Js intercepts global ajax request
Have you ever had the following needs: need to add a unified signature to all ajax requests, need to count the number of times a certain interface is requested, need to limit the method of http requests to get or post, need to analyze other people's network protocols Wait, so how? Think about it, if you can intercept all ajax requests, then the problem will become very simple!
How to use
1. Directly introduce the script
-
Introduce ajaxhook.js
<script src="wendu.ajaxhook.js"></script>
Copy after login -
Intercept the required ajax callback or function.
hookAjax({ //拦截回调 onreadystatechange:function(xhr){ console.log("onreadystatechange called: %O",xhr) }, onload:function(xhr){ console.log("onload called: %O",xhr) }, //拦截函数 open:function(arg){ console.log("open called: method:%s,url:%s,async:%s",arg[0],arg[1],arg[2]) } })
Copy after login
ok, we use the get method of jQuery (v3.1) to test:
// get current page source code $.get().done(function(d){ console.log(d.substr(0,30)+"...") })
Result:
> open called: method:GET,url:http://localhost:63342/Ajax-hook/demo.html,async:true > onload called: XMLHttpRequest > <!DOCTYPE html> <html> <head l...
The interception was successful! We can also see that jQuery3.1 has abandoned onreadystatechange and used onload instead.
2. In the module building tool environment under CommonJs
Assuming that it is under webpack, the first step is to install the ajax-hook npm plug-in
npm install ajax-hook --save-dev
The second step is to introduce the module And call the api:
const ah=require("ajax-hook") ah.hookAjax({ onreadystatechange:function(xhr){ ... }, onload:function(xhr){ ... }, ... }) ... ah.unHookAjax()
API
hookAjax(ob)
ob, the type is an object, and the key is the callback or function you want to intercept. value is our interception function.
Return value: Original XMLHttpRequest. If you have a write request and don't want to be intercepted, you can use new this.
unHookAjax()
Uninstall interception; after uninstallation, interception will be invalid.
Change ajax behavior
Intercept all ajax requests, detect the request method, if it is "GET", interrupt the request and give a prompt
hookAjax({ open:function(arg){ if(arg[0]=="GET"){ console.log("Request was aborted! method must be post! ") return true; } } })
Intercept all ajax requests and add timestamps to the requests
hookAjax({ open:function(arg){ arg[1]+="?timestamp="+Date.now(); } })
Modify the data "responseText" returned by the request
hookAjax({ onload:function(xhr){ //请求到的数据首部添加"hook!" xhr.responseText="hook!"+xhr.responseText; } })
Result:
hook!<!DOCTYPE html> <html> <h...
With these examples, I believe it was mentioned at the beginning requirements are easily fulfilled. Finally, test the output of unHook
hookAjax({ onreadystatechange:function(xhr){ console.log("onreadystatechange called: %O",xhr) //return true }, onload:function(xhr){ console.log("onload called") xhr.responseText="hook"+xhr.responseText; //return true; }, open:function(arg){ console.log("open called: method:%s,url:%s,async:%s",arg[0],arg[1],arg[2]) arg[1]+="?hook_tag=1"; }, send:function(arg){ console.log("send called: %O",arg[0]) } }) $.get().done(function(d){ console.log(d.substr(0,30)+"...") //use original XMLHttpRequest console.log("unhook") unHookAjax() $.get().done(function(d){ console.log(d.substr(0,10)) }) })
:
open called: method:GET,url:http://localhost:63342/Ajax-hook/demo.html,async:true send called: null onload called hook<!DOCTYPE html> <html> <he... unhook <!DOCTYPE
Note
The return value of the interception function is a boolean. If it is true, the ajax request will be blocked. , the default is false, and the request will not be blocked.
The parameters of all callback interception functions are the current XMLHttpRequest instance, such as onreadystatechange, onload; all interception functions of the ajax original method will pass the original parameters to the interception function in the form of an array, You can modify this in the interceptor function.
Related recommendations:
ajax callback to open a new form to prevent the browser from intercepting effective methods
About using JavaScript to intercept the form's submit method implementation
The above is the detailed content of Js intercepts global ajax request. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


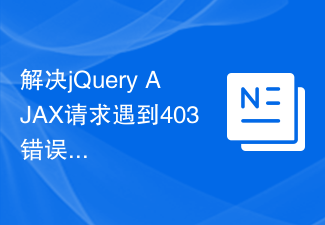
Title: Methods and code examples to resolve 403 errors in jQuery AJAX requests. The 403 error refers to a request that the server prohibits access to a resource. This error usually occurs because the request lacks permissions or is rejected by the server. When making jQueryAJAX requests, you sometimes encounter this situation. This article will introduce how to solve this problem and provide code examples. Solution: Check permissions: First ensure that the requested URL address is correct and verify that you have sufficient permissions to access the resource.
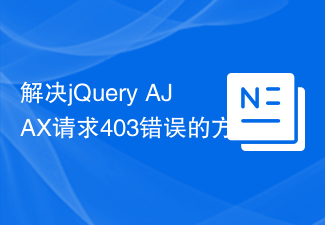
jQuery is a popular JavaScript library used to simplify client-side development. AJAX is a technology that sends asynchronous requests and interacts with the server without reloading the entire web page. However, when using jQuery to make AJAX requests, you sometimes encounter 403 errors. 403 errors are usually server-denied access errors, possibly due to security policy or permission issues. In this article, we will discuss how to resolve jQueryAJAX request encountering 403 error
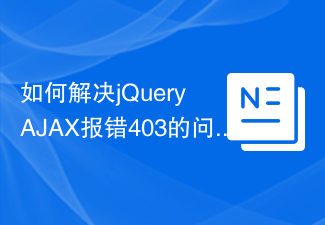
How to solve the problem of jQueryAJAX error 403? When developing web applications, jQuery is often used to send asynchronous requests. However, sometimes you may encounter error code 403 when using jQueryAJAX, indicating that access is forbidden by the server. This is usually caused by server-side security settings, but there are ways to work around it. This article will introduce how to solve the problem of jQueryAJAX error 403 and provide specific code examples. 1. to make
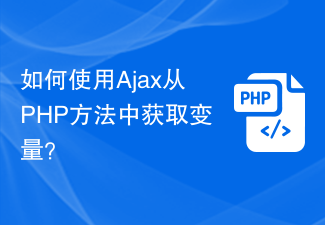
Using Ajax to obtain variables from PHP methods is a common scenario in web development. Through Ajax, the page can be dynamically obtained without refreshing the data. In this article, we will introduce how to use Ajax to get variables from PHP methods, and provide specific code examples. First, we need to write a PHP file to handle the Ajax request and return the required variables. Here is sample code for a simple PHP file getData.php:
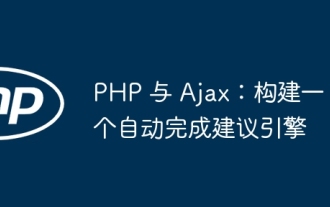
Build an autocomplete suggestion engine using PHP and Ajax: Server-side script: handles Ajax requests and returns suggestions (autocomplete.php). Client script: Send Ajax request and display suggestions (autocomplete.js). Practical case: Include script in HTML page and specify search-input element identifier.
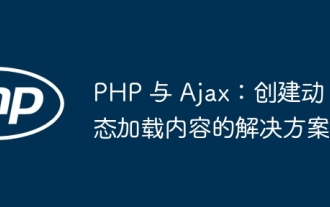
Ajax (Asynchronous JavaScript and XML) allows adding dynamic content without reloading the page. Using PHP and Ajax, you can dynamically load a product list: HTML creates a page with a container element, and the Ajax request adds the data to that element after loading it. JavaScript uses Ajax to send a request to the server through XMLHttpRequest to obtain product data in JSON format from the server. PHP uses MySQL to query product data from the database and encode it into JSON format. JavaScript parses the JSON data and displays it in the page container. Clicking the button triggers an Ajax request to load the product list.
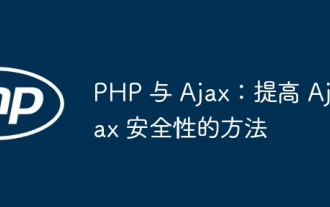
In order to improve Ajax security, there are several methods: CSRF protection: generate a token and send it to the client, add it to the server side in the request for verification. XSS protection: Use htmlspecialchars() to filter input to prevent malicious script injection. Content-Security-Policy header: Restrict the loading of malicious resources and specify the sources from which scripts and style sheets are allowed to be loaded. Validate server-side input: Validate input received from Ajax requests to prevent attackers from exploiting input vulnerabilities. Use secure Ajax libraries: Take advantage of automatic CSRF protection modules provided by libraries such as jQuery.
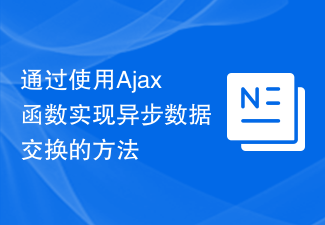
How to use Ajax functions to achieve asynchronous data interaction With the development of the Internet and Web technology, data interaction between the front end and the back end has become very important. Traditional data interaction methods, such as page refresh and form submission, can no longer meet user needs. Ajax (Asynchronous JavaScript and XML) has become an important tool for asynchronous data interaction. Ajax enables the web to use JavaScript and the XMLHttpRequest object
