


Detailed explanation of the differences between the two overloaded versions in PHP
"Overloading" is an implementation of polymorphism of the class. Function overloading means that an identifier is used as multiple function names, and these functions with the same name can be distinguished by the number of parameters or parameter types of the function, so that no confusion occurs when calling. The main advantage of this is that you don't have to write multiple functions for different parameter types or number of parameters.
Multiple functions use the same name, but the parameter list, that is, the number or (and) number of parametersdata type can be different. When calling, although the method name is the same, the parameter list is different depending on the parameters. The table can automatically call the corresponding function.
PHP4 only implements some of the simple functions of
Object-oriented, but the support for objects will be much more powerful in PHP5.
For polymorphic implementation, PHP4 only supports override but not overload. But we can use some tricks to "simulate" the implementation of overloading.
Although PHP5 can support overwriting and overloading, the specific implementation of overloading is quite different from other languages.
1, "simulate" overloading in PHP4
Try the following code:
<?php //根据参数个数选择执行不同的方法(在 PHP4 中模拟"重载"(多态的一种) class Myclass { function Myclass() { $method = "method" . func_num_args(); $this->$method(); } function method1($x) { echo "method1"; } function method2($x, $y) { echo 'method2'; } } //通过在类中的额外的处理,使用这个类对用户是透明的: $obj1 = new Myclass('A'); //将调用 method1 $obj2 = new Myclass('B','C'); //将调用 method2 ?>
constructor Use the func_num_args() function to get the number of parameters and automatically execute the method1 or method2 method. We can improve the above example by combining the functions func_get_arg(i) and func_get_args().
2, Use overloading in PHP5
First look at the following examples:
<?php class Myclass { public $attriable; public $one = "this is one"; public $two = "this is two"; function construct() { } function one($one) { $this->one=$one; $this->attriable = $this->one; } function one($one, $two) { $this->one=$one; $this->two=$two; $this->attriable = $this->one . $this->two; } function display() { echo $this->attriable; } } $one = "this is my class"; $two = "Im the best"; $myclass = new myclass(); $myclass->one($one); $myclass->display(); $myclass->one($one, $two); $myclass->display(); //本例的做法,在 PHP 中是不正确的! ?>
People who have used overloading in C++, Java, and C#, It is very customary to write the PHP code for the above overloaded implementation. But this is not true in PHP5. PHP5 is not an imitation of the aforementioned languages, but has its own set of methods for implementing
method overloading (whether it is good or bad will not be discussed here). Although the classes of PHP5 are much more powerful than those of PHP4, the issue of "overloading" has not been "improved" as we expected. In "strongly" typed languages, "overloading" can be achieved through different parameter types, such as C++, Java, C#, etc. In languages where "fixed parameters" are passed, it can also be passed by the number of parameters, such as Java, but PHP is a weakly typed language, so there will be no "overloading" like the above.
Overloading in PHP5 can be performed through several special methods: get, set, and call. PHP will call these methods when the Zend engine tries to access a member and cannot find it.
In the following example, get and set replace all accesses to the attribute variable array. If necessary, you can also implement any type of filtering you want. For example, a script can disable setting property values, start with a certain prefix, or include a certain type of value. The call method illustrates how you can call an undefined method. When you call an undefined method, the method name and the parameters received by the method will be passed to the call method, and PHP will return the call value to the undefined method.
<?php class Overloader { private $properties = array(); function get($property_name) { if(isset($this->properties[$property_name])) { return($this->properties[$property_name]); } else { return(NULL); } } function set($property_name, $value) { $this->properties[$property_name] = $value; } public function call($method, $p) { print("Invoking $method()<br>\n"); //print("Arguments: "); //print_r($args); if($method == 'display') { if(is_object($p[0])) $this->displayObject($p[0]); else if(is_array($p[0])) $this->displayArray($p[0]); else $this->displayScalar($p[0]); } } public function displayObject($p) { echo ("你传入的是个对象,内容如下:<br>"); print_r($p); echo "<hr>"; } public function displayArray($p) { echo ("你传入的是个数组,内容如下:<br>"); print_r($p); echo "<hr>"; } public function displayScalar($p) { echo ("你传入的是个单独变量,内容如下:<br>" . $p); echo "<hr>"; } } $o = new Overloader(); //调用 set() 给一个不存在的属性变量赋值 $o->dynaProp = "Dynamic Content"; //调用 get() print($o->dynaProp . "<br>\n"); //调用 call() //$o->dynaMethod("Leon", "Zeev"); $o->display(array(1,2,3)); $o->display('Cat'); ?>
In the above code, the display() method is called, and the corresponding code segment in the class can be called according to the type and number of parameters, thus realizing the object method. Overload.
The above is the detailed content of Detailed explanation of the differences between the two overloaded versions in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



In PHP, you can effectively prevent CSRF attacks by using unpredictable tokens. Specific methods include: 1. Generate and embed CSRF tokens in the form; 2. Verify the validity of the token when processing the request.
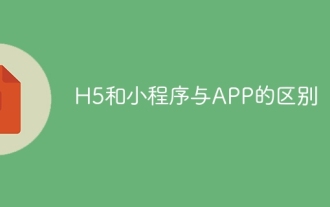
H5. The main difference between mini programs and APP is: technical architecture: H5 is based on web technology, and mini programs and APP are independent applications. Experience and functions: H5 is light and easy to use, with limited functions; mini programs are lightweight and have good interactiveness; APPs are powerful and have smooth experience. Compatibility: H5 is cross-platform compatible, applets and APPs are restricted by the platform. Development cost: H5 has low development cost, medium mini programs, and highest APP. Applicable scenarios: H5 is suitable for information display, applets are suitable for lightweight applications, and APPs are suitable for complex functions.
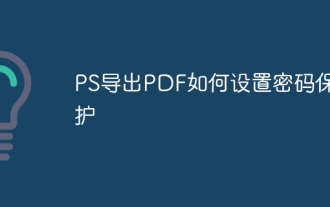
Export password-protected PDF in Photoshop: Open the image file. Click "File"> "Export"> "Export as PDF". Set the "Security" option and enter the same password twice. Click "Export" to generate a PDF file.
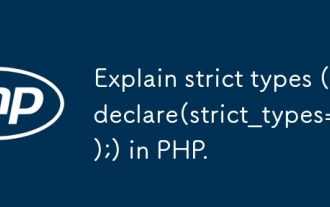
Strict types in PHP are enabled by adding declare(strict_types=1); at the top of the file. 1) It forces type checking of function parameters and return values to prevent implicit type conversion. 2) Using strict types can improve the reliability and predictability of the code, reduce bugs, and improve maintainability and readability.

In PHP, the final keyword is used to prevent classes from being inherited and methods being overwritten. 1) When marking the class as final, the class cannot be inherited. 2) When marking the method as final, the method cannot be rewritten by the subclass. Using final keywords ensures the stability and security of your code.

The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
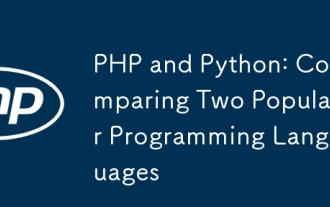
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
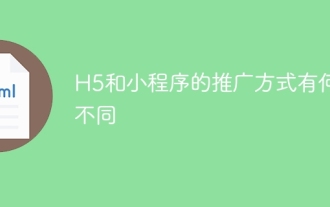
There are differences in the promotion methods of H5 and mini programs: platform dependence: H5 depends on the browser, and mini programs rely on specific platforms (such as WeChat). User experience: The H5 experience is poor, and the mini program provides a smooth experience similar to native applications. Communication method: H5 is spread through links, and mini programs are shared or searched through the platform. H5 promotion methods: social sharing, email marketing, QR code, SEO, paid advertising. Mini program promotion methods: platform promotion, social sharing, offline promotion, ASO, cooperation with other platforms.
