


Introduction to three methods of converting strings into numbers in JavaScript
In our daily development process, no matter what language we are developing, we will encounter the need to convert strings into numbers. I believe many friends know many ways to achieve this, so we Today I will give you a detailed introduction to the three methods of JavaScript to convert strings into numbers!
The value obtained when js reads a text box or other form data is of string type, for example, two text boxes a and b. If the value of a is 11, and the value of b is 9, then a.value should be smaller than b.value, because they are both in the form of strings. I found articles on converting js strings to numbers on the Internet. This is a relatively complete method. There are three main methods
Conversion function, forced
type conversion, use js variable weak type conversion. 1. Conversion function:
js provides two conversion functions: parseInt() and parseFloat(). The former converts the value into an
integer, and the latter converts the value into a floating point number. Only by calling these methods on the String type can these two functions run correctly; for other types, NaN (Not a Number) is returned. Some examples are as follows:
The code is as follows:
parseInt("1234blue"); //returns 1234 parseInt("0xA"); //returns 10 parseInt("22.5"); //returns 22 parseInt("blue"); //returns NaN
The parseInt() method also has a base mode, which can convert binary, octal, hexadecimal or any other base Convert the string to an integer. The base is specified by the second parameter of the parseInt() method. The example is as follows:
The code is as follows:
parseInt("AF", 16); //returns 175 parseInt("10", 2); //returns 2 parseInt("10", 8); //returns 8 parseInt("10", 10); //returns 10
If the decimal number contains leading 0, then it is best to use base 10, so that Don't accidentally get octal values. For example:
The code is as follows:
parseInt("010"); //returns 8 parseInt("010", 8); //returns 8 parseInt("010", 10); //returns 10
The parseFloat() method is similar to the parseInt() method.
Another difference in using the parseFloat() method is that the string must represent a floating point number in decimal form, and parseFloat() has no base mode.
The following is an example of using the parseFloat() method:
The code is as follows:
parseFloat("1234blue"); //returns 1234.0 parseFloat("0xA"); //returns NaN parseFloat("22.5"); //returns 22.5 parseFloat("22.34.5"); //returns 22.34 parseFloat("0908"); //returns 908 parseFloat("blue"); //returns NaN
2. Forced type conversion
You can also use forced type conversion ( type casting) handles converting the type of a value. Use a cast to access a specific value, even if it is of another type.
The three forced type conversions available in ECMAScript are as follows:Boolean(value) - Convert the given value into Boolean type;
Number(value) - Convert the given value into a number (Can be an integer or floating point number);
String(value) - Convert the given value into a string.
Using one of these three functions to convert a value will create a new value that stores the value directly converted from the original value. This can have unintended consequences.
The Boolean() function returns true when the value to be converted is a string of at least one character, a non-zero number, or an
object
(this is discussed in the next section). If the value is an empty string, the number 0, undefined, or null, it returns false. You can use the following code snippet to test the forced type conversion of Boolean.
The code is as follows:
Boolean(""); //false – empty string Boolean("hi"); //true – non-empty string Boolean(100); //true – non-zero number Boolean(null); //false - null Boolean(0); //false - zero Boolean(new Object()); //true – object
The forced type conversion of Number() is similar to the parseInt() and parseFloat() methods, except that it converts the entire value instead of part of the value. The example is as follows:
The code is as follows:
Usage result
Number(false) 0 Number(true) 1 Number(undefined) NaN Number(null) 0 Number( "5.5 ") 5.5 Number( "56 ") 56 Number( "5.6.7 ") NaN Number(new Object()) NaN Number(100) 100
The last forced type conversion method, String(), is the simplest, the example is as follows:
The code is as follows:
var s1 = String(null); //"null" var oNull = null; var s2 = oNull.toString(); //won't work, causes an error
3. Use weak type conversion of js variables
Give a small example, you will understand it at a glance.
The code is as follows:
<script> var str= '012.345 '; var x = str-0; x = x*1; </script>
The above example takes advantage of the weak type characteristics of js and only performs arithmetic operations to achieve type conversion from string to number. However, this method is not recommended.
Summary: This article introduces to you in detail three methods of converting strings to numbers in JavaScript through code examples. They are all different. You can choose the one that suits you according to your own needs. I hope it will be helpful to your work!
Related recommendations:
Implementation methods for converting js strings into numbers and numbers into strings
JavaScript Advanced (4) Convert js string to number
PHP Statistics Chinese Characters Length of string Convert string to number
The above is the detailed content of Introduction to three methods of converting strings into numbers in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


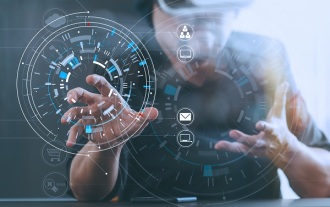
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
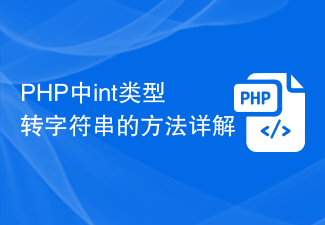
Detailed explanation of the method of converting int type to string in PHP In PHP development, we often encounter the need to convert int type to string type. This conversion can be achieved in a variety of ways. This article will introduce several common methods in detail, with specific code examples to help readers better understand. 1. Use PHP’s built-in function strval(). PHP provides a built-in function strval() that can convert variables of different types into string types. When we need to convert int type to string type,
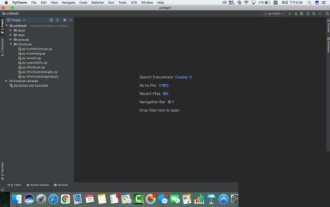
1. First open pycharm and enter the pycharm homepage. 2. Then create a new python script, right-click - click new - click pythonfile. 3. Enter a string, code: s="-". 4. Then you need to repeat the symbols in the string 20 times, code: s1=s*20. 5. Enter the print output code, code: print(s1). 6. Finally run the script and you will see our return value at the bottom: - repeated 20 times.
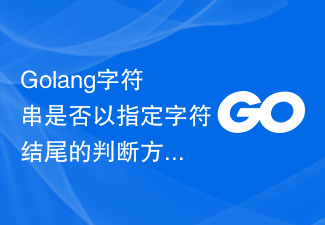
Title: How to determine whether a string ends with a specific character in Golang. In the Go language, sometimes we need to determine whether a string ends with a specific character. This is very common when processing strings. This article will introduce how to use the Go language to implement this function, and provide code examples for your reference. First, let's take a look at how to determine whether a string ends with a specified character in Golang. The characters in a string in Golang can be obtained through indexing, and the length of the string can be
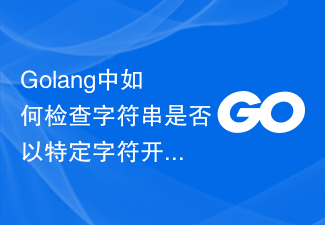
How to check if a string starts with a specific character in Golang? When programming in Golang, you often encounter situations where you need to check whether a string begins with a specific character. To meet this requirement, we can use the functions provided by the strings package in Golang to achieve this. Next, we will introduce in detail how to use Golang to check whether a string starts with a specific character, with specific code examples. In Golang, we can use HasPrefix from the strings package
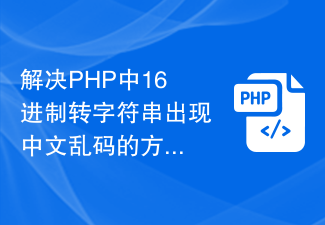
Methods to solve Chinese garbled characters when converting hexadecimal strings in PHP. In PHP programming, sometimes we encounter situations where we need to convert strings represented by hexadecimal into normal Chinese characters. However, in the process of this conversion, sometimes you will encounter the problem of Chinese garbled characters. This article will provide you with a method to solve the problem of Chinese garbled characters when converting hexadecimal to string in PHP, and give specific code examples. Use the hex2bin() function for hexadecimal conversion. PHP’s built-in hex2bin() function can convert 1
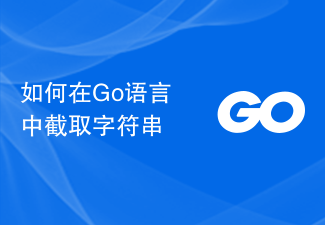
Go language is a powerful and flexible programming language that provides rich string processing functions, including string interception. In the Go language, we can use slices to intercept strings. Next, we will introduce in detail how to intercept strings in Go language, with specific code examples. 1. Use slicing to intercept a string. In the Go language, you can use slicing expressions to intercept a part of a string. The syntax of slice expression is as follows: slice:=str[start:end]where, s
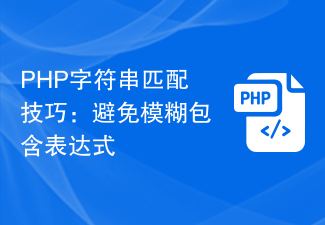
PHP String Matching Tips: Avoid Ambiguous Included Expressions In PHP development, string matching is a common task, usually used to find specific text content or to verify the format of input. However, sometimes we need to avoid using ambiguous inclusion expressions to ensure match accuracy. This article will introduce some techniques to avoid ambiguous inclusion expressions when doing string matching in PHP, and provide specific code examples. Use preg_match() function for exact matching In PHP, you can use preg_mat
