PHP coding standards and suggestions for newbies
If you want to be an excellent programmer, good coding standards are very important. So how can you write good code? In this article, we will give you some PHP coding standards and suggestions.
Coding specifications
PHP code files must start with the
<?php //开头 // 不结尾
PHP code files must be encoded in UTF-8 without BOM.
例sublime, setting增加,"show_encoding" : true
The number of characters per line should not exceed 80 characters
例,sublime "word_wrap": "true", "wrap_width": 80,
Tap key 4 spaces
例,sublime "tab_size": 4,
Only classes (traits) should be defined in PHP code /Function/Constant/Other operations that will produce side effects (such as generating file output and modifying .ini configuration files, etc.), you can only choose one.
例, a.php class A { } b.php function demo() { } c.php define('A', value); d.php ini_set('some_vars', value);
The naming of classes/trait/Interface must follow the StudlyCaps camel case naming convention starting with an uppercase letter.
class StudlyCaps { } trait StudlyCaps { } Interface StudlyCaps { }
Constants in a class must have all letters in capital letters, and words separated by underscores.
define('FOO_BAR', 'something more'); const FOO_BAR = value;
The method name (in class/trait) must conform to the camelCase-style camel case naming convention starting with lowercase.
class StudlyCaps { public function studlyCaps() { // coding... } }
Function names must conform to the snake_case-style underscore naming convention.
function snake_case() { // coding... }
Private (private) method (in class/trait) name must conform to _camelCase-style leading underscore lowercase beginning camel case Naming conventions.
class StudlyCaps { private function _studlyCaps() { // coding... } }
The first word of the method name is a verb.
class StudlyCaps { public function doSomething() { // coding... } }
Variables must conform to the camelCase-style naming convention of camel case starting with lowercase.
class StudlyCaps { public function doSomething() { $someVariable = 'demo'; // coding... } }
When a method/function has multiple parameters, there must be a space between them
class StudlyCaps { public function doSomething($variableOne, $variableTwo) { // coding... } }
- ##Operator/expression must have a space
$a = $b + $c; $a = $b . $c;
- each A blank line must be inserted after the namespace declaration block and the use declaration block.
namespace Standard; // 空一行 use Test\TestClass;//use引入类 // 空一行
- The opening curly brace "{"must be written on a line after the function declaration, and the closing curly brace"}" is also It must be written on its own line after the function body.
class StudlyCaps { }
- The opening curly brace { of the method/function must be written on its own line after the function declaration, and the closing curly brace} must also be written On a line of its own after the function body.
class StudlyCaps { public function studlyCaps() { // coding... } } function snake_case() { // coding... }
- The attributes and methods of the class must be added with access modifiers (private, protected and public), abstract and final must be declared during access before the modifier, and static must be declared after the access modifier.
abstract class StudlyCaps { abstract public function studlyCaps(); final public static function studlyCapsOne() { } }
- There must be a space after the keyword of the control structure, but there must not be when calling a method or function.
if ($valueOne === $valueTwo) { // code... } switch ($valueThree) { case 'value': // code... break; default: // code... break; } do { // code... } while ($valueFour <= 10); while ($valueFive <= 10) { // code... } for ($i = 0; $i < $valueSix; $i++) { // code... } $demo = new Demo() $demo->doSomething(); do_something();</p></li></ul> <p></p> <ul class=" list-paddingleft-2"><li>The opening brace { of the control structure must be written on the same line as the declaration, and the closing brace } must be written after the body In a line of its own. <p></p> </li></ul> <pre class="brush:php;toolbar:false">if ($valueOne === $valueTwo) { // code... } switch ($valueThree) { case 'value': // code... break; default: // code... break; } do { // code... } while ($valueFour <= 10); while ($valueFive <= 10) { // code... } for ($i = 0; $i < $valueSix; $i++) { // code... }
- There must be no spaces after the opening left bracket and before the closing right bracket of the control structure.
if ($valueOne === $valueTwo) {// 控制结构(右边和)左边不加空格 // code... }
- sql is too long
// heredoc语法 $sql = <<<SQL SELECT delivery_id FROM d_test WHERE delivery_id IN (123,234) GROUP BY delivery_id HAVING SUM(send_number) <= 0; SQL;
- if and other control structure conditions are too long
- Method or function parameters are greater than three Line break
- Chain operation exceeds two
- After array php5.4, use []
- single quotes multiple quotes
- There are no variables in the string, single quotes
- There are variables in the string, double quotes
- Declare a class or method or function, add a description & attribute description & author
- api method provides test sample example
- Use try...catch...
- To call multiple methods (more than 3) continuously, use foreach
- Copyright statement at the top of the file
if ($a > 0 && $b > 0 && $c > 0 && $d > 0 && $e > 0) { }
public function tooLangFunction( $valueOne = '', $valueTwo = '', $valueThree = '', $valueFour = '', $valueFive = '', $valueSix = '') { //coding... }
$this->nameTest->functionOne() ->functionTwo() ->functionThree();
$a = [ 'aaa' => 'aaa', 'bbb' => 'bbb' ];
$str = 'str'; $arg = "$str";
/** * 类描述 * * desc */ class StandardExample { /** * 常量描述. * * @var string */ const THIS_IS_A_CONST = ''; /** * 属性描述. * * @var string */ public $nameTest = ''; /** * 构造函数. * * 构造函数描述 * @author name <email> * @param string $value 形参名称/描述 * @return 返回值类型 返回值描述 * 返回值类型:string,array,object,mixed(多种,不确定的),void(无返回值) */ public function __construct($value = '') { // coding... }
/** * 成员方法名称. * * 成员方法描述 * * @param string $value 形参名称/描述 * * @example domain/api/controller/action?argu1=111&argu2=222 */ public function testFunction($value = '') { // code... }
try { // coding... } catch (\Exception $e) { // coding... }
// 改写doSome为doSomething class StandardExample { /** * 方法列表 * * @var array */ private $_functionList = []; public function __construct($functionList = array()) { $this->_functionList = $value; } public function doSome() { $this->functionOne(); $this->functionTwo(); $this->functionThree(); $this->functionFour(); } public function doSomething() { foreach($this->_functionList as $function) { $this->$function(); } } ... }
// +---------------------------------------------------------------------- // | Company Name xx服务 // +---------------------------------------------------------------------- // | Copyright (c) 2017 http://domain All rights reserved. // +---------------------------------------------------------------------- // | Author: name <email> // +----------------------------------------------------------------------
以上内容就给新手程序员的一些编写代码的规范及建议,希望能帮助到大家。
相关推荐:
The above is the detailed content of PHP coding standards and suggestions for newbies. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


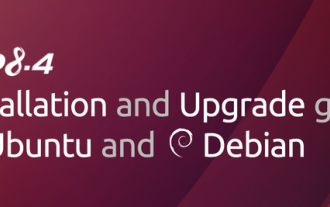
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
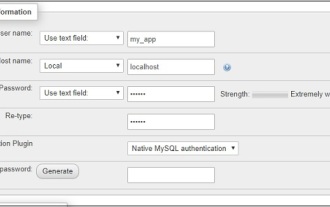
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
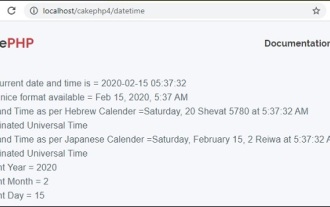
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
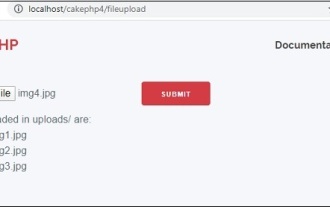
To work on file upload we are going to use the form helper. Here, is an example for file upload.
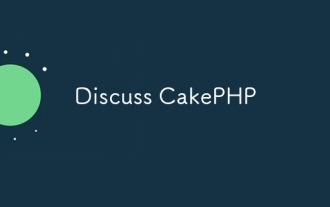
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
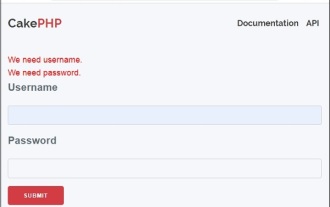
Validator can be created by adding the following two lines in the controller.
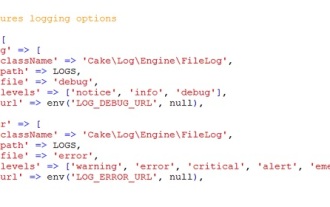
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
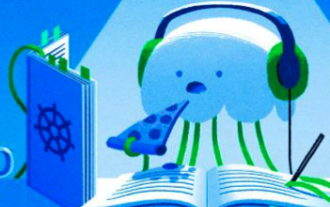
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
