Detailed examples of iterators and generators in Python
Iterator
Iterable
Definition
class Iterable(metaclass=ABCMeta): __slots__ = () @abstractmethod def __iter__(self): while False: yield None @classmethod def __subclasshook__(cls, C): if cls is Iterable: if any("__iter__" in B.__dict__ for B in C.__mro__): return True return NotImplemented
It can be seen from the definition that Iterable
must contain __iter__
Function
Iterator
Definition
class Iterator(Iterable): __slots__ = () @abstractmethod def __next__(self): 'Return the next item from the iterator. When exhausted, raise StopIteration' raise StopIteration def __iter__(self): return self @classmethod def __subclasshook__(cls, C): if cls is Iterator: if (any("__next__" in B.__dict__ for B in C.__mro__) and any("__iter__" in B.__dict__ for B in C.__mro__)): return True return NotImplemented
It can be seen from the definition that Iterator
contains__next__
And __iter__
function, when next exceeds the scope, it will throw StopIteration
event
type relationship
#! /usr/bin/python #-*-coding:utf-8-*- from collections import Iterator,Iterable # 迭代器 s = 'abc' l = [1,2,3] d=iter(l) print(isinstance(s,Iterable)) # True print(isinstance(l,Iterable)) # True print(isinstance(s,Iterator)) # False print(isinstance(l,Iterator)) # False print(isinstance(d,Iterable)) # True print(isinstance(d,Iterator)) # True
Theoretically you You can use next()
to execute __next__()
until the iterator throws StopIteration
Actually the system provides for .. in ..
way to parse iterator
l = [1,2,3,4] for i in l: print(i) # 执行结果 # 1 # 2 # 3 # 4
generatorgenerator
The essence of the generator is an iterator
#! /usr/bin/python #-*-coding:utf-8-*- from collections import Iterator,Iterable s = (x*2 for x in range(5)) print(s) print('Is Iterable:' + str(isinstance(s,Iterable))) print('Is Iterator:' + str(isinstance(s,Iterator))) for x in s: print(x) # 执行结果 # <generator object <genexpr> at 0x000001E61C11F048> # Is Iterable:True # Is Iterator:True # 0 # 2 # 4 # 6 # 8
function If yield
exists, the function is a generator object. Each time the next
function is executed, the function will start executing at the previous yield
. And return at the next yield
(equivalent to return
)
def foo(): print("First") yield 1 print("Second") yield 2 f = foo() print(f) a = next(f) print(a) b = next(f) print(b) # <generator object foo at 0x0000020B697F50F8> # First # 1 # Second # 2
Example
#! /usr/bin/python #-*-coding:utf-8-*- def add(s,x): return s+x def gen(): for i in range(4): yield i base = gen() # 由于gen函数中存在yield,所以 # for 循环本质是创建了两个generator object,而非执行函数 # base = (add(i,10) for i in base) # base = (add(i,10) for i in base) for n in [1,10]: base = (add(i,n) for i in base) # 这里才开始展开生成器 # 第一个生成器展开 # base = (add(i,10) for i in base) # base = (add(i,10) for i in range(4)) # base = (10,11,12,13) # # 第二个生成器展开 # base = (add(i,10) for i in (10,11,12,13)) # base = (20,21,22,23) print(list(base)) # [20,21,22,23]
The above is the detailed content of Detailed examples of iterators and generators in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
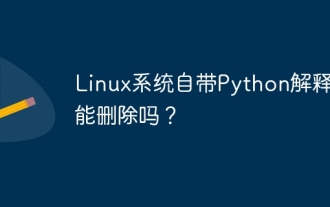
Regarding the problem of removing the Python interpreter that comes with Linux systems, many Linux distributions will preinstall the Python interpreter when installed, and it does not use the package manager...
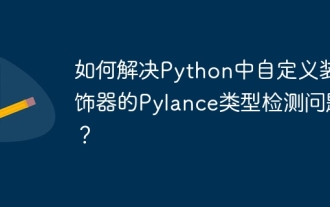
Pylance type detection problem solution when using custom decorator In Python programming, decorator is a powerful tool that can be used to add rows...
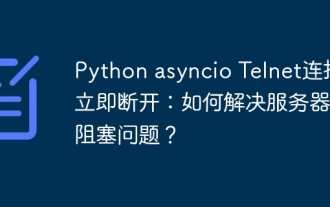
About Pythonasyncio...
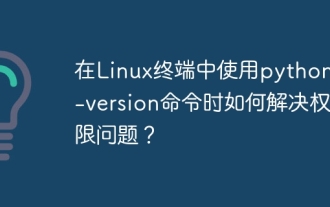
Using python in Linux terminal...
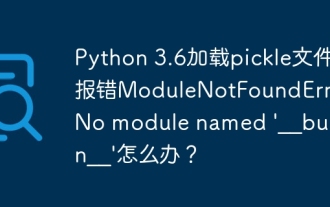
Loading pickle file in Python 3.6 environment error: ModuleNotFoundError:Nomodulenamed...
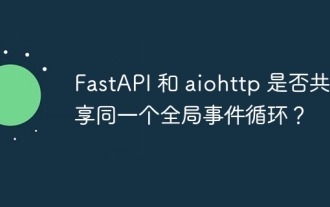
Compatibility issues between Python asynchronous libraries In Python, asynchronous programming has become the process of high concurrency and I/O...
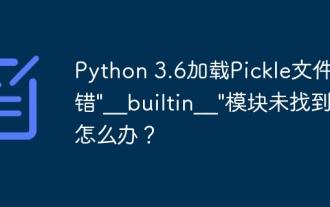
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...
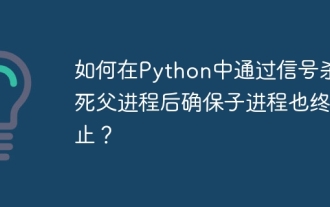
The problem and solution of the child process continuing to run when using signals to kill the parent process. In Python programming, after killing the parent process through signals, the child process still...
