How to write a web music player using html5
Function Introduction
The audio and video tags were launched in html5, which allows us to play audio and video directly without using other plug-ins. Next we will use the audio tag of html5 and its related attributes and methods to create a simple music player. Regarding the production of web music players in HTML5, it mainly includes the following functions:
1. Playback and pause, previous and next songs
2. Adjust volume and playback progress bar
3. Switch the current song according to the list
Let’s take a look at the final effect:
The structure of this music player is mainly divided into three parts : Song information, player and playlist, let’s focus on the player part. First, put three audio tags in the player for playback:
<audio id="music1">浏览器不支持audio标签 <source src="media/Beyond - 光辉岁月.mp3"></source> </audio> <audio id="music2">浏览器不支持audio标签 <source src="media/Daniel Powter - Free Loop.mp3"></source> </audio> <audio id="music3">浏览器不支持audio标签 <source src="media/周杰伦、费玉清 - 千里之外.mp3"></source> </audio>
The playlist below also corresponds to three audio tags:
<div id="playList"> <ul> <li id="m0">Beyond-光辉岁月</li> <li id="m1">Daniel Powter-Free Loop</li> <li id="m2">周杰伦、费玉清-千里之外</li> </ul> </div> 接下来我们就开始逐步实现上面提到的功能吧,先来完成播放和暂停功能,在按下播放按钮时我们要做到进度条随歌曲进度前进,播放时间也逐渐增加,同时播放按钮变成暂停按钮,播放列表的样式也对应改变。
Before doing the function, we must first put the three audio tags After the tag obtains the ID, it is stored in an array for subsequent use.
var music1= document.getElementById("music1"); var music2= document.getElementById("music2"); var music3= document.getElementById("music3"); var mList = [music1,music2,music3];
Play and pause:
We can now complete the function of the play button. First, set a flag to mark the playback status of the music, and then set a default for the index index of the array. Value:
Then judge the playback status, call the corresponding function, and modify the flag value and the corresponding item style of the list:
var flag = true; var index = 0; function playMusic(){ if(flag&&mList[index].paused){ mList[index].play(); document.getElementById("m"+index).style.backgroundColor = "#A71307"; document.getElementById("m"+index).style.color = "white"; progressBar(); playTimes(); play.style.backgroundImage = "url(media/pause.png)"; flag = false; }else{ mList[index].pause(); flag = true; play.style.backgroundImage = "url(media/play.png)"; } }
Multiple functions are called in the code of the above HTML page , where play and pause are the methods that come with the audio tag, while other functions are defined by ourselves. Let's take a look at how these functions are implemented and what functions they correspond to.
Progress bar and playback time:
The first is the progress bar function, which obtains the entire duration of the song, and then calculates the position of the progress bar based on the current playback progress multiplied by the total length of the progress bar. .
function progressBar(){ var lenth=mList[index].duration; timer1=setInterval(function(){ cur=mList[index].currentTime;//获取当前的播放时间 progress.style.width=""+parseFloat(cur/lenth)*300+"px"; progressBtn.style.left= 60+parseFloat(cur/lenth)*300+"px"; },10) }
The following is the function of changing the playback time. Here we set up a timing function and execute it every once in a while to change the playback time. Because the song duration we obtained is calculated in seconds, we need to use the if statement to convert the duration judgment and change the playback time to display in minutes and seconds.
function playTimes(){ timer2=setInterval(function(){ cur=parseInt(mList[index].currentTime);//秒数 var minute=parseInt(cur/60); if (minute<10) { if(cur%60<10){ playTime.innerHTML="0"+minute+":0"+cur%60+""; }else{ playTime.innerHTML="0"+minute+":"+cur%60+""; } } else{ if(cur%60<10){ playTime.innerText= minute+":0"+cur%60+""; }else{ playTime.innerText= minute+":"+cur%60+""; } } },1000); }
Adjust playback progress and volume:
Next, let’s complete the functions of adjusting playback progress and adjusting volume through the progress bar.
The function of adjusting the playback progress is implemented by using the event object. Because the offsetX attribute is only available in IE events, it is recommended to use IE browser to view the effect. First add an event listener to the progress bar. When the mouse is clicked on the progress bar, the mouse position is obtained, and the current playback progress is calculated based on the position divided by the total length of the progress bar, and then the song is set.
//调整播放进度 total.addEventListener("click",function(event){ var e = event || window.event; document.onmousedown = function(event){ var e = event || window.event; var mousePos1 = e.offsetX; var maxValue1 = total.scrollWidth; mList[index].currentTime = (mousePos1/300)*mList[index].duration; progress.style.width = mousePos1+"px"; progressBtn.style.left = 60+ mousePos1 +"px"; } })
The following is the volume adjustment function. We use dragging to adjust the volume. The idea is to add event monitoring to the button ball of the volume bar, and then calculate the button ball relative to the volume bar based on the dragged position. The overall position, and finally the current volume is obtained by multiplying the calculation result with the volume:
volBtn.addEventListener("mousedown",function(event){ var e = event || window.event; var that =this; //阻止球的默认拖拽事件 e.preventDefault(); document.onmousemove = function(event){ var e = event || window.event; var mousePos2 = e.offsetY; var maxValue2 = vol.scrollHeight; if(mousePos2<0){ mousePos2 = 0; } if(mousePos2>maxValue2){ mousePos2=maxValue2; } mList[index].volume = (1-mousePos2/maxValue2); console.log(mList[index].volume); volBtn.style.top = (mousePos2)+"px"; volBar.style.height = 60-(mousePos2)+"px"; document.onmouseup = function(event){ document.onmousemove = null; document.onmouseup = null; } } })
Song switching
Finally, we will implement the more complex song switching function.
Let’s first look at using the previous and next buttons to switch. There are several issues we should pay attention to when switching music: First, we need to stop the currently playing music and switch to the next one. A piece of music; secondly, the progress bar and playback time must be cleared and recalculated; thirdly, the song information must be changed accordingly, and the playlist style under the player must also change. After figuring out the above three points, we can start to implement the function.
//上一曲 function prevM(){ clearInterval(timer1); clearInterval(timer2); stopM(); qingkong(); cleanProgress(); --index; if(index==-1){ index=mList.length-1; } clearListBgc(); document.getElementById("m"+index).style.backgroundColor = "#A71307"; document.getElementById("m"+index).style.color = "white"; changeInfo(index); mList[index].play(); progressBar(); playTimes(); if (mList[index].paused) { play.style.backgroundImage = "url(media/play.png)"; }else{ play.style.backgroundImage = "url(media/pause.png)"; } } //下一曲 function nextM(){ clearInterval(timer1); clearInterval(timer2); stopM(); qingkong(); cleanProgress(); ++index; if(index==mList.length){ index=0; } clearListBgc(); document.getElementById("m"+index).style.backgroundColor = "#A71307"; document.getElementById("m"+index).style.color = "white"; changeInfo(index); mList[index].play(); progressBar(); playTimes(); if (mList[index].paused) { play.style.backgroundImage = "url(media/play.png)"; }else{ play.style.backgroundImage = "url(media/pause.png)"; } }
The code below is to click on the list to switch songs.
m0.onclick = function (){ clearInterval(timer1); clearInterval(timer2); qingkong(); flag = false; stopM(); index = 0; pauseall(); play.style.backgroundImage = "url(media/pause.png)"; clearListBgc(); document.getElementById("m0").style.backgroundColor = "#A71307"; document.getElementById("m"+index).style.color = "white"; mList[index].play(); cleanProgress(); progressBar(); changeInfo(index); playTimes(); } m1.onclick = function (){ clearInterval(timer1); clearInterval(timer2); flag = false; qingkong(); stopM(); index = 1; pauseall(); clearListBgc(); play.style.backgroundImage = "url(media/pause.png)"; document.getElementById("m1").style.backgroundColor = "#A71307"; document.getElementById("m"+index).style.color = "white"; mList[index].play(); cleanProgress(); changeInfo(index); progressBar(); playTimes(); } m2.onclick = function (){ clearInterval(timer1); clearInterval(timer2); flag = false; qingkong(); stopM(); index = 2; pauseall(); play.style.backgroundImage = "url(media/pause.png)"; clearListBgc(); document.getElementById("m2").style.backgroundColor = "#A71307"; document.getElementById("m"+index).style.color = "white"; mList[index].play(); cleanProgress(); changeInfo(index); progressBar(); playTimes(); }
The idea of switching songs through playlists is similar to switching through buttons. It just sets which song should be played currently according to the corresponding list item.
The first is to switch song information: Several methods are called in the function of switching songs above. Let's take a look at the uses of these methods.
First switch the song information:
function changeInfo(index){ if (index==0) { musicName.innerHTML = "光辉岁月"; singer.innerHTML = "Beyond"; } if (index==1) { musicName.innerHTML = "Free Loop"; singer.innerHTML = "Daniel Powter"; } if (index==2) { musicName.innerHTML = "千里之外"; singer.innerHTML = "周杰伦、费玉清"; } }
Then clear the two timers:
//将进度条置0 function cleanProgress(timer1){ if(timer1!=undefined){ clearInterval(timer1); } progress.style.width="0"; progressBtn.style.left="60px"; } function qingkong(timer2){ if(timer2!=undefined){ clearInterval(timer2); } }
Then stop the playing music and restore the playing time.
function stopM(){ if(mList[index].played){ mList[index].pause(); mList[index].currentTime=0; flag=false; } }
At this point, we have basically completed the music player written in html5. After completion, the effect is still very good. Hurry up and collect it. I hope you can write your own player using HTML5 based on the above tutorial.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


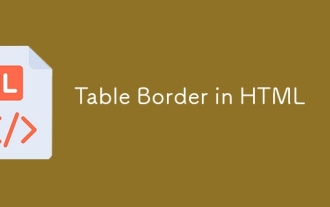
Guide to Table Border in HTML. Here we discuss multiple ways for defining table-border with examples of the Table Border in HTML.
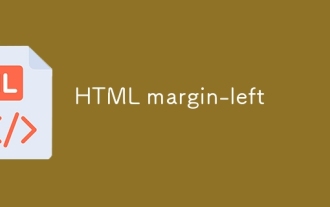
Guide to HTML margin-left. Here we discuss a brief overview on HTML margin-left and its Examples along with its Code Implementation.
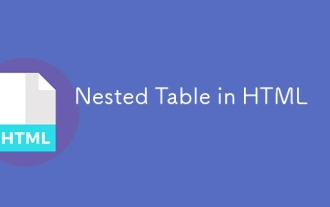
This is a guide to Nested Table in HTML. Here we discuss how to create a table within the table along with the respective examples.
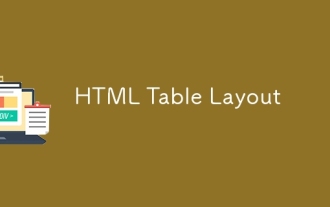
Guide to HTML Table Layout. Here we discuss the Values of HTML Table Layout along with the examples and outputs n detail.
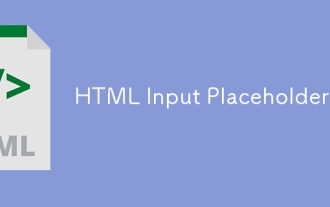
Guide to HTML Input Placeholder. Here we discuss the Examples of HTML Input Placeholder along with the codes and outputs.
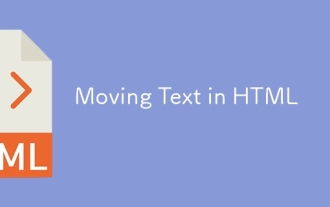
Guide to Moving Text in HTML. Here we discuss an introduction, how marquee tag work with syntax and examples to implement.
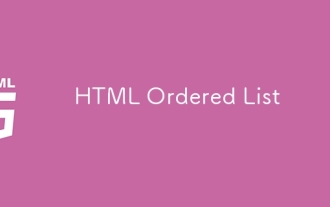
Guide to the HTML Ordered List. Here we also discuss introduction of HTML Ordered list and types along with their example respectively
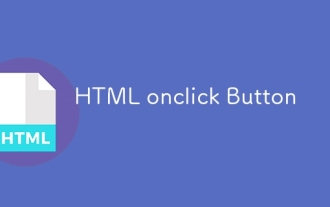
Guide to HTML onclick Button. Here we discuss their introduction, working, examples and onclick Event in various events respectively.