Value passing problem of VUE2.0 components
##Transfer between Vue1.0 componentsUse $on() to listen for events;
Use $emit() to trigger events on it;
Use $dispatch() to dispatch events, which bubble along the parent chain;
Use $broadcast() to broadcast events, and events are propagated downward to all descendants
1. Pass the value from the child component to the parent component:
##Child.vue<template>
<p class="child">
<h1>子组件</h1>
<button v-on:click="childToParent">想父组件传值</button>
</p>
</template>
<script>
export default{
name: 'child',
data(){
return {}
},
methods: {
childToParent(){
this.$emit("childToParentMsg", "子组件向父组件传值");
}
}
}
</script>parent.vue
<template>
<p class="parent">
<h1>父组件</h1>
<Child v-on:childToParentMsg="showChildToParentMsg" ></Child>
</p>
</template>
<script>
import Child from './child/Child.vue'
export default{
name:"parent",
data(){
return {
}
},
methods: {
showChildToParentMsg:function(data){
alert("父组件显示信息:"+data)
}
},
components: {Child}
}
</script>
2. Parent component passes value to child component
parent.vue <template>
<p class="parent">
<h1>父组件</h1>
<Child v-bind:parentToChild="parentMsg"></Child>
</p>
</template>
<script>
import Child from './child/Child.vue'
export default{
name:"parent",
data(){
return {
parentMsg:'父组件向子组件传值'
}
},
components: {Child}
}
</script>
child.vue<template>
<p class="child">
<h1>子组件</h1>
<span>子组件显示信息:{{parentToChild}}</span><br>
</p>
</template>
<script>
export default{
name: 'child',
data(){
return {}
},
props:["parentToChild"]
}
</script>
3. Use eventBus.js to pass value ---Brother Value transfer between components
##eventBus.jsimport Vue from 'Vue'
export default new Vue()
<template>
<p id="app">
<secondChild></secondChild>
<firstChild></firstChild>
</p>
</template>
<script>
import FirstChild from './components/FirstChild'
import SecondChild from './components/SecondChild'
export default {
name: 'app',
components: {
FirstChild,
SecondChild,
}
}
</script>
<template>
<p class="firstChild">
<input type="text" placeholder="请输入文字" v-model="message">
<button @click="showMessage">向组件传值</button>
<br>
<span>{{message}}</span>
</p>
</template>
<script>
import bus from '../assets/eventBus';
export default{
name: 'firstChild',
data () {
return {
message: '你好'
}
},
methods: {
showMessage () {
alert(this.message) bus.$emit('userDefinedEvent', this.message);//传值
}
}
}
</script>
<template>
<p id="SecondChild">
<h1>{{message}}</h1>
</p>
</template>
<script>
import bus from '../assets/eventBus';
export default{
name:'SecondChild',
data(){
return {
message: ''
}
},
mounted(){
var self = this;
bus.$on('userDefinedEvent',function(message){
self.message = message;//接值
});
}
}
The above is the detailed content of Value passing problem of VUE2.0 components. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


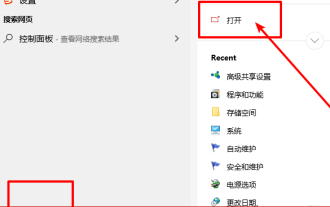
Many users always encounter some problems when playing some games on win10, such as screen freezes and blurred screens. At this time, we can solve the problem by turning on the directplay function, and the operation method of the function is also Very simple. How to install directplay, the old component of win10 1. Enter "Control Panel" in the search box and open it 2. Select large icons as the viewing method 3. Find "Programs and Features" 4. Click on the left to enable or turn off win functions 5. Select the old version here Just check the box
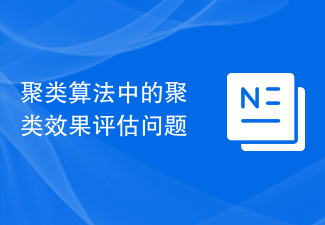
The clustering effect evaluation problem in the clustering algorithm requires specific code examples. Clustering is an unsupervised learning method that groups similar samples into one category by clustering data. In clustering algorithms, how to evaluate the effect of clustering is an important issue. This article will introduce several commonly used clustering effect evaluation indicators and give corresponding code examples. 1. Clustering effect evaluation index Silhouette Coefficient Silhouette coefficient evaluates the clustering effect by calculating the closeness of the sample and the degree of separation from other clusters.
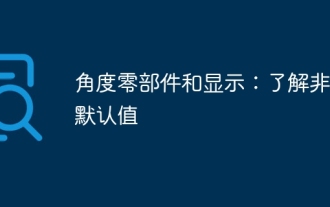
The default display behavior for components in the Angular framework is not for block-level elements. This design choice promotes encapsulation of component styles and encourages developers to consciously define how each component is displayed. By explicitly setting the CSS property display, the display of Angular components can be fully controlled to achieve the desired layout and responsiveness.
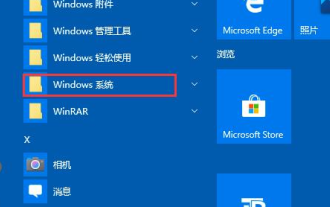
Win10 old version components need to be turned on by users themselves in the settings, because many components are usually closed by default. First we need to enter the settings. The operation is very simple. Just follow the steps below. Where are the win10 old version components? Open 1. Click Start, then click "Win System" 2. Click to enter the Control Panel 3. Then click the program below 4. Click "Enable or turn off Win functions" 5. Here you can choose what you want to open
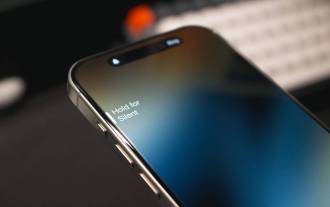
Known for its powerful performance and versatile features, the iPhone is not immune to the occasional hiccup or technical difficulty, a common trait among complex electronic devices. Experiencing iPhone problems can be frustrating, but usually no alarm is needed. In this comprehensive guide, we aim to demystify some of the most commonly encountered challenges associated with iPhone usage. Our step-by-step approach is designed to help you resolve these common issues, providing practical solutions and troubleshooting tips to get your equipment back in peak working order. Whether you're facing a glitch or a more complex problem, this article can help you resolve them effectively. General Troubleshooting Tips Before delving into specific troubleshooting steps, here are some helpful

To solve the problem that jQuery.val() cannot be used, specific code examples are required. For front-end developers, using jQuery is one of the common operations. Among them, using the .val() method to get or set the value of a form element is a very common operation. However, in some specific cases, the problem of not being able to use the .val() method may arise. This article will introduce some common situations and solutions, and provide specific code examples. Problem Description When using jQuery to develop front-end pages, sometimes you will encounter
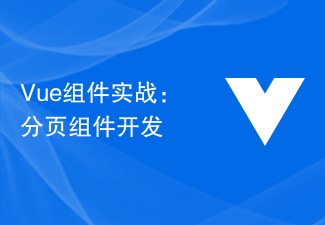
Vue component practice: Introduction to paging component development In web applications, the paging function is an essential component. A good paging component should be simple and clear in presentation, rich in functions, and easy to integrate and use. In this article, we will introduce how to use the Vue.js framework to develop a highly customizable paging component. We will explain in detail how to develop using Vue components through code examples. Technology stack Vue.js2.xJavaScript (ES6) HTML5 and CSS3 development environment
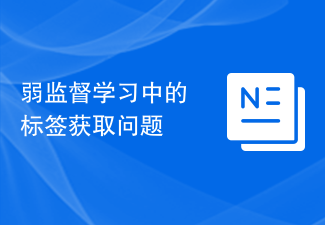
The label acquisition problem in weakly supervised learning requires specific code examples. Introduction: Weakly supervised learning is a machine learning method that uses weak labels for training. Different from traditional supervised learning, weakly supervised learning only needs to use fewer labels to train the model, rather than each sample needs to have an accurate label. However, in weakly supervised learning, how to accurately obtain useful information from weak labels is a key issue. This article will introduce the label acquisition problem in weakly supervised learning and give specific code examples. Introduction to the label acquisition problem in weakly supervised learning:
