


Detailed explanation of JavaScript queue function and asynchronous_javascript skills
This article mainly introduces the relevant information of JavaScriptqueue function and asynchronous execution in detail. It has certain reference value. Interested friends can refer to it
Edit Note: I saw a similar queue function when reviewing other people's JavaScript code, but I didn't understand it. It turns out that this is to ensure that the functions are called in order. After reading this article, I found that it can also be used for asynchronous execution, etc.
Suppose you have several functions fn1, fn2 and fn3 that need to be called in sequence. The simplest way is of course:
fn1(); fn2(); fn3();
But sometimes these functions are added one by one during runtime. When calling, you don’t know what functions there are; at this time, you can pre-define an array, push the functions into it when adding functions, and take them out from the array one by one in order when needed, and call them in sequence:
var stack = []; // 执行其他操作,定义fn1 stack.push(fn1); // 执行其他操作,定义fn2、fn3 stack.push(fn2, fn3); // 调用的时候 stack.forEach(function(fn) { fn() });
It doesn’t matter whether the function has a name or not. You can just pass in the anonymous function directly. Let’s test it:
var stack = []; function fn1() { console.log('第一个调用'); } stack.push(fn1); function fn2() { console.log('第二个调用'); } stack.push(fn2, function() { console.log('第三个调用') }); stack.forEach(function(fn) { fn() }); // 按顺序输出'第一个调用'、'第二个调用'、'第三个调用'
This implementation works fine so far, but we have ignored one situation, which is the call of asynchronous functions. Asynchrony is an unavoidable topic in JavaScript. I am not going to discuss the various terms and concepts related to asynchronous in JavaScript here. Readers are asked to check it out by themselves (such as a famous commentary). If you know that the following code will output 1, 3, and 2, then please continue reading:
console.log(1); setTimeout(function() { console.log(2); }, 0); console.log(3);
If there is a function in the stack queue that is a similar asynchronous function, our implementation will be messed up:
var stack = []; function fn1() { console.log('第一个调用') }; stack.push(fn1); function fn2() { setTimeout(function fn2Timeout() { console.log('第二个调用'); }, 0); } stack.push(fn2, function() { console.log('第三个调用') }); stack.forEach(function(fn) { fn() }); // 输出'第一个调用'、'第三个调用'、'第二个调用'
The problem is obvious, fn2 is indeed called in sequence, but the function fn2Timeout() { console.log('second call') } in setTimeout is not executed immediately (even if timeout is set to 0) ; Return immediately after fn2 is called, and then execute fn3. After fn3 is executed, it is really fn2Timeout's turn.
How to deal with it? After our analysis, the key here is fn2Timeout. We must wait until it is actually executed before calling fn3. Ideally, it looks like this:
function fn2() { setTimeout(function() { fn2Timeout(); fn3(); }, 0); }
But doing so is equivalent to removing the original fn2Timeout and replacing it with one. New function, then insert the original fn2Timeout and fn3. This method of dynamically changing the original function has a special term called Monkey Patch. According to the mantra of our programmers: "It can definitely be done", but it is a bit awkward to write, and it is easy to get yourself involved. Is there a better way?
We take a step back and do not insist on waiting for fn2Timeout to be completely executed before executing fn3. Instead, we call it on the last line of the fn2Timeout function body:
function fn2() { setTimeout(function fn2Timeout() { console.log('第二个调用'); fn3(); // 注{1} }, 0); }
This looks better, but when defining fn2 There is no fn3 yet, where did this fn3 come from?
There is another problem. Since fn3 needs to be called in fn2, we cannot call fn3 through stack.forEach, otherwise fn3 will be called twice.
We cannot hardcode fn3 into fn2. On the contrary, we only need to find the next function of fn2 in the stack at the end of fn2Timeout, and then call:
function fn2() { setTimeout(function fn2Timeout() { console.log('第二个调用'); next(); }, 0); }
This next function is responsible for finding the next function in the stack and executing it. Let’s implement next now:
var index = 0; function next() { var fn = stack[index]; index = index + 1; // 其实也可以用shift 把fn 拿出来 if (typeof fn === 'function') fn(); }
next uses stack[index] to get the function in the stack. Every time next is called, the index will be incremented by 1, so as to achieve the purpose of fetching the next function.
Next is used like this:
var stack = []; // 定义index 和next function fn1() { console.log('第一个调用'); next(); // stack 中每一个函数都必须调用`next` }; stack.push(fn1); function fn2() { setTimeout(function fn2Timeout() { console.log('第二个调用'); next(); // 调用`next` }, 0); } stack.push(fn2, function() { console.log('第三个调用'); next(); // 最后一个可以不调用,调用也没用。 }); next(); // 调用next,最终按顺序输出'第一个调用'、'第二个调用'、'第三个调用'。
Now that the stack.forEach line has been deleted, we call next by ourselves. Next will find the first function fn1 in the stack to execute, and call next in fn1. Go Find the next function fn2 and execute it, then call next in fn2, and so on.
Every function must call next. If it is not written in a certain function, the program will end directly after executing the function without any mechanism to continue.
After understanding this implementation of function queue, you should be able to solve the following interview question:
// 实现一个LazyMan,可以按照以下方式调用: LazyMan(“Hank”) /* 输出: Hi! This is Hank! */ LazyMan(“Hank”).sleep(10).eat(“dinner”)输出 /* 输出: Hi! This is Hank! // 等待10秒.. Wake up after 10 Eat dinner~ */ LazyMan(“Hank”).eat(“dinner”).eat(“supper”) /* 输出: Hi This is Hank! Eat dinner~ Eat supper~ */ LazyMan(“Hank”).sleepFirst(5).eat(“supper”) /* 等待5秒,输出 Wake up after 5 Hi This is Hank! Eat supper */ // 以此类推。
Node.js The famous connect framework This is how middlewarequeue is implemented. If you are interested, you can take a look at its source code or this interpretation of "What is connect middleware".
If you are careful, you may see that this next can only be placed at the end of the function for the time being. If it is placed in the middle, the original problem will still occur:
function fn() { console.log(1); next(); console.log(2); // next()如果调用了异步函数,console.log(2)就会先执行 }
redux and koa pass Different implementations can put next in the middle of the function, and then turn back and execute the code below next after executing the subsequent functions. This is very clever. Write again when you have time.
The above is the detailed content of Detailed explanation of JavaScript queue function and asynchronous_javascript skills. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


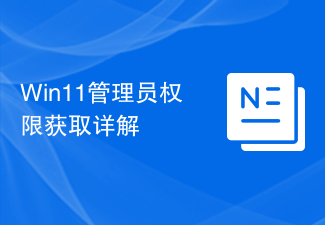
Windows operating system is one of the most popular operating systems in the world, and its new version Win11 has attracted much attention. In the Win11 system, obtaining administrator rights is an important operation. Administrator rights allow users to perform more operations and settings on the system. This article will introduce in detail how to obtain administrator permissions in Win11 system and how to effectively manage permissions. In the Win11 system, administrator rights are divided into two types: local administrator and domain administrator. A local administrator has full administrative rights to the local computer
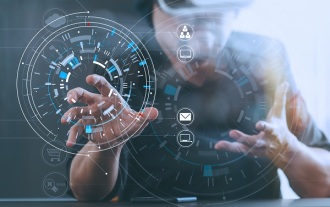
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
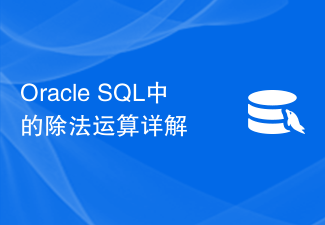
Detailed explanation of division operation in OracleSQL In OracleSQL, division operation is a common and important mathematical operation, used to calculate the result of dividing two numbers. Division is often used in database queries, so understanding the division operation and its usage in OracleSQL is one of the essential skills for database developers. This article will discuss the relevant knowledge of division operations in OracleSQL in detail and provide specific code examples for readers' reference. 1. Division operation in OracleSQL
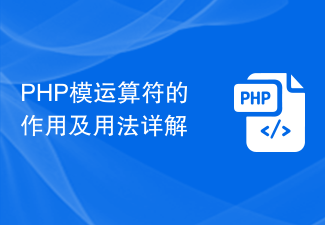
The modulo operator (%) in PHP is used to obtain the remainder of the division of two numbers. In this article, we will discuss the role and usage of the modulo operator in detail, and provide specific code examples to help readers better understand. 1. The role of the modulo operator In mathematics, when we divide an integer by another integer, we get a quotient and a remainder. For example, when we divide 10 by 3, the quotient is 3 and the remainder is 1. The modulo operator is used to obtain this remainder. 2. Usage of the modulo operator In PHP, use the % symbol to represent the modulus
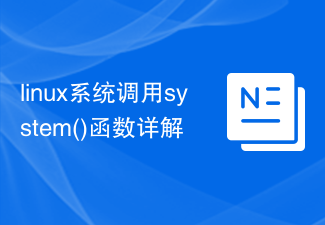
Detailed explanation of Linux system call system() function System call is a very important part of the Linux operating system. It provides a way to interact with the system kernel. Among them, the system() function is one of the commonly used system call functions. This article will introduce the use of the system() function in detail and provide corresponding code examples. Basic Concepts of System Calls System calls are a way for user programs to interact with the operating system kernel. User programs request the operating system by calling system call functions
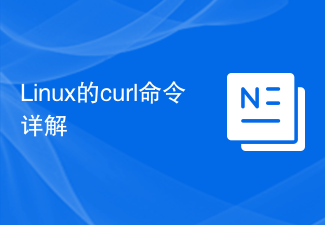
Detailed explanation of Linux's curl command Summary: curl is a powerful command line tool used for data communication with the server. This article will introduce the basic usage of the curl command and provide actual code examples to help readers better understand and apply the command. 1. What is curl? curl is a command line tool used to send and receive various network requests. It supports multiple protocols, such as HTTP, FTP, TELNET, etc., and provides rich functions, such as file upload, file download, data transmission, proxy
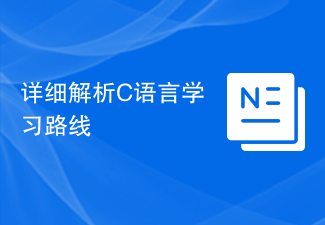
As a programming language widely used in the field of software development, C language is the first choice for many beginner programmers. Learning C language can not only help us establish the basic knowledge of programming, but also improve our problem-solving and thinking abilities. This article will introduce in detail a C language learning roadmap to help beginners better plan their learning process. 1. Learn basic grammar Before starting to learn C language, we first need to understand the basic grammar rules of C language. This includes variables and data types, operators, control statements (such as if statements,
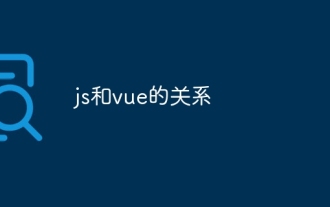
The relationship between js and vue: 1. JS as the cornerstone of Web development; 2. The rise of Vue.js as a front-end framework; 3. The complementary relationship between JS and Vue; 4. The practical application of JS and Vue.
