


Summary of several methods to delete specific elements from JavaScript arrays
Deleting specified elements from js arrays is a problem that each of us encounters. There is a lot of information on this online, but some are too old and some are not comprehensive enough. So I will organize it myself. This article mainly summarizes and introduces various methods for deleting specific elements in JavaScript arrays. Friends in need can refer to it.
Preface
Maybe when it comes to deleting specific elements of an array, you estimate that there is more than one way to achieve it, so let’s take a look. Check out these methods I’ve summarized, they may be helpful to you! Not much to say, let’s take a look at the detailed introduction.
Source array
var arr = ["George", "John", "Thomas", "James", "Adrew", "Martin"];
Pseudo deletion
What is pseudo-deletion? That is to say, setting the array element value to null;
arr[ arr.indexOf( 'Thomas' ) ] = null;
The deleted array looks like this:
["George", "John", null, "James", "Adrew", "Martin"]
But please note that this means that arrayArraythat is, the length of variable arr remains unchanged
Delete completely
What is complete deletion? You may also think of this question literally, which is to actually delete the element values of the array Array, and will change the length of the array. You can use the splice method of the built-in array object Array. To realize this requirement! Speaking of the splice method, let’s talk about its specific parameters:
Array.prototype.splice = function(start,deleteCount,items) {};
The above is the prototype definition of the splice method of the built-in object Array. The Chinese meaning is: splicing. The meaning of its parameters is:
start: starting point index value
- ##deleteCount: number of elements to be deleted
- items: elements replaced/appended after deletion
When the parameter is not added, it means deleting the element, and it must be combined with the parameter value of deleteCount
If deleteCount is 1 and a parameter value is given in the items parameter position, it means replacing
If deleteCount is 1 and the items parameter position is given to more than one parameter value, it means replacing and appending elements
arr.splice( arr.indexOf( null ), 1 );
["George", "John", "James", "Adrew", "Martin"]
spliceFunction - Replace elements
["George", "John", "James", "Adrew", "Martin"]
arr.splice( arr.indexOf( 'James' ), 1, 'Tom' );
["George", "John", "Tom", "Adrew", "Martin"]
splice function - replace and append Element
["George", "John", "Tom", "Adrew", "Martin"]
arr.splice( arr.indexOf( 'Tom' ), 1, 'Judy', 'Linda', 'Alisa' );
["George", "John", "Judy", "Linda", "Alisa", "Adrew", "Martin"]
splice function - append elements
["George", "John", "Judy", "Linda", "Alisa", "Adrew", "Martin"]
arr.splice( arr.indexOf( 'Linda' ) + 1, 0, 'Bill', 'Blake' );
["George", "John", "Judy", "Linda", "Bill", "Blake", "Alisa", "Adrew", "Martin"]
- Starting position
arr.indexOf( 'Linda' ) + 1
is after the array element Linda
- The number of deleted elements parameter is set here to 0. This is the key to appending elements, which means that the elements are not deleted.
- 'Bill', 'Blake' This is the built-in The last parameter items of the splice method of the object Array represents 0 or more. The meaning will be different depending on the deleteCount parameter value. Here the deleteCount parameter is 0 and items has two values to represent this parameter, as shown That is to say, append the element value 'Bill', 'Blake'
Delete the first element in the array
arr.shift();
["John", "Judy", "Linda", "Bill", "Blake", "Alisa", "Adrew", "Martin"]
Delete the last element in the array
arr.pop();
["John", "Judy", "Linda", "Bill", "Blake", "Alisa", "Adrew"]
Related recommendations:
Detailed explanation of JavaScript module mode
Various writing methods of javaScript encapsulation
JavaScript Detailed explanation of observer pattern examplesThe above is the detailed content of Summary of several methods to delete specific elements from JavaScript arrays. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
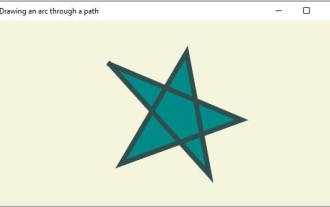
The javafx.scene.shape package provides some classes with which you can draw various 2D shapes, but these are just primitive shapes like lines, circles, polygons and ellipses etc... So if you want to draw complex For custom shapes, you need to use the Path class. Path class Path class You can draw custom paths using this geometric outline that represents a shape. To draw custom paths, JavaFX provides various path elements, all of which are available as classes in the javafx.scene.shape package. LineTo - This class represents the path element line. It helps you draw a straight line from the current coordinates to the specified (new) coordinates. HlineTo - This is the table
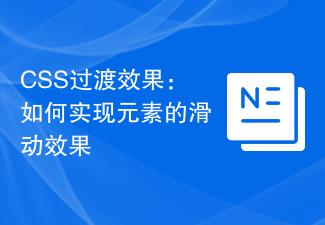
CSS transition effect: How to achieve the sliding effect of elements Introduction: In web design, the dynamic effect of elements can improve the user experience, among which the sliding effect is a common and popular transition effect. Through the transition property of CSS, we can easily achieve the sliding animation effect of elements. This article will introduce how to use CSS transition properties to achieve the sliding effect of elements, and provide specific code examples to help readers better understand and apply. 1. Introduction to CSS transition attribute transition CSS transition attribute tra
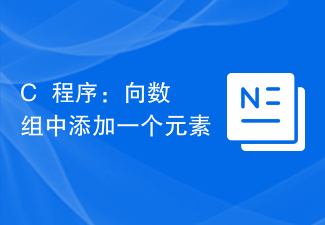
An array is a linear sequential data structure used to hold homogeneous data in contiguous memory locations. Like other data structures, arrays must have the ability to insert, delete, traverse, and update elements in some efficient way. In C++, our arrays are static. C++ also provides some dynamic array structures. For a static array, Z elements may be stored in the array. So far we have n elements. In this article, we will learn how to insert elements at the end of an array (also known as appending elements) in C++. Understand the concept through examples. The usage of ‘this’ keyword is as follows: GivenarrayA=[10,14,65,85,96,12,35,74,69]Afterin
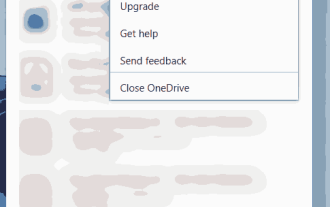
The OneDrive app on your system stores all your files and folders in the cloud. But sometimes users don't want certain files or folders to be stored and take up OneDrive space that is limited to 5 GB without a subscription. To do this, there is a setting in the OneDrive app that allows users to select files or folders to sync on the cloud. If you are also looking for this, then this article will help you select folders or files to sync in OneDrive on Windows 11. How to select certain folders to sync in OneDrive in Windows 11 Note: Make sure the OneDrive app is connected and synced
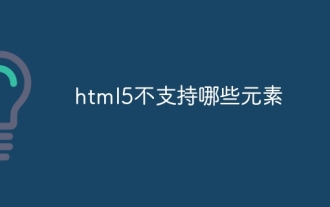
Elements that HTML5 does not support are purely expressive elements, frame-based elements, application elements, replaceable elements and old form elements. Detailed introduction: 1. Purely expressive elements, such as font, center, s, u, etc., these elements are usually used to control text style and layout; 2. Frame-based elements, such as frame, frameset and noframes, these elements are used in In the past, it was used to create web page layouts and split windows; 3. Application-related elements, such as applet, isinde, etc.
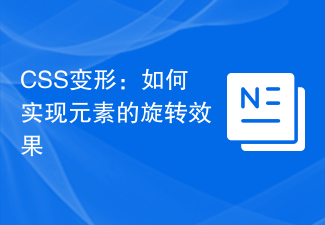
CSS transformation: How to achieve the rotation effect of elements requires specific code examples. In web design, animation effects are one of the important ways to improve user experience and attract user attention, and rotation animation is one of the more classic ones. In CSS, you can use the "transform" attribute to achieve various deformation effects of elements, including rotation. This article will introduce in detail how to use CSS "transform" to achieve the rotation effect of elements, and provide specific code examples. 1. How to use CSS’s “transf
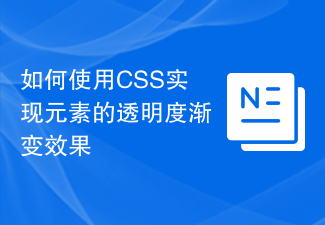
How to use CSS to achieve the transparency gradient effect of elements In web development, adding transition effects to web page elements is one of the important means to improve user experience. The gradient effect of transparency can not only make the page smoother, but also highlight the key content of the element. This article will introduce how to use CSS to achieve the transparency gradient effect of elements and provide specific code examples. Using the CSS transition attribute To achieve the transparency gradient effect of an element, we need to use the CSS transition attribute. t
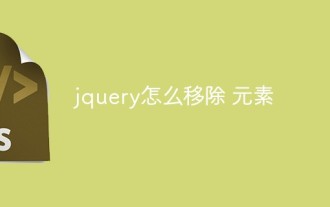
How to remove elements with jquery: 1. Delete the selected element and its sub-elements through the jQuery remove() method. The syntax is "$("#div1").remove();"; 2. Delete through the jQuery empty() method. The child elements of the selected element, the syntax is "$("#div1").empty();".
