Detailed explanation of how the for loop works in Python
If you are not very clear about the for loop in Python, then I suggest you read this article. This article mainly introduces you to the relevant information about how the for loop in Python works. It is very detailed and has certain reference and learning value for everyone. Friends who need it can take a look below.
Preface
for...in is the most commonly used statement by Python programmers. The for loop is used to iterate the containerObject# Elements in ##, these objects can be lists, tuples, dictionaries, collections, files, or even custom classes or functions, for example:
acts on lists
>>> for elem in [1,2,3]: ... print(elem) ... 1 2 3
Applies to tuples
>>> for i in ("zhang", "san", 30): ... print(i) ... zhang san 30
>>> for c in "abc": ... print(c) ... a b c
Action on collection
>>> for i in {"a","b","c"}: ... print(i) ... b a c
Action In dictionary
>>> for k in {"age":10, "name":"wang"}: ... print(k) ... age name
Apply to file
##
>>> for line in open("requirement.txt"): ... print(line, end="") ... Fabric==1.12.0 Markdown==2.6.7
Some people may not know You have to ask, why do so many different types of objects support the for statement? What other types of objects can be used in the for statement? Before answering this question, we must first understand the execution principle behind the for loop.
The for loop is the process of iterating the container. What is iteration? Iteration is to read elements from a container object one by one until there are no more elements in the container. So, which objects support iterative operations? Can any object be used? Try customizing a class first and see if it works:
>>> class MyRange: ... def init(self, num): ... self.num = num ... >>> for i in MyRange(10): ... print(i) ... Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: 'MyRange' object is not iterable
The error stack log tells us very clearly that MyRange is not an iterable object, so it cannot be used Iteration, so what kind of object can be called an iterable object (iterable)?
Iterable objects need to implement the iter method and return an iterator. What is an iterator? Iterators only need to implement the next method. Now let's verify why the list supports iteration:
>>> x = [1,2,3] >>> its = x.iter() # x有此方法,说明列表是可迭代对象 >>> its <list_iterator object at 0x100f32198> >>> its.next() # its有此方法,说明its是迭代器 1 >>> its.next() 2 >>> its.next() 3 >>> its.next() Traceback (most recent call last): File "<stdin>", line 1, in <module> StopIteration
From the test results, the list is an iterable object because it implements the iter method and returns An iterator object (list_iterator) because it implements the next method. We see that it continuously calls the next method, which actually continuously iterates to obtain the elements in the container until there are no more elements in the container and a StopIteration exception is thrown. So how does the for statement loop? At this point, I am afraid you have guessed it. The steps are:
- # First determine whether the object is an iterable object. If not, an error will be reported directly and a TypeError exception will be thrown. If so, , call the iter method and return an iterator
- Continuously call the next method of the iterator, each time returning a value in the iterator in order
- At the end of the iteration, if there are no more elements,
- throw an exception
StopIteration. Python will handle this exception by itself and will not expose it to developers
The same is true for tuples, dictionaries, and strings. After understanding the execution principle of for, we can implement our own iterators for use in for loops.
The previous MyRange error is because it does not implement these two methods in the iterator protocol. Now continue to improve:
class MyRange: def init(self, num): self.i = 0 self.num = num def iter(self): return self def next(self): if self.i < self.num: i = self.i self.i += 1 return i else: # 达到某个条件时必须抛出此异常,否则会无止境地迭代下去 raise StopIteration()
Because it implements next method, so MyRange itself is already an iterator, so iter returns the object itself. Now try using it in a for loop:
for i in MyRange(3): print(i) # 输出 0 1 2
Have you noticed that the custom MyRange function is very similar to the built-in function range. The essence of a for loop is to continuously call the next method of the iterator until a StopIteration exception occurs, so any iterable object can be used in a for loop.
The above is the detailed content of Detailed explanation of how the for loop works in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




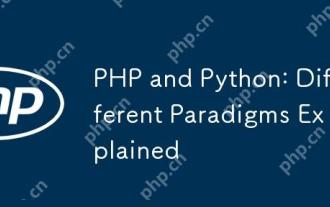
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
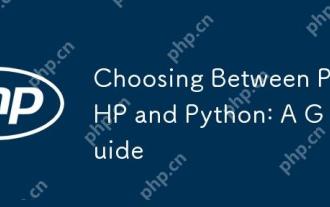
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
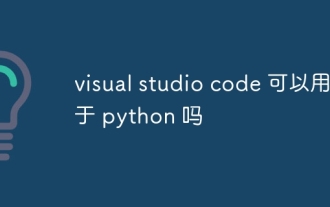
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
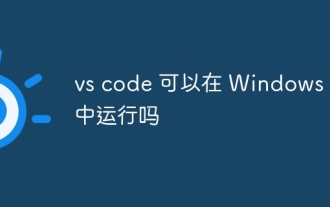
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
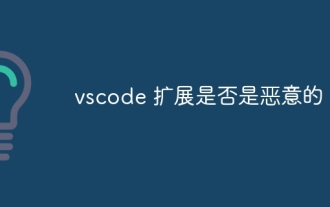
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
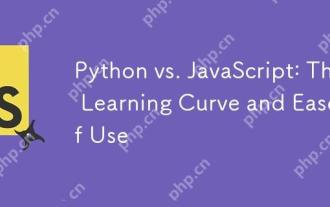
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
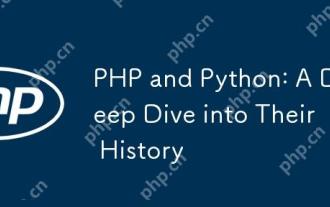
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
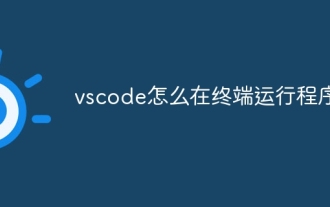
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
