How to implement batch upload and download of files with Ajax
This time I will show you how to implement batch upload and download of files with Ajax. What are the precautions for implementing batch upload and download of files with Ajax? The following is a practical case, let's take a look.
I uploaded and downloaded files today. To summarize briefly, the basic web project establishment and SpringMVC framework construction will not be written in detail here.Upload form:
<form id="uploadfiles" enctype="multipart/form-data"> <input type="file" multiple="multiple" id="file_upload" name="file_upload" /> <input type="button" value="上传" onclick="upload()" /> </form>
Upload Ajax:
<script type="text/javascript"> /* * 上传文件 */ function upload(){ var formData = new FormData($( "#uploadfiles" )[0]); $.ajax({ type: "post", url: "./path/upload", dataType: "json", data: formData, /** *必须false才会自动加上正确的Content-Type */ contentType : false, /** * 必须false才会避开jQuery对 formdata 的默认处理 * XMLHttpRequest会对 formdata 进行正确的处理 */ processData : false, success: function(data){//从后端返回数据进行处理 if(data){ alert("上传成功!"); }else{ alert("上传失败!"); } }, error: function(err) {//提交出错 $("#msg").html(JSON.stringify(err));//打出响应信息 alert("服务器无响应"); } }); } </script>
spring.xml configuration plus:
<!-- 配置文件上传 --> <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <!-- 默认编码 --> <property name="defaultEncoding" value="utf-8" /> <!-- 文件大小最大值 --> <property name="maxUploadSize" value="10485760000" /> <!-- 内存中的最大值 --> <property name="maxInMemorySize" value="40960" /> </bean> controller: /* * 上传多个文件 */ @RequestMapping(value = "/upload", produces = "application/json;charset=UTF-8") public @ResponseBody boolean uploadFiles(@RequestParam("file_upload") MultipartFile [] files) { boolean result = false; String realPath; for(int i=0;i<files.length;i++){ if (!files[i].isEmpty()) { String uniqueName=files[i].getOriginalFilename();//得到文件名 realPath="E:"+File.separator+uniqueName;//文件上传的路径这里为E盘 files[i].transferTo(new File(realPath)); // 转存文件 result = true; } catch (Exception e) { e.printStackTrace(); } } } return result; }
/* * 下载多个文件 */ @RequestMapping(value = "/download") public void downloadFiles(HttpServletResponse response) { String str= request.getParameter("rows");//下载文件信息,包括文件名、存储路径等 JSONArray path=(JSONArray) JSONArray.parse(request.getParameter("rows")); Path paths[]=new Path[path.size()]; paths = JSONArray.parseArray(str, Path.class).toArray(paths); String uri = "d:"+ File.separator + "mldn.zip";//临时文件存储路径 File zipFile = new File(uri) ; // 定义压缩文件名称 ZipOutputStream zipOut = null;// 声明压缩流对象 InputStream input = null; //将要压缩的文件加入到压缩输出流中 try { zipOut = new ZipOutputStream(new FileOutputStream(zipFile)); } catch (FileNotFoundException e) { e.printStackTrace(); } for(int i = 0;i<paths.length;i++){ File file = new File(paths[i].getUri()+File.separator+paths[i].getFilename()); try { input = new FileInputStream(file) ;// 定义文件的输入流 zipOut.putNextEntry(new ZipEntry(file.getName())) ; // 设置ZipEntry对象 } catch (Exception e) { e.printStackTrace(); } } //将文件写入到压缩文件中 int temp = 0 ; try { while((temp=input.read())!=-1){ // 读取内容 zipOut.write(temp) ; // 写到压缩文件中 } } catch (IOException e) { e.printStackTrace(); }finally{ try { input.close() ; zipOut.close() ; } catch (IOException e) { e.printStackTrace(); } } try { // 以流的形式下载文件。 BufferedInputStream fis = new BufferedInputStream(new FileInputStream(zipFile)); byte[] buffer = new byte[fis.available()]; fis.read(buffer); fis.close(); // 清空response response.reset(); OutputStream toClient = new BufferedOutputStream(response.getOutputStream()); response.setContentType("application/x-msdownload;"); response.setHeader("Content-Disposition", "attachment;filename=" + zipFile.getName()); toClient.write(buffer); toClient.flush(); toClient.close(); zipFile.delete(); //将生成的服务器端文件删除 } catch (IOException ex) { ex.printStackTrace(); } }
file download. But you can use js to generate a form, use this form to submit parameters, and return "stream" type data.
Example:function download(){ var form=$("<form>");//定义一个form表单 form.attr("style","display:none"); form.attr("target",""); form.attr("method","post"); form.attr("action","./path/download");//请求url var input1=$("<input>"); input1.attr("type","hidden"); input1.attr("name","rows");//设置属性的名字 input1.attr("value",“test”);//设置属性的值 $("body").append(form);//将表单放置在web中 form.append(input1); form.submit();//表单提交 }
I believe you have mastered the method after reading the case in this article. For more exciting information, please pay attention to other related articles on the php Chinese website! Recommended reading:
Ajax+Servlet to implement non-refresh drop-down linkage (with code)
How to use Ajax asynchronously Check if the username is duplicate
The above is the detailed content of How to implement batch upload and download of files with Ajax. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


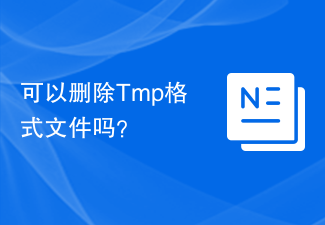
Tmp format files are a temporary file format usually generated by a computer system or program during execution. The purpose of these files is to store temporary data to help the program run properly or improve performance. Once the program execution is completed or the computer is restarted, these tmp files are often no longer necessary. Therefore, for Tmp format files, they are essentially deletable. Moreover, deleting these tmp files can free up hard disk space and ensure the normal operation of the computer. However, before deleting Tmp format files, we need to
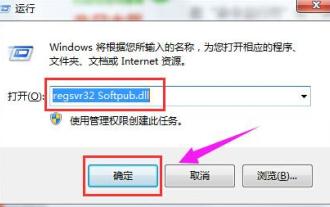
When deleting or decompressing a folder on your computer, sometimes a prompt dialog box "Error 0x80004005: Unspecified Error" will pop up. How should you solve this situation? There are actually many reasons why the error code 0x80004005 is prompted, but most of them are caused by viruses. We can re-register the dll to solve the problem. Below, the editor will explain to you the experience of handling the 0x80004005 error code. Some users are prompted with error code 0X80004005 when using their computers. The 0x80004005 error is mainly caused by the computer not correctly registering certain dynamic link library files, or by a firewall that does not allow HTTPS connections between the computer and the Internet. So how about
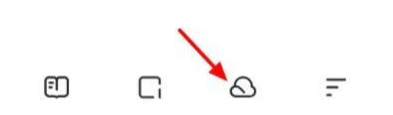
Quark Netdisk and Baidu Netdisk are currently the most commonly used Netdisk software for storing files. If you want to save the files in Quark Netdisk to Baidu Netdisk, how do you do it? In this issue, the editor has compiled the tutorial steps for transferring files from Quark Network Disk computer to Baidu Network Disk. Let’s take a look at how to operate it. How to save Quark network disk files to Baidu network disk? To transfer files from Quark Network Disk to Baidu Network Disk, you first need to download the required files from Quark Network Disk, then select the target folder in the Baidu Network Disk client and open it. Then, drag and drop the files downloaded from Quark Cloud Disk into the folder opened by the Baidu Cloud Disk client, or use the upload function to add the files to Baidu Cloud Disk. Make sure to check whether the file was successfully transferred in Baidu Cloud Disk after the upload is completed. That's it
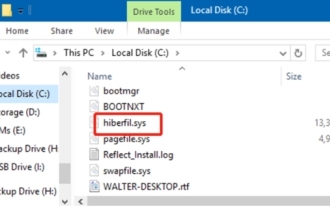
Recently, many netizens have asked the editor, what is the file hiberfil.sys? Can hiberfil.sys take up a lot of C drive space and be deleted? The editor can tell you that the hiberfil.sys file can be deleted. Let’s take a look at the details below. hiberfil.sys is a hidden file in the Windows system and also a system hibernation file. It is usually stored in the root directory of the C drive, and its size is equivalent to the size of the system's installed memory. This file is used when the computer is hibernated and contains the memory data of the current system so that it can be quickly restored to the previous state during recovery. Since its size is equal to the memory capacity, it may take up a larger amount of hard drive space. hiber
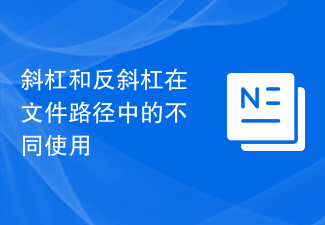
A file path is a string used by the operating system to identify and locate a file or folder. In file paths, there are two common symbols separating paths, namely forward slash (/) and backslash (). These two symbols have different uses and meanings in different operating systems. The forward slash (/) is a commonly used path separator in Unix and Linux systems. On these systems, file paths start from the root directory (/) and are separated by forward slashes between each directory. For example, the path /home/user/Docume
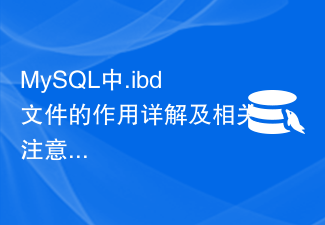
Detailed explanation of the role of .ibd files in MySQL and related precautions MySQL is a popular relational database management system, and the data in the database is stored in different files. Among them, the .ibd file is a data file in the InnoDB storage engine, used to store data and indexes in tables. This article will provide a detailed analysis of the role of the .ibd file in MySQL and provide relevant code examples to help readers better understand. 1. The role of .ibd files: storing data: .ibd files are InnoDB storage
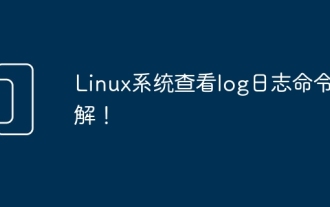
In Linux systems, you can use the following command to view the contents of the log file: tail command: The tail command is used to display the content at the end of the log file. It is a common command to view the latest log information. tail [option] [file name] Commonly used options include: -n: Specify the number of lines to be displayed, the default is 10 lines. -f: Monitor the file content in real time and automatically display the new content when the file is updated. Example: tail-n20logfile.txt#Display the last 20 lines of the logfile.txt file tail-flogfile.txt#Monitor the updated content of the logfile.txt file in real time head command: The head command is used to display the beginning of the log file

Working with files in the Linux operating system requires the use of various commands and techniques that enable developers to efficiently create and execute files, code, programs, scripts, and other things. In the Linux environment, files with the extension ".a" have great importance as static libraries. These libraries play an important role in software development, allowing developers to efficiently manage and share common functionality across multiple programs. For effective software development in a Linux environment, it is crucial to understand how to create and run ".a" files. This article will introduce how to comprehensively install and configure the Linux ".a" file. Let's explore the definition, purpose, structure, and methods of creating and executing the Linux ".a" file. What is L
