Summary and detailed explanation of this pointer in javaScript
The following editor will bring you a summary of this pointing based on javaScript. The editor thinks it’s pretty good, so I’ll share it with you now and give it as a reference. Let’s follow the editor and take a look.
The pointing of this in JavaScript has always been a worry for front-end colleagues, and it is also the first choice for interview questions. Now let’s summarize the pointing of this in js. First, you need to understand a few concepts:
1: Global variables are mounted under the window object by default
2 : Generally, this points to its caller
3: In the arrow function of es6, this points to the creator, not the caller
4: You can change the pointer of this through call, apply, and bind
Let’s analyze it in detail
1: When calling the function
## (non-strict mode)
const func = function () { console.log(this); const func2 = function () { console.log(this); }; func2(); //Window }; func(); //Window
(strict mode)
'use strict' const func = function () { console.log(this); const func2 = function () { console.log(this); }; func2(); //undefined }; func(); //undefined
2: As an object method
const user = { userName: '小张', age: 18, selfIntroduction: function () { const str = '我的名字是:' + this.userName + ",年龄是:" + this.age; console.log(str); const loop = function () { console.log('我的名字是:' + this.userName + ",年龄是:" + this.age); }; loop(); //我的名字是:undefined,年龄是:undefined } }; user.selfIntroduction(); //我的名字是:小张,年龄是:18
const user = { userName: '小张', age: 18, selfIntroduction: function () { const str = '我的名字是:' + this.userName + ",年龄是:" + this.age; console.log(str); const that=this; const loop = function () { console.log('我的名字是:' + that.userName + ",年龄是:" + that.age); }; loop(); //我的名字是:小张,年龄是:18 } }; user.selfIntroduction(); //我的名字是:小张,年龄是:18
const user={ userName:'小张', age:18, selfIntroduction:function(){ const str='我的名字是:'+this.userName+",年龄是:"+this.age; console.log(str); } }; const other =user.selfIntroduction; other(); //我的名字是:undefined,年龄是:undefined const data={ userName:'小李', age:19, }; data.selfIntroduction=user.selfIntroduction; data.selfIntroduction(); //我的名字是:小李,年龄是:19
3: Triggered as an event in html
<body> <p id="btn">点击我</p> </body>
const btn=document.getElementById('btn'); btn.addEventListener('click',function () { console.log(this); //<p id="btn">点击我</p> })
4: new keyword (constructor)
##
const fun=function(userName){ this.userName=userName; } const user=new fun('郭德纲'); console.log(user.userName); //郭德纲
I won’t go into details on this. The new keyword constructs an object instance and assigns it to user, so userName becomes an attribute of the user object.
5:es6 (arrow function)
const func1=()=>{ console.log(this); }; func1(); //Window
const data={ userName:'校长', selfIntroduction:function(){ console.log(this); //Object {userName: "校长", selfIntroduction: function} const func2=()=>{ console.log(this); //Object {userName: "校长", selfIntroduction: function} } func2(); } } data.selfIntroduction();
Everyone is looking at the third rule I mentioned at the beginning: In the arrow function of es6, this points to the creator, not the caller. fun1 is created under the global function, so this points to the global window, and fun2 is created under the object data. this points to the data object, so inside the func2 function this points to the data object. I personally think that the this point of the es6 arrow function is an improvement on the JavaScript design flaws I mentioned above (personal knowledge).
#6: Change the point of this The three functions call, apply, and bind can artificially change the point of this of the function. I won’t go into detail about the differences between the three here. I will explain the differences between the three in detail in future blogs. Now let’s take one as an example
const func=function(){ console.log(this); }; func(); //window func.apply({userName:"郭德纲"}); //Object {userName: "郭德纲"}
These three methods can artificially change the pointer of this. The difference is that call and apply will bind the method to this. It is executed immediately afterwards, and the bind method returns an executable function.
To sum up a lot of this, it is the 4 points I mentioned at the beginning
1: Global variables are mounted by default in Under the window object
2: Generally this points to its caller 3: In the arrow function of es6, this points to the creator, not the caller 4: Through call, apply, Bind can change the pointer of this To be honest, it is my first time to write a blog. I am really nervous. Will anyone read my blog? Could it be written incorrectly? ...I have thought a lot better and have summarized: I welcome corrections on any shortcomings.
The above is the detailed content of Summary and detailed explanation of this pointer in javaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


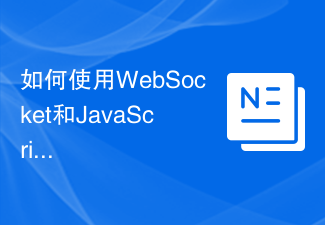
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
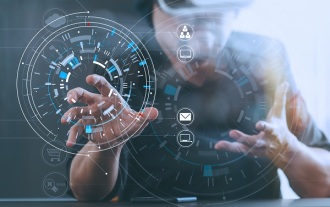
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
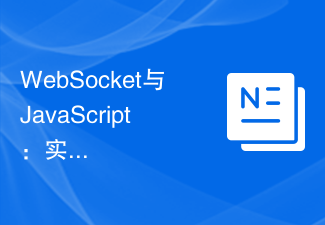
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
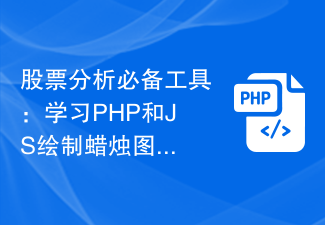
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
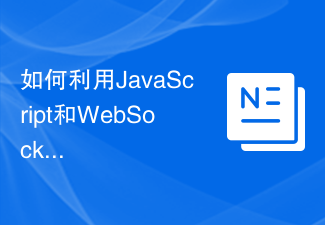
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
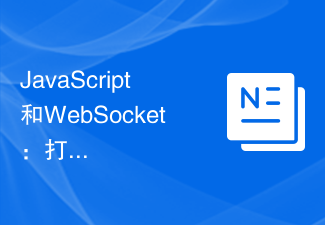
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
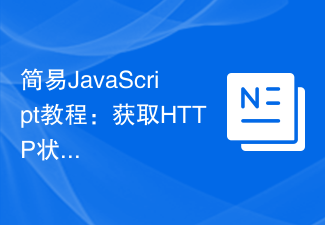
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
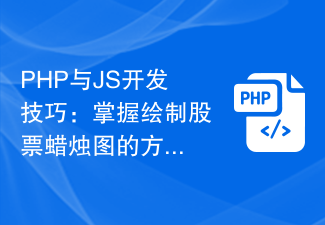
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
