Yii2 implements QQ Internet login
This article mainly introduces the OAuth extension and QQ interconnection login methods in Yii2, and analyzes the relevant configuration of the OAuth extension and the implementation skills of QQ interconnection login with examples. Friends in need can refer to it. I hope it will be helpful to everyone.
The details are as follows:
php composer.phar require --prefer-dist yiisoft/yii2-authclient "*"
Quick start Quick start
Change the Yii2 configuration file config/main.php and add the following content to components
'components' => [ 'authClientCollection' => [ 'class' => 'yii\authclient\Collection', 'clients' => [ 'google' => [ 'class' => 'yii\authclient\clients\GoogleOpenId' ], 'facebook' => [ 'class' => 'yii\authclient\clients\Facebook', 'clientId' => 'facebook_client_id', 'clientSecret' => 'facebook_client_secret', ], ], ] ... ]
Change the entry file, usually app/controllers/SiteController.php, add code in function actions, and add the callback function successCallback, roughly as follows
class SiteController extends Controller { public function actions() { return [ 'auth' => [ 'class' => 'yii\authclient\AuthAction', 'successCallback' => [$this, 'successCallback'], ], ] } public function successCallback($client) { $attributes = $client->getUserAttributes(); // user login or signup comes here } }
In the logged-in Views, add the following code
<?= yii\authclient\widgets\AuthChoice::widget([ 'baseAuthUrl' => ['site/auth'] ])?>
The above is the official documentation. Now let’s access QQ Internet
To add QQ login components, I put them in common/components/QqOAuth.php. Source The code is as follows
<?php namespace common\components; use yii\authclient\OAuth2; use yii\base\Exception; use yii\helpers\Json; /** * * ~~~ * 'components' => [ * 'authClientCollection' => [ * 'class' => 'yii\authclient\Collection', * 'clients' => [ * 'qq' => [ * 'class' => 'common\components\QqOAuth', * 'clientId' => 'qq_client_id', * 'clientSecret' => 'qq_client_secret', * ], * ], * ] * ... * ] * ~~~ * * @see http://connect.qq.com/ * * @author easypao <admin@easypao.com> * @since 2.0 */ class QqOAuth extends OAuth2 { public $authUrl = 'https://graph.qq.com/oauth2.0/authorize'; public $tokenUrl = 'https://graph.qq.com/oauth2.0/token'; public $apiBaseUrl = 'https://graph.qq.com'; public function init() { parent::init(); if ($this->scope === null) { $this->scope = implode(',', [ 'get_user_info', ]); } } protected function initUserAttributes() { $openid = $this->api('oauth2.0/me', 'GET'); $qquser = $this->api("user/get_user_info", 'GET', ['oauth_consumer_key'=>$openid['client_id'], 'openid'=>$openid['openid']]); $qquser['openid']=$openid['openid']; return $qquser; } protected function defaultName() { return 'qq'; } protected function defaultTitle() { return 'Qq'; } /** * 该扩展初始的处理方法似乎QQ互联不能用,应此改写了方法 * @see \yii\authclient\BaseOAuth::processResponse() */ protected function processResponse($rawResponse, $contentType = self::CONTENT_TYPE_AUTO) { if (empty($rawResponse)) { return []; } switch ($contentType) { case self::CONTENT_TYPE_AUTO: { $contentType = $this->determineContentTypeByRaw($rawResponse); if ($contentType == self::CONTENT_TYPE_AUTO) { //以下代码是特别针对QQ互联登录的,也是与原方法不一样的地方 if(strpos($rawResponse, "callback") !== false){ $lpos = strpos($rawResponse, "("); $rpos = strrpos($rawResponse, ")"); $rawResponse = substr($rawResponse, $lpos + 1, $rpos - $lpos -1); $response = $this->processResponse($rawResponse, self::CONTENT_TYPE_JSON); break; } //代码添加结束 throw new Exception('Unable to determine response content type automatically.'); } $response = $this->processResponse($rawResponse, $contentType); break; } case self::CONTENT_TYPE_JSON: { $response = Json::decode($rawResponse, true); if (isset($response['error'])) { throw new Exception('Response error: ' . $response['error']); } break; } case self::CONTENT_TYPE_URLENCODED: { $response = []; parse_str($rawResponse, $response); break; } case self::CONTENT_TYPE_XML: { $response = $this->convertXmlToArray($rawResponse); break; } default: { throw new Exception('Unknown response type "' . $contentType . '".'); } } return $response; } }
Change the config/main.php file and add it in components, roughly as follows
'components' => [ 'authClientCollection' => [ 'class' => 'yii\authclient\Collection', 'clients' => [ 'qq' => [ 'class'=>'common\components\QqOAuth', 'clientId'=>'your_qq_clientid', 'clientSecret'=>'your_qq_secret' ], ], ] ]
SiteController.php just like the official one
public function successCallback($client) { $attributes = $client->getUserAttributes(); // 用户的信息在$attributes中,以下是您根据您的实际情况增加的代码 // 如果您同时有QQ互联登录,新浪微博等,可以通过 $client->id 来区别。 }
Finally log in Add the QQ login link to the view file
<a href="/site/auth?authclient=qq">使用QQ快速登录</a>
Related recommendations:
Encountered when accessing QQ login OAuth2.0 Pit sharing
oAuth authentication and authorization
Registration of components in Yii2 and Detailed explanation of the creation method
The above is the detailed content of Yii2 implements QQ Internet login. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
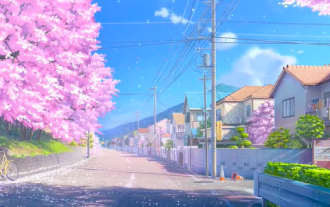
When you log in to someone else's steam account on your computer, and that other person's account happens to have wallpaper software, steam will automatically download the wallpapers subscribed to the other person's account after switching back to your own account. Users can solve this problem by turning off steam cloud synchronization. What to do if wallpaperengine downloads other people's wallpapers after logging into another account 1. Log in to your own steam account, find cloud synchronization in settings, and turn off steam cloud synchronization. 2. Log in to someone else's Steam account you logged in before, open the Wallpaper Creative Workshop, find the subscription content, and then cancel all subscriptions. (In case you cannot find the wallpaper in the future, you can collect it first and then cancel the subscription) 3. Switch back to your own steam
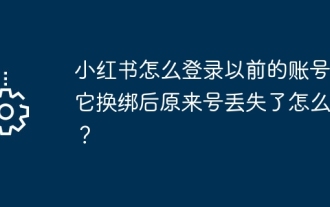
With the rapid development of social media, Xiaohongshu has become a popular platform for many young people to share their lives and explore new products. During use, sometimes users may encounter difficulties logging into previous accounts. This article will discuss in detail how to solve the problem of logging into the old account on Xiaohongshu, and how to deal with the possibility of losing the original account after changing the binding. 1. How to log in to Xiaohongshu’s previous account? 1. Retrieve password and log in. If you do not log in to Xiaohongshu for a long time, your account may be recycled by the system. In order to restore access rights, you can try to log in to your account again by retrieving your password. The operation steps are as follows: (1) Open the Xiaohongshu App or official website and click the "Login" button. (2) Select "Retrieve Password". (3) Enter the mobile phone number you used when registering your account
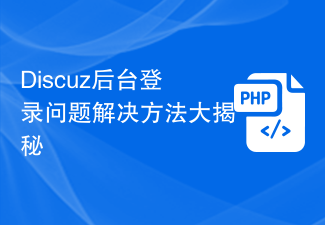
The solution to the Discuz background login problem is revealed. Specific code examples are needed. With the rapid development of the Internet, website construction has become more and more common, and Discuz, as a commonly used forum website building system, has been favored by many webmasters. However, precisely because of its powerful functions, sometimes we encounter some problems when using Discuz, such as background login problems. Today, we will reveal the solution to the Discuz background login problem and provide specific code examples. We hope to help those in need.
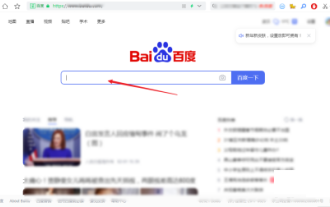
Recently, some friends have asked me how to log in to the Kuaishou computer version. Here is the login method for the Kuaishou computer version. Friends who need it can come and learn more. Step 1: First, search Kuaishou official website on Baidu on your computer’s browser. Step 2: Select the first item in the search results list. Step 3: After entering the main page of Kuaishou official website, click on the video option. Step 4: Click on the user avatar in the upper right corner. Step 5: Click the QR code to log in in the pop-up login menu. Step 6: Then open Kuaishou on your phone and click on the icon in the upper left corner. Step 7: Click on the QR code logo. Step 8: After clicking the scan icon in the upper right corner of the My QR code interface, scan the QR code on your computer. Step 9: Finally log in to the computer version of Kuaishou
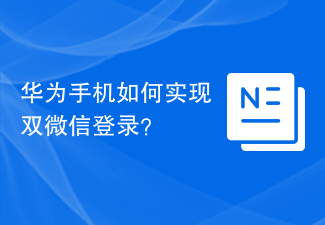
How to implement dual WeChat login on Huawei mobile phones? With the rise of social media, WeChat has become one of the indispensable communication tools in people's daily lives. However, many people may encounter a problem: logging into multiple WeChat accounts at the same time on the same mobile phone. For Huawei mobile phone users, it is not difficult to achieve dual WeChat login. This article will introduce how to achieve dual WeChat login on Huawei mobile phones. First of all, the EMUI system that comes with Huawei mobile phones provides a very convenient function - dual application opening. Through the application dual opening function, users can simultaneously
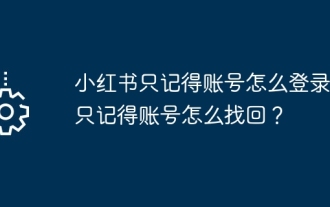
Xiaohongshu has now been integrated into the daily lives of many people, and its rich content and convenient operation methods make users enjoy it. Sometimes, we may forget the account password. It is really annoying to only remember the account but not be able to log in. 1. How to log in if Xiaohongshu only remembers the account? When we forget our password, we can log in to Xiaohongshu through the verification code on our mobile phone. The specific operations are as follows: 1. Open the Xiaohongshu App or the web version of Xiaohongshu; 2. Click the "Login" button and select "Account and Password Login"; 3. Click the "Forgot your password?" button; 4. Enter your account number. Click "Next"; 5. The system will send a verification code to your mobile phone, enter the verification code and click "OK"; 6. Set a new password and confirm. You can also use a third-party account (such as
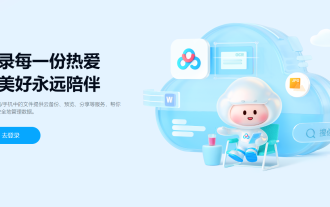
Baidu Netdisk can not only store various software resources, but also share them with others. It supports multi-terminal synchronization. If your computer does not have a client downloaded, you can choose to enter the web version. So how to log in to Baidu Netdisk web version? Let’s take a look at the detailed introduction. Baidu Netdisk web version login entrance: https://pan.baidu.com (copy the link to open in the browser) Software introduction 1. Sharing Provides file sharing function, users can organize files and share them with friends in need. 2. Cloud: It does not take up too much memory. Most files are saved in the cloud, effectively saving computer space. 3. Photo album: Supports the cloud photo album function, import photos to the cloud disk, and then organize them for everyone to view.
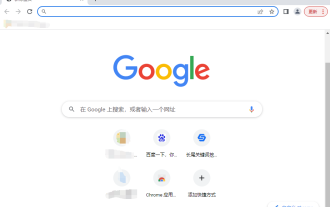
What should I do if I can’t log in to my account on Google Chrome? When many users use this software, certain functions require users to log in to their Google account before they can use it. However, they have tried many times but failed to log in successfully. Faced with this problem, many users do not know how to solve it, so In this issue, the editor is here to share the solution with you. I hope that the content of today’s software tutorial can be helpful to everyone. The solution is as follows: 1. Click on a browser on the desktop, and after opening it, you will see something like this. 2. If a login pops up at this time, click it. If you can't see it, click the upper right corner. 3. Click Login, then enter your account number. You do not need to enter the account after @, and click Next. 4. Enter the password. When you see this prompt, click Enable
