Detailed explanation of passing and copying in js
We know that js has several basic data types and other complex data types including (objects, arrays, functions). The assignment of basic data types is actually a copy of the value. We call it value transfer, and the variable after assignment There is no other relationship with the original variable except that the value is equal.
let x = 666 let y = x let m = 'abc' let n = m y = 888 n = 'def' console.log(x, y)//666,888 console.log(m, n)//'abc','def'
The transfer of complex data types is not like this, because when a variable is bound to a complex data type, what is recorded is not the value of the complex data, but an address where the data is stored. Information, when assigning this variable to another variable, only the address is passed. These two variables actually point to a data information. When any variable is changed, the other variables will be affected. This kind of transfer The method is called pass by reference
let obj1 = { a : '1', b : 2 } let obj2 = obj1 obj2.b = 3 console.log(obj1,obj2)//{a: "1", b: 3},{a: "1", b: 3}
Copy
We know that the assignment of complex data types is pass by reference. The variables before and after the assignment will affect each other. In actual projects, we often do not want this to happen. , for example:
We use data (an Array) in two places in a view. One is that a list only needs to display the data in order, and the other is that a chart needs to display the data in reverse order. When doing data processing, we will have a problem at this time. If the first list after data.reverse() is also in reverse order, this is not what we want. We need a method to only copy the values of the array, and this new array The data address of the original array is different. This copy method is called a copy of the array.
let obj1 = {a:1, b:{c:2}} let shallowCopy = (src)=> { let dst = {} for (let prop in src) { if (src.hasOwnProperty(prop)) { dst[prop] = src[prop] } } return dst } let obj2 = shallowCopy(obj1) console.log(obj1,obj2) //@1 obj1.a = 6 console.log(obj2.a) //@2 obj2.b.c = 666 console.log(obj1.b.c) //@3 obj2.b = { c: 888 } console.log(obj1.b.c) //@4
It can be seen from the above example that the first layer attribute of obj1 is a copy of the attribute value, and there is no copy of the inherited address, but the second layer is that the b attribute does share a memory address. This is a shallow copy, but At @4, obj1 has not been affected by obj2 because attribute b is an object. This kind of reassignment by reference transfer will cause the computer to reallocate a new memory to store data and record address information, so at this time obj1. b.c and obj2.b.c are no longer an attribute value of the record
It can also be understood as: copying is for transfer. Direct assignment of complex data types is transfer by reference and cannot be called copying. Copying is For a simple data backup of the original data, the memory address information of the data is not exactly the same. This is because the copy is also divided into shallow copy and deep copy.
The non-nested copy of complex data types means that only the first layer of data information is copied, which is a shallow copy. If the first layer of data has complex data types, the reference passing method is still used. , what is copied is still the address information, and the multi-layer nested copy of array objects etc. implemented through other methods is a deep copy.
Let’s take a look at how arrays and objects implement deep and shallow copies:
Copy of arrays
slice method
let arr1 = [1,2,[3,4]] let arr2 = arr1.slice(0) arr2[2].push(5) arr2.push(6) console.log(arr1,arr2)
Copy after loginCopy after loginconcat method
let arr1 = [1,2,[3,4]] let arr2 = arr1.concat() arr2[2].push(5) arr2.push(6) console.log(arr1,arr2)
Copy after loginCopy after loginfor loop
let arr1 = [1,2,[3,4]] let arr2 = [] for(let i = 0; i<arr1.length; i++){ arr2.push(arr1[i]) } arr2[2].push(5) arr2.push(6) console.log(arr1,arr2)
Copy after login…Operator
let arr1 = [1,2,[3,4]] let [...arr2] = arr1 arr2[2].push(5) arr2.push(6) console.log(arr1,arr2)
Copy after loginCopy after login
The copies of the above four types of arrays are all shallow copies. To realize the deep copy of the array, it must be implemented recursively
let deepClone = (src)=> { let result (src instanceof Array) ? (result = []) :(result = {}) for (let key in src) { result[key] = (typeof src[key] === 'object') ? deepClone(src[key]) : src[key]//数组和对象的type都是object } return result } let arr1 = [1,2,[3,4]] let arr2 = deepClone(arr1) arr2[2].push(5) arr2.push(6) console.log(arr1,arr2)
It can be found that the methods arr1[2] and arr2[2] are different, the same as above The deep copy method is also applicable to the copy of the object
Object
Universal for loop
let obj1 = {a:1,b:{c:2}} let obj2 = {} for(let key in obj1){ obj2[key] = obj1[key] } obj1.b.c = 6 console.log(obj1,obj2)
Copy after loginCopy after login...operator
let obj1 = {a:1,b:{c:2}} let {...obj2} = obj1 obj1.b.c = 6 console.log(obj1,obj2)
Copy after loginCopy after loginObject.assign()
let obj1 = {a:1,b:{c:2}} let obj2 = Object.assign({},obj1) obj1.b.c = 6 console.log(obj1,obj2)
Copy after login
The above three methods are shallow copies of objects, and we will introduce two types of deep copies of objects. Method:
Convert to string and then back to object
let obj1 = {a:1,b:{c:2}} let obj2 = JSON.parse(JSON.stringify(obj1)) obj1.b.c = 6 console.log(obj1,obj2)
Copy after loginCopy after logindeepClone method is the deepClone method of the above array
Related concepts
Pure function
Given an input to a function and returns a unique output, a function that does not have any impact on the external environment is called a pure function. The variables defined within it will be recycled by the garbage collection mechanism after the function returns.
But if the parameter of the function is an array, object or function, a reference is passed in, and the operation on it will affect the original data. The function written in this way will have incidental effects and make it readable. Sexuality becomes low.
The way to reduce the impact is to make a deep copy of the incoming parameters and assign them to a new variable, so that the original parameters are tampered with.
Let’s look at an example of a pure function:
let pureFunc = (animal)=> { let newAnimal = JSON.parse(JSON.stringify(animal)) newAnimal.type = 'cat' newAnimal.name = 'Miao' return newAnimal } let wang = { type: 'dog', name: 'Wang' } let miao = pureFunc(wang) console.log(wang,miao)
Through the above example, we can see that the value of wang has not been changed by the pure function.
Let’s think about the following example again. If you answer it correctly, it means you have a deep understanding of what this article talks about (remind everyone—>Reassignment of references)
let afterChange = (obj)=>{ obj.a = 6 obj = { a: 8, b: 9 } return obj } let objIns = { a: 1, b: 2 } let objIns2 = afterChange(objIns) console.log(objIns, objIns2)
The above is my understanding of the reference and transmission of js. Please forgive me if there is any inappropriateness. Thanks!
You can also read some other articles to deepen your understanding. I recommend this Explaining Value vs. Reference in Javascript.
We often encounter the situation of assigning one variable to another variable in the project. This is actually the transfer of js, but different data types behave differently after assignment. Let's study it together. one time
传递
我们知道js有几种基本的数据类型和其他的复杂数据类型包括(对象,数组,函数),基本数据类型的赋值其实就是值的拷贝,我们称之为值传递,赋值后的变量和原来的变量除了值相等之外并无其他关联
let x = 666 let y = x let m = 'abc' let n = m y = 888 n = 'def' console.log(x, y)//666,888 console.log(m, n)//'abc','def'
复杂数据类型的传递并不是这样子的,因为将一个变量绑定到一个复杂数据类型的时候记录的并不是这个复杂数据的值,而是存放了这个数据的一个地址信息,当将这个变量赋值给另一个变量的时候只是将地址传递了过去,这两个变量指向的其实是一个数据信息,当改变任意一个变量的时候,另外的变量都会受到影响,这种传递方式我们称之为引用传递
let obj1 = { a : '1', b : 2 } let obj2 = obj1 obj2.b = 3 console.log(obj1,obj2)//{a: "1", b: 3},{a: "1", b: 3}
拷贝
我们知道复杂数据类型的赋值是引用传递,赋值前后的变量会相互影响,在实际项目中我们经常不希望这样子,譬如:
我们在一个view里面有两处用到了data(是一个Array),其一是一个list只要把data按顺序展示即可,其二是一个chart需要把data逆序之后再做数据处理,这时候我们就犯难了,如果直接data.reverse()之后第一处的列表也是逆序的了,这不是我们想见的,我们需要一个方法只复制数组的值,并且这个新数组和原数组的数据地址并不一样,这种复制方式我们称为数组的拷贝。
let obj1 = {a:1, b:{c:2}} let shallowCopy = (src)=> { let dst = {} for (let prop in src) { if (src.hasOwnProperty(prop)) { dst[prop] = src[prop] } } return dst } let obj2 = shallowCopy(obj1) console.log(obj1,obj2) //@1 obj1.a = 6 console.log(obj2.a) //@2 obj2.b.c = 666 console.log(obj1.b.c) //@3 obj2.b = { c: 888 } console.log(obj1.b.c) //@4
上面的例子可以看出来obj1的第一层属性是复制属性值,没有继承地址的拷贝,但是第二层就是b属性确实共享一块内存地址的,这就是浅拷贝,但是在@4处obj1却没有收到obj2的影响,是因为属性b是一个对象,这种引用传递的重新赋值,计算机会重新分配一块新的内存来存放数据和记录地址信息,所以这时obj1.b.c和obj2.b.c已经不是记录的一个属性值了
也可以理解为:拷贝是之于传递的,直接对复杂数据类型进行赋值是引用传递,不能称之为拷贝,拷贝是对原数据的单纯的数据备份,数据的内存地址信息并不完全一样,这是因为拷贝还分为浅拷贝和深拷贝。
对复杂数据类型的非嵌套的拷贝,就是只拷贝第一层的数据信息的拷贝是浅拷贝,如果第一层的数据有复杂数据类型,则依然采用引用传递的方式,复制的仍然是地址信息,通过其他方式实现的数组对象等的多层嵌套拷贝就是深拷贝。
下面我们再来看下数组和对象如何来实现深浅拷贝:
数组的拷贝
slice方法
let arr1 = [1,2,[3,4]] let arr2 = arr1.slice(0) arr2[2].push(5) arr2.push(6) console.log(arr1,arr2)
Copy after loginCopy after loginconcat方法
let arr1 = [1,2,[3,4]] let arr2 = arr1.concat() arr2[2].push(5) arr2.push(6) console.log(arr1,arr2)
Copy after loginCopy after loginfor循环
let arr1 = [1,2,[3,4]] let arr2 = [] for(let i = 0; i<arr1.length; i++){ arr2.push(arr1[i]) } arr2[2].push(5) arr2.push(6) console.log(arr1,arr2)
Copy after login…运算符
let arr1 = [1,2,[3,4]] let [...arr2] = arr1 arr2[2].push(5) arr2.push(6) console.log(arr1,arr2)
Copy after loginCopy after login
以上4种数组的拷贝都是浅拷贝,要实现数组的深拷贝就要递归实现
let deepClone = (src)=> { let result (src instanceof Array) ? (result = []) :(result = {}) for (let key in src) { result[key] = (typeof src[key] === 'object') ? deepClone(src[key]) : src[key]//数组和对象的type都是object } return result } let arr1 = [1,2,[3,4]] let arr2 = deepClone(arr1) arr2[2].push(5) arr2.push(6) console.log(arr1,arr2)
可以发现用方面的方法arr1[2]和arr2[2]不一样,同样上面的深拷贝的方法也适用于对象
对象的拷贝
万能的for循环
let obj1 = {a:1,b:{c:2}} let obj2 = {} for(let key in obj1){ obj2[key] = obj1[key] } obj1.b.c = 6 console.log(obj1,obj2)
Copy after loginCopy after login…运算符
let obj1 = {a:1,b:{c:2}} let {...obj2} = obj1 obj1.b.c = 6 console.log(obj1,obj2)
Copy after loginCopy after loginObject.assign()
let obj1 = {a:1,b:{c:2}} let obj2 = Object.assign({},obj1) obj1.b.c = 6 console.log(obj1,obj2)
Copy after login
上面3种方法是对象的浅拷贝,再介绍2种对象的深拷贝的方法:
转为字符串再转回对象
let obj1 = {a:1,b:{c:2}} let obj2 = JSON.parse(JSON.stringify(obj1)) obj1.b.c = 6 console.log(obj1,obj2)
Copy after loginCopy after logindeepClone方法,就是上面的数组的deepClone方法
相关的概念
纯函数
给定函数一个输入返回一个唯一的输出,并且不对外部环境附带任何影响的函数我们称为纯函数,其内定义的变量在函数返回后都会被垃圾回收机制回收掉。
但是如果函数的参数是数组、对象或函数时,传入的是一个引用,对其操作会影响到原来的数据,这样子写的函数会产生附带的影响,使得可读性变低。
降低影响的方式就是对传入参数进行深度拷贝,并赋给一个新的变量,方式原来的参数被篡改。
我们来看一个纯函数的例子:
let pureFunc = (animal)=> { let newAnimal = JSON.parse(JSON.stringify(animal)) newAnimal.type = 'cat' newAnimal.name = 'Miao' return newAnimal } let wang = { type: 'dog', name: 'Wang' } let miao = pureFunc(wang) console.log(wang,miao)
通过上面的例子可以看到wang并没有被纯函数所改变值。
大家再来思考一下下面的例子,如果答对了说明你已经对本文所讲的东西有了很深刻的理解了(提醒大家一下—>引用的重新赋值)
let afterChange = (obj)=>{ obj.a = 6 obj = { a: 8, b: 9 } return obj } let objIns = { a: 1, b: 2 } let objIns2 = afterChange(objIns) console.log(objIns, objIns2)
以上就是我对js的引用和传递的理解,如有不当之处敬请谅解,Thanks!
相关推荐:
The above is the detailed content of Detailed explanation of passing and copying in js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
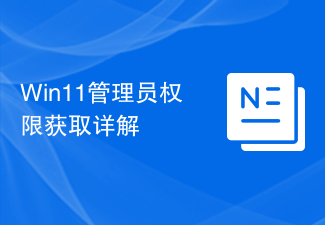
Windows operating system is one of the most popular operating systems in the world, and its new version Win11 has attracted much attention. In the Win11 system, obtaining administrator rights is an important operation. Administrator rights allow users to perform more operations and settings on the system. This article will introduce in detail how to obtain administrator permissions in Win11 system and how to effectively manage permissions. In the Win11 system, administrator rights are divided into two types: local administrator and domain administrator. A local administrator has full administrative rights to the local computer
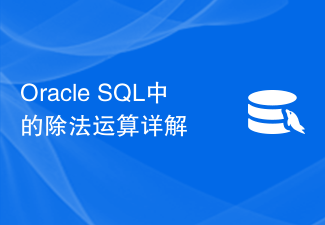
Detailed explanation of division operation in OracleSQL In OracleSQL, division operation is a common and important mathematical operation, used to calculate the result of dividing two numbers. Division is often used in database queries, so understanding the division operation and its usage in OracleSQL is one of the essential skills for database developers. This article will discuss the relevant knowledge of division operations in OracleSQL in detail and provide specific code examples for readers' reference. 1. Division operation in OracleSQL
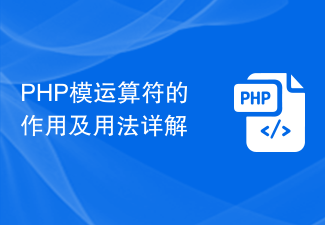
The modulo operator (%) in PHP is used to obtain the remainder of the division of two numbers. In this article, we will discuss the role and usage of the modulo operator in detail, and provide specific code examples to help readers better understand. 1. The role of the modulo operator In mathematics, when we divide an integer by another integer, we get a quotient and a remainder. For example, when we divide 10 by 3, the quotient is 3 and the remainder is 1. The modulo operator is used to obtain this remainder. 2. Usage of the modulo operator In PHP, use the % symbol to represent the modulus
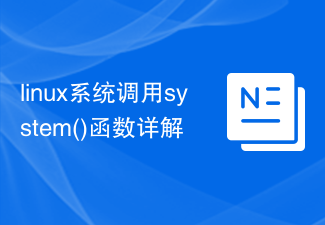
Detailed explanation of Linux system call system() function System call is a very important part of the Linux operating system. It provides a way to interact with the system kernel. Among them, the system() function is one of the commonly used system call functions. This article will introduce the use of the system() function in detail and provide corresponding code examples. Basic Concepts of System Calls System calls are a way for user programs to interact with the operating system kernel. User programs request the operating system by calling system call functions
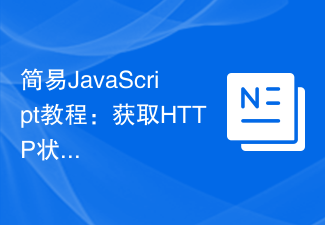
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
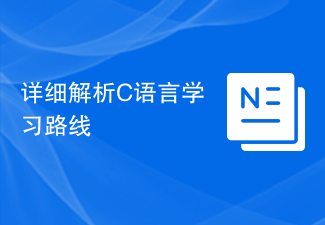
As a programming language widely used in the field of software development, C language is the first choice for many beginner programmers. Learning C language can not only help us establish the basic knowledge of programming, but also improve our problem-solving and thinking abilities. This article will introduce in detail a C language learning roadmap to help beginners better plan their learning process. 1. Learn basic grammar Before starting to learn C language, we first need to understand the basic grammar rules of C language. This includes variables and data types, operators, control statements (such as if statements,
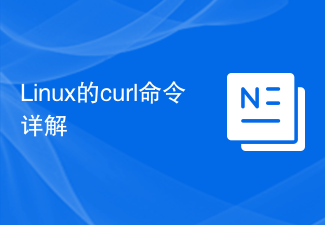
Detailed explanation of Linux's curl command Summary: curl is a powerful command line tool used for data communication with the server. This article will introduce the basic usage of the curl command and provide actual code examples to help readers better understand and apply the command. 1. What is curl? curl is a command line tool used to send and receive various network requests. It supports multiple protocols, such as HTTP, FTP, TELNET, etc., and provides rich functions, such as file upload, file download, data transmission, proxy
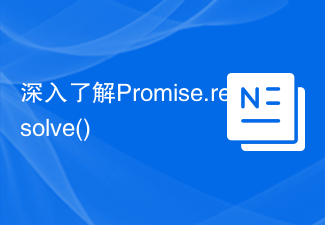
Detailed explanation of Promise.resolve() requires specific code examples. Promise is a mechanism in JavaScript for handling asynchronous operations. In actual development, it is often necessary to handle some asynchronous tasks that need to be executed in sequence, and the Promise.resolve() method is used to return a Promise object that has been fulfilled. Promise.resolve() is a static method of the Promise class, which accepts a
