Detailed explanation of how to use Java to query, modify and connect MySQL
This article mainly introduces how to connect, query and modify the MySQL database in Java. Friends in need can refer to
0. General process:
(1) Call the Class.forName() method to load the driver.
(2) Call the getConnection() method of the DriverManager object to obtain a Connection object.
(3) Create a Statement object and prepare a SQL statement. This SQL statement can be a Statement object (a statement to be executed immediately), a PreparedStatement statement (a precompiled statement) or a CallableStatement object (a stored procedure call). statement).
(4) Call methods such as excuteQuery() to execute SQL statements and save the results in the ResultSet object; or call methods such as executeUpdate() to execute SQL statements without returning the results of the ResultSet object.
(5) Perform display and other equivalent processing on the returned ResultSet object.
(6) Release resources.
1. Connect to the database
(1) Download the Mysql connection driver
After downloading, place it in F:\Doctoral Research Unzip it in Information\Database Learning\mysql related program files.
(2) Load the JDBC driver
Operation method: In Eclipse, select the corresponding project, click Java Build Path in Project-Properties, and add mysql-connector-java- in Libraries 5.1.21-bin.jar, click OK.
(3) Build a simple database as follows:
import java.sql.*; public class GetConnection { public static void main(String[] args){ try{ //调用Class.forName()方法加载驱动程序 Class.forName("com.mysql.jdbc.Driver"); System.out.println("成功加载MySQL驱动!"); }catch(ClassNotFoundException e1){ System.out.println("找不到MySQL驱动!"); e1.printStackTrace(); } String url="jdbc:mysql://localhost:3306/mysql"; //JDBC的URL //调用DriverManager对象的getConnection()方法,获得一个Connection对象 Connection conn; try { conn = DriverManager.getConnection(url, "root",""); //创建一个Statement对象 Statement stmt = conn.createStatement(); //创建Statement对象 System.out.print("成功连接到数据库!"); stmt.close(); conn.close(); } catch (SQLException e){ e.printStackTrace(); } } }
2. Query the data table
When querying a data table, you need to use the ResultSet interface, which is similar to a data table. Through the instance of this interface, you can obtain the retrieval result set and the interface information of the corresponding data table.
import java.sql.*; public class SelectTable { public static void main(String[] args){ try{ //调用Class.forName()方法加载驱动程序 Class.forName("com.mysql.jdbc.Driver"); System.out.println("成功加载MySQL驱动!"); String url="jdbc:mysql://localhost:3306/aniu"; //JDBC的URL Connection conn; conn = DriverManager.getConnection(url, "root",""); Statement stmt = conn.createStatement(); //创建Statement对象 System.out.println("成功连接到数据库!"); String sql = "select * from stu"; //要执行的SQL ResultSet rs = stmt.executeQuery(sql);//创建数据对象 System.out.println("编号"+"\t"+"姓名"+"\t"+"年龄"); while (rs.next()){ System.out.print(rs.getInt(1) + "\t"); System.out.print(rs.getString(2) + "\t"); System.out.print(rs.getInt(3) + "\t"); System.out.println(); } rs.close(); stmt.close(); conn.close(); }catch(Exception e) { e.printStackTrace(); } } }
3. Modify and delete the database
//修改删除数据 import java.sql.*; public class UpdateDeleteDemo { public static void main(String[] args)throws Exception{ try{ //调用Class.forName()方法加载驱动程序 Class.forName("com.mysql.jdbc.Driver"); System.out.println("成功加载MySQL驱动!"); String url="jdbc:mysql://localhost:3306/aniu"; //JDBC的URL Connection conn; conn = DriverManager.getConnection(url, "root",""); Statement stmt = conn.createStatement(); //创建Statement对象 System.out.println("成功连接到数据库!"); //查询数据的代码 String sql = "select * from stu"; //要执行的SQL ResultSet rs = stmt.executeQuery(sql);//创建数据对象 System.out.println("编号"+"\t"+"姓名"+"\t"+"年龄"); while (rs.next()){ System.out.print(rs.getInt(1) + "\t"); System.out.print(rs.getString(2) + "\t"); System.out.print(rs.getInt(3) + "\t"); System.out.println(); } //修改数据的代码 String sql2 = "update stu set name=? where number=?"; PreparedStatement pst = conn.prepareStatement(sql2); pst.setString(1,"8888"); pst.setInt(2,198); pst.executeUpdate(); //删除数据的代码 String sql3 = "delete from stu where number=?"; pst = conn.prepareStatement(sql3); pst.setInt(1,701); pst.executeUpdate(); ResultSet rs2 = stmt.executeQuery(sql);//创建数据对象 System.out.println("编号"+"\t"+"姓名"+"\t"+"年龄"); while (rs.next()){ System.out.print(rs2.getInt(1) + "\t"); System.out.print(rs2.getString(2) + "\t"); System.out.print(rs2.getInt(3) + "\t"); System.out.println(); } rs.close(); stmt.close(); conn.close(); }catch(Exception e) { e.printStackTrace(); } } }
The above is the detailed content of Detailed explanation of how to use Java to query, modify and connect MySQL. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


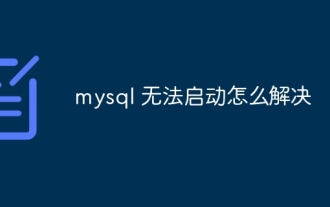
There are many reasons why MySQL startup fails, and it can be diagnosed by checking the error log. Common causes include port conflicts (check port occupancy and modify configuration), permission issues (check service running user permissions), configuration file errors (check parameter settings), data directory corruption (restore data or rebuild table space), InnoDB table space issues (check ibdata1 files), plug-in loading failure (check error log). When solving problems, you should analyze them based on the error log, find the root cause of the problem, and develop the habit of backing up data regularly to prevent and solve problems.
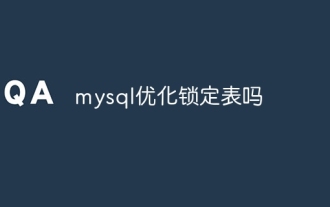
MySQL uses shared locks and exclusive locks to manage concurrency, providing three lock types: table locks, row locks and page locks. Row locks can improve concurrency, and use the FOR UPDATE statement to add exclusive locks to rows. Pessimistic locks assume conflicts, and optimistic locks judge the data through the version number. Common lock table problems manifest as slow querying, use the SHOW PROCESSLIST command to view the queries held by the lock. Optimization measures include selecting appropriate indexes, reducing transaction scope, batch operations, and optimizing SQL statements.
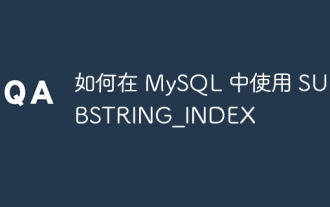
In MySQL database operations, string processing is an inevitable link. The SUBSTRING_INDEX function is designed for this, which can efficiently extract substrings based on separators. SUBSTRING_INDEX function application example The following example shows the flexibility and practicality of the SUBSTRING_INDEX function: Extract specific parts from the URL For example, extract domain name: SELECTSUBSTRING_INDEX('www.mysql.com','.',2); Extract file extension to easily get file extension: SELECTSUBSTRING_INDEX('file.pdf','.',-1); Processing does not exist
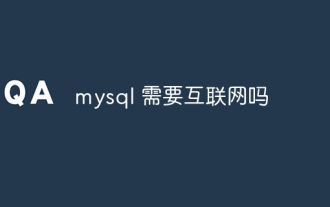
MySQL can run without network connections for basic data storage and management. However, network connection is required for interaction with other systems, remote access, or using advanced features such as replication and clustering. Additionally, security measures (such as firewalls), performance optimization (choose the right network connection), and data backup are critical to connecting to the Internet.
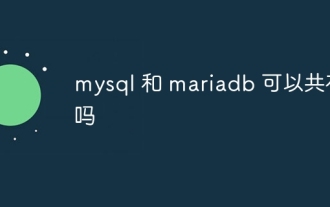
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
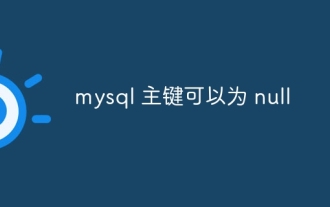
The MySQL primary key cannot be empty because the primary key is a key attribute that uniquely identifies each row in the database. If the primary key can be empty, the record cannot be uniquely identifies, which will lead to data confusion. When using self-incremental integer columns or UUIDs as primary keys, you should consider factors such as efficiency and space occupancy and choose an appropriate solution.
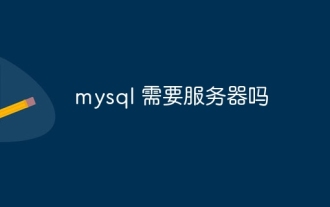
For production environments, a server is usually required to run MySQL, for reasons including performance, reliability, security, and scalability. Servers usually have more powerful hardware, redundant configurations and stricter security measures. For small, low-load applications, MySQL can be run on local machines, but resource consumption, security risks and maintenance costs need to be carefully considered. For greater reliability and security, MySQL should be deployed on cloud or other servers. Choosing the appropriate server configuration requires evaluation based on application load and data volume.
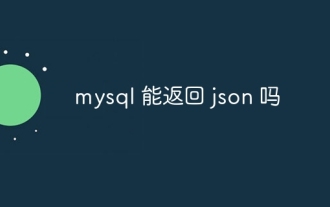
MySQL can return JSON data. The JSON_EXTRACT function extracts field values. For complex queries, you can consider using the WHERE clause to filter JSON data, but pay attention to its performance impact. MySQL's support for JSON is constantly increasing, and it is recommended to pay attention to the latest version and features.
