Introduction to variables and data types in Python
1. Variables and data types
1.1 Variables
1. Each variable stores a value—the information associated with the variable.
2. Variables can be not only integers or floating point numbers, but also strings, and can be of any data type.
1.1.1 Naming and using variables
Variable names can only contain letters, numbers and underscores, and cannot begin with numbers. Variable names cannot contain spaces, but can be separated by underscores. Python keywords and function names cannot be used as variable names. Variable names should be short and descriptive. Be careful with the lowercase l and uppercase O, as they can be mistaken for the numbers 1 and 0.
1.1.2 Avoid naming errors when using variables
The interpreter will provide a traceback when an error occurs. A traceback is a record that points out where trouble occurred.
1.2 String str
1.String is a series of characters. It is a data type. In Python, all strings enclosed by quotes can be single quotes or double quotes.
2. The Unicode standard is also constantly evolving, but the most commonly used one is to use two bytes to represent a character (if you want to use very remote characters, you need 4 bytes). Modern operating systems and most programming languages support Unicode directly. Convert Unicode encoding into "variable length encoding" UTF-8 encoding.
3.Python uses single quotes or double quotes with b prefix to represent bytes type data: x = b'ABC'. Str expressed in Unicode can be encoded into specified bytes through the encode() method.
'ABC'.encode('ascii') b'ABC |
Conversely, if we read a byte stream from the network or disk, the data read is bytes. To change bytes into str, you need to use the decode() method:
b'ABC'.decode('ascii') 'ABC |
4. For the encoding of a single character, Python provides the ord() function to obtain the integer representation of the character, and the chr() function converts the encoding to the corresponding Characters:
##>>> ord('A')65>> > ord('中')20013>>> chr(66)'B'>> > chr(25991)'文' |
'cats, rats, bats' >>> ' '.join(['My', 'name', 'is', 'Simon']) 'My name is Simon' >>> 'ABC'.join(['My', 'name', 'is', 'Simon']) 'MyABCnameABCisABCSimon' |
['My', 'name', ' is', 'Simon'] |
##>>> 'Hello'.rjust(20, '*')'******* ********Hello' >>> 'Hello'.ljust(20, '-')'Hello-------- -------' |
case sensitive. If the wrong case is entered, the program will report an error.
17. Merge - Python uses the plus sign + to merge strings
|
That is Carol\'s cat. |
Syntax Error:
is an error that is encountered from time to time. In a string enclosed in single quotes, if an apostrophe is included, it will cause an error. Double quotes won't.21.Print() prints, the comma will be one space empty.
22. The pyperclip module has copy() and paste() functions that can send text to or receive text from the computer's clipboard. 23. String has a replace() method
'Abc' ##1.2.2 empty Value |
##1.2.4 Assignment
In Python, the equal sign = is an assignment statement, and any data type can be assigned a value To a variable, the same variable can be assigned repeatedly, and it can be a variable of different types:
a = 123 # a is an integer
print(a) Assignment statement : a, b = b, a + b |
1.2.5 FormattingThere are two types of formatting in Python The first one is implemented with %, and the second one is { } format. |
% operator is Used to format strings. Within the string, %s means replacing with a string, %d means replacing with an integer, there are several %? placeholders, followed by several variables or values, and the order must be corresponding. If there is only one | %?
##%d Integer%f Floating point number
Among them, formatted integers and floating point numbers can also specify whether to add 0 And the number of digits in integers and decimals: |
If you are not sure what to use, %s will always work, it will convert any data type to a string. Sometimes, the % in the string is an ordinary character and needs to be escaped. Use %% to represent a %. The second formatting method, format, replaces % with {}. |
##>>> print("My name is {} and I am {} years old this year".format ("Xiao Li", 20))
My name is Xiao Li, I am 20 years old this year
##>>> print("My name is {1 }, this year is {0} years old".format("Xiao Li", 20))My name is 20, this year Xiao Li is years old |
My name is Xiao Li, I am 20 years old this year |
Whitespace - refers to any non-printing characters such as spaces, tabs, and newlines.
Escape character\ can escape many characters\t tab character \n newline
character\ itself also needs to be escaped, so the character represented by \\ is \
If there are many characters in the string that need to be escaped, you can use r'' in Python to represent ''. The internal string is not escaped by default:
\ \ >>> print(r'\\\t\\') \\\t\\ |
1.3.1 Integer int
can be performed Arithmetic.
Since computers use binary, it is sometimes more convenient to use hexadecimal to represent integers. Hexadecimal is represented by the 0x prefix and 0-9, a-f, for example: 0xff00, 0xa5b4c3d2, etc.
Division of integersis exact. In Python, there are two kinds of division. One division is /, /The result of division is a floating point number. Even if two integers are exactly divisible, the result is a floating point number. Another kind of division is //, called floor division. The division of two integers is still an integer. % Take the remainder.
1.3.2 Floating point number float
Python calls numbers with decimals floating point numbers. The reason why they are called floating point numbers is because when expressed in scientific notation, a floating point number The decimal point position is variable. For example, 1.23x10
9and 12.3x108 are completely equal. For very large or small floating point numbers, they must be expressed in scientific notation. Replace 10 with e. 1.23x109 is 1.23e9, or 12.3e8, 0.000012 can be written as 1.2e-5, etc.
1.3.3 Use the function str() to avoid errors
Data type checking can be implemented using the built-in function isinstance():
if not isinstance(x, (int, float)): raise TypeError('bad operand type') if x >= 0: ’ s ’ ’ through through through through through through through through out out off off out off out through out through out out through out right Through out through over over over ‐ After ‐ ‐ n w w w w‐ ‐ to to to to to to to to to come a 1.4 Notes #1. Statements starting with # are comments. Comments are for human viewing and can be any content. The interpreter will ignore the comments. Each other line is a statement, and when the statement ends with a colon:, the indented statement is considered a code block. #. . . . . . |
#!/usr/bin/env python3# -*- coding: utf-8 -*- |
The Zen of Python, by Tim Peters Beautiful is better than ugly. |
- Complex is better than complicated.
- Flat is better than nested.
- Sparse is better than dense.
- Readability counts.
- Special cases aren't special enough to break the rules.
- Although practicality beats purity.
- Errors should never pass silently.
- Unless explicitly silenced.
- In the face of ambiguity, refuse the temptation to guess.
- There should be one-- and preferably only one --obvious way to do it. ##Although that way may not be obvious at first unless you're Dutch.
- Now is better than never.
- Although never is often better than *right* now.
- If the implementation is hard to explain, it's a bad idea.
- If the implementation is easy to explain, it may be a good idea.
- Namespaces are one honking great idea -- let's do more of those!
The above is the detailed content of Introduction to variables and data types in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


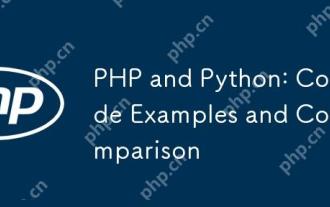
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
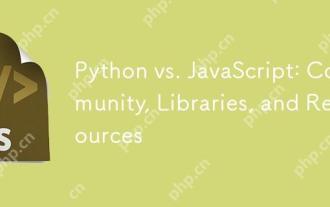
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
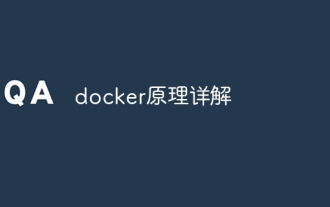
Docker uses Linux kernel features to provide an efficient and isolated application running environment. Its working principle is as follows: 1. The mirror is used as a read-only template, which contains everything you need to run the application; 2. The Union File System (UnionFS) stacks multiple file systems, only storing the differences, saving space and speeding up; 3. The daemon manages the mirrors and containers, and the client uses them for interaction; 4. Namespaces and cgroups implement container isolation and resource limitations; 5. Multiple network modes support container interconnection. Only by understanding these core concepts can you better utilize Docker.
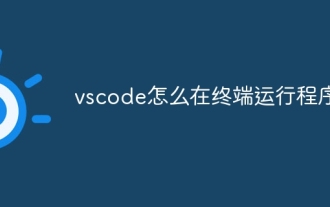
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
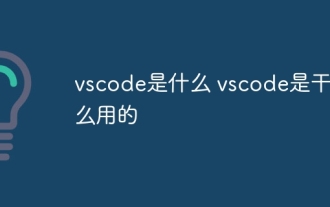
VS Code is the full name Visual Studio Code, which is a free and open source cross-platform code editor and development environment developed by Microsoft. It supports a wide range of programming languages and provides syntax highlighting, code automatic completion, code snippets and smart prompts to improve development efficiency. Through a rich extension ecosystem, users can add extensions to specific needs and languages, such as debuggers, code formatting tools, and Git integrations. VS Code also includes an intuitive debugger that helps quickly find and resolve bugs in your code.
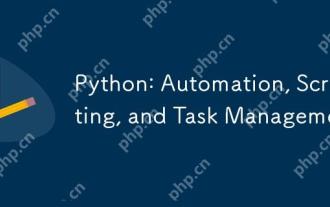
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
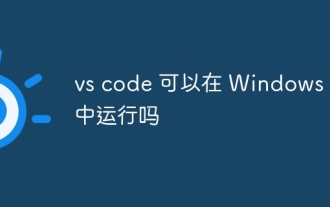
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
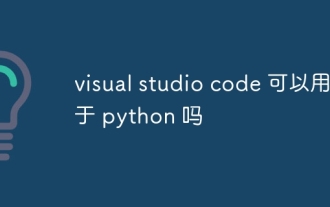
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
