


Methods to connect multiple clients in python multi-threaded socket programming
This article mainly introduces multi-client access in python multi-threaded socket programming in detail, which has certain reference value. Interested friends can refer to
Implementing socket communication in Python The server side is relatively complex, but the client side is very simple, so the client side is basically implemented using the sockct module, while the service side has many modules that can be used, as follows:
1. Client
#!/usr/bin/env python #coding:utf-8 ''' file:client.py date:9/9/17 3:43 PM author:lockey email:lockey@123.com desc:socket编程客户端,python3.6.2 ''' import socket,sys HOST = '192.168.1.6' PORT = 8998 ADDR =(HOST,PORT) BUFSIZE = 1024 sock = socket.socket() try: sock.connect(ADDR) print('have connected with server') while True: data = input('lockey# ') if len(data)>0: print('send:',data) sock.sendall(data.encode('utf-8')) #不要用send() recv_data = sock.recv(BUFSIZE) print('receive:',recv_data.decode('utf-8')) else: sock.close() break except Exception: print('error') sock.close() sys.exit()
2. SocketServer module
#!/usr/bin/env python #coding:utf-8 ''' file:client.py date:9/9/17 3:43 PM author:lockey email:lockey@123.com desc:socket编程服务器端,python3.6.2 ''' from socketserver import BaseRequestHandler,ThreadingTCPServer import threading BUF_SIZE=1024 class Handler(BaseRequestHandler): def handle(self): address,pid = self.client_address print('%s connected!'%address) while True: data = self.request.recv(BUF_SIZE) if len(data)>0: print('receive=',data.decode('utf-8')) cur_thread = threading.current_thread() #response = '{}:{}'.format(cur_thread.ident,data) self.request.sendall('response'.encode('utf-8')) print('send:','response') else: print('close') break if __name__ == '__main__': HOST = '192.168.1.6' PORT = 8998 ADDR = (HOST,PORT) server = ThreadingTCPServer(ADDR,Handler) #参数为监听地址和已建立连接的处理类 print('listening') server.serve_forever() #监听,建立好TCP连接后,为该连接创建新的socket和线程,并由处理类中的handle方法处理 print(server)
The above is the detailed content of Methods to connect multiple clients in python multi-threaded socket programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
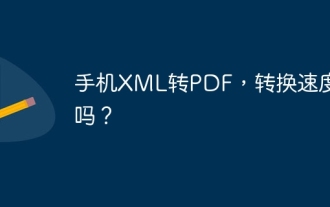
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
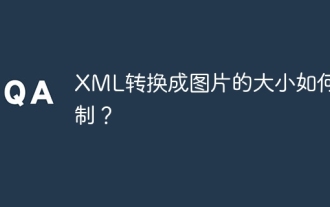
To generate images through XML, you need to use graph libraries (such as Pillow and JFreeChart) as bridges to generate images based on metadata (size, color) in XML. The key to controlling the size of the image is to adjust the values of the <width> and <height> tags in XML. However, in practical applications, the complexity of XML structure, the fineness of graph drawing, the speed of image generation and memory consumption, and the selection of image formats all have an impact on the generated image size. Therefore, it is necessary to have a deep understanding of XML structure, proficient in the graphics library, and consider factors such as optimization algorithms and image format selection.
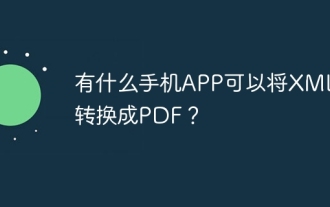
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
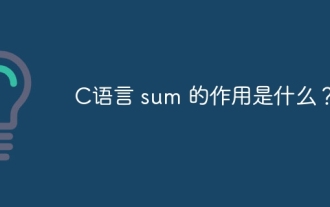
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
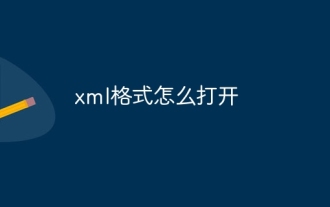
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
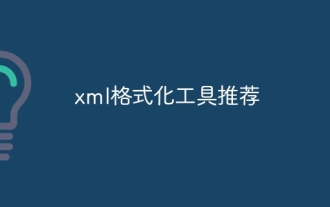
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
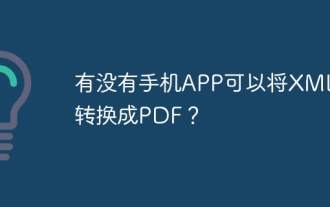
There is no APP that can convert all XML files into PDFs because the XML structure is flexible and diverse. The core of XML to PDF is to convert the data structure into a page layout, which requires parsing XML and generating PDF. Common methods include parsing XML using Python libraries such as ElementTree and generating PDFs using ReportLab library. For complex XML, it may be necessary to use XSLT transformation structures. When optimizing performance, consider using multithreaded or multiprocesses and select the appropriate library.
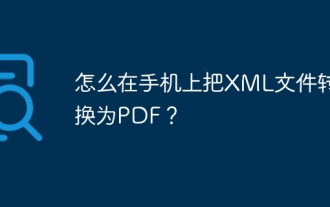
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
