JavaScript usage sharing
An overview
1. What is JavaScript?
Based on objects and driven by events Interpreted scripting language.
2. JavaScript syntax features
are case-sensitive, which is different from HTML.
#The semicolon at the end is optional.
#Variables are weakly typed: variables are uniformly var when defined, and the type is determined when assigned.
3. UI thread
There is a thread in the browser for page display, called UI thread , after submitting to the server, the UI thread clears the page and waits for feedback from the loading server. If the waiting time is too long, the page will be blank.
4.this
is mainly used in methods and represents the current object, that is, the direct caller of the method.
Two data types
1. JavaScript data types
String, Number, Math , Array, boolean, Date, Object, RegExp, null.
2. Variable definition
Global variables: Variables declared outside the function are global variables and are used throughout the page The range is valid. Assign a value to an undeclared variable, which is converted into a global variable.
# Local variables: Variables declared inside a function are local variables and are only valid inside the function.
# this: If this reference is used when declaring a variable inside a function, the variable can be referenced through an object outside the function.
3.String
Common methods:
length: Get the length of the string.
==: Use "==" in JS to compare whether two strings are equal.
indexOf(subStr): The index value of the first occurrence of the specified character.
lastIndexOf(subStr): The index value of the last occurrence of the specified character.
substr(begin[len]): Get the characters of the specified length starting from the specified position.
substring(begin[end]): Get the characters in the specified index range.
replace(regExp,substr): Replace characters that satisfy the format with specified characters.
concat(str01...): Concatenate multiple strings and return a new string.
split(delim[limit]): Split characters using the specified delimiter and return the split result in the form of an array. Limit is the number of splits, optional.
4.Number
## toFixed(n): Keep n decimals.
5.Math
- ## random(): Randomly returns a 0-1 number.
- round(x): Round.
- max(x...): Get the maximum value.
- min(x...): Get the minimum value.
- ## getTime(): Get from 1970-01-01 The number of milliseconds since.
Create:
var reg=/xxxx/;
var ret=new RegExp("");
常用方法:
test(str):判断字符串是否满足指定的格式。
8.Array
⑴数据类型
不同与java语言,JS中的数组在创建时不需要指定长度与数据类型,可以存储多种类型数据。
⑵创建(3种方式)
var arr01=[数组中的值];//创建时赋值 var arr01=new Array(数组中的值);//创建时赋值 //先声明,后赋值 var arr01=new Array(); arr01[0]=1; arr01[1]=2; var arr01=new Array(6);//创建时指定数组长度
⑶常用操作
arr[0]:获取指定索引位置的元素,也可以为指定索引位置赋值。
arr.length:获取数组长度。
push(data):向数组尾部添加元素并返回操作完成后的数组长度。
unshift(data):向数组头部添加一个元素并返回数组长度。
pop():删除数组的最后一个元素并返回删除的元素。
shift():删除并返回数组的第一个元素。
for(index in arr):遍历数组,arr[index]获取数组中的元素。
sort(function(a,b){return a-b;}):如果数组中存储的是字符,不需要指定函数,按照字母升序排序;如果数组中存储的是数字,需要设定排序函数,a-b升序排列,b-a降序排列。
reverse():反转排序。
join(connector):使用指定的连接符将数组连接成字符串,未指定连接符时默认采用","作为连接符。
toString():等效于join(),将数组转化为一个由","连接的字符串。
slice(begin,end):获取指定索引范围的元素,包含begin,不包含end。
splice(begin,howmany,newValue...):从指定索引位置(包含该位置)开始删除howwmany个元素,插入新的元素。
concat(anotherArr...):将参数数组的元素依次追加到该数组中并返回一个新的数组,原数组不变。
9.Object
⑴用于自定义对象,对象的创建方式:
//第一种方式,对象的声明与实例化一体 var obj={name:"张三",age:20,myFunction:function(){}}; //第二种方式,将函数当做构造方法 function person(name,age){ this.name=name; this.age=age; this.myfunction=function(){ alert(this.name); } } var tom=new function("tom",20); //第三种方式 person=function(){ this.name=name; this.age=age; this.myfunction:function(){ } } var tom=new function("tom",20);
⑵fn01:The meaning of fn02
Assign fn02 to fn01, fn01 has exactly the same content as fn02.
Three attributes
The user describes the characteristics of the object and instantiates the object.
Commonly used attributes:
href="#": Jump to the beginning of the page.
title is displayed directly as the text of the element. When the element cannot display text directly, it is displayed as a prompt message when the mouse stays above it.
Four window
The browser window object is a global object and the top-level object of all objects. Reference Properties or functions can be omitted.
1. Common attributes
document: an object representing all HTML elements in the window.
closed: boolean type, used to determine whether the current window has been closed.
Location: Get the URL of the current page; access the specified resource, which can be HTML, Servlet or other server resources. WhenOpen in the previous page. location=url has the same function as location.href=url.
History: An object representing the browsing history of the current window. On the premise of forming a browsing record, you can jump to the previous and next pages, history.go(-1) jumps to the previous page, and history.go(1) jumps to the next page.
parent: Indicates the parent window that contains the current window.
opener: Indicates opening the parent window of the current window, commonly used in parent-child window communication.
2. Commonly used methods:
## alert(content): Pop up a warning dialogue frame.
confirm(content): Pops up a confirmation dialog box and returns the confirmation result, true or false.
Prompt: Prompt dialog box, used for user input and return input results.
# setTimeout(): scheduled execution.
setInterval(): executed periodically.
open(): Load the specified URL in a new window.
close(): Close the current window.
parseInt(): Convert characters into int numbers.
eval: Parse ordinary strings into JS code.
typeof: Returns the type of data.
isNaN: Determine whether the data is a number.
五DOM
Document Object Model is a technology for operating documents, treating the document as a tree The object of hierarchical result arrangement, document is an object representing the document.
1. Element
Label node in the document.
Subnodes: element nodes, text nodes, comment nodes, excluding attribute nodes.
2. Create an object
document.createElement(tagName): Create an element based on the tag name. The tag as a parameter does not contain <>.
3. Get the object
document.all.id: Get the object based on the id.
formId.tagId: Get the elements under the form through id, or you can get the elements under the form through "formName.tagName".
document.getElementById(id): Get the object based on id.
document.getElementsByName(name): Get an object based on name. Because there may be multiple objects with the same name, the objects obtained are plural.
document.getElementsByTagName(tagName):通过标签名称获取对象。
4.属性
document.location:同document.location.href作用相同,用于访问指定资源,该资源可以是HTML,也可以是Servlet以及其他服务器资源,不能获取当前页面的URL。
5.常用方法
document.write(content):向页面输出。
六 函数
在JS中调用函数不必像在java中那样必须为每一个形参赋值,只需为需要的形参赋值即可,其他形参位可以空着。每一个函数都有其对应的作用范围,不同作用范围的函数定义在不同的节点上。
1.常用函数
javascript:void(0):保留超链接样式,只执行js代码,不进行页面跳转。
2.动态添加函数
动态地向元素添加函数有两种方式:
⑴使用JS:
使用JS获取对象以后,通过对象调用函数不包含括号),将自定义的函数function赋给该函数
obj.action=function(){};
⑵使用JQuery:
$(selector).action(function(){});
两种方式不能混用,即不能这样使用:$(selector).action=function(){}。
3.插入
java输出代码块与EL表达式都可以插入JavaScript代码中,插入时将其视作字符串处理,包裹在单引号或者双引号中,如:
alert("<%=name%>"); alert("${name}");
Seven Events
The behavior caused by the action is called an event. Multiple functions can be added to an event, and the multiple functions will be executed in sequence in the order of addition. Different elements correspond to different events .
1. Commonly used events:
onclick: Triggered when the mouse is clicked.
# onchange: Triggered when the options of the drop-down list box change.
onblur: triggered when focus is lost.
onfocus: Triggered when focus is obtained.
onmouseover: Triggered when the mouse moves over the element.
onmousemove: Triggered when the mouse moves on the element.
onmouseout: Triggered when the mouse leaves the element.
onselect: Triggered when the text field is selected.
Eight form submission
1. Button submission
Single form submission Click the submit button to submit the form.
2. Code submission
Execute the document.forms["formName"].submit() code and submit the form through the code.
Nine parent-child window communication
Parent-child window communication refers to a window transmitting information to the window that opens the window, that is, from the child window to the parent The window transmits information, and the transmission of information from the parent window to the child window can be completed through QueryString.
The key to the child window transmitting information to the parent window is to obtain the parent window object in the child window, obtain the parent window object through window.opener, and then use it as in the parent window.The same operation occurs in the window.
十table
1.Common operations on table objects
table.rows: Array object containing all rows in the table, table.rows.length gets the length, that is, the number of rows.
insertRow(index): Insert a row at the specified position. If the position is not specified, insert at the end.
deleteRow(index): Delete the row at the specified position. If the position is not specified, delete the last row.
2 Commonly used operations on tr objects
rowIndex: Get the index position, that is, the row number .
insertCell(index): Insert a column at the specified position. If not specified, insert at the end.
deleteCell(index): Delete the column at the specified position. If the position is not specified, delete the last column.
The above is the detailed content of JavaScript usage sharing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
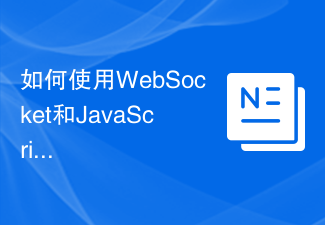
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
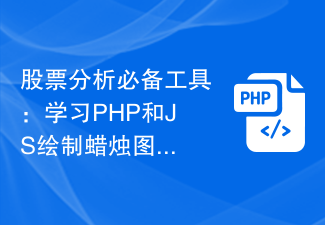
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
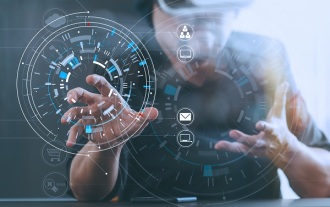
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
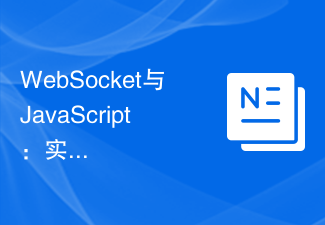
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
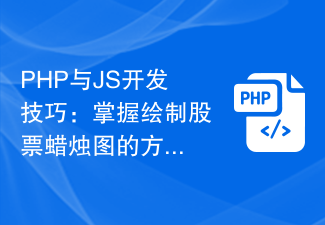
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
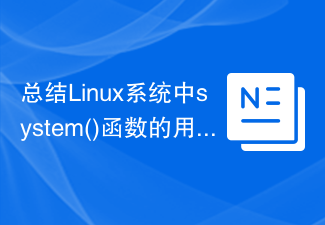
Summary of the system() function under Linux In the Linux system, the system() function is a very commonly used function, which can be used to execute command line commands. This article will introduce the system() function in detail and provide some specific code examples. 1. Basic usage of the system() function. The declaration of the system() function is as follows: intsystem(constchar*command); where the command parameter is a character.
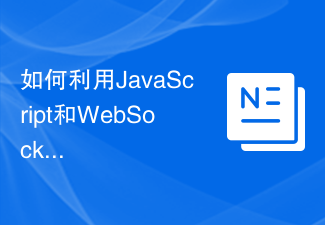
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
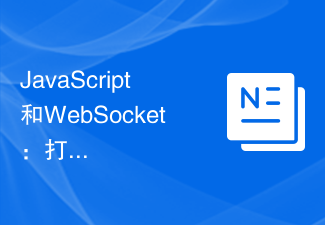
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
