


Introducing the usage of read in linux
1. Basic reading
The read command receives input from standard input (keyboard) or input from other file descriptors (discussed later). After getting the input, the read command puts the data into a standard variable. The following is the simplest form of the read command
::
#!/bin/bash echo -n "Enter your name:" //参数-n的作用是不换行,echo默认是换行 read name //从键盘输入 echo "hello $name,welcome to my program" //显示信息 exit 0 //退出shell程序。 //********************************
Since the read command provides the -p parameter, a prompt can be specified directly on the read command line.
So the above script can be abbreviated as the following script::
#!/bin/bash read -p "Enter your name:" name echo "hello $name, welcome to my program" exit 0
In the above read, the variable after read only has one name, or there can be multiple. At this time, if multiple data are input, then The first data is given to the first variable, and the second data is given to the second variable. If there are too many input data, all the values will be given to the first variable. Will not end if too little input.
//******************************************
You do not need to specify variables in the read command line. If you do not specify a variable, the read command will place the received data in the environment variable REPLY.
For example::
read -p "Enter a number"
The environment variable REPLY contains all the data entered and can be used in shell scripts like other variables. Environment variable REPLY.
2. Timing input.
There are potential dangers in using the read command. The script will most likely stop and wait for user input. If the script must continue execution regardless of whether data is entered, a timer can be specified using the -t option.
-t option specifies the number of seconds the read command waits for input. When the timer expires, the read command returns a non-zero exit status;
#!/bin/bash if read -t 5 -p "please enter your name:" name then echo "hello $name ,welcome to my script" else echo "sorry,too slow" fi exit 0
In addition to inputting the timer, you can also set the read command to count the characters entered. When the number of characters entered reaches the predetermined number, it automatically exits and assigns the entered data to variables.
#!/bin/bash read -n1 -p "Do you want to continue [Y/N]?" answer case $answer in Y | y) echo "fine ,continue";; N | n) echo "ok,good bye";; *) echo "error choice";; esac exit 0
This example uses the -n option, followed by the value 1, to instruct the read command to exit as soon as it receives one character. Just press a character to answer, and the read command immediately
accepts the input and passes it to a variable. No need to press enter.
3. Silent reading (input is not displayed on the monitor)
Sometimes script user input is required, but the entered data is not expected to be displayed on the monitor. A typical example is entering a password, but of course there are many other data that need to be hidden. The
-s option enables the data entered in the read command not to be displayed on the monitor (actually, the data is displayed, but the read command sets the text color to the same color as the background).
#!/bin/bash read -s -p "Enter your password:" pass echo "your password is $pass" exit 0
4. Read files
Finally, you can also use the read command to read files on the Linux system.
Every time the read command is called, "one line" of text in the file will be read. When the file has no readable lines, the read command will exit with a non-zero status.
The key to reading a file is how to transfer the data in the text to the read command.
The most common method is to use the cat command on the file and pipe the results directly to the while command containing the read command
Example::
#!/bin/bash count=1 //赋值语句,不加空格 cat test | while read line //cat 命令的输出作为read命令的输入,read读到的值放在line中 do echo "Line $count:$line" count=$[ $count + 1 ] //注意中括号中的空格。 done echo "finish" exit
The above is the detailed content of Introducing the usage of read in linux. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
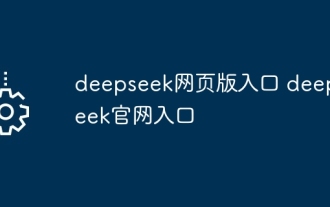
DeepSeek is a powerful intelligent search and analysis tool that provides two access methods: web version and official website. The web version is convenient and efficient, and can be used without installation; the official website provides comprehensive product information, download resources and support services. Whether individuals or corporate users, they can easily obtain and analyze massive data through DeepSeek to improve work efficiency, assist decision-making and promote innovation.
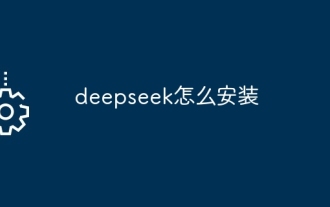
There are many ways to install DeepSeek, including: compile from source (for experienced developers) using precompiled packages (for Windows users) using Docker containers (for most convenient, no need to worry about compatibility) No matter which method you choose, Please read the official documents carefully and prepare them fully to avoid unnecessary trouble.
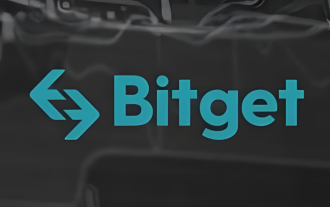
BITGet is a cryptocurrency exchange that provides a variety of trading services including spot trading, contract trading and derivatives. Founded in 2018, the exchange is headquartered in Singapore and is committed to providing users with a safe and reliable trading platform. BITGet offers a variety of trading pairs, including BTC/USDT, ETH/USDT and XRP/USDT. Additionally, the exchange has a reputation for security and liquidity and offers a variety of features such as premium order types, leveraged trading and 24/7 customer support.
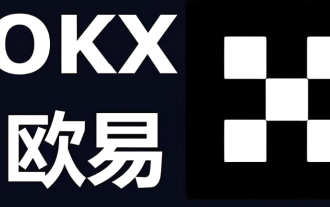
Ouyi OKX, the world's leading digital asset exchange, has now launched an official installation package to provide a safe and convenient trading experience. The OKX installation package of Ouyi does not need to be accessed through a browser. It can directly install independent applications on the device, creating a stable and efficient trading platform for users. The installation process is simple and easy to understand. Users only need to download the latest version of the installation package and follow the prompts to complete the installation step by step.
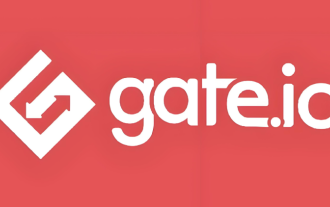
Gate.io is a popular cryptocurrency exchange that users can use by downloading its installation package and installing it on their devices. The steps to obtain the installation package are as follows: Visit the official website of Gate.io, click "Download", select the corresponding operating system (Windows, Mac or Linux), and download the installation package to your computer. It is recommended to temporarily disable antivirus software or firewall during installation to ensure smooth installation. After completion, the user needs to create a Gate.io account to start using it.
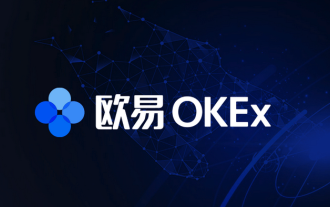
Ouyi, also known as OKX, is a world-leading cryptocurrency trading platform. The article provides a download portal for Ouyi's official installation package, which facilitates users to install Ouyi client on different devices. This installation package supports Windows, Mac, Android and iOS systems. Users can choose the corresponding version to download according to their device type. After the installation is completed, users can register or log in to the Ouyi account, start trading cryptocurrencies and enjoy other services provided by the platform.
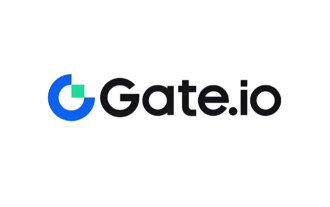
Gate.io is a highly acclaimed cryptocurrency trading platform known for its extensive token selection, low transaction fees and a user-friendly interface. With its advanced security features and excellent customer service, Gate.io provides traders with a reliable and convenient cryptocurrency trading environment. If you want to join Gate.io, please click the link provided to download the official registration installation package to start your cryptocurrency trading journey.
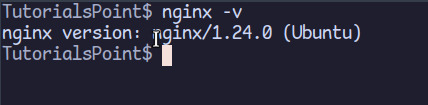
This tutorial guides you through installing and configuring Nginx and phpMyAdmin on an Ubuntu system, potentially alongside an existing Apache server. We'll cover setting up Nginx, resolving potential port conflicts with Apache, installing MariaDB (
