Specific analysis of prototype pattern in Java design patterns
This article mainly introduces the prototype pattern of design patterns. The editor thinks it is quite good. Now I will share it with you and give you a reference. Let’s follow the editor and take a look.
Definition: Use prototype instances to specify the types of objects to be created, and create new objects by copying these prototypes.
Type: Create a class pattern
Class diagram:
The prototype mode is mainly used for copying objects. Its core is the prototype class Prototype in the class diagram. The Prototype class needs to meet the following two conditions:
Implement the Cloneable interface. There is a Cloneable interface in the Java language, which has only one function, which is to notify the virtual machine at runtime that it is safe to use the clone method on classes that implement this interface. In the Java virtual machine, only classes that implement this interface can be copied, otherwise a CloneNotSupportedException will be thrown at runtime.
Override the clone method in the Object class. In Java, the parent class of all classes is the Object class. There is a clone method in the Object class, which is used to return a copy of the object. However, its scope is protected and cannot be called by ordinary classes. Therefore, the Prototype class needs to clone The scope of the method is changed to the public type.
The prototype pattern is a relatively simple pattern and very easy to understand. Implementing an interface and rewriting a method completes the prototype pattern. In practical applications, prototype patterns rarely appear alone. Often mixed with other patterns, its prototype class Prototype is often replaced by abstract classes.
Implementation code:
##
class Prototype implements Cloneable { public Prototype clone(){ Prototype prototype = null; try{ prototype = (Prototype)superclone(); }catch(CloneNotSupportedException e){ e.printStackTrace(); } return prototype; } } class ConcretePrototype extends Prototype{ public void show(){ System.out.println("原型模式实现类"); } } public class Client { public static void main(String[] args){ ConcretePrototype cp = new ConcretePrototype(); for(int i=0; i< 10; i++){ ConcretePrototype clonecp = (ConcretePrototype)cpclone(); clonecp.show(); } } }
Advantages and applicable scenarios of prototype mode
Notes on prototype mode
- Copying an object using prototype mode will not call the constructor of the class. Because the copy of the object is completed by calling the clone method of the Object class, it copies the data directly in the memory, so the constructor of the class is not called. Not only will the code in the constructor not be executed, but even the access permissions will be invalid for prototype mode. Remember the singleton pattern? In the singleton mode, as long as the access permission of the constructor is set to private, a singleton can be implemented. However, the clone method directly ignores the permissions of the constructor method. Therefore, the singleton mode conflicts with the prototype mode, so special attention should be paid when using it.
- Deep copy and shallow copy. The clone method of the Object class will only copy the basic data types in the object. Arrays, container objects, reference objects, etc. will not be copied. This is a shallow copy. If you want to implement deep copy, you must copy the array, container object, reference object, etc. in the prototype mode separately. For example:
public class Prototype implements Cloneable { private ArrayList list = new ArrayList(); public Prototype clone(){ Prototype prototype = null; try{ prototype = (Prototype)superclone(); prototype.list = (ArrayList) this.list.clone(); }catch(CloneNotSupportedException e){ e.printStackTrace(); } return prototype; } }
The above is the detailed content of Specific analysis of prototype pattern in Java design patterns. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


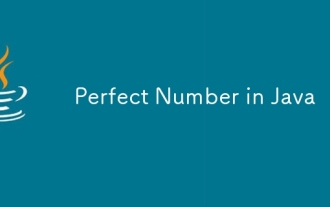
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
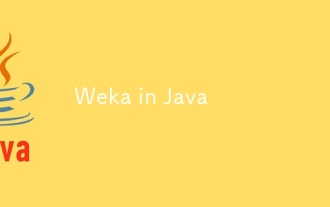
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
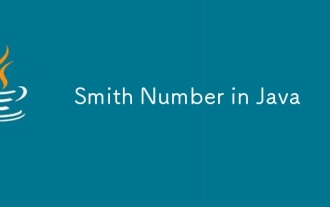
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
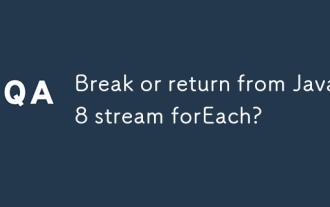
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
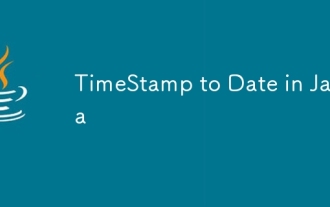
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
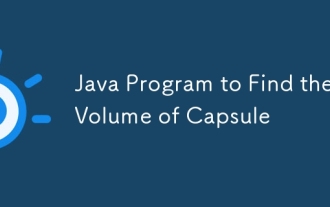
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
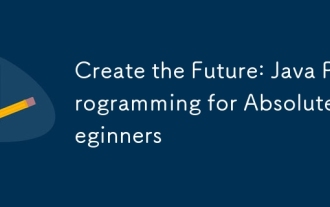
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
