Detailed introduction to Django middleware
I have been writing scripts for a few weeks, and today I finally started to get in touch with web frameworks~ To learn Python’s web framework, Django is almost a required course. This time, I will start with it first. The main task is to Add the version number in the setting, attach the version number when rendering the path of static css and js, such as "example.v1124.css", and then remove the version number in the request request. Why do you do that? Because if you do this, the path to the static file output on the front end will be added with the version number. In this way, when a new static file is developed, the client will forcefully refresh the local cache. In order to achieve this purpose, you must first It must be configured in the settings file, so that the version number can be read directly from the settings every time it is modified.
1. Request cycle of Django middleware
We Send a request Request from the browser and get a response content HttpResponse. The process of passing this request to Django is as follows:
In other words, every request is first Through the process_request function in the middleware, this function returns None or HttpResponse object. If it returns the former, continue processing other middleware. If it returns an HttpResponse, the processing is aborted and returned to the web page.
2. Customize and register middleware
1. Create middleware
Create the middleware py file in the project root directory:


1 class RequestExeute(object): 2 def process_request(self, request): 3 print('process_request') 4 5 def process_view(self, request, callback, callback_args, callback_kwargs): 6 print('process_view') 7 8 def process_exception(self, request, exception): 9 """10 当views函数出错时执行11 :param request: 12 :param exception: 13 :return: 14 """15 print('process_exception')16 17 def process_response(self, request, response):18 """19 必须return HttpResponse20 :param request: 21 :param response: 22 :return: 23 """24 print('process_response')25 return response26 27 def process_template_response(self, request, response):28 """29 视图函数的返回值中,如果有render方法,才被调用30 :param request:31 :param response:32 :return:33 """34 print('process_template_response')35 return response
2. Register middleware
In the settings.py file, register in the order you want to execute :
ps. In versions 1.9 and earlier, the keyword of middleware is: MIDDLEWARE_CLASSES
MIDDLEWARE_CLASSES = ('zqxt.middleware.BlockedIpMiddleware', ...其它的中间件 )
3. Middle Execution sequence of methods in the file
1. Normal situation


1 from django.shortcuts import HttpResponse 2 from django.utils.deprecation import MiddlewareMixin 3 class M1(MiddlewareMixin): 4 def process_request(self, request): 5 print('m1.request') 6 # return HttpResponse('request_m1') 7 8 def process_view(self, request, callback, callback_args, callback_kwargs): 9 print('m1.view')10 # response = callback(request, *callback_args, **callback_kwargs)11 # return response12 13 def process_response(self, request, response):14 print('m1.response')15 return response16 17 def process_exception(self, request, exception):18 print('m1.process_exception')19 return HttpResponse('m1.process_exception')20 21 def process_template_response(self, request, response):22 """23 视图函数的返回值中,如果有render方法,才被调用24 :param request:25 :param response:26 :return:27 """28 print('m1.process_template_response')29 return response30 31 32 class M2(MiddlewareMixin):33 def process_request(self, request):34 print('m2.request')35 36 def process_view(self, request, callback, callback_args, callback_kwargs):37 print('m2.view')38 # response = callback(request, *callback_args, **callback_kwargs)39 # return response40 41 def process_response(self, request, response):42 print('m2.response')43 return response44 45 def process_exception(self, request, exception):46 print('m2.process_exception')47 return HttpResponse('m2.process_exception')48 49 def process_template_response(self, request, response):50 """51 视图函数的返回值中,如果有render方法,才被调用52 :param request:53 :param response:54 :return:55 """56 print('m2.process_template_response')57 return response
Execution order:
m1.request
m2.request
m1.view
m2.view
m2.response
m1.response
2. In version 1.10 and later
If process_request returns an HttpResponse object, return forward from the process_response of the current middleware


1 from django.shortcuts import HttpResponse 2 from django.utils.deprecation import MiddlewareMixin 3 class M1(MiddlewareMixin): 4 def process_request(self, request): 5 print('m1.request') 6 return HttpResponse('request_m1') 7 8 def process_view(self, request, callback, callback_args, callback_kwargs): 9 print('m1.view')10 # response = callback(request, *callback_args, **callback_kwargs)11 # return response12 13 def process_response(self, request, response):14 print('m1.response')15 return response16 17 def process_exception(self, request, exception):18 print('m1.process_exception')19 return HttpResponse('m1.process_exception')20 21 def process_template_response(self, request, response):22 """23 视图函数的返回值中,如果有render方法,才被调用24 :param request:25 :param response:26 :return:27 """28 print('m1.process_template_response')29 return response30 31 32 class M2(MiddlewareMixin):33 def process_request(self, request):34 print('m2.request')35 36 def process_view(self, request, callback, callback_args, callback_kwargs):37 print('m2.view')38 # response = callback(request, *callback_args, **callback_kwargs)39 # return response40 41 def process_response(self, request, response):42 print('m2.response')43 return response44 45 def process_exception(self, request, exception):46 print('m2.process_exception')47 return HttpResponse('m2.process_exception')48 49 def process_template_response(self, request, response):50 """51 视图函数的返回值中,如果有render方法,才被调用52 :param request:53 :param response:54 :return:55 """56 print('m2.process_template_response')57 return response58 59 60 # class RequestExeute(object):61 # def process_request(self, request):62 # print('process_request')63 #64 # def process_view(self, request, callback, callback_args, callback_kwargs):65 # print('process_view')66 #67 # def process_exception(self, request, exception):68 # """69 # 当views函数出错时执行70 # :param request:71 # :param exception:72 # :return:73 # """74 # print('process_exception')75 #76 # def process_response(self, request, response):77 # """78 # 必须return HttpResponse79 # :param request:80 # :param response:81 # :return:82 # """83 # print('process_response')84 # return response85 #86 # def process_template_response(self, request, response):87 # """88 # 视图函数的返回值中,如果有render方法,才被调用89 # :param request:90 # :param response:91 # :return:92 # """93 # print('process_template_response')94 # return response
Execution order:
m1.request
m1.response
process_view is similar to process_request:
1 from django.shortcuts import HttpResponse 2 from django.utils.deprecation import MiddlewareMixin 3 class M1(MiddlewareMixin): 4 def process_request(self, request): 5 print('m1.request') 6 # return HttpResponse('request_m1') 7 8 def process_view(self, request, callback, callback_args, callback_kwargs): 9 print('m1.view')10 response = callback(request, *callback_args, **callback_kwargs)11 return response12 13 def process_response(self, request, response):14 print('m1.response')15 return response16 17 def process_exception(self, request, exception):18 print('m1.process_exception')19 return HttpResponse('m1.process_exception')20 21 def process_template_response(self, request, response):22 """23 视图函数的返回值中,如果有render方法,才被调用24 :param request:25 :param response:26 :return:27 """28 print('m1.process_template_response')29 return response30 31 32 class M2(MiddlewareMixin):33 def process_request(self, request):34 print('m2.request')35 36 def process_view(self, request, callback, callback_args, callback_kwargs):37 print('m2.view')38 # response = callback(request, *callback_args, **callback_kwargs)39 # return response40 41 def process_response(self, request, response):42 print('m2.response')43 return response44 45 def process_exception(self, request, exception):46 print('m2.process_exception')47 return HttpResponse('m2.process_exception')48 49 def process_template_response(self, request, response):50 """51 视图函数的返回值中,如果有render方法,才被调用52 :param request:53 :param response:54 :return:55 """56 print('m2.process_template_response')57 return response
Execution order:
m1.request m2.request m1.view m2.response m1.respons
Copy after login
3. Before version 1.10
If process_request returns an HttpResponse object , then the process_request after the current middleware will not be executed, and the process_response of the last middleware will be returned forward
The above is the detailed content of Detailed introduction to Django middleware. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
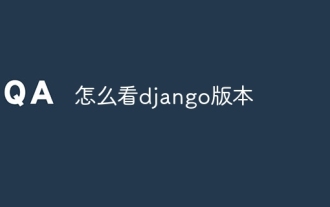
Steps to check the Django version: 1. Open a terminal or command prompt window; 2. Make sure Django has been installed. If Django is not installed, you can use the package management tool to install it and enter the pip install django command; 3. After the installation is complete , you can use python -m django --version to check the Django version.
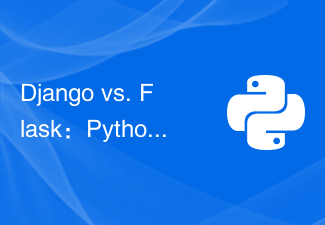
Django and Flask are both leaders in Python Web frameworks, and they both have their own advantages and applicable scenarios. This article will conduct a comparative analysis of these two frameworks and provide specific code examples. Development Introduction Django is a full-featured Web framework, its main purpose is to quickly develop complex Web applications. Django provides many built-in functions, such as ORM (Object Relational Mapping), forms, authentication, management backend, etc. These features allow Django to handle large
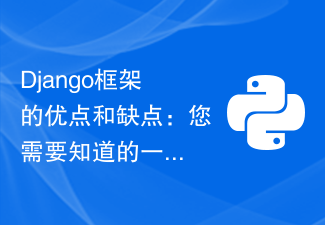
Django is a complete development framework that covers all aspects of the web development life cycle. Currently, this framework is one of the most popular web frameworks worldwide. If you plan to use Django to build your own web applications, then you need to understand the advantages and disadvantages of the Django framework. Here's everything you need to know, including specific code examples. Django advantages: 1. Rapid development-Djang can quickly develop web applications. It provides a rich library and internal
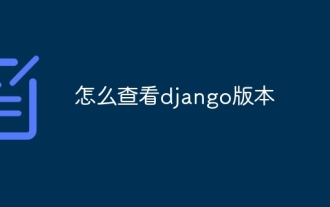
How to check the django version: 1. To check through the command line, enter the "python -m django --version" command in the terminal or command line window; 2. To check in the Python interactive environment, enter "import django print(django. get_version())" code; 3. Check the settings file of the Django project and find a list named INSTALLED_APPS, which contains installed application information.
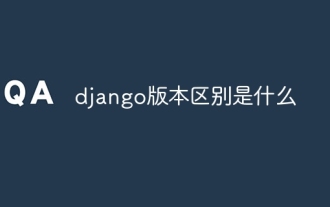
The differences are: 1. Django 1.x series: This is an early version of Django, including versions 1.0, 1.1, 1.2, 1.3, 1.4, 1.5, 1.6, 1.7, 1.8 and 1.9. These versions mainly provide basic web development functions; 2. Django 2.x series: This is the mid-term version of Django, including 2.0, 2.1, 2.2 and other versions; 3. Django 3.x series: This is the latest version series of Django. Including versions 3.0, 3, etc.
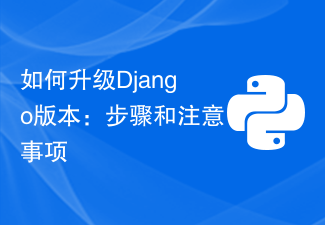
How to upgrade Django version: steps and considerations, specific code examples required Introduction: Django is a powerful Python Web framework that is continuously updated and upgraded to provide better performance and more features. However, for developers using older versions of Django, upgrading Django may face some challenges. This article will introduce the steps and precautions on how to upgrade the Django version, and provide specific code examples. 1. Back up project files before upgrading Djan
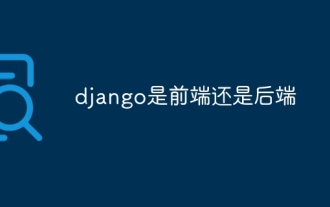
django is the backend. Details: Although Django is primarily a backend framework, it is closely related to front-end development. Through features such as Django's template engine, static file management, and RESTful API, front-end developers can collaborate with back-end developers to build powerful, scalable web applications.
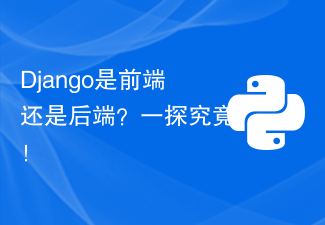
Django is a web application framework written in Python that emphasizes rapid development and clean methods. Although Django is a web framework, to answer the question whether Django is a front-end or a back-end, you need to have a deep understanding of the concepts of front-end and back-end. The front end refers to the interface that users directly interact with, and the back end refers to server-side programs. They interact with data through the HTTP protocol. When the front-end and back-end are separated, the front-end and back-end programs can be developed independently to implement business logic and interactive effects respectively, and data exchange.
