What is shallow and deep copy in JS
Shallow copy and deep copy in JavaScript
After learning JavaScript for such a long time, you must not be familiar with shallow copy and deep copy. Today in the project Since it has been used in , sooner or later we have to clarify our thoughts. Before understanding, let’s start with the stack where JavaScript data types are stored!
Now we come with questions study!
1: What is a stack?
We all know: In the computer field, stacks are two data structures that can only insert and delete data items at one end (called the top of the stack).
Stack: Queue priority, first in first out; automatically allocated and released by the operation system , storing the parameter values of the function and the value of the local variable wait. It operates like a stack in a data structure.
Heap: first in, last out; Dynamicly allocated space is generally allocated and released by the programmer. If the programmer does not release it, the program ends It may be recycled by the OS, and the allocation method is similar to a linked list.
The above all belong to the basic part of the computer, so I won’t go into details here. Let’s contact JavaScript to analyze the stack.
2: What is the connection between basic types and reference types in JavaScript and the stack?
JavaScript data types are divided into two major types:
1. Basic types: Undefined, Null, Boolean, Number and String, these 5 basic data types can Directly access , they are allocated according to the value, and are simple data segments stored in stack memory . The data size is determined and the memory space size can be allocated.
2. Reference type: That is, an object stored in heap memory. The variable actually stores a pointer, which points to another location.
We know what a stack is and the data types of JavaScript. Let’s explain their copying according to the data type of js:
var obj1 = {name:'bangbang',age:18}; var b = obj1; var c = obj1.age; console.log(b.name); //bangbang console.log(c); //18//改变b和c的值 b.name = 'yanniu'; c = 22; console.log(obj1.name); //yanniu console.log(obj1.age); //18
As can be seen from the above: when we change the data of b At that time, we saw that the data of obj1.name was also changing, but when we changed the data of c, we found that the value of obj1.age did not change. This shows that: the b and obj1 variables operate on the same object, and c and obj1 Completely independent. The diagram is as follows:
3: What is shallow copy?
According to the above statement, Basic typeWhen copying, it only opens up a new space in the memory and its parent element (again we call the copied object the parent element) They are things that each other does not want to do, so deep and shallow copies are relative to the reference type , so that we can save the parent object of the reference type! hey-hey! Let’s see next!
For example:
var father1 = {name:'shangdi',age:1000,job:['teacher','cook']};//浅拷贝函数 function copy(obj){ var childs = {}; for(var key in obj){ childs[key] = obj[key]; } return childs; }var child1 = copy(father1); console.log(child1); //{ name: 'shangdi', age: 1000 } console.log(typeof child1); //object //改变子对象的name属性,发现对父对象的name没有影响 哈哈! child1.name = 'bangbang'; console.log(father1); //{ name: 'shangdi', age: 1000 } console.log(child1); //{ name: 'bangbang', age: 1000 } //注意:这次改变子对象的job属性也就是改变数组,//发现对父对象的job竟然有影响,吓死宝宝了,那怎么办呢,那这个copy有什么用呢是吧! child1.job.push('programer'); console.log(father1); //{ name: 'shangdi',age: 1000,job: [ 'teacher', 'cook', 'programer' ] } console.log(child1); //{ name: 'shangdi',age: 1000,job: [ 'teacher', 'cook', 'programer' ] }
It can be analyzed from the above: During shallow copy, when we change the array of the child object, the parent object also changes, that is to say: The child object and the parent When objects are shallow copied, they point to the same memory array: As shown in the figure:
If we want the copy of the child object to have no connection with the parent object, then we must use deep copy! hey-hey! A son can’t completely follow his father!
Four: What is deep copy?
Deep copy is to copy the parent object to the child object, and the memory and subsequent operations of both will not affect each other copy!
function deepCopy(obj){ var o; switch(typeof obj){ case 'undefined': break; case 'string' : o = obj + '';break; case 'number' : o = obj - 0;break; case 'boolean' : o = obj;break; case 'object' : if(obj === null){ o = null; }else{ if(obj instanceof Array){ o = []; for(var i = 0, len = obj.length; i < len; i++){ o.push(deepCopy(obj[i])); } }else{ o = {}; for(var k in obj){ o[k] = deepCopy(obj[k]); } } } break; default: o = obj;break; } return o; }
Here are some cloning methods for your reference, but they are different, try it yourself:
Method 2: The simplest
function deepCopy(obj) { return JSON.parse(JSON.stringify(obj)); }
Method 3:
function deepCopy(obj){ var newobj, obj; if (obj.constructor == Object){ newobj = new obj.constructor(); }else{ newobj = new obj.constructor(obj.valueOf());//valueOf()方法返回 Array 对象的原始值 } for(var key in obj){ if ( newobj[key] != obj[key] ){ if ( typeof(obj[key]) == 'object' ){ newobj[key] = deepCopy(obj[key]); }else{ newobj[key] = obj[key]; } } } newobj.toString = obj.toString; newobj.valueOf = obj.valueOf; return newobj; }
Method 4:
var cloneObj = function(obj){ var str, newobj = obj.constructor === Array ? [] : {}; if(typeof obj !== 'object'){ return; } else if(window.JSON){ str = JSON.stringify(obj), //系列化对象 newobj = JSON.parse(str); //还原 } else { for(var i in obj){ newobj[i] = typeof obj[i] === 'object' ? cloneObj(obj[i]) : obj[i]; } } return newobj; };
Method Five: (JavaScript Object-Oriented Programming Guide)
function deepCopy(p,c){ c = c || {}; for (var i in p){ if(p.hasOwnProperty(i)){ if(typeof p[i] === 'object'){ c[i] = Array.isArray(p[i]) ? [] : {}; deepCopy(p[i],c[i]); }else{ c[i] = p[i]; } } } return c; }
The above is the detailed content of What is shallow and deep copy in JS. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


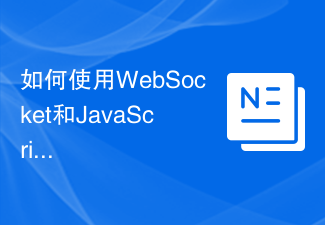
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
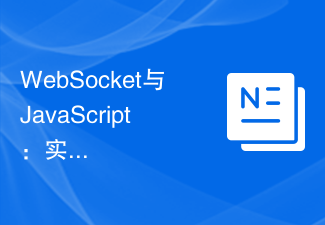
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
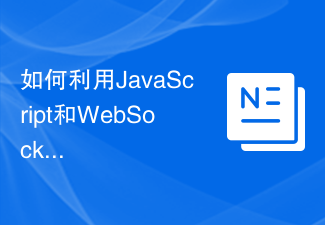
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
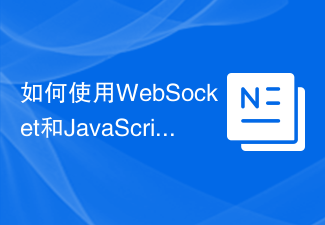
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
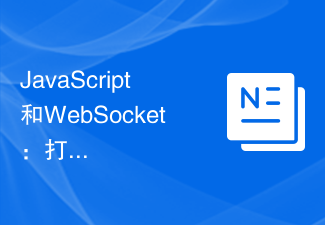
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
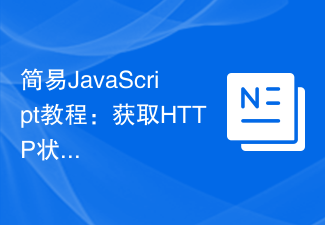
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
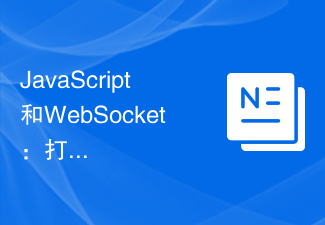
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
