


Example sharing about .env files and model operations in Laravel 5.2
1. .env file
.env file is the environment configuration file of the application. This file will be used when configuring application parameters, database connection, and cache processing .
// 应用相关参数 APP_ENV=local APP_DEBUG=true //应用调试模式 APP_KEY=base64:hMYz0BMJDJARKgrmaV93YQY/p9SatnV8m0kT4LVJR5w= //应用key APP_URL=http://localhost // 数据库连接参数 DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravelblog DB_USERNAME=root DB_PASSWORD= DB_PREFIX='hd_' // 缓存相关参数 CACHE_DRIVER=file SESSION_DRIVER=file QUEUE_DRIVER=sync // Redis 连接参数 REDIS_HOST=127.0.0.1 REDIS_PASSWORD=null REDIS_PORT=6379 // 邮件相关参数 MAIL_DRIVER=smtp MAIL_HOST=mailtrap.io MAIL_PORT=2525 MAIL_USERNAME=null MAIL_PASSWORD=null MAIL_ENCRYPTION=null
Among them, regarding the explanation of this APP_KEY, there are the following comments in the config/app.php file:
/* |-------------------------------------------------------------------------- | Encryption Key |-------------------------------------------------------------------------- | | This key is used by the Illuminate encrypter service and should be set | to a random, 32 character string, otherwise these encrypted strings | will not be safe. Please do this before deploying an application! | */ 'key' => env('APP_KEY'), 'cipher' => 'AES-256-CBC',
key key reads the APP_KEY of the .env file, which is usually a 32-bit random string. The cipher key determines the length of APP_KEY, which is generally AES-256-CBC (default) indicating that the key is 32 characters long, or AES-128-CBC means 16 bits.
So, in order to ensure the security of the session and encryption service, APP_KEY must be set, use the Artisan command to generate:
php artisan key:generate
In this way, A new APP_KEY will be written to the .env file.
2. Model operation
1. DB class
// 插入 DB::insert('insert into hd_user(username, password) values(?, ?)', ['admin', 123456]); // 查询 DB::select('select * from hd_user where username = ?', ['admin']); // 更新 DB::update('update hd_user set password= ? where username = ?', [654321, 'admin']); // 删除 DB::delete('delete from hd_user where username = ?', ['admin']);
Note: dd() function is similar to print_r (), used to print variable information, is an auxiliary function of Laravel.
2. Query Builder
The table method of the DB class returns a query builder for the given table.
// 查询所有 DB::table('user')->get(); // 查询多条 DB::table('user')->where('age', '>', 20)->get(); // 查询一条 DB::table('user')->where('age', '>', 20)->first(); // 查询特定字段 DB::table('user')->select('name', 'email as user_email')->get(); // distinct() 方法去重复 $users = DB::table('user')->distinct()->get();
3. Eloquent ORM
1. Create model
php artisan make:model User
2. Table name, primary key, timestamp
Table name: The plural of the default model class name is used as the table name, which can be overridden by defining the protected $table attribute in the model class.
Primary key: The default primary key name is "id", which can be overridden by defining the protected $primaryKey attribute in the model class.
Timestamp: created_at and updated_a fields will be managed by default. You can define the public $timestamps attribute as false in the model class.
3. Data operation
In the controller method:
// 插入 $user = new User; $user->username = 'admin'; $user->save(); // 查询 // 查询所有 User::get(); // 查询多条 User::where('age', '>', '20')->get(); // 查询一条 user::find(1); // 更新 $user = User::find(1); // 查找主键为1的一条记录 $user->username = 'new name'; $user->save(); // 或者用 update() 方法 // 删除 // 方法1.先获取记录再删除 User::find(1)->delete(); // 方法2.通过主键直接删除 User::destroy(1, 2); // 方法3.通过 where 条件删除 User::where('username', 'admin')->delete();
The above is the detailed content of Example sharing about .env files and model operations in Laravel 5.2. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


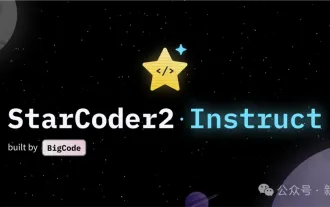
At the forefront of software technology, UIUC Zhang Lingming's group, together with researchers from the BigCode organization, recently announced the StarCoder2-15B-Instruct large code model. This innovative achievement achieved a significant breakthrough in code generation tasks, successfully surpassing CodeLlama-70B-Instruct and reaching the top of the code generation performance list. The unique feature of StarCoder2-15B-Instruct is its pure self-alignment strategy. The entire training process is open, transparent, and completely autonomous and controllable. The model generates thousands of instructions via StarCoder2-15B in response to fine-tuning the StarCoder-15B base model without relying on expensive manual annotation.

1. Introduction Over the past few years, YOLOs have become the dominant paradigm in the field of real-time object detection due to its effective balance between computational cost and detection performance. Researchers have explored YOLO's architectural design, optimization goals, data expansion strategies, etc., and have made significant progress. At the same time, relying on non-maximum suppression (NMS) for post-processing hinders end-to-end deployment of YOLO and adversely affects inference latency. In YOLOs, the design of various components lacks comprehensive and thorough inspection, resulting in significant computational redundancy and limiting the capabilities of the model. It offers suboptimal efficiency, and relatively large potential for performance improvement. In this work, the goal is to further improve the performance efficiency boundary of YOLO from both post-processing and model architecture. to this end
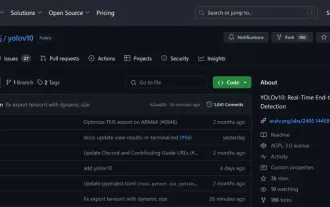
The benchmark YOLO series of target detection systems has once again received a major upgrade. Since the release of YOLOv9 in February this year, the baton of the YOLO (YouOnlyLookOnce) series has been passed to the hands of researchers at Tsinghua University. Last weekend, the news of the launch of YOLOv10 attracted the attention of the AI community. It is considered a breakthrough framework in the field of computer vision and is known for its real-time end-to-end object detection capabilities, continuing the legacy of the YOLO series by providing a powerful solution that combines efficiency and accuracy. Paper address: https://arxiv.org/pdf/2405.14458 Project address: https://github.com/THU-MIG/yo
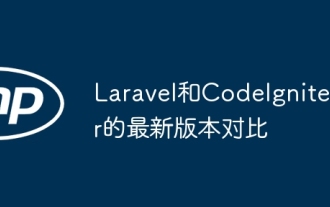
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
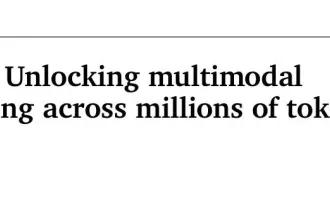
In February this year, Google launched the multi-modal large model Gemini 1.5, which greatly improved performance and speed through engineering and infrastructure optimization, MoE architecture and other strategies. With longer context, stronger reasoning capabilities, and better handling of cross-modal content. This Friday, Google DeepMind officially released the technical report of Gemini 1.5, which covers the Flash version and other recent upgrades. The document is 153 pages long. Technical report link: https://storage.googleapis.com/deepmind-media/gemini/gemini_v1_5_report.pdf In this report, Google introduces Gemini1
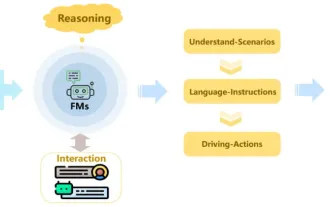
Written above & the author’s personal understanding: Recently, with the development and breakthroughs of deep learning technology, large-scale foundation models (Foundation Models) have achieved significant results in the fields of natural language processing and computer vision. The application of basic models in autonomous driving also has great development prospects, which can improve the understanding and reasoning of scenarios. Through pre-training on rich language and visual data, the basic model can understand and interpret various elements in autonomous driving scenarios and perform reasoning, providing language and action commands for driving decision-making and planning. The base model can be data augmented with an understanding of the driving scenario to provide those rare feasible features in long-tail distributions that are unlikely to be encountered during routine driving and data collection.
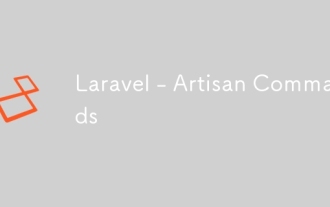
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
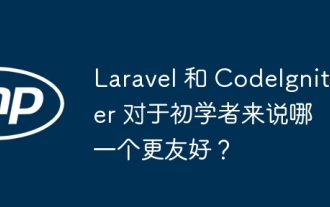
For beginners, CodeIgniter has a gentler learning curve and fewer features, but covers basic needs. Laravel offers a wider feature set but has a slightly steeper learning curve. In terms of performance, both Laravel and CodeIgniter perform well. Laravel has more extensive documentation and active community support, while CodeIgniter is simpler, lightweight, and has strong security features. In the practical case of building a blogging application, Laravel's EloquentORM simplifies data manipulation, while CodeIgniter requires more manual configuration.
