Practical analysis of writing React component projects
Because the design idea of React is extremely unique, it is a revolutionary innovation, has outstanding performance, and the code logic is very simple. Therefore, more and more people are paying attention to and using it. This article shares with you the entire process of writing React component project practices through examples. Friends who are interested in this can refer to it.
When I first started writing React, I saw many ways to write components. There are a hundred ways to write a hundred tutorials. While React itself has matured, there doesn't seem to be a "right" way to use it. So I summarize here the experience of using React that our team has accumulated over the years. I hope this article is useful to you, whether you are a beginner or a veteran.
Before you start:
We use ES6 and ES7 syntax. If you are not very clear about the difference between display components and container components, it is recommended that you start by reading this article. If you have any suggestions or questions Duqing left a message in the comments about class-based components
Nowadays, React components are generally developed using class-based components. Next we will write our component in the same line:
import React, { Component } from 'react'; import { observer } from 'mobx-react'; import ExpandableForm from './ExpandableForm'; import './styles/ProfileContainer.css';
I like css in javascript. However, this method of writing styles is still too new. So we introduce css files in each component. Moreover, locally introduced imports and global imports will be separated by a blank line.
Initializing State
import React, { Component } from 'react' import { observer } from 'mobx-react' import ExpandableForm from './ExpandableForm' import './styles/ProfileContainer.css' export default class ProfileContainer extends Component { state = { expanded: false }
You can use the old method to initialize state
in constructor
. More related information can be found here. But we choose a clearer approach.
Also, we make sure to add export default
in front of the class. (Translator's note: Although this may not be correct when using redux).
propTypes and defaultProps
import React, { Component } from 'react' import { observer } from 'mobx-react' import { string, object } from 'prop-types' import ExpandableForm from './ExpandableForm' import './styles/ProfileContainer.css' export default class ProfileContainer extends Component { state = { expanded: false } static propTypes = { model: object.isRequired, title: string } static defaultProps = { model: { id: 0 }, title: 'Your Name' } // ... }
propTypes
and defaultProps
are static properties. Define it as early as possible in the component class so that other developers can notice it immediately when reading the code. They can serve as documentation.
If you use React 15.3.0 or higher, you need to introduce the prop-types
package instead of using React.PropTypes
. More content moves here.
All your components should have prop types.
Method
import React, { Component } from 'react' import { observer } from 'mobx-react' import { string, object } from 'prop-types' import ExpandableForm from './ExpandableForm' import './styles/ProfileContainer.css' export default class ProfileContainer extends Component { state = { expanded: false } static propTypes = { model: object.isRequired, title: string } static defaultProps = { model: { id: 0 }, title: 'Your Name' } handleSubmit = (e) => { e.preventDefault() this.props.model.save() } handleNameChange = (e) => { this.props.model.changeName(e.target.value) } handleExpand = (e) => { e.preventDefault() this.setState({ expanded: !this.state.expanded }) } // ... }
In class components, when you pass methods to child components, you need to make sure They are called with the correct this. This is usually done when passing it to a child component: this.handleSubmit.bind(this)
.
It’s much simpler to use the ES6 arrow method. It automatically maintains the correct context (this
).
Pass a method to setState
In the above example, there is this line:
this.setState({ expanded: !this.state.expanded });
setState
is actually asynchronous! In order to improve performance, React will call setState
that is called multiple times together. Therefore, the state may not change immediately after calling setState
.
So, when calling setState
, you cannot rely on the current state value. Because i doesn't know its value at all.
Solution: Pass a method to setState
, and pass the state value before the call as a parameter to this method. Take a look at the example:
this.setState(prevState => ({ expanded: !prevState.expanded }))
Thanks to Austin Wood for the help.
Disassemble the component
import React, { Component } from 'react' import { observer } from 'mobx-react' import { string, object } from 'prop-types' import ExpandableForm from './ExpandableForm' import './styles/ProfileContainer.css' export default class ProfileContainer extends Component { state = { expanded: false } static propTypes = { model: object.isRequired, title: string } static defaultProps = { model: { id: 0 }, title: 'Your Name' } handleSubmit = (e) => { e.preventDefault() this.props.model.save() } handleNameChange = (e) => { this.props.model.changeName(e.target.value) } handleExpand = (e) => { e.preventDefault() this.setState(prevState => ({ expanded: !prevState.expanded })) } render() { const { model, title } = this.props return () } }
{title}
If there are multiple lines of props
, each prop should occupy a separate line. Just like the above example. The best way to achieve this goal is to use a set of tools: Prettier
.
Decorator
@observer export default class ProfileContainer extends Component {
If you know some libraries, such as mobx, you can use the above example way to modify class components. A decorator is a method that passes a class component as a parameter.
Decorators allow you to write more flexible and readable components. If you don’t want to use a decorator, you can do this:
class ProfileContainer extends Component { // Component code } export default observer(ProfileContainer)
Closure
Try to avoid passing closures in child components, such as:
<input type="text" value={model.name} // onChange={(e) => { model.name = e.target.value }} // ^ Not this. Use the below: onChange={this.handleChange} placeholder="Your Name"/>
Note: If input
is a React component, this will automatically trigger its redrawing, regardless of whether other props have changed.
Consistency checking is the most resource-consuming part of React. Don't add extra work here. The best way to handle the problem in the above example is to pass in a class method, which will be more readable and easier to debug. For example:
import React, { Component } from 'react' import { observer } from 'mobx-react' import { string, object } from 'prop-types' // Separate local imports from dependencies import ExpandableForm from './ExpandableForm' import './styles/ProfileContainer.css' // Use decorators if needed @observer export default class ProfileContainer extends Component { state = { expanded: false } // Initialize state here (ES7) or in a constructor method (ES6) // Declare propTypes as static properties as early as possible static propTypes = { model: object.isRequired, title: string } // Default props below propTypes static defaultProps = { model: { id: 0 }, title: 'Your Name' } // Use fat arrow functions for methods to preserve context (this will thus be the component instance) handleSubmit = (e) => { e.preventDefault() this.props.model.save() } handleNameChange = (e) => { this.props.model.name = e.target.value } handleExpand = (e) => { e.preventDefault() this.setState(prevState => ({ expanded: !prevState.expanded })) } render() { // Destructure props for readability const { model, title } = this.props return (// Newline props if there are more than two ) } }
{title}
{ model.name = e.target.value }} // Avoid creating new closures in the render method- use methods like below onChange={this.handleNameChange} placeholder="Your Name"/>
Method component
This type of component has no state, no props, and no methods. They are pure components that contain minimal changes. Use them often.
propTypes
import React from 'react' import { observer } from 'mobx-react' import { func, bool } from 'prop-types' import './styles/Form.css' ExpandableForm.propTypes = { onSubmit: func.isRequired, expanded: bool } // Component declaration
We define propTypes
before the declaration of the component.
Decompose Props and defaultProps
import React from 'react' import { observer } from 'mobx-react' import { func, bool } from 'prop-types' import './styles/Form.css' ExpandableForm.propTypes = { onSubmit: func.isRequired, expanded: bool, onExpand: func.isRequired } function ExpandableForm(props) { const formStyle = props.expanded ? {height: 'auto'} : {height: 0} return ( <form style={formStyle} onSubmit={props.onSubmit}> {props.children} <button onClick={props.onExpand}>Expand</button> </form> ) }
Our component is a method. Its parameters are props
. We can extend this component like this:
import React from 'react' import { observer } from 'mobx-react' import { func, bool } from 'prop-types' import './styles/Form.css' ExpandableForm.propTypes = { onSubmit: func.isRequired, expanded: bool, onExpand: func.isRequired } function ExpandableForm({ onExpand, expanded = false, children, onSubmit }) { const formStyle = expanded ? {height: 'auto'} : {height: 0} return ( <form style={formStyle} onSubmit={onSubmit}> {children} <button onClick={onExpand}>Expand</button> </form> ) }
现在我们也可以使用默认参数来扮演默认props的角色,这样有很好的可读性。如果expanded
没有定义,那么我们就把它设置为false
。
但是,尽量避免使用如下的例子:
const ExpandableForm = ({ onExpand, expanded, children }) => {
看起来很现代,但是这个方法是未命名的。
如果你的Babel配置正确,未命名的方法并不会是什么大问题。但是,如果Babel有问题的话,那么这个组件里的任何错误都显示为发生在 <>里的,这调试起来就非常麻烦了。
匿名方法也会引起Jest其他的问题。由于会引起各种难以理解的问题,而且也没有什么实际的好处。我们推荐使用function
,少使用const
。
装饰方法组件
由于方法组件没法使用装饰器,只能把它作为参数传入别的方法里。
import React from 'react' import { observer } from 'mobx-react' import { func, bool } from 'prop-types' import './styles/Form.css' ExpandableForm.propTypes = { onSubmit: func.isRequired, expanded: bool, onExpand: func.isRequired } function ExpandableForm({ onExpand, expanded = false, children, onSubmit }) { const formStyle = expanded ? {height: 'auto'} : {height: 0} return ( <form style={formStyle} onSubmit={onSubmit}> {children} <button onClick={onExpand}>Expand</button> </form> ) } export default observer(ExpandableForm)
只能这样处理:export default observer(ExpandableForm)
。
这就是组件的全部代码:
import React from 'react' import { observer } from 'mobx-react' import { func, bool } from 'prop-types' // Separate local imports from dependencies import './styles/Form.css' // Declare propTypes here, before the component (taking advantage of JS function hoisting) // You want these to be as visible as possible ExpandableForm.propTypes = { onSubmit: func.isRequired, expanded: bool, onExpand: func.isRequired } // Destructure props like so, and use default arguments as a way of setting defaultProps function ExpandableForm({ onExpand, expanded = false, children, onSubmit }) { const formStyle = expanded ? { height: 'auto' } : { height: 0 } return ( <form style={formStyle} onSubmit={onSubmit}> {children} <button onClick={onExpand}>Expand</button> </form> ) } // Wrap the component instead of decorating it export default observer(ExpandableForm)
某些情况下,你会做很多的条件判断:
<p id="lb-footer"> {props.downloadMode && currentImage && !currentImage.video && currentImage.blogText ? !currentImage.submitted && !currentImage.posted ? <p>Please contact us for content usage</p> : currentImage && currentImage.selected ? <button onClick={props.onSelectImage} className="btn btn-selected">Deselect</button> : currentImage && currentImage.submitted ? <button className="btn btn-submitted" disabled>Submitted</button> : currentImage && currentImage.posted ? <button className="btn btn-posted" disabled>Posted</button> : <button onClick={props.onSelectImage} className="btn btn-unselected">Select post</button> } </p>
这么多层的条件判断可不是什么好现象。
有第三方库JSX-Control Statements可以解决这个问题。但是与其增加一个依赖,还不如这样来解决:
<p id="lb-footer"> { (() => { if(downloadMode && !videoSrc) { if(isApproved && isPosted) { return <p>Right click image and select "Save Image As.." to download</p> } else { return <p>Please contact us for content usage</p> } } // ... })() } </p>
使用大括号包起来的IIFE,然后把你的if
表达式都放进去。返回你要返回的组件。
相关推荐:
The above is the detailed content of Practical analysis of writing React component projects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


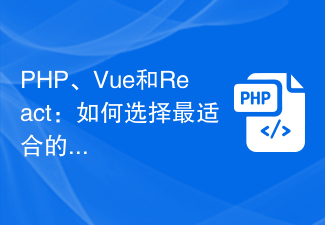
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
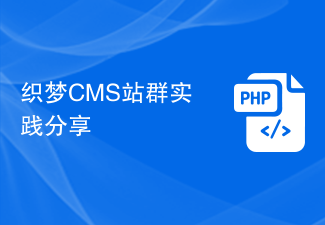
Dream Weaver CMS Station Group Practice Sharing In recent years, with the rapid development of the Internet, website construction has become more and more important. When building multiple websites, site group technology has become a very effective method. Among the many website construction tools, Dreamweaver CMS has become the first choice of many website enthusiasts due to its flexibility and ease of use. This article will share some practical experience about Dreamweaver CMS station group, as well as some specific code examples, hoping to provide some help to readers who are exploring station group technology. 1. What is Dreamweaver CMS station group? Dream Weaver CMS
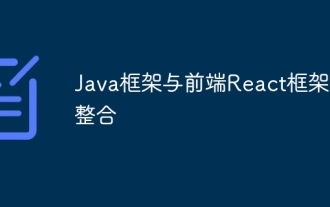
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
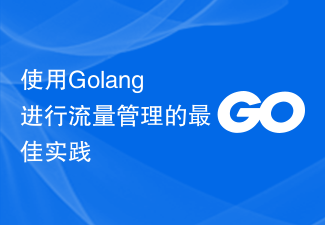
Golang is a powerful and efficient programming language that is widely used to build web services and applications. In network services, traffic management is a crucial part. It can help us control and optimize data transmission on the network and ensure the stability and performance of services. This article will introduce the best practices for traffic management using Golang and provide specific code examples. 1. Use Golang’s net package for basic traffic management. Golang’s net package provides a way to handle network data.
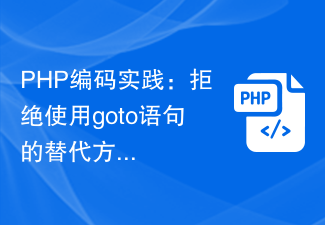
PHP Coding Practices: Refusal to Use Alternatives to Goto Statements In recent years, with the continuous updating and iteration of programming languages, programmers have begun to pay more attention to coding specifications and best practices. In PHP programming, the goto statement has existed as a control flow statement for a long time, but in practical applications it often leads to a decrease in the readability and maintainability of the code. This article will share some alternatives to help developers refuse to use goto statements and improve code quality. 1. Why refuse to use goto statement? First, let's think about why
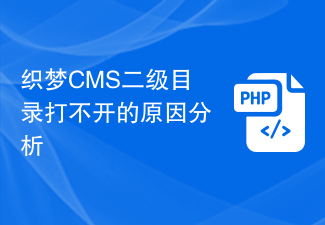
Title: Analysis of the reasons and solutions for why the secondary directory of DreamWeaver CMS cannot be opened. Dreamweaver CMS (DedeCMS) is a powerful open source content management system that is widely used in the construction of various websites. However, sometimes during the process of building a website, you may encounter a situation where the secondary directory cannot be opened, which brings trouble to the normal operation of the website. In this article, we will analyze the possible reasons why the secondary directory cannot be opened and provide specific code examples to solve this problem. 1. Possible cause analysis: Pseudo-static rule configuration problem: during use
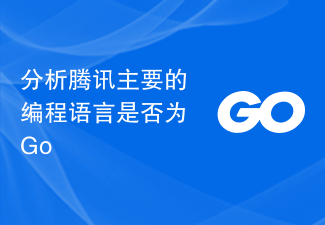
Title: Is Tencent’s main programming language Go: An in-depth analysis. As China’s leading technology company, Tencent has always attracted much attention in its choice of programming languages. In recent years, some people believe that Tencent mainly adopts Go as its main programming language. This article will conduct an in-depth analysis of whether Tencent's main programming language is Go, and give specific code examples to support this view. 1. Application of Go language in Tencent Go is an open source programming language developed by Google. Its efficiency, concurrency and simplicity are loved by many developers.
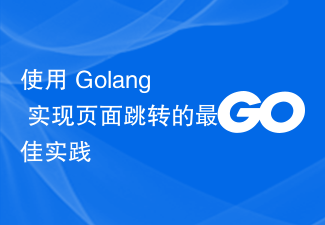
Best practices for using Golang to implement page jumps When developing web applications, page jumps are a common functional requirement. In Golang, we can use some libraries to implement page jumps, such as using the Gin framework to handle routing and page jumps. This article will introduce the best practices on how to implement page jumps in Golang, and give specific code examples. Introduction to Gin framework Gin is a web framework written in Go language, which is powerful and easy to use.
