


Analyze express middleware mechanism and its implementation principle
This article mainly introduces the express middleware mechanism and implementation principle. The editor thinks it is quite good. Now I will share it with you and give it as a reference. Let’s follow the editor to take a look
Introduction
The middleware mechanism allows us to add a processing step to a given process, thereby The input or output of this process has an impact, or produces some intermediate effects, states, or intercepts this process. The middleware mechanism is similar to tomcat's filter, both of which are specific implementations of the chain of responsibility model.
express middleware use case
let express = require('express') let app = express() //解析request 的body app.use(bodyParser.json()) //解析 cookie app.use(cookieParser()) //拦截 app.get('/hello', function (req, res) { res.send('Hello World!'); });
Simulate the middleware mechanism and simulate the middle of parsing the request File
First simulate a request
request = { //模拟的request requestLine: 'POST /iven_ HTTP/1.1', headers: 'Host:www.php.cn\r\nCookie:BAIDUID=E063E9B2690116090FE24E01ACDDF4AD:FG=1;BD_HOME=0', requestBody: 'key1=value1&key2=value2&key3=value3', }
A http
request is divided into The request line, request header, and request body are separated by \r\n\r\n
, that is, a blank line. It is assumed that the three have been separated, requestLine
(request line) contains method type, request url, and http version number. These three are distinguished by spaces. Each part of headers
(request header) is separated by \r\n
to separate, requestBody
(request body) uses & to distinguish parameters
Simulation middleware mechanism
The agreed middleware must be A function that accepts three parameters: request, response, next
function App() { if (!(this instanceof App)) return new App(); this.init(); } App.prototype = { constructor: App, init: function() { this.request = { //模拟的request requestLine: 'POST /iven_ HTTP/1.1', headers: 'Host:www.php.cn\r\nCookie:BAIDUID=E063E9B2690116090FE24E01ACDDF4AD:FG=1;BD_HOME=0', requestBody: 'key1=value1&key2=value2&key3=value3', }; this.response = {}; //模拟的response this.chain = []; //存放中间件的一个数组 this.index = 0; //当前执行的中间件在chain中的位置 }, use: function(handle) { //这里默认 handle 是函数,并且这里不做判断 this.chain.push(handle); }, next: function() { //当调用next时执行index所指向的中间件 if (this.index >= this.chain.length) return; let middleware = this.chain[this.index]; this.index++; middleware(this.request, this.response, this.next.bind(this)); }, }
Middleware for request processing
function lineParser(req, res, next) { let items = req.requestLine.split(' '); req.methond = items[0]; req.url = items[1]; req.version = items[2]; next(); //执行下一个中间件 } function headersParser(req, res, next) { let items = req.headers.split('\r\n'); let header = {} for(let i in items) { let item = items[i].split(':'); let key = item[0]; let value = item[1]; header[key] = value; } req.header = header; next(); //执行下一个中间件 } function bodyParser(req, res, next) { let bodyStr = req.requestBody; let body = {}; let items = bodyStr.split('&'); for(let i in items) { let item = items[i].split('='); let key = item[0]; let value = item[1]; body[key] = value; } req.body = body; next(); //执行下一个中间件 } function middleware3(req, res, next) { console.log('url: '+req.url); console.log('methond: '+req.methond); console.log('version: '+req.version); console.log(req.body); console.log(req.header); next(); //执行下一个中间件 }
Test code
##
let app = App(); app.use(lineParser); app.use(headersParser); app.use(bodyParser); app.use(middleware3); app.next();
Overall code
function App() { if (!(this instanceof App)) return new App(); this.init(); } App.prototype = { constructor: App, init: function() { this.request = { //模拟的request requestLine: 'POST /iven_ HTTP/1.1', headers: 'Host:www.php.cn\r\nCookie:BAIDUID=E063E9B2690116090FE24E01ACDDF4AD:FG=1;BD_HOME=0', requestBody: 'key1=value1&key2=value2&key3=value3', }; this.response = {}; //模拟的response this.chain = []; //存放中间件的一个数组 this.index = 0; //当前执行的中间件在chain中的位置 }, use: function(handle) { //这里默认 handle 是函数,并且这里不做判断 this.chain.push(handle); }, next: function() { //当调用next时执行index所指向的中间件 if (this.index >= this.chain.length) return; let middleware = this.chain[this.index]; this.index++; middleware(this.request, this.response, this.next.bind(this)); }, } function lineParser(req, res, next) { let items = req.requestLine.split(' '); req.methond = items[0]; req.url = items[1]; req.version = items[2]; next(); //执行下一个中间件 } function headersParser(req, res, next) { let items = req.headers.split('\r\n'); let header = {} for(let i in items) { let item = items[i].split(':'); let key = item[0]; let value = item[1]; header[key] = value; } req.header = header; next(); //执行下一个中间件 } function bodyParser(req, res, next) { let bodyStr = req.requestBody; let body = {}; let items = bodyStr.split('&'); for(let i in items) { let item = items[i].split('='); let key = item[0]; let value = item[1]; body[key] = value; } req.body = body; next(); //执行下一个中间件 } function middleware3(req, res, next) { console.log('url: '+req.url); console.log('methond: '+req.methond); console.log('version: '+req.version); console.log(req.body); console.log(req.header); next(); //执行下一个中间件 } let app = App(); app.use(lineParser); app.use(headersParser); app.use(bodyParser); app.use(middleware3); app.next();
Run result
##
url: /iven_ methond: POST version: HTTP/1.1 {key1: "value1", key2: "value2", key3: "value3"} {Host: "www.php.cn", Cookie: "BAIDUID=E063E9B2690116090FE24E01ACDDF4AD"}
The above is the detailed content of Analyze express middleware mechanism and its implementation principle. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










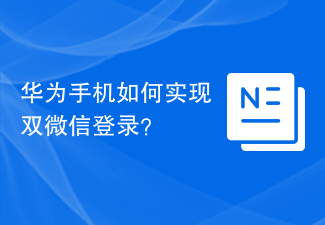
How to implement dual WeChat login on Huawei mobile phones? With the rise of social media, WeChat has become one of the indispensable communication tools in people's daily lives. However, many people may encounter a problem: logging into multiple WeChat accounts at the same time on the same mobile phone. For Huawei mobile phone users, it is not difficult to achieve dual WeChat login. This article will introduce how to achieve dual WeChat login on Huawei mobile phones. First of all, the EMUI system that comes with Huawei mobile phones provides a very convenient function - dual application opening. Through the application dual opening function, users can simultaneously
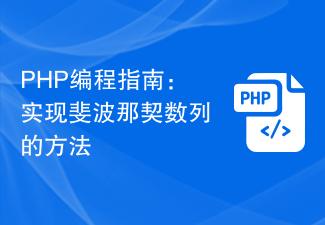
The programming language PHP is a powerful tool for web development, capable of supporting a variety of different programming logics and algorithms. Among them, implementing the Fibonacci sequence is a common and classic programming problem. In this article, we will introduce how to use the PHP programming language to implement the Fibonacci sequence, and attach specific code examples. The Fibonacci sequence is a mathematical sequence defined as follows: the first and second elements of the sequence are 1, and starting from the third element, the value of each element is equal to the sum of the previous two elements. The first few elements of the sequence
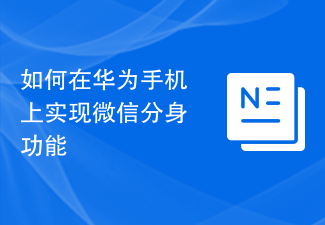
How to implement the WeChat clone function on Huawei mobile phones With the popularity of social software and people's increasing emphasis on privacy and security, the WeChat clone function has gradually become the focus of people's attention. The WeChat clone function can help users log in to multiple WeChat accounts on the same mobile phone at the same time, making it easier to manage and use. It is not difficult to implement the WeChat clone function on Huawei mobile phones. You only need to follow the following steps. Step 1: Make sure that the mobile phone system version and WeChat version meet the requirements. First, make sure that your Huawei mobile phone system version has been updated to the latest version, as well as the WeChat App.
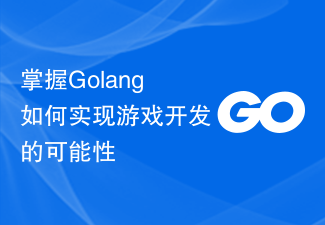
In today's software development field, Golang (Go language), as an efficient, concise and highly concurrency programming language, is increasingly favored by developers. Its rich standard library and efficient concurrency features make it a high-profile choice in the field of game development. This article will explore how to use Golang for game development and demonstrate its powerful possibilities through specific code examples. 1. Golang’s advantages in game development. As a statically typed language, Golang is used in building large-scale game systems.
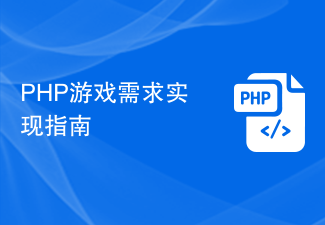
PHP Game Requirements Implementation Guide With the popularity and development of the Internet, the web game market is becoming more and more popular. Many developers hope to use the PHP language to develop their own web games, and implementing game requirements is a key step. This article will introduce how to use PHP language to implement common game requirements and provide specific code examples. 1. Create game characters In web games, game characters are a very important element. We need to define the attributes of the game character, such as name, level, experience value, etc., and provide methods to operate these
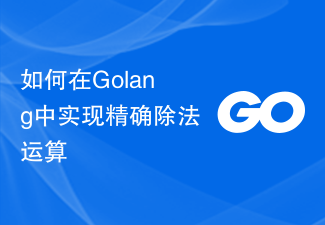
Implementing exact division operations in Golang is a common need, especially in scenarios involving financial calculations or other scenarios that require high-precision calculations. Golang's built-in division operator "/" is calculated for floating point numbers, and sometimes there is a problem of precision loss. In order to solve this problem, we can use third-party libraries or custom functions to implement exact division operations. A common approach is to use the Rat type from the math/big package, which provides a representation of fractions and can be used to implement exact division operations.
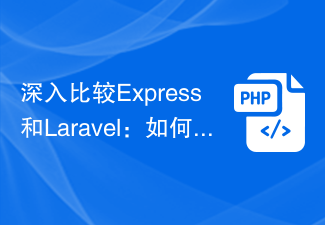
In-depth comparison of Express and Laravel: How to choose the best framework? When choosing a back-end framework suitable for your project, Express and Laravel are undoubtedly two popular choices among developers. Express is a lightweight framework based on Node.js, while Laravel is a popular framework based on PHP. This article will provide an in-depth comparison of the advantages and disadvantages of these two frameworks and provide specific code examples to help developers choose the framework that best suits their needs. Performance and scalabilityExpr
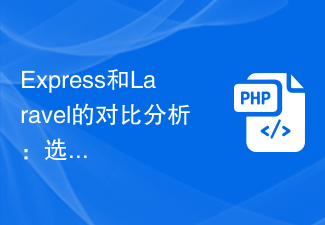
Express and Laravel are two very popular web frameworks, representing the excellent frameworks of the two major development languages of JavaScript and PHP respectively. This article will conduct a comparative analysis of these two frameworks to help developers choose a framework that is more suitable for their project needs. 1. Framework Introduction Express is a web application framework based on the Node.js platform. It provides a series of powerful functions and tools that enable developers to quickly build high-performance web applications. Express
