How to dynamically insert CSS in JavaScript_javascript skills
When writing components, sometimes I want to encapsulate some CSS styles related to component features in JS, so that they are more cohesive and easy to change. JS dynamically inserts CSS in two steps: Create 1. A style object
2. Use the insertRule or addRule method of stylesheet to add styles
1. View the style sheet
Look at document.styleSheets first and open a page at will
The first three are CSS files introduced through link tags, and the fourth is CSS inlined in the page through style tags. It has the following attributes
Each cssRule has the following attributes
The cssText is exactly the source code written in style.
2. Dynamically insert CSS
First, you need to create a style object and return its stylesheet object
/* * 创建一个 style, 返回其 stylesheet 对象 * 注意:IE6/7/8中使用 style.stylesheet,其它浏览器 style.sheet */ function createStyleSheet() { var head = document.head || document.getElementsByTagName('head')[0]; var style = document.createElement('style'); style.type = 'text/css'; head.appendChild(style); return style.sheet ||style.styleSheet; }
Add the function addCssRule as follows
/* * 动态添加 CSS 样式 * @param selector {string} 选择器 * @param rules {string} CSS样式规则 * @param index {number} 插入规则的位置, 靠后的规则会覆盖靠前的 */ function addCssRule(selector, rules, index) { index = index || 0; if (sheet.insertRule) { sheet.insertRule(selector + "{" + rules + "}", index); } else if (sheet.addRule) { sheet.addRule(selector, rules, index); } }
It should be noted that standard browsers support insertRule, and lower versions of IE support addRule.
The complete code is as follows
/* * 动态添加 CSS 样式 * @param selector {string} 选择器 * @param rules {string} CSS样式规则 * @param index {number} 插入规则的位置, 靠后的规则会覆盖靠前的 */ var addCssRule = function() { // 创建一个 style, 返回其 stylesheet 对象 // 注意:IE6/7/8中使用 style.stylesheet,其它浏览器 style.sheet function createStyleSheet() { var head = document.head || document.getElementsByTagName('head')[0]; var style = document.createElement('style'); style.type = 'text/css'; head.appendChild(style); return style.sheet ||style.styleSheet; } // 创建 stylesheet 对象 var sheet = createStyleSheet(); // 返回接口函数 return function(selector, rules, index) { index = index || 0; if (sheet.insertRule) { sheet.insertRule(selector + "{" + rules + "}", index); } else if (sheet.addRule) { sheet.addRule(selector, rules, index); } } }();
If it only supports mobile terminals or modern browsers, you can remove the code judged by lower versions of IE
/* * 动态添加 CSS 样式 * @param selector {string} 选择器 * @param rules {string} CSS样式规则 * @param index {number} 插入规则的位置, 靠后的规则会覆盖靠前的,默认在后面插入 */ var addCssRule = function() { // 创建一个 style, 返回其 stylesheet 对象 function createStyleSheet() { var style = document.createElement('style'); style.type = 'text/css'; document.head.appendChild(style); return style.sheet; } // 创建 stylesheet 对象 var sheet = createStyleSheet(); // 返回接口函数 return function(selector, rules, index) { index = index || 0; sheet.insertRule(selector + "{" + rules + "}", index); } }();
The above is the method of dynamically inserting CSS in JavaScript. I hope it will be helpful to everyone's learning.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


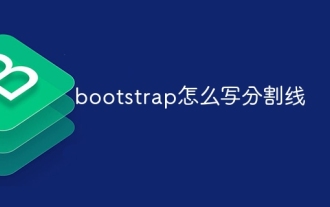
There are two ways to create a Bootstrap split line: using the tag, which creates a horizontal split line. Use the CSS border property to create custom style split lines.
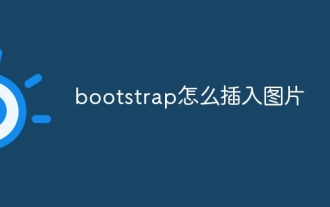
There are several ways to insert images in Bootstrap: insert images directly, using the HTML img tag. With the Bootstrap image component, you can provide responsive images and more styles. Set the image size, use the img-fluid class to make the image adaptable. Set the border, using the img-bordered class. Set the rounded corners and use the img-rounded class. Set the shadow, use the shadow class. Resize and position the image, using CSS style. Using the background image, use the background-image CSS property.
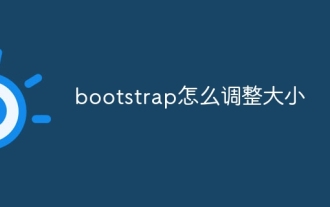
To adjust the size of elements in Bootstrap, you can use the dimension class, which includes: adjusting width: .col-, .w-, .mw-adjust height: .h-, .min-h-, .max-h-

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.
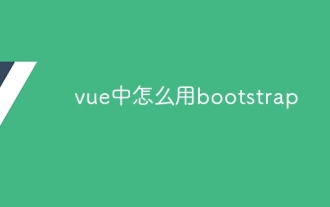
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
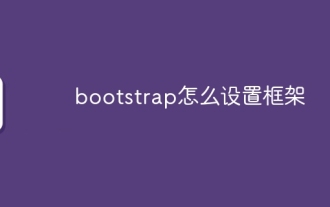
To set up the Bootstrap framework, you need to follow these steps: 1. Reference the Bootstrap file via CDN; 2. Download and host the file on your own server; 3. Include the Bootstrap file in HTML; 4. Compile Sass/Less as needed; 5. Import a custom file (optional). Once setup is complete, you can use Bootstrap's grid systems, components, and styles to create responsive websites and applications.
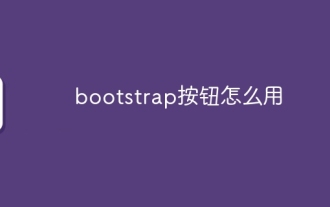
How to use the Bootstrap button? Introduce Bootstrap CSS to create button elements and add Bootstrap button class to add button text
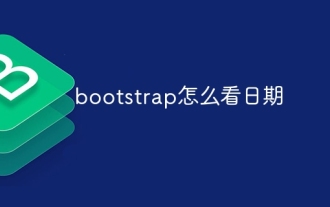
Answer: You can use the date picker component of Bootstrap to view dates in the page. Steps: Introduce the Bootstrap framework. Create a date selector input box in HTML. Bootstrap will automatically add styles to the selector. Use JavaScript to get the selected date.
