Detailed explanation of new features of php7
This time I will summarize the new features of php7. The following is a practical case. Let’s follow the editor’s footsteps and take a look.
php7 new features
Scalar type declaration (requires the parameter to be a specific type, if the parameter type does not match, a fatal error will occur in php5 , php7 will throw TypeError exception)
There are two modes: mandatory (default) and strict mode.
Type parameters are:
1. string
2. int
3. Float
4. Bool
They expand other types introduced in PHP5
# 1. Class name
2. Interface
3. Array
4. Callback type
For example:
<span style="font-size:18px;">function sumOfInts(int ...$ints) { return array_sum($ints); } var_dump(sumOfInts(2, '3', 4.1));</span>
Output:
int(9)
Return type declaration
php7 adds support for return type, which is very similar to parameter type declaration Similarly, the return type
declaration specifies the type of the function's return value. The available types are the same as those available in the parameter declaration.
For example:
<span style="font-size:18px;"><?php function arraysSum(array ...$arrays): array { return array_map(function(array $array): int { return array_sum($array); }, $arrays); } print_r(arraysSum([1,2,3], [4,5,6], [7,8,9])); 以上例程会输出: Array ( [0] => 6 [1] => 15 [2] => 24 )</span>
NULL coalescing operator
If If the variable exists and the value is not NULL, it will return its own value, otherwise it will return its second parameter
$username = $_GET['user'] ?? 'nobody';
The results of the upper and lower expressions are the same.
$username = isset($_GET['user']) ? $_GET['user'] : 'nobody';
#Combined comparison operators
<span style="font-size:18px;"><?php // Integers echo 1 <=> 1; // 0 echo 1 <=> 2; // -1 echo 2 <=> 1; // 1 // Floats echo 1.5 <=> 1.5; // 0 echo 1.5 <=> 2.5; // -1 echo 2.5 <=> 1.5; // 1 // Strings echo "a" <=> "a"; // 0 echo "a" <=> "b"; // -1 echo "b" <=> "a"; // 1 ?></span>
By define () Define constant array
<?php define('ANIMALS', [ 'dog', 'cat', 'bird' ]); echo ANIMALS[1]; // outputs "cat" ?> php5.6 中 仅能通过const 定义 <?php const $a = [ 'a','b','c', ]; echo $a[1] ; //输出 “b” ?>
Anonymous class ##
Can now support new class To instantiate an anonymous class, this can be used to replace some completion classes that are used only once
Provide filtering for unserialize()<span style="font-size:18px;"><?php
// 把所有的对象转换为 __PHP_Incomplete_Class object
$data = unserialize($foo, ["allowed_classes" => false]);
// 除了MyClass,MyClass2 都转换为 into __PHP_Incomplete_Class object
$data = unserialize($foo, ["allowed_classes" => ["MyClass", "MyClass2"]);
// 默认的行为,接受所有的参数
$data = unserialize($foo, ["allowed_classes" => true]);
</span>
IntlChar
The newly added IntlChar class is designed to expose more ICU functions. This class itself defines many static methods for Manipulate unicode characters in multiple character sets
If you want to use this class, you need to install the Intl extension first
ExpectedThe intention is to use backwards and enhance the previous assert() method, which makes enabling assertions in a production environment cost-free and provides the ability to throw characteristic exceptions when assertions fail.
Now assert() is a language construct that allows the first argument to be an expression rather than just a string to be evaluated or a boolean to be testedUse use to declare a set of classes ## as follows:
<span style="font-size:18px;"><?php // Pre PHP 7 code use some\namespace\ClassA; use some\namespace\ClassB; use some\namespace\ClassC as C; use function some\namespace\fn_a; use function some\namespace\fn_b; use function some\namespace\fn_c; use const some\namespace\ConstA; use const some\namespace\ConstB; use const some\namespace\ConstC; // PHP 7+ code use some\namespace\{ClassA, ClassB, ClassC as C}; use function some\namespace\{fn_a, fn_b, fn_c}; use const some\namespace\{ConstA, ConstB, ConstC}; ?> </span>
is as follows:
<span style="font-size:18px;"><?php $gen = (function() { yield 1; yield 2; return 3; })(); foreach ($gen as $val) { echo $val, PHP_EOL; } echo $gen->getReturn(), PHP_EOL;// 换行,为了提高源码可读性 以上例程会输出: 1 2 3 </span>
is as follows:
<span style="font-size:18px;"><?php function gen() { yield 1; yield 2; yield from gen2(); } function gen2() { yield 3; yield 4; } foreach (gen() as $val) { echo $val, PHP_EOL; } ?> 以上例程会输出: 1 2 3 4 </span>
The integer division function intp()
is added as follows :
<span style="font-size:18px;"><?php var_dump(intp(10, 3)); ?> 以上例程会输出: int(3) </span>
Session options
You can now accept an array parameter through session_start(), which can be written from session in php.ini The configuration instructions parameters support session.lazy_write, read_and_close, cache_limiter, etc.
are as follows:
<span style="font-size:18px;"><?php session_start([ 'cache_limiter' => 'private', 'read_and_close' => true, ]); ?> </span>
New preg_replace_callback_array() function
Can perform better than preg_replace_callback()
##Add two new functions, random_bytes() and random_int()
Please see the following for specific usage methods:
random_bytes()
random_int()
list() no longer supports automatic unpacking
Before, list did not provide guarantees for operating objects that implemented ArrayAccess. Now this problem has been solved
The above is the detailed content of Detailed explanation of new features of php7. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
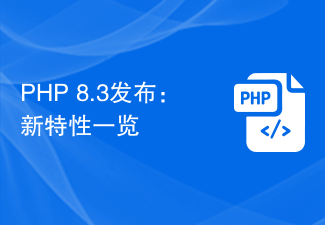
PHP8.3 released: Overview of new features As technology continues to develop and needs change, programming languages are constantly updated and improved. As a scripting language widely used in web development, PHP has been constantly improving to provide developers with more powerful and efficient tools. The recently released PHP 8.3 version brings many long-awaited new features and improvements. Let’s take a look at an overview of these new features. Initialization of non-null properties In past versions of PHP, if a class property was not explicitly assigned a value, its value
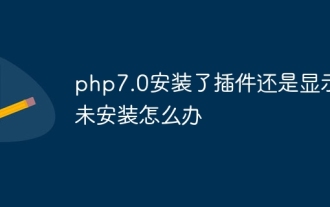
To resolve the plugin not showing installed issue in PHP 7.0: Check the plugin configuration and enable the plugin. Restart PHP to apply configuration changes. Check the plugin file permissions to make sure they are correct. Install missing dependencies to ensure the plugin functions properly. If all other steps fail, rebuild PHP. Other possible causes include incompatible plugin versions, loading the wrong version, or PHP configuration issues.
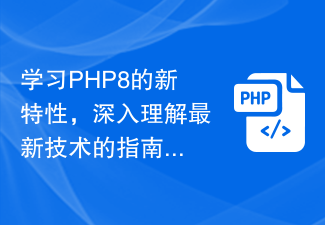
An in-depth analysis of the new features of PHP8 to help you master the latest technology. As time goes by, the PHP programming language has been constantly evolving and improving. The recently released PHP8 version provides developers with many exciting new features and improvements, bringing more convenience and efficiency to our development work. In this article, we will analyze the new features of PHP8 in depth and provide specific code examples to help you better master these latest technologies. JIT compiler PHP8 introduces JIT (Just-In-Time) compilation
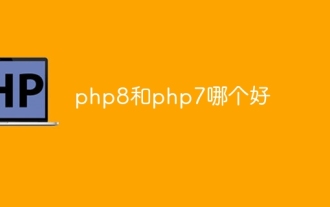
Compared with PHP7, PHP8 has some advantages and improvements in terms of performance, new features and syntax improvements, type system, error handling and extensions. However, choosing which version to use depends on your specific needs and project circumstances. Detailed introduction: 1. Performance improvement, PHP8 introduces the Just-in-Time (JIT) compiler, which can improve the execution speed of the code; 2. New features and syntax improvements, PHP8 supports the declaration of named parameters and optional parameters, making functions Calling is more flexible; anonymous classes, type declarations of properties, etc. are introduced.
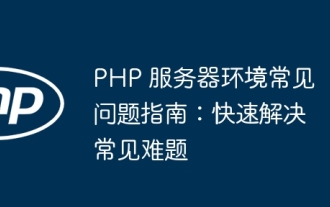
Common solutions for PHP server environments include ensuring that the correct PHP version is installed and that relevant files have been copied to the module directory. Disable SELinux temporarily or permanently. Check and configure PHP.ini to ensure that necessary extensions have been added and set up correctly. Start or restart the PHP-FPM service. Check the DNS settings for resolution issues.
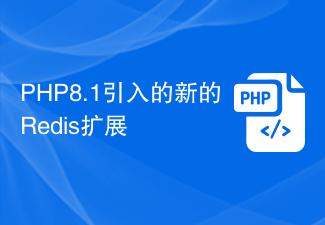
The new Redis extension introduced in PHP8.1 With the rapid development of the Internet, a large amount of data needs to be stored and processed. In order to improve the efficiency and performance of data processing, caching has become an indispensable part. In PHP development, Redis, as a high-performance key-value storage system, is widely used in caching and data storage scenarios. In order to further improve the experience of using Redis in PHP, PHP8.1 introduces a new Redis extension. This article will introduce the new functions of this extension and provide
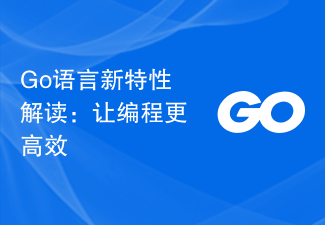
[Interpretation of new features of Go language: To make programming more efficient, specific code examples are needed] In recent years, Go language has attracted much attention in the field of software development, and its simple and efficient design concept has attracted more and more developers. As a statically typed programming language, Go language continues to introduce new features to improve development efficiency and simplify the code writing process. This article will provide an in-depth explanation of the latest features of the Go language and discuss how to experience the convenience brought by these new features through specific code examples. Modular development (GoModules) Go language from 1
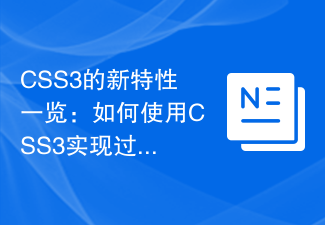
Overview of the new features of CSS3: How to use CSS3 to achieve transition effects CSS3 is the latest version of CSS. Among the many new features, the most interesting and practical one should be the transition effect. Transition effects can make our pages smoother and more beautiful during interaction, giving users a good visual experience. This article will introduce the basic usage of CSS3 transition effects, with corresponding code examples. transition-property attribute: Specify the CSS property transition effect that needs to be transitioned
