Analyze the use and principle of sort method in JS
This article mainly analyzes the use and principle of the sort method in JS. Friends who need it can refer to it. I hope it can help everyone. Let’s take a look with the editor below.
Native JS provides two methods for reordering: reverse() and sort(). There is nothing to say about reverse(), which just directly reverses the array, such as the following example:
var arr = [1, 2, 3, 4, 5, -1, -10, 9, 0]; arr.reverse(); alert(arr); //0, 9, -10, -1, 5, 4, 3, 2, 1
The running result is the reverse order of the array, there is nothing to say.
Let’s talk about the sort() method in detail:
The sort method can be called directly without passing in any parameters. You can also pass in a comparison function as a parameter. We will talk about the comparison function later. To explain, let’s first talk about when parameters are not passed.
When no parameters are passed in, the sort() method will call the default sorting method, that is, first call the toString() transformation method of each array item, and then sort the characters according to the string Unicode encoding order. Strings are sorted, such as the following example:
var arr = [1, 2, 3, 15, 22, 33]; arr.sort(); alert(arr); //1, 15, 2, 22, 3, 33
The output result may not be the same as imagined, but it is sorted according to the order of these numbers in the Unicode character set.
So how can we let him sort the array according to our ideas? That's right, you just pass in a function as a parameter to specify the sorting method. (Note that the parameter must be a function, not anything else)
This function can be written as follows:
function cmp (value1, value2) { if (value1 < value2) { return 1; } else if (value1 > value2) { return -1; } else { return 0; } }
Let’s explain this function. If value2 is greater than value1, then 1 will be returned. , that is, true, then the exchange will be performed, so that the larger value2 will be placed in the front, and the smaller value1 will be placed in the back. In this way, the overall arrangement will be sorted from largest to smallest. The following is an example of practical application:
var arr = [1, 2, 3, 15, 22, 33, 44, 55, 0, -1, 22, 55]; function cmp (value1, value2) { if (value1 < value2) { return 1; } else if (value1 > value2) { return -1; } else { return 0; } } arr.sort(cmp); alert(arr);
Running results:
There is also a simplified way to write the cmp function:
function cmp (a, b) { return b - a; }
This function has the same effect as the above function. When b is relatively large, it will return a number greater than or equal to 1, that is, true, so that the exchange will be performed. The overall result is also a descending sort. You can Write the code yourself and try it.
So, now that the numbers in the array have been sorted, how to sort based on a certain attribute value in the array object?
A feature of JS functions that we need to use here is to use functions as return values. You can nest a layer of functions to receive object attribute names, indicating which attribute to sort according to:
<script type="text/javascript"> var arr = [ { name: 'guo', age: 20}, { name: 'yu', age: 19}, { name: 'liu', age: 15} ]; function cmp (property) { return function (a, b){ var value1 = a[property]; var value2 = b[property]; return value1 - value2; } } arr.sort(cmp('age')); for (var i=0; i<3; i++) { alert(arr[i].name); } //liu, yu, guo </script>
Some people may not understand the current cmp function, so you can debug this code to see what is going on:
As you can see, the cmp function The property in only serves as an identifier, but in fact the data in arr is passed to the internal function, and then the parameters of the internal function are used to obtain the values that need to be compared based on the property.
The above is the detailed content of Analyze the use and principle of sort method in JS. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


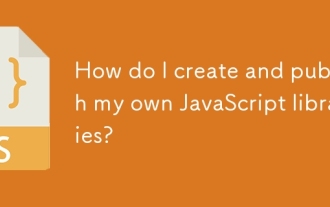
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
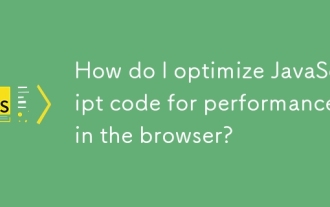
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
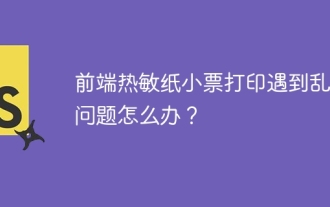
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
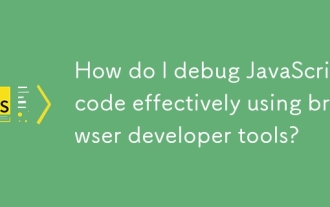
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
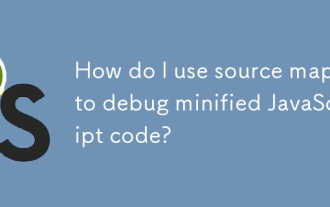
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
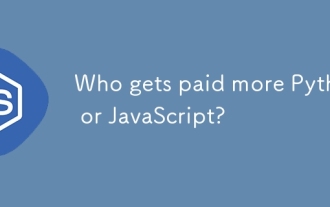
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
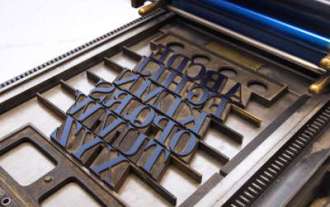
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
