Introduction to Python's common built-in functions
The so-called built-in functions can be used directly without import. The following article mainly introduces some common built-in functions for basic learning of Python. The article introduces them to you in great detail through sample codes. Friends who need them can refer to them. Let's follow the editor to learn together.
Preface
Python provides many built-in functions to handle many types. The function of these built-in functions is that they can often Perform similar operations on multiple types of objects, that is, operations common to multiple types of objects. I won’t go into more details below. Let’s take a look at the detailed introduction.
map()
The map() function accepts two parameters, one is a function and the other is an iterable object (Iterable), map Apply the passed function to each element of the iterable object in turn, and return the result as an iterator.
For example, there is a function f(x)=x^2
, and you want to apply this function to a list[1,2,3,4, 5,6,7,8,9]
上:
Using a simple loop can achieve:
>>> def f(x): ... return x * x ... L = [] for n in [1, 2, 3, 4, 5, 6, 7, 8, 9]: L.append(f(n)) print(L)
Using advanced Function map()
:
>>> r = map(f, [1, 2, 3, 4, 5, 6, 7, 8, 9]) >>> list(r) [1, 4, 9, 16, 25, 36, 49, 64, 81]
The result r is an iterator, and the iterator is a lazy sequence, passed list()
The function lets it calculate the entire sequence and return a list.
If you want to convert all the numbers in this list into strings, use map()
. It’s simple:
>>> list(map(str, [1, 2, 3, 4, 5, 6, 7, 8, 9])) ['1', '2', '3', '4', '5', '6', '7', '8', '9']
Small exercise: Use the map()
function to change the non-standard English name entered by the user into a standard name with the first letter capitalized and other lowercase letters. Input['adam', 'LISA', 'barT'],
Output['Adam', 'Lisa', 'Bart']
def normalize(name): return name.capitalize() l1=["adam","LISA","barT"] l2=list(map(normalize,l1)) print(l2)
reduce()
reduce()
The function also accepts two parameters, one is a function and the other is Iterable object, reduce will perform cumulative calculation on the result of applying the passed function to each element of the iterable object. Then return the final result.
The effect is: reduce(f, [x1, x2, x3, x4]) = f(f(f(x1, x2), x3), x4)
For example, convert the sequence [1,2,3,4,5]
into an integer 12345:
>>> from functools import reduce >>> def fn(x, y): ... return x * 10 + y ... >>> reduce(fn, [1, 2, 3, 4, 5]) 12345
Small exercise: Write a prod()
function that can accept a list and use reduce to calculate the product:
from functools import reduce def pro (x,y): return x * y def prod(L): return reduce(pro,L) print(prod([1,3,5,7]))
map()
andreduce()
Comprehensive exercise: Write the str2float function to convert the string '123.456' into a floating point type 123.456
CHAR_TO_FLOAT = { '0': 0,'1': 1,'2': 2,'3': 3,'4': 4,'5': 5,'6': 6,'7': 7,'8': 8,'9': 9, '.': -1 } def str2float(s): nums = map(lambda ch:CHAR_TO_FLOAT[ch],s) point = 0 def to_float(f,n): nonlocal point if n==-1: point =1 return f if point ==0: return f*10+n else: point =point *10 return f + n/point return reduce(to_float,nums,0)#第三个参数0是初始值,对应to_float中f
filter()
filter()
The function is used to filter the sequence, filter()
also accepts a function and a sequence, filter()
Apply the passed function to each element in turn, and then decide whether to keep or discard the element based on whether the return value is True or False.
For example, delete even numbers in the list:
def is_odd(n): return n % 2 == 1 list(filter(is_odd, [1, 2, 4, 5, 6, 9, 10, 15])) # 结果: [1, 5, 9, 15]
Small exercise: Use filter() to find prime numbers
One method of calculating prime numbers is the Ehrlich sieve method. Its algorithm is very simple to understand:
First, list all natural numbers starting from 2 and construct a sequence:
2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, ...
Take the first number 2 of the sequence, which must be a prime number, and then use 2 to filter out the multiples of 2 in the sequence:
3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, ...
Get the first number 3 of the new sequence, It must be a prime number, and then use 3 to filter out the multiples of 3 in the sequence:
5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, ...
Take the first number 5 of the new sequence, and then use 5 to filter out the multiples of 5 in the sequence:
7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, ...
Keep sifting and you can get all Prime number.
Use Python to implement this algorithm. First construct a period sequence starting from 3:
def _odd_iter(): n = 1 while True: n = n + 2 yield n #这是一个生成器,并且是一个无线序列
Define a filtering function:
def _not_pisible(n): return lambda x: x % n > 0
Define a generator to continuously return the next prime number:
def primes(): yield 2 it = _odd_iter() # 初始序列 while True: n = next(it) # 返回序列的第一个数 yield n it = filter(_not_pisible(n), it) # 构造新序列
Print prime numbers within 100:
for n in primes(): if n < 100: print(n) else: break
sorted()
Python’s built-in sorted()
function can sort the list Sort:
>>> sorted([36, 5, -12, 9, -21]) [-21, -12, 5, 9, 36]
sorted()
The function is also a higher-order function and can also accept a key function to implement custom sorting:
>>> sorted([36, 5, -12, 9, -21], key=abs) [5, 9, -12, -21, 36]
The function specified by key will act on each element of the list and be sorted according to the result returned by the key function.
默认情况下,对字符串排序,是按照ASCII的大小比较的,由于'Z' < 'a',结果,大写字母Z会排在小写字母a的前面。如果想忽略大小写可都转换成小写来比较:
>>> sorted(['bob', 'about', 'Zoo', 'Credit'], key=str.lower) ['about', 'bob', 'Credit', 'Zoo']
要进行反向排序,不必改动key函数,可以传入第三个参数reverse=True
:
>>> sorted(['bob', 'about', 'Zoo', 'Credit'], key=str.lower, reverse=True) ['Zoo', 'Credit', 'bob', 'about']
小练习:假设我们用一组tuple表示学生名字和成绩:L = [('Bob', 75), ('Adam', 92), ('Bart', 66), ('Lisa', 88)]
。用sorted()
对上述列表分别按c成绩从高到低排序:
L = [('Bob', 75), ('Adam', 92), ('Bart', 66), ('Lisa', 88)] def by_score(t): for i in t: return t[1] L2=sorted(L,key= by_score) print(L2)
运用匿名函数更简洁:
L2=sorted(L,key=lambda t:t[1]) print(L2)
The above is the detailed content of Introduction to Python's common built-in functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


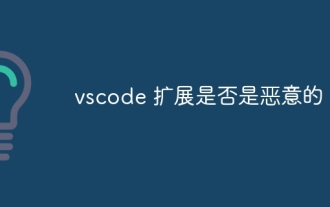
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
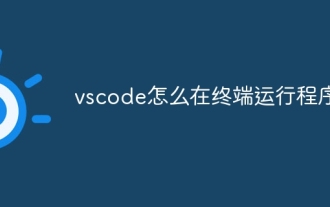
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
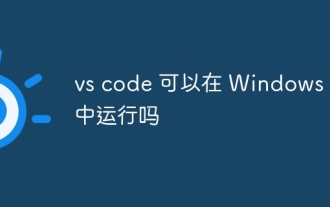
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
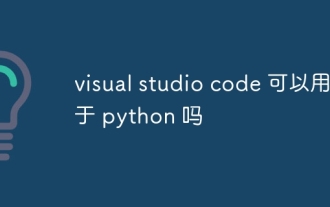
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
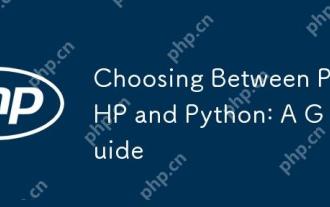
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
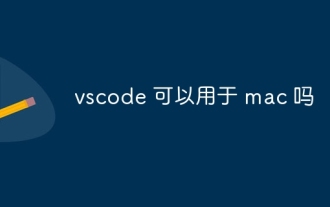
VS Code is available on Mac. It has powerful extensions, Git integration, terminal and debugger, and also offers a wealth of setup options. However, for particularly large projects or highly professional development, VS Code may have performance or functional limitations.
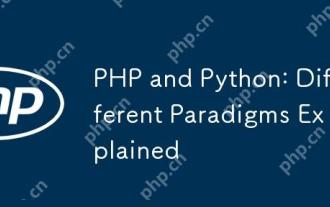
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
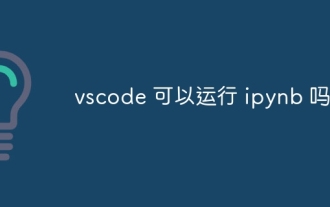
The key to running Jupyter Notebook in VS Code is to ensure that the Python environment is properly configured, understand that the code execution order is consistent with the cell order, and be aware of large files or external libraries that may affect performance. The code completion and debugging functions provided by VS Code can greatly improve coding efficiency and reduce errors.
