empty object array. Loop through the resulting array and create a new object for each row. Use a foreach loop to assign the key-value pairs of each row to the corresponding properties of the new object. Adds a new object to the object array. Close the database connection.
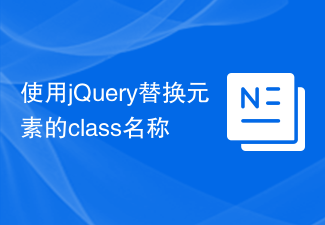
jQuery is a classic JavaScript library that is widely used in web development. It simplifies operations such as handling events, manipulating DOM elements, and performing animations on web pages. When using jQuery, you often encounter situations where you need to replace the class name of an element. This article will introduce some practical methods and specific code examples. 1. Use the removeClass() and addClass() methods jQuery provides the removeClass() method for deletion
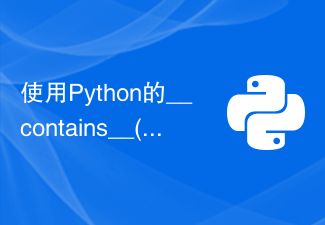
Use Python's __contains__() function to define the containment operation of an object. Python is a concise and powerful programming language that provides many powerful features to handle various types of data. One of them is to implement the containment operation of objects by defining the __contains__() function. This article will introduce how to use the __contains__() function to define the containment operation of an object, and give some sample code. The __contains__() function is Pytho
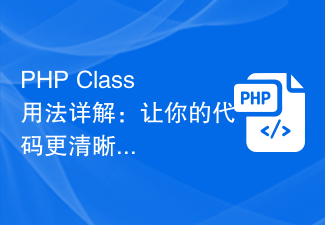
When writing PHP code, using classes is a very common practice. By using classes, we can encapsulate related functions and data in a single unit, making the code clearer, easier to read, and easier to maintain. This article will introduce the usage of PHPClass in detail and provide specific code examples to help readers better understand how to apply classes to optimize code in actual projects. 1. Create and use classes In PHP, you can use the keyword class to define a class and define properties and methods in the class.
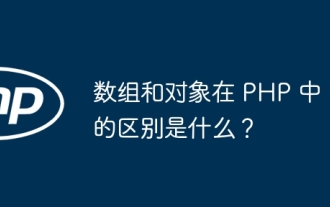
In PHP, an array is an ordered sequence, and elements are accessed by index; an object is an entity with properties and methods, created through the new keyword. Array access is via index, object access is via properties/methods. Array values are passed and object references are passed.
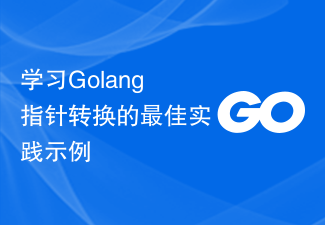
Golang is a powerful and efficient programming language that can be used to develop various applications and services. In Golang, pointers are a very important concept, which can help us operate data more flexibly and efficiently. Pointer conversion refers to the process of pointer operations between different types. This article will use specific examples to learn the best practices of pointer conversion in Golang. 1. Basic concepts In Golang, each variable has an address, and the address is the location of the variable in memory.
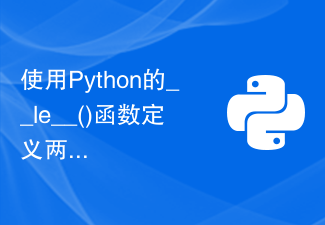
Title: Using Python's __le__() function to define a less than or equal comparison of two objects In Python, we can define comparison operations between objects by using special methods. One of them is the __le__() function, which is used to define less than or equal comparisons. The __le__() function is a magic method in Python and is a special function used to implement the "less than or equal" operation. When we compare two objects using the less than or equal operator (<=), Python
