Summary of the eight types of operators in JavaScript
1. Unary operator
1. delete operator: deletes references to previously defined object properties or methods. For example:
var o=new Object; o.name="superman"; alert(o.name); //输出 "superman" delete o.name; alert(o.name); //输出 "undefined"
Remove the name attribute and set it to undefined (that is, the value of the uninitialized variable created). delete cannot delete properties and methods that are not defined by the developer (that is, defined by ECMAScript).
For example, the following code will cause an error: delete o.toString();
2. void operator: Returns undefined for any value. This operator is typically used to avoid outputting values that should not be output.
For example: in the HTML page Click Me
Click the link, the link in the webpage disappears, and the display "[object]" (in IE, "[object Window]" is displayed in Firefox, and the link is still the same in Google). Because the window.open() method returns a reference to the newly opened window. The object is then converted into a string to be displayed. To avoid this result, you can use the void operator to call the window.open() function:
<a href="javascript:void(window.open('about:blank'))">Click Me</a>
This causes the window.open() call to return undefined, which is not a valid value and will not be displayed in the browser in the browser window.
3. Pre-increment/pre-decrement operator: The usage is the same as in C. Operations occur before expressions are evaluated. Such as: ++i; --i.
4. Post-increment/post-decrement operator: Postfix operators perform increment or decrement operations after calculating the expressions containing them. Such as: i++; i--.
5. One-yuan addition and one-yuan subtraction
One-yuan addition essentially has no effect on numbers: var iNum=25; iNum=+iNum; alert(iNum); //Output 25
One-yuan addition When the operator operates on a string, the way it calculates the string is similar to parseInt(). The main difference is that for strings starting with "0x" (representing hexadecimal numbers), unary operators can Convert to decimal value. Therefore, converting "010" using unary addition will always result in 10, while "0xB" will be converted to 11.
var sNum="25"; alert(typeof sNum); //Output "string"
var iNum=+sNum; alert(typeof iNum); //Output "number"
Uniary subtraction is the right Negate a numerical value. Similar to the unary addition operator, the unary subtraction operator will also convert a string into an approximate number and will also negate the value.
var sNum = "25"; alert(typeof sNum); //Output "string"
var iNum = -sNum; alert(iNum); //Output "-25"
alert(typeof iNum ); //Output "number"
2. Bit operators: a series of operators related to binary
The bit operator NOT consists of the negation sign (~) express.
The bit operator AND is represented by the ampersand (&).
The bitwise operator OR is represented by the symbol (|).
The bitwise operator XOR is represented by the symbol (^).
The left shift operation is represented by two less than signs (<<).
The signed right shift operator is represented by two greater than signs (>>).
Unsigned right shift is represented by three greater than signs (>>>).
3. Boolean operators
There are three types of Boolean operators, namely NOT, AND and OR.
1. Logical NOT, represented by an exclamation point (!). This operator is typically used to control loops. Unlike the logical OR and logical AND operators, the logical NOT operator must return a Boolean value. The behavior of the logical NOT operator is as follows:
If the operand is an object, return false.
If the operand is the number 0, return true.
If the operand is any number other than 0, return false.
If the operand is null, return true.
If the operand is NaN, return true.
If the operand is undefined, an error occurs.
Example: var b = true;
while(!b){……}
2. Logical AND operator, represented by double ampersand (&&). There is only one situation when the result is true: true && true = true;
The operand of the logical AND operation can be of any type, not just Boolean values. If an operand is not a primitive Boolean value, the logical AND operation does not necessarily return a Boolean value:
If one operand is an object and the other is a Boolean value, return the object.
If both operands are objects, return the second object.
If an operand is null, return null.
If an operand is NaN, return NaN.
If an operand is undefined, an error occurs.
If the first operand is false, then no matter what the value of the second operand is, the result cannot be true.
3. Logical OR operator, represented by double vertical bars (||). There is only one case where the result is false: false || false=false; other cases are true.
Similar to the logical AND operator, if an operand is not a Boolean value, the logical OR operation does not necessarily return a Boolean value:
If one operand is an object and the other is a Boolean value, the object is returned.
If both operands are objects, return the first object.
If an operand is null, return null.
If an operand is NaN, return NaN.
If an operand is undefined, an error occurs.
Logical OR is also a simple operation. For the logical OR operator, if the first operand value is true, the second operand will not be calculated.
4. Multiplicative operator
1. The multiplication operator, represented by an asterisk (*), is used to multiply two numbers. But when dealing with special values, multiplication in ECMAScript also has some special behaviors:
If the operands are all numbers, perform regular multiplication operations. If the result is too large or too small, the generated result is Infinity or -Infinity. .
If an operand is NaN, the result is NaN.
Infinity multiplied by 0, the result is NaN.
Infinity multiplied by any number other than 0, the result is Infinity or -Infinity, determined by the sign of the second operand.
2. The division operator, represented by a slash (/), divides the first number by the second number. For special values, the special behavior is as follows:
If the operands are all numbers, perform regular trigger operations. If the result is too large or too small, the generated result is Infinity or -Infinity.
If an operand is NaN, the result is NaN.
0 divides a non-infinity number, the result is NaN.
Infinity is divided by any number other than 0, and the result is Infinity or -Infinity, determined by the sign of the second operand.
3. Modulo operator, expressed with percent sign (%). If the operands are both numbers, perform regular arithmetic division and return the remainder. Special behavior:
If the dividend is Infinity, or the divisor is 0, the result is NaN.
If the divisor is an infinite number, the result is the dividend.
If the dividend is 0, the result is 0.
5. Additive operator
1. Addition operator (+), special behavior:
A certain operand is NaN, and the result is NaN.
Infinity Add -Infinity, the result is NaN.
If an operand is a string, then the following rules apply:
If both operands are strings, concatenate the second string to the first string.
If only one operand is a string, convert the other operand into a string, and the result is a string concatenated by the two strings.
2. Subtraction operator (-), if both operands are numbers, arithmetic subtraction will be performed. Special rules:
If the operand is NaN, the result is NaN.
An operand is not a number, and the result is NaN.
六、关系运算符
关系运算符小于、大于、小于等于和大于等于执行的是两个数的比较运算,比较方式与算术比较运算相同。每个关系运算符都返回一个Boolean值。
对于字符串,第一个字符串中每个字符的代码都会与第二个字符串中对应位置上的字符的代码进行数值比较。
1、大写字母的代码都小于小写字母的代码,所以要得到按照真正的字母顺序比较结果,必须把两个运算数转换成相同的大小写形式,再进行比较。
2、在比较两个字符串形式的数字时,比较的是它们的字符代码。
3、比较一个字符串和数字,ECMAScript都会把字符串转换为数字,然后按照数字顺序比较它们。如果字符串不能转换成数字,那么返回NaN,任何包含NaN的关系运算都要返回false。因此,这样会返回false。
七、等性运算符
1、等号(==) 和非等号(!=),为确定两个运算数是否相等,这两个运算符都会进行类型转换。执行类型转换的基本规则如下:
如果一个运算数是Boolean值,在检查相等性之前,把它转换成数字值。False转换成0,true转换成1。
如果一个运算数是字符串,另一个是数字,在检查相等性之前,要尝试把字符串转换成数字。
如果一个运算数是对象,另一个是字符串,在检查等性之前,要尝试把对象转换成字符串(调用toString()方法)。
如果一个运算数是对象,另一个是数字,在检查等性之前,要尝试把对象转换成数字。
在进行比较时,该运算符还遵守下列规则:
值null和undefined相等。
在检查相等性时,不能把null和undefined转换成其他值。
如果某个运算数是NaN,等号将返回false,非等号将返回true。即使两个运算数都是NaN,等号仍然返回false,因为根据规则,NaN不等于NaN。
如果两个运算数都是对象,那么比较的是他们的引用值。如果两个运算数指向同一个对象,那么等号返回true,否则两个运算数不等。
2、全等号和非全等号
等号和非等号的同类运算符是全等号和非全等号。这两个运算符所做的与等号和非等号相同,只是他们在检查相等性前,不执行类型转换。
全等号由三个等号(===)表示,只有在无需类型转换运算数就相等的情况下,才返回true。
var sNum="55"; var iNum=55; alert(sNum==iNum); //输出 "true" alert(sNum===iNum); //输出 "false"
非全等号由两个感叹号加两个等号(!==)表示,只有在无需类型转换运算数不相等的情况下,才返回true。
var sNum="55"; var iNum=55; alert(sNum != iNum); //输出 "false" alert(sNum !== iNum); //输出 "true"
八、其他运算符
1、条件运算符,即三元运算符 : variable = boolean_expression ? true_value : false_value;
2、赋值运算符(=) 复合赋值运算符:+=、-=、*=、/=、%=、<<=、>>=、>>>=
3、逗号运算符 用逗号运算符可以在一条语句中执行多个运算。 如: var iNum1=1,iNum2=2;
The above is the detailed content of Summary of the eight types of operators in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


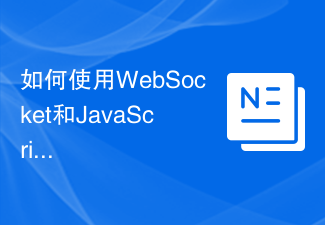
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
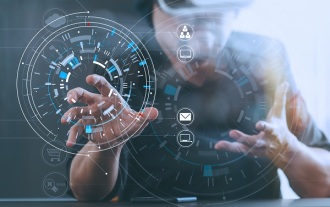
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
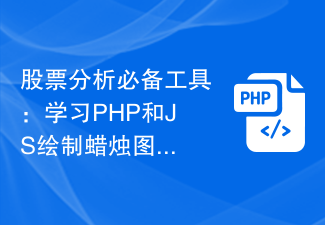
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
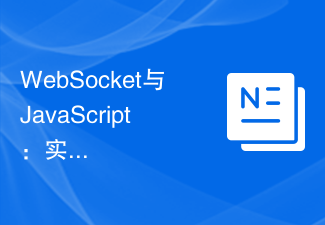
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
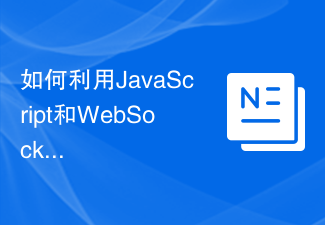
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
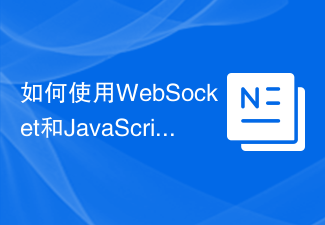
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
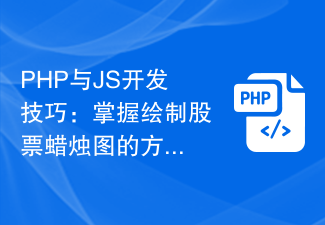
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
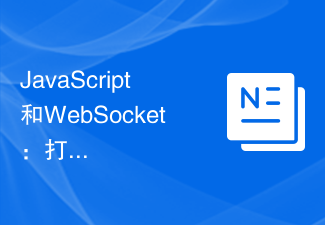
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
