Detailed explanation of inheritance methods in JS
This article mainly introduces the detailed explanation of inheritance methods in JavaScript. This article explains the concept of js inheritance, prototypal inheritance and class inheritance, prototype chain inheritance, class inheritance, combination inheritance, prototypal inheritance, etc., which requires Friends can refer to
The concept of js inheritance
The following two inheritance methods are commonly used in js:
Prototype chain inheritance (between objects Inheritance)
Class inheritance (Inheritance between constructors)
Since js is not a truly object-oriented language like java, js is based on objects, it There is no concept of classes. Therefore, if you want to implement inheritance, you can use the prototype mechanism of js or the apply and call methods to achieve
In object-oriented languages, we use classes to create a custom object. However, everything in js is an object, so how to create a custom object? This requires the use of js prototype:
We can simply regard prototype as a template. The newly created custom objects are all copies of this template (prototype) (actually not a copy but a copy) Link, but this link is invisible. There is an invisible Proto pointer inside the newly instantiated object, pointing to the prototype object).
js can simulate the functions of the class through constructors and prototypes. In addition, the implementation of js class inheritance also relies on the prototype chain.
Prototypal inheritance and class inheritance
Class inheritance is to call the supertype constructor inside the subtype constructor.
Strict class inheritance is not very common, and is usually used in combination:
The code is as follows:
function Super(){ this.colors=["red","blue"]; } function Sub(){ Super.call(this); }
Prototypal inheritance uses existing objects Creating a new object and pointing the prototype of the subclass to the parent class is equivalent to joining the prototype chain of the parent class
Prototype chain inheritance
In order to let the subclass To inherit the attributes (including methods) of the parent class, you first need to define a constructor. Then, assign the new instance of the parent class to the constructor's prototype. The code is as follows:
The code is as follows:
<script> function Parent(){ this.name = 'mike'; } function Child(){ this.age = 12; } Child.prototype = new Parent();//Child继承Parent,通过原型,形成链条 var test = new Child(); alert(test.age); alert(test.name);//得到被继承的属性 //继续原型链继承 function Brother(){ //brother构造 this.weight = 60; } Brother.prototype = new Child();//继续原型链继承 var brother = new Brother(); alert(brother.name);//继承了Parent和Child,弹出mike alert(brother.age);//弹出12 </script>
There is still one missing link in the above prototype chain inheritance, which is Object. All constructors inherit from Object. Inheriting Object is completed automatically and does not require us to inherit manually. So what is their affiliation?
Determine the relationship between prototypes and instances
The relationship between prototypes and instances can be determined in two ways. Operator instanceof and isPrototypeof() methods:
The code is as follows:
alert(brother instanceof Object)//true alert(test instanceof Brother);//false,test 是brother的超类 alert(brother instanceof Child);//true alert(brother instanceof Parent);//true
As long as it is a prototype that appears in the prototype chain, it can be said to be the prototype of the instance derived from the prototype chain , therefore, the isPrototypeof() method will also return true
In js, the inherited function is called the supertype (parent class, base class is also acceptable), and the inherited function is called the subtype (subclass, Derived class). There are two main problems with using prototypal inheritance:
First, overriding the prototype with literals will break the relationship, using the prototype of the reference type, and the subtype cannot pass parameters to the supertype.
Pseudo classes solve the problem of reference sharing and the inability to pass parameters of super types. We can use the "borrowed constructor" technology
Borrowed constructor (class inheritance)
The code is as follows:
<script> function Parent(age){ this.name = ['mike','jack','smith']; this.age = age; } function Child(age){ Parent.call(this,age); } var test = new Child(21); alert(test.age);//21 alert(test.name);//mike,jack,smith test.name.push('bill'); alert(test.name);//mike,jack,smith,bill </script>
Although borrowing the constructor solves the two problems just now, without a prototype, reuse is impossible, so we need a prototype chain + a borrowed constructor mode, this mode is called combined inheritance
Combined inheritance
The code is as follows:
<script> function Parent(age){ this.name = ['mike','jack','smith']; this.age = age; } Parent.prototype.run = function () { return this.name + ' are both' + this.age; }; function Child(age){ Parent.call(this,age);//对象冒充,给超类型传参 } Child.prototype = new Parent();//原型链继承 var test = new Child(21);//写new Parent(21)也行 alert(test.run());//mike,jack,smith are both21 </script>
Combined inheritance is a commonly used one An inheritance method, the idea behind it is to use the prototype chain to realize the inheritance of prototype properties and methods , and to realize the inheritance of instance properties by borrowing the constructor. In this way, function reuse is achieved by defining methods on the prototype, and each instance is guaranteed to have its own attributes.
Usage of call(): Call a method of an object and replace the current object with another object.
The code is as follows:
call([thisObj[,arg1[, arg2[, [,.argN]]]]])
Prototypal inheritance
<script> function obj(o){ function F(){} F.prototype = o; return new F(); } var box = { name : 'trigkit4', arr : ['brother','sister','baba'] }; var b1 = obj(box); alert(b1.name);//trigkit4 b1.name = 'mike'; alert(b1.name);//mike alert(b1.arr);//brother,sister,baba b1.arr.push('parents'); alert(b1.arr);//brother,sister,baba,parents var b2 = obj(box); alert(b2.name);//trigkit4 alert(b2.arr);//brother,sister,baba,parents </script>
Parasitic inheritance
This inheritance method combines the prototype +factory pattern in order to encapsulate the creation process.
<script> function create(o){ var f= obj(o); f.run = function () { return this.arr;//同样,会共享引用 }; return f; } </script>
Small problems with combined inheritance
组合式继承是js最常用的继承模式,但组合继承的超类型在使用过程中会被调用两次;一次是创建子类型的时候,另一次是在子类型构造函数的内部
代码如下:
<script> function Parent(name){ this.name = name; this.arr = ['哥哥','妹妹','父母']; } Parent.prototype.run = function () { return this.name; }; function Child(name,age){ Parent.call(this,age);//第二次调用 this.age = age; } Child.prototype = new Parent();//第一次调用 </script>
以上代码是之前的组合继承,那么寄生组合继承,解决了两次调用的问题。
寄生组合式继承
代码如下:
<script> function obj(o){ function F(){} F.prototype = o; return new F(); } function create(parent,test){ var f = obj(parent.prototype);//创建对象 f.constructor = test;//增强对象 } function Parent(name){ this.name = name; this.arr = ['brother','sister','parents']; } Parent.prototype.run = function () { return this.name; }; function Child(name,age){ Parent.call(this,name); this.age =age; } inheritPrototype(Parent,Child);//通过这里实现继承 var test = new Child('trigkit4',21); test.arr.push('nephew'); alert(test.arr);// alert(test.run());//只共享了方法 var test2 = new Child('jack',22); alert(test2.arr);//引用问题解决 </script>
call和apply
全局函数apply和call可以用来改变函数中this的指向,如下:
代码如下:
// 定义一个全局函数 function foo() { console.log(this.fruit); } // 定义一个全局变量 var fruit = "apple"; // 自定义一个对象 var pack = { fruit: "orange" }; // 等价于window.foo(); foo.apply(window); // "apple",此时this等于window // 此时foo中的this === pack foo.apply(pack); // "orange"
The above is the detailed content of Detailed explanation of inheritance methods in JS. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


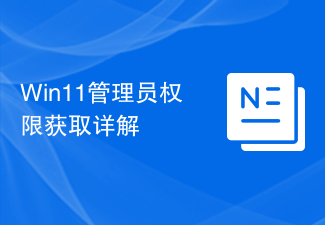
Windows operating system is one of the most popular operating systems in the world, and its new version Win11 has attracted much attention. In the Win11 system, obtaining administrator rights is an important operation. Administrator rights allow users to perform more operations and settings on the system. This article will introduce in detail how to obtain administrator permissions in Win11 system and how to effectively manage permissions. In the Win11 system, administrator rights are divided into two types: local administrator and domain administrator. A local administrator has full administrative rights to the local computer
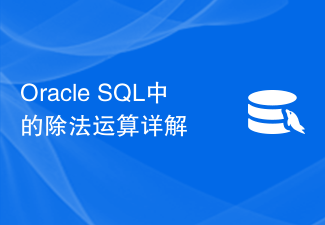
Detailed explanation of division operation in OracleSQL In OracleSQL, division operation is a common and important mathematical operation, used to calculate the result of dividing two numbers. Division is often used in database queries, so understanding the division operation and its usage in OracleSQL is one of the essential skills for database developers. This article will discuss the relevant knowledge of division operations in OracleSQL in detail and provide specific code examples for readers' reference. 1. Division operation in OracleSQL
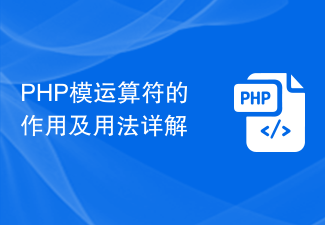
The modulo operator (%) in PHP is used to obtain the remainder of the division of two numbers. In this article, we will discuss the role and usage of the modulo operator in detail, and provide specific code examples to help readers better understand. 1. The role of the modulo operator In mathematics, when we divide an integer by another integer, we get a quotient and a remainder. For example, when we divide 10 by 3, the quotient is 3 and the remainder is 1. The modulo operator is used to obtain this remainder. 2. Usage of the modulo operator In PHP, use the % symbol to represent the modulus
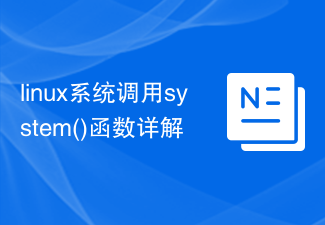
Detailed explanation of Linux system call system() function System call is a very important part of the Linux operating system. It provides a way to interact with the system kernel. Among them, the system() function is one of the commonly used system call functions. This article will introduce the use of the system() function in detail and provide corresponding code examples. Basic Concepts of System Calls System calls are a way for user programs to interact with the operating system kernel. User programs request the operating system by calling system call functions
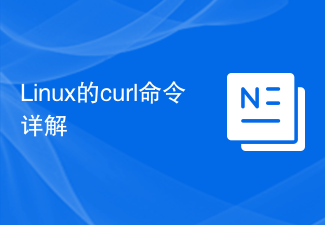
Detailed explanation of Linux's curl command Summary: curl is a powerful command line tool used for data communication with the server. This article will introduce the basic usage of the curl command and provide actual code examples to help readers better understand and apply the command. 1. What is curl? curl is a command line tool used to send and receive various network requests. It supports multiple protocols, such as HTTP, FTP, TELNET, etc., and provides rich functions, such as file upload, file download, data transmission, proxy
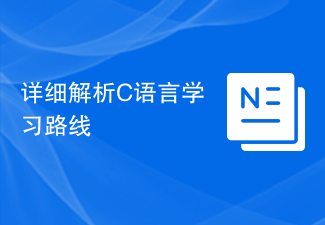
As a programming language widely used in the field of software development, C language is the first choice for many beginner programmers. Learning C language can not only help us establish the basic knowledge of programming, but also improve our problem-solving and thinking abilities. This article will introduce in detail a C language learning roadmap to help beginners better plan their learning process. 1. Learn basic grammar Before starting to learn C language, we first need to understand the basic grammar rules of C language. This includes variables and data types, operators, control statements (such as if statements,
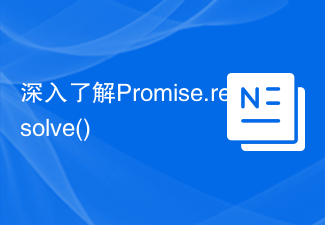
Detailed explanation of Promise.resolve() requires specific code examples. Promise is a mechanism in JavaScript for handling asynchronous operations. In actual development, it is often necessary to handle some asynchronous tasks that need to be executed in sequence, and the Promise.resolve() method is used to return a Promise object that has been fulfilled. Promise.resolve() is a static method of the Promise class, which accepts a
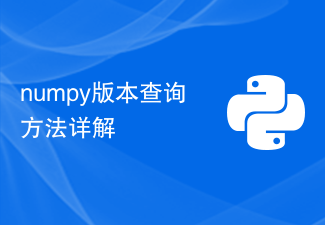
Numpy is a Python scientific computing library that provides a wealth of array operation functions and tools. When upgrading the Numpy version, you need to query the current version to ensure compatibility. This article will introduce the method of Numpy version query in detail and provide specific code examples. Method 1: Use Python code to query the Numpy version. You can easily query the Numpy version using Python code. The following is the implementation method and sample code: importnumpyasnpprint(np
