PHP parsing xml format data tool class example sharing
This article mainly introduces the PHP parsing xml format data tool class, involving PHP's related operation skills for adding, obtaining, and parsing xml format data nodes. Friends in need can refer to it. I hope it can help everyone.
The example in this article describes the PHP parsing xml format data tool class. Share it with everyone for your reference, the details are as follows:
class ome_xml { /** * xml资源 * * @var resource * @see xml_parser_create() */ public $parser; /** * 资源编码 * * @var string */ public $srcenc; /** * target encoding * * @var string */ public $dstenc; /** * the original struct * * @access private * @var array */ public $_struct = array(); /** * Constructor * * @access public * @param mixed [$srcenc] source encoding * @param mixed [$dstenc] target encoding * @return void * @since */ function SofeeXmlParser($srcenc = null, $dstenc = null) { $this->srcenc = $srcenc; $this->dstenc = $dstenc; // initialize the variable. $this->parser = null; $this->_struct = array(); } /** * Parses the XML file * * @access public * @param string [$file] the XML file name * @return void * @since */ function xml2array($file) { //$this->SofeeXmlParser('utf-8'); $data = file_get_contents($file); $this->parseString($data); return $this->getTree(); } function xml3array($file){ $data = file_get_contents($file); $this->parseString($data); return $this->_struct; } /** * Parses a string. * * @access public * @param string data XML data * @return void */ function parseString($data) { if ($this->srcenc === null) { $this->parser = xml_parser_create(); } else { if($this->parser = xml_parser_create($this->srcenc)) { return 'Unable to create XML parser resource with '. $this->srcenc .' encoding.'; } } if ($this->dstenc !== null) { @xml_parser_set_option($this->parser, XML_OPTION_TARGET_ENCODING, $this->dstenc) or die('Invalid target encoding'); } xml_parser_set_option($this->parser, XML_OPTION_CASE_FOLDING, 0); // lowercase tags xml_parser_set_option($this->parser, XML_OPTION_SKIP_WHITE, 1); // skip empty tags if (!xml_parse_into_struct($this->parser, $data, $this->_struct)) { /*printf("XML error: %s at line %d", xml_error_string(xml_get_error_code($this->parser)), xml_get_current_line_number($this->parser) );*/ $this->free(); return false; } $this->_count = count($this->_struct); $this->free(); } /** * return the data struction * * @access public * @return array */ function getTree() { $i = 0; $tree = array(); $tree = $this->addNode( $tree, $this->_struct[$i]['tag'], (isset($this->_struct[$i]['value'])) ? $this->_struct[$i]['value'] : '', (isset($this->_struct[$i]['attributes'])) ? $this->_struct[$i]['attributes'] : '', $this->getChild($i) ); unset($this->_struct); return $tree; } /** * recursion the children node data * * @access public * @param integer [$i] the last struct index * @return array */ function getChild(&$i) { // contain node data $children = array(); // loop while (++$i < $this->_count) { // node tag name $tagname = $this->_struct[$i]['tag']; $value = isset($this->_struct[$i]['value']) ? $this->_struct[$i]['value'] : ''; $attributes = isset($this->_struct[$i]['attributes']) ? $this->_struct[$i]['attributes'] : ''; switch ($this->_struct[$i]['type']) { case 'open': // node has more children $child = $this->getChild($i); // append the children data to the current node $children = $this->addNode($children, $tagname, $value, $attributes, $child); break; case 'complete': // at end of current branch $children = $this->addNode($children, $tagname, $value, $attributes); break; case 'cdata': // node has CDATA after one of it's children $children['value'] .= $value; break; case 'close': // end of node, return collected data return $children; break; } } //return $children; } /** * Appends some values to an array * * @access public * @param array [$target] * @param string [$key] * @param string [$value] * @param array [$attributes] * @param array [$inner] the children * @return void * @since */ function addNode($target, $key, $value = '', $attributes = '', $child = '') { if (!isset($target[$key]['value']) && !isset($target[$key][0])) { if ($child != '') { $target[$key] = $child; } if ($attributes != '') { foreach ($attributes as $k => $v) { $target[$key][$k] = $v; } } $target[$key]['value'] = $value; } else { if (!isset($target[$key][0])) { // is string or other $oldvalue = $target[$key]; $target[$key] = array(); $target[$key][0] = $oldvalue; $index = 1; } else { // is array $index = count($target[$key]); } if ($child != '') { $target[$key][$index] = $child; } if ($attributes != '') { foreach ($attributes as $k => $v) { $target[$key][$index][$k] = $v; } } $target[$key][$index]['value'] = $value; } return $target; } /** * Free the resources * * @access public * @return void **/ function free() { if (isset($this->parser) && is_resource($this->parser)) { xml_parser_free($this->parser); unset($this->parser); } }
Related recommendations:
Detailed explanation of PHP's addition, deletion, modification and checking of xml files
php implements output xml attributes
java converts XML documents into json format data
The above is the detailed content of PHP parsing xml format data tool class example sharing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


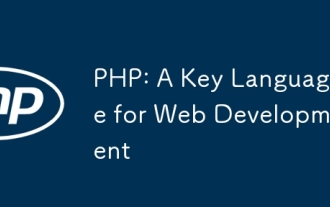
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
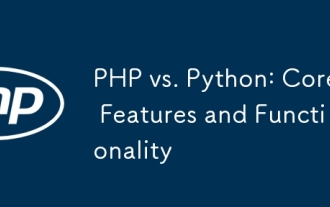
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
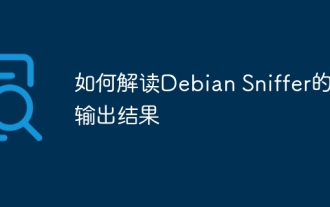
DebianSniffer is a network sniffer tool used to capture and analyze network packet timestamps: displays the time for packet capture, usually in seconds. Source IP address (SourceIP): The network address of the device that sent the packet. Destination IP address (DestinationIP): The network address of the device receiving the data packet. SourcePort: The port number used by the device sending the packet. Destinatio
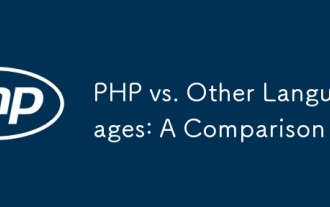
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
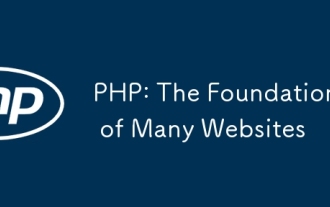
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
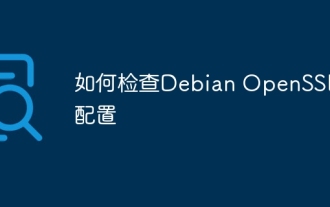
This article introduces several methods to check the OpenSSL configuration of the Debian system to help you quickly grasp the security status of the system. 1. Confirm the OpenSSL version First, verify whether OpenSSL has been installed and version information. Enter the following command in the terminal: If opensslversion is not installed, the system will prompt an error. 2. View the configuration file. The main configuration file of OpenSSL is usually located in /etc/ssl/openssl.cnf. You can use a text editor (such as nano) to view: sudonano/etc/ssl/openssl.cnf This file contains important configuration information such as key, certificate path, and encryption algorithm. 3. Utilize OPE
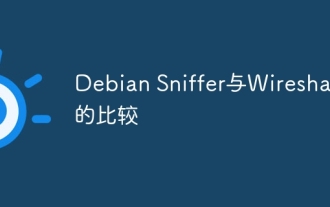
This article discusses the network analysis tool Wireshark and its alternatives in Debian systems. It should be clear that there is no standard network analysis tool called "DebianSniffer". Wireshark is the industry's leading network protocol analyzer, while Debian systems offer other tools with similar functionality. Functional Feature Comparison Wireshark: This is a powerful network protocol analyzer that supports real-time network data capture and in-depth viewing of data packet content, and provides rich protocol support, filtering and search functions to facilitate the diagnosis of network problems. Alternative tools in the Debian system: The Debian system includes networks such as tcpdump and tshark
