Detailed explanation of PHP and AJAX examples
In this article we will share with you the basics of AJAX, PHP and AJAX, JSON format, and AJAX in jQuery. I hope this article can help you.
1.AJAX basics
(1)AJAX (Asynchronous JavaScript and XML): abbreviation for asynchronous javascript and xml.
(2)AJAX is not a programming language, but a technology that can update part of a web page without loading the entire page.
(3) Disadvantages of traditional web pages: If you need to update content or submit a form, you need to reload the entire page; Advantages of using AJAX: By exchanging a small amount of data with the server in the background, the web page can achieve asynchronous partial updates . It has a very good user experience for filling out complex forms.
(4) AJAX synchronization and asynchronousness: Synchronization simply means loading the entire page; asynchronously can load part of the page. Specifically, the connection between the client and the server. Before the advent of Ajax technology, data was exchanged synchronously, which would be very troublesome if you filled out more complex forms. With the XMLHttpRequest object, the synchronous world becomes an asynchronous world. Data can be exchanged with the server through the XMLHttpRequest object in the background.
(5) XMLHttpRequest object creation: var requerst=new XMLHttpRequest();
Just instantiate the object directly! Note: IE5 and IE6 do not support this definition. If you want to implement the browser The page is compatible, as shown in the following code:
var request; if(window.XMLHttpRequest){ request = new XMLHttpRequest(); // IE7+,Firefox,Chrome,Opera,Safari... }else{ request = new ActiveObject("Microsoft.XMLHTTP"); // IE6,IE5 }
(6) HTTP request: http is a stateless protocol: to put it bluntly, it is a protocol that cannot establish persistent connections and has no memory. Further: realize debugging of web pages through the process of page request and response.
(6.1) A complete HTTP request process usually has the following 7 steps:
Establishing a TCP connection
Web browser sends request command to Web server
Web browser sends request header information
Web server response
Web server sends response header information
Web server sends data to browser
-
Web server closes TCP connection
(6.2) http request generally consists of four parts:
HTTP request method or action, such as GET or POST request
The URL being requested must know what the requested address is.
The request header contains some client environment information, authentication information, etc.
The request body is the request body. The request body can include the query payment information, form information, etc. submitted by the customer.
(6.3 )GET and POST[Collect the name value from the form with method="post/get"]
To put it bluntly, they all collect the value passed from the form. The passed values, one is displayed in the form of url (unsafe), and the other is displayed in the form of value (safe); GET is generally used to obtain information, use url to pass parameters , limited to 2000 characters, visible to everyone, not safe. POST is generally used to send data from a form. The parameters are in the http request body and there is no limit on the number of messages sent.
(6.4)Http response generally consists of three parts:
A status code composed of a number and text, used to show whether the request is successful or failed;
Response header, including server type, date and time, content type and length, etc.;
Response body, which is the response text.
(6.5)Http status code consists of three digits, the first digit defines the type of status code:
1XX: Information type , indicating that the web browser request has been received and is being further processed;
2XX: Success, indicating that the user request has been received, understood and processed correctly, for example: 200 OK
#3XX: Redirect, indicating that the request was unsuccessful and the client must take further action
4XX: Client error, indicating that the request submitted by the client has an error , such as 404 NOT FOUND, means that the document referenced in the request does not exist.
5XX: Server error, indicating that the server cannot complete the processing of the request.
(7)XMLHttpRequest sends a request: (object method)
open(method request method, url request address, async request synchronization or Asynchronous (asynchronous is true, synchronous is false, in fact, the default is true)); ///Call Asynchronous request
send(string);(Use the get method When, the parameters can be left unfilled or null, because the information is included in the address bar url; when using post, it must be filled in); // Send the request to the server
request.open("POST","create.php",true); request.setRequestHeader("Content-type","application/x-www-form-urlencoded");//设置http的头信息,告诉web服务器要发送一个表单;注意:setRequest请求一定要放在open和send之间,否则会抛出异常 request.send("name=王大锤&sex=男");
responseText: In simple terms : is to receive the data sent back by the server in response. Get the corresponding data as a string.
- responsXML: Obtain the corresponding data in XML form. Now it is generally converted to data in JSON form.
- status and statusText: Return the http status code in numeric and text form.
- getAllResponseHeader(): Get all response headers.
- getResponseHeader(): Query the value of a field in the response.
0:请求未初始化,open还没有调用。
1:服务器连接已建立,open已经调用了。
2:请求已经接收,也就是接收到头信息了。
3:请求处理中,也就是接收到响应主体了。
4:请求已完成,且响应已就绪,也就是响应完成了。
举个栗子:涵盖了ajax的大部分内容(典型的xhr建立ajax的过程)
var request = new XMLHttpRequest();//创建XHR对象 request.open("GET","get.php",true);//调用异步请求 request.send();//发送异步请求 //对事件进行监听,判断服务器是否正确得做出了响应 request.onreadystatechange = function(){ if(request.readyState===4 && request.status === 200){ request.reponseText;//接收服务器响应回送的数据 } }
2.PHP与AJAX
实战:[服务器端与客户端]实现查询员工和新建员工的后台接口
(1)客户端部分代码:
//新建员工客户端代码 document.getElementById("save").onclick=function(){ var request=new XMLHttpRequest();//创建XHR对象 request.open("POST","action.php");//调用异步请求 //data拼接的URL //document.getElementById("staffName").value获取表单中用户写入的值 var data = "username=" + document.getElementById("staffName").value + "&num=" + document.getElementById("staffNumber").value + "&workname=" + document.getElementById("staffJob").value; request.setRequestHeader("Content-type","application/x-www-form-urlencoded");//设置http的头信息 request.send(data);//发送异步请求 //对事件进行监听,判断服务器是否能正确做出响应 request.onreadystatechange=function(){ if(request.readyState===4){ if(request.status===200){ //innerHTML不仅可以读取元素内容,还可以将内容写入元素 //ajax引擎对象通过responseText属性或者responseXML属性接收服务器回送的数据,然后在静态页面中对其进行处理,使页面达到局部刷新的效果 document.getElementById("createResult").innerHTML=request.responseText; }else{ alter("发生错误:"+request.status); } } } }
(2)服务器端部分代码:
//查询员工服务器端代码 <?php header('Content-type:text/html;charset=utf8'); $con=mysqli_connect("127.0.0.1","",""); mysqli_select_db($con,"function"); $sql="SELECT * FROM `ajax`"; $query=mysqli_query($con,$sql); //数据库数据的总条数$number $number=mysqli_num_rows($query); for($i=0;$i<$number;$i++){ //将每条数据转换成关联数组打印出来 $arr=mysqli_fetch_row($query); //print_r($arr); //echo $arr[2];die; if($_GET['num']==$arr[2]){ echo "找到员工:编号$arr[2],姓名:$arr[1],职位:$arr[3]"; break; } } ?>
3.JSON格式
(1)json:javascript对象表示法。
(2)json是存储和交换文本信息的语法,类似xml。采用键值对的方式组织,易于人们阅读与机器解析。
(3)json是独立于语言的,不管什么语言都可以解析json,只要按json的规则来就行。
(4)json的长度比xml小;json读写的速度更快;json可以使用js内建方法直接解析,转化成js对象,非常方便。
(5)json语法规则:json数据的书写格式是:名称/值对。
名称/值对组合中的名称写在前面(在双引号中),值对写在后面(同样在双引号中),中间用冒号隔开,比如"name":"王大锤"
。注意:json与javaScript对象表示法不同,javaScript对象表示法的键值不需要用引号,但是json的键值要用引号。
注意:json可以是整型、浮点型、字符串(在双引号中)、布尔值(true或false)、数组(在方括号中)、对象(在花括号中)、null等数据类型。
举个栗子:
{//定义了一个json对象 "staff":[//定义了一个数组 {"name":"王大锤","age":21},//定义了一个name对象 {"name":"叫兽","age":35} ] }
(6)json解析:eval()和JSON.parse()两种方式解析成JSON形式
两种方法比较:建议使用JSON.parse()方法解析成JSON形式
eval();不安全,如果json中有函数或js程序代码(alert或一个window.location.href()跳转链接病毒网站等),会优先执行代码,危险操作。JSON.parse();对json文件具有校验功能,如果json文件里面有程序脚本,会解析报错。
举个栗子:
var jsondata='{ "staff":[{ "name":"王大锤", "age":22 }, { "name":"叫兽", "age":23 }, { "name":"王尼玛", "age":24 } ] }'; var jsonobj=JSON.parse(jsondata);//JSON.parse解析JSON var jsonobj=eval('(' + jsondata + ')');//eval解析JSON alert(jsonobj.staff[0].name);
注意:上面书写是错误的,只为了看清楚。逗号不是分隔符,逗号是文本内容,所有的都应该紧凑写,不能自己为了看清楚,人为用空格分开。(这里找了一个小时错误...)
(7)json验证工具:JSONLint
(8)举个栗子:
使用json,首先需要服务器端的约定, 用此种方法能够减少更多的判断,以更加优雅的形式显示 { //前端规则验证、后端数据验证 "success":true,//是否正确执行(表单等规则验证) "msg":"×××"//请求的响应值是否成功(http响应返回的信息) }
服务器端部分代码:
//echo "参数错误,员工信息填写不全"; echo '{"success":false,"msg":"参数错误,员工信息填写不全"}'; if($query){ //echo "员工:" . $_POST["username"] . " 信息保存成功!"; echo '{"success":true,"msg":"员工保存成功"}'; }else{ //echo "员工:" . $_POST["username"] . " 信息保存失败!"; echo '{"success":false,"msg":"员工保存失败"}'; }
客户端json代码:其它不变,将服务器端响应传过来的responseText(文本形式)转换为(JSON形式),将json字符串转化为了一个json对象data,然后就能够以对象的形式处理数据
request.onreadystatechange=function(){ if(request.readyState===4){ if(request.status===200){ //将服务器端响应传过来的responseText(文本形式)转换为(JSON形式) //将json字符串转化为了一个json对象data var data=JSON.parse(request.responseText); if(data.success){ document.getElementById("createResult").innerHTML=data.msg; }else{ document.getElementById("createResult").innerHTML="出现错误"+data.msg; } } } }
4.jQuery中的AJAX
(1)使用jquery实现ajax请求:作用:避免兼容问题,代码简洁,操作快捷方便。
(2)语法:jQuery.ajax([settings])
type:类型,“POST”或“GET”,默认为“GET”。
url:发送请求的地址。
data:是一个对象,连同请求发送到服务器的数据。
dataType:预期服务器返回的数据类型。如果不指定,jQuery将自动根据HTTP包MIME信息来智能判断,一般我们采用json格式,可以设置为“json”。
success:是一个方法,请求成功后的毁掉函数。传入返回后的数据,以及包含成功代码的字符串。
error:是一个方法,请求失败时调用此函数。传入XMLHttpRequest对象。
相关推荐:
The above is the detailed content of Detailed explanation of PHP and AJAX examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


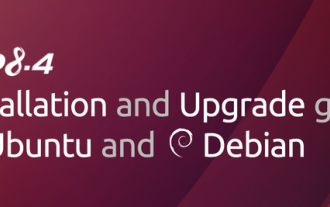
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
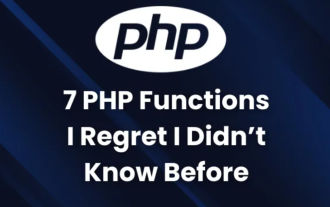
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
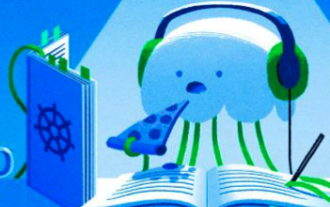
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
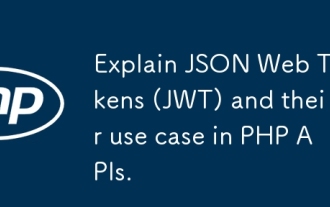
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
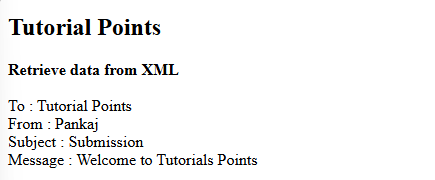
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
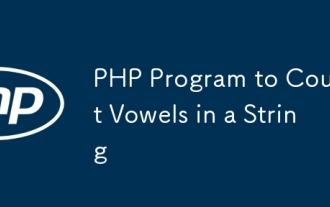
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
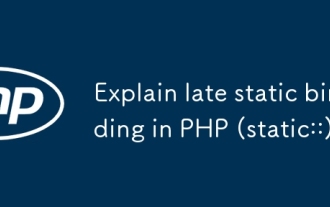
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
