Examples of image processing and base64 encoding conversion in Java
This article mainly introduces examples of conversion between Java processing images and base64 encoding. It has certain reference value. Those who are interested can learn about it
Today’s project has optimized the function of uploading avatars. Use the imagecropper plug-in to achieve the effect of cropping images.
The images cropped by this plug-in are all base64 encrypted strings. Uploading avatars also involves the issue of how to convert the encrypted strings into images.
The following is the code:
/** * @Description: 将base64编码字符串转换为图片 * @Author: * @CreateTime: * @param imgStr base64编码字符串 * @param path 图片路径-具体到文件 * @return */ public static boolean generateImage(String imgStr, String path) { if (imgStr == null) return false; BASE64Decoder decoder = new BASE64Decoder(); try { // 解密 byte[] b = decoder.decodeBuffer(imgStr); // 处理数据 for (int i = 0; i < b.length; ++i) { if (b[i] < 0) { b[i] += 256; } } OutputStream out = new FileOutputStream(path); out.write(b); out.flush(); out.close(); return true; } catch (Exception e) { return false; } }
Decryption naturally also involves encryption. The following is the encryption:
/** * @Description: 根据图片地址转换为base64编码字符串 * @Author: * @CreateTime: * @return */ public static String getImageStr(String imgFile) { InputStream inputStream = null; byte[] data = null; try { inputStream = new FileInputStream(imgFile); data = new byte[inputStream.available()]; inputStream.read(data); inputStream.close(); } catch (IOException e) { e.printStackTrace(); } // 加密 BASE64Encoder encoder = new BASE64Encoder(); return encoder.encode(data); }
Post a test main function
/** * 示例 */ public static void main(String[] args) { String strImg = getImageStr("F:/86619-106.jpg"); System.out.println(strImg); generateImage(strImg, "F:/86619-107.jpg"); }
Okay, let’s call it a day.
However, it should be noted that the base64-encoded string returned by the general plug-in has a prefix.
"data:image/jpeg;base64," This must be removed before decoding.
The above is the detailed content of Examples of image processing and base64 encoding conversion in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


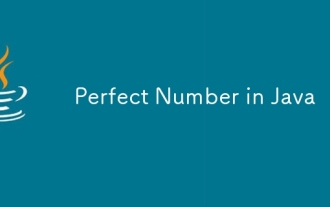
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
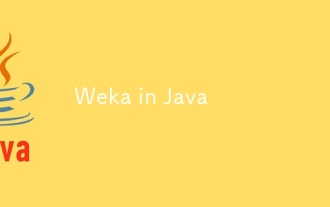
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
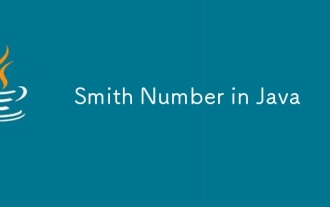
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
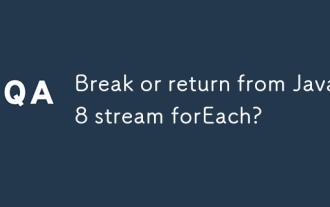
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
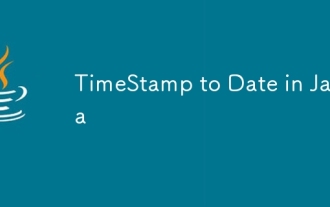
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
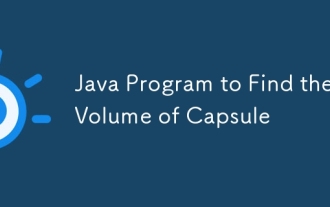
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
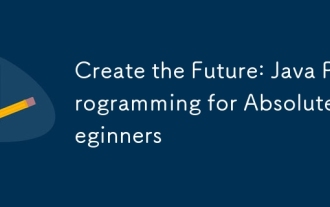
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
