A closer look at scope and variables in JavaScript
This article talks about the scope and variables in JavaScript. If you don’t know about the scope and variables in JavaScript or are interested in the scope and variables in JavaScript, then we will Get up and read this article. Okay, without further ado, let’s get to the point
Variable Scope
##Scope: It is the area where variables are declared and the accessible scope of variables and functions. Variables declared globally are globally visible and accessible. If variables declared inside a function are only accessible within the function, they are called local variables.
A few points to note:
1.There is no block-level scope in JavaScript (in ES5 and before ES6) , only function scope and global scope. Variables defined inside the for loop have function-level scope.
2. If a variable is not declared within a function or is declared without var, it is a global variable and has a global scope. Special: var a = b = c = 0; b and c are global variables.
3.The scope of the variable is based on the time it is declared, because the scope of the variable is in the parsing stage of the js code The formulation of the rules has been completed.
Simple case:
//变量的作用域 var t = 90; //全局作用域,在js代码中任何一个地方都可以访问 function f1(){ //f1函数 在全局作用域中 var t2 = 10; //t2是f1函数内部变量,只有在f1内可访问 console.log(t2); function f2(){ //f2函数 在f1函数的作用域中 var t3 = 20;//只能在f2函数内部才能访问 console.log(t3); return t2*t3; // 访问了父级作用域中的t3 } return f2(); } var m = f1(); console.log(m);
2. No block-level scope
#I mentioned earlier that there is no block-level scope in JavaScript. This is for es5 and es6 before 9 (let const, etc.). for loop, while loopThe scope of the variables defined in it is the function-level scope.
For example:
//没有块级作用域 function f1(){ for(var i=0;i<10;i++){//i变量是在for中定义的 console.log(i);//打印1-9 } console.log(i);//可以访问到i变量 打印10 而在c++ Java等语言中是不行的 } f1();
3. Variable promotion ( hositing)
When the js engine executes the js code, it will first create the global EC (context) and functions EC (if there is a function), when creating the EC, the variables declared in the current application domain have been initialized to undefined. How to initialize it? The js engine will first look for the variable definition of var in the current scope. If it finds this definition, then
will raise it to the front of the scope and save it in memory (that is, the variable object VO in EC) , set to undefined.
Case:
var a = 10; function f1(){ //在这里首先会创建f1的执行上下文 并把里面的变量初始化为undefined console.log(a); //代码执行到这里的时候, js引擎会去当前作用域内存中问有没有这个变量的声明,发现有,那么就给他初始的undefined //假如说下面没有var变量进行定义a,那么js就会向父级作用域中去找这个变量,直到找到为止 var a = 19; //在这里给a赋值了19 console.log(a); // 打印了19 } f1(); console.log(a); //这里无疑是10 没什么问题
So, When the js engine creates a context, it will promote variables when needed. It can be said to be a security protection mechanism, which is discussed in detail in ES6.
Note: When the variable declaration and function declaration have the same name, the function has higher priority.
console.log(b); //打印b(){} var b = 9; function b(){ } console.log(b); //打印9
Since the function is promoted to the front, what is printed at the beginning is undoubtedly b(){}, because js is dynamic Language, reassign b to 9, overwriting the previous function.
Case 1:if ("a" in window) { var a = 1; } console.log(a);
First I saw your code, what is your answer? Could it be Uncaught ReferenceError: a is not defined?
Tell you, the answer is 1
First of all, you must know that var a = 1 actually defines a global variable (belonging to the attributes under the window object), because if is not a block scope, and there was no block scope in JavaScript before es5. So this
conditional judgmentis established. Look again:
if (!("a" in window)) { var a = 1; } console.log(a);
So what should this be? The answer is undefined, because the condition is not established and the assignment to a is not successful. The default is undefined
案例二:
fun(); console.log(a); console.log(b); console.log(c); function fun(){ var a = b = c = 10; console.log("fun中的a="+a); console.log("fun中的b="+b); console.log("fun中的c="+c); }
你得答案是什么?
答案是:
由于a没有定义,所以直接报错,下面的两行代码被阻止执行了,假如把外面的console.log(a)注释掉呢?
fun(); //console.log(a); console.log(b); console.log(c); function fun(){ var a = b = c = 10; console.log("fun中的a="+a); console.log("fun中的b="+b); console.log("fun中的c="+c); }
输出的是:
为什么外面b c都会是10呢? 原因就是var a = b = c = 10 ;其中b c就是全局变量,如果你想定义三个内部变量,那么应该这样定义:
var a = 10 ,b = 10, c = 10;
弄懂了以上这些区别,基本上变量提升就没什么大问题了。以上就是本篇文章的所有内容,大家要是还不太了解的话,可以自己多实现两边就很容易掌握了哦!
相关推荐:
The above is the detailed content of A closer look at scope and variables in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


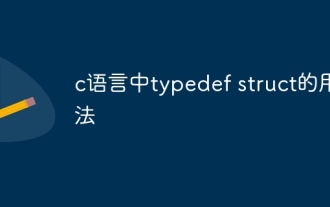
typedef struct is used in C language to create structure type aliases to simplify the use of structures. It aliases a new data type to an existing structure by specifying the structure alias. Benefits include enhanced readability, code reuse, and type checking. Note: The structure must be defined before using an alias. The alias must be unique in the program and only valid within the scope in which it is declared.
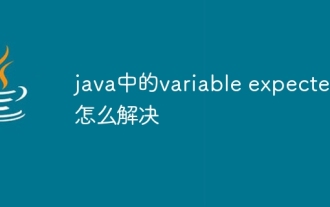
Variable expected value exceptions in Java can be solved by: initializing variables; using default values; using null values; using checks and assignments; and knowing the scope of local variables.
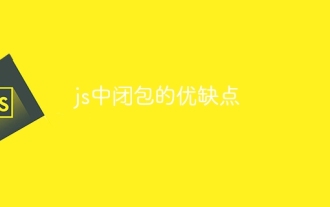
Advantages of JavaScript closures include maintaining variable scope, enabling modular code, deferred execution, and event handling; disadvantages include memory leaks, increased complexity, performance overhead, and scope chain effects.
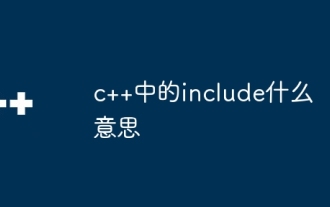
The #include preprocessor directive in C++ inserts the contents of an external source file into the current source file, copying its contents to the corresponding location in the current source file. Mainly used to include header files that contain declarations needed in the code, such as #include <iostream> to include standard input/output functions.
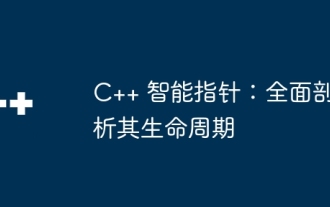
Life cycle of C++ smart pointers: Creation: Smart pointers are created when memory is allocated. Ownership transfer: Transfer ownership through a move operation. Release: Memory is released when a smart pointer goes out of scope or is explicitly released. Object destruction: When the pointed object is destroyed, the smart pointer becomes an invalid pointer.
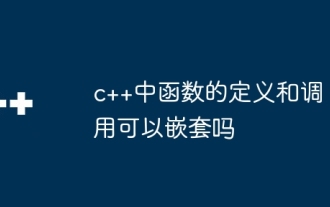
Can. C++ allows nested function definitions and calls. External functions can define built-in functions, and internal functions can be called directly within the scope. Nested functions enhance encapsulation, reusability, and scope control. However, internal functions cannot directly access local variables of external functions, and the return value type must be consistent with the external function declaration. Internal functions cannot be self-recursive.
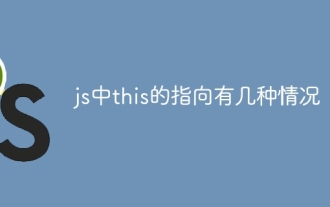
In JavaScript, the pointing types of this include: 1. Global object; 2. Function call; 3. Constructor call; 4. Event handler; 5. Arrow function (inheriting outer this). Additionally, you can explicitly set what this points to using the bind(), call(), and apply() methods.
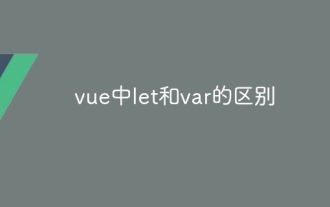
In Vue, there is a difference in scope when declaring variables between let and var: Scope: var has global scope and let has block-level scope. Block-level scope: var does not create a block-level scope, let creates a block-level scope. Redeclaration: var allows redeclaration of variables in the same scope, let does not.
