How does ajax's Fileupload implement multiple file uploads?
This time I will show you how to implement multiple file uploads with ajax's Fileupload, and how to implement multiple file uploads with ajax's Fileupload. What are the precautions? . The following is a practical case. Let's take a look. one time.
Open Google and search for "ajaxFileupload' 'Multiple file upload'. You can find many similar ones, so why should I write them down?
One is to express gratitude for the contributions of the previous masters; the second is a summary of my own knowledge; the third is that I have made changes based on the original ones. I record them here and may help other friends.
Anyone who has used this plug-in knows the basic usage of this plug-in. I will not talk nonsense and go directly to the code.
I need to implement multiple file uploads. The previous method was to define multiple inputs with different IDs, and then put the ajaxfileuplod method in for loop. This method was seen online. , I don’t think it’s very good, but I found it later on the Internet, which is more advanced, and I directly changed the source code (because the author hasn’t updated it for a long time, and it really can’t meet the requirements). Next, let’s see how I changed it.
Quoting the online practice:
1. Look at the code before modification
var oldElement = jQuery('#' + fileElementId); var newElement = jQuery(oldElement).clone(); jQuery(oldElement).attr('id', fileId); jQuery(oldElement).before(newElement); jQuery(oldElement).appendTo(form);
It is easy to see that this is to add the input with the id to from. Then to implement multiple file uploads, change it to the following:
if(typeof(fileElementId) == 'string'){ fileElementId = [fileElementId]; } for(var i in fileElementId){ var oldElement = jQuery('#' + fileElementId[i]); var newElement = jQuery(oldElement).clone(); jQuery(oldElement).attr('id', fileId); jQuery(oldElement).before(newElement); jQuery(oldElement).appendTo(form); }
After this change, the initialization code will be written like this:
$.ajaxFileUpload({ url:'/ajax.php', fileElementId:['id1','id2']//原先是fileElementId:'id' 只能上传一个 });
At this point, you can indeed upload multiple files. , but for me, a new problem comes again. With multiple IDs, the files of my interface are not fixed, but are dynamically loaded. So the IDs need to be dynamically generated. I think it is too troublesome. Why not just use the name? Then change the above code to the following:
if(typeof(fileElementId) == 'string'){ fileElementId = [fileElementId]; } for(var i in fileElementId){ //按name取值 var oldElement = jQuery("input[name="+fileElementId[i]+"]"); oldElement.each(function() { var newElement = jQuery($(this)).clone(); jQuery(oldElement).attr('id', fileId); jQuery(oldElement).before(newElement); jQuery(oldElement).appendTo(form); }); }
If you change it this way, you can upload multiple groups of files. Let's see how I apply it.
html:
<p> <img id="loading" src="scripts/ajaxFileUploader/loading.gif" style="display:none;"> <table cellpadding="0" cellspacing="0" class="tableForm" id="calculation_model"> <thead> <tr> <th>多组多个文件</th> </tr> </thead> <tbody> <tr> <td>第一组</td> <td>第二组</td> </tr> <tr> <td><input type="file" name="gridDoc" class="input"></td> <td><input type="file" name="caseDoc" class="input"></td> </tr> </tbody> <tfoot> <tr> <td><button class="button" id="up1">Upload</button></td> <td><button class="button" id="addInput" >添加一组</button></td> </tr> </tfoot> </table> </p>
js:
/** * Created with IntelliJ IDEA. * User: Administrator * Date: 13-7-3 * Time: 上午9:20 * To change this template use File | Settings | File Templates. */ $(document).ready(function () { $("#up1").click(function(){ var temp = ["gridDoc","caseDoc"]; ajaxFileUpload(temp); }); $("#addInput").click(function(){ addInputFile(); }); }); //动态添加一组文件 function addInputFile(){ $("#calculation_model").append(" <tr>"+ "<td><input type='file' name='gridDoc' class='input'></td> "+ "<td><input type='file' name='caseDoc' class='input'></td> "+ "</tr>"); } //直接使用下载下来的文件里的demo代码 function ajaxFileUpload(id) { //starting setting some animation when the ajax starts and completes $("#loading").ajaxStart(function(){ $(this).show(); }).ajaxComplete(function(){ $(this).hide(); }); /* prepareing ajax file upload url: the url of script file handling the uploaded files fileElementId: the file type of input element id and it will be the index of $_FILES Array() dataType: it support json, xml secureuri:use secure protocol success: call back function when the ajax complete error: callback function when the ajax failed */ $.ajaxFileUpload({ url:'upload.action', //secureuri:false, fileElementId:id, dataType: 'json' } ) return false; }
I use struts2 in the background. The upload of strtus2 is relatively simple. As long as you declare the agreed name, you can get the file object. , and the name, the code is as follows:
package com.ssy.action; import com.opensymphony.xwork2.ActionSupport; import org.apache.commons.io.FileUtils; import org.apache.struts2.util.ServletContextAware; import javax.servlet.ServletContext; import java.io.*; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Random; /** * Created with IntelliJ IDEA. * User: Administrator * Date: 13-7-2 * Time: 下午4:08 * To change this template use File | Settings | File Templates. */ public class Fileupload extends ActionSupport implements ServletContextAware { private File[] gridDoc,caseDoc; private String[] gridDocFileName,caseDocFileName; private ServletContext context; public String execute(){ for (int i = 0;i<gridDocFileName.length;i++) { System.out.println(gridDocFileName[i]); } for (int i = 0;i<caseDocFileName.length;i++) { System.out.println(caseDocFileName[i]); } //System.out.println(doc1FileName); //System.out.println(doc2FileName); String targetDirectory = context.getRealPath("/uploadFile"); /* *这里我只取得 第一组的文件进行上传,第二组的类似 */ try{ for (int i = 0; i < gridDoc.length; i++) { String targetFileName = generateFileName(gridDocFileName[i]); File target = new File(targetDirectory, targetFileName); FileUtils.copyFile(gridDoc[i], target); } }catch (Exception e){ e.printStackTrace(); } return SUCCESS; } public File[] getGridDoc() { return gridDoc; } public void setGridDoc(File[] gridDoc) { this.gridDoc = gridDoc; } public File[] getCaseDoc() { return caseDoc; } public void setCaseDoc(File[] caseDoc) { this.caseDoc = caseDoc; } public String[] getGridDocFileName() { return gridDocFileName; } public void setGridDocFileName(String[] gridDocFileName) { this.gridDocFileName = gridDocFileName; } public String[] getCaseDocFileName() { return caseDocFileName; } public void setCaseDocFileName(String[] caseDocFileName) { this.caseDocFileName = caseDocFileName; } /** * 用日期和随机数格式化文件名避免冲突 * @param fileName * @return */ private String generateFileName(String fileName) { System.out.println(fileName); SimpleDateFormat sf = new SimpleDateFormat("yyMMddHHmmss"); String formatDate = sf.format(new Date()); int random = new Random().nextInt(10000); int position = fileName.lastIndexOf("."); String extension = fileName.substring(position); return formatDate + random + extension; } }
After writing this, I have questions. Why did the master change multiple files before and still get the ID, and how to get it in the background? I still don’t understand it. , is the code I changed feasible? Is there a bug? This has yet to be tested. If you see any problems, please point them out and learn together
Finally, attached is my modified plug-in
ajaxfileupload plug-in
I believe you will read the case in this article You have mastered the method. For more exciting information, please pay attention to other related articles on the php Chinese website!
Recommended reading:
Ajax+Servlet to implement non-refresh drop-down linkage (with code)
How to use Ajax asynchronously Check if the username is duplicate
The above is the detailed content of How does ajax's Fileupload implement multiple file uploads?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
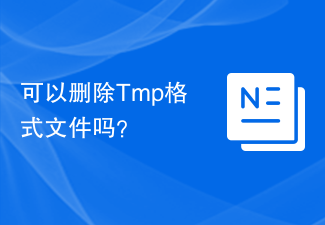
Tmp format files are a temporary file format usually generated by a computer system or program during execution. The purpose of these files is to store temporary data to help the program run properly or improve performance. Once the program execution is completed or the computer is restarted, these tmp files are often no longer necessary. Therefore, for Tmp format files, they are essentially deletable. Moreover, deleting these tmp files can free up hard disk space and ensure the normal operation of the computer. However, before deleting Tmp format files, we need to
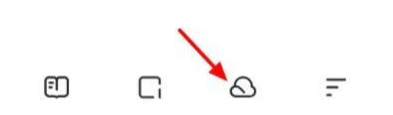
Quark Netdisk and Baidu Netdisk are currently the most commonly used Netdisk software for storing files. If you want to save the files in Quark Netdisk to Baidu Netdisk, how do you do it? In this issue, the editor has compiled the tutorial steps for transferring files from Quark Network Disk computer to Baidu Network Disk. Let’s take a look at how to operate it. How to save Quark network disk files to Baidu network disk? To transfer files from Quark Network Disk to Baidu Network Disk, you first need to download the required files from Quark Network Disk, then select the target folder in the Baidu Network Disk client and open it. Then, drag and drop the files downloaded from Quark Cloud Disk into the folder opened by the Baidu Cloud Disk client, or use the upload function to add the files to Baidu Cloud Disk. Make sure to check whether the file was successfully transferred in Baidu Cloud Disk after the upload is completed. That's it
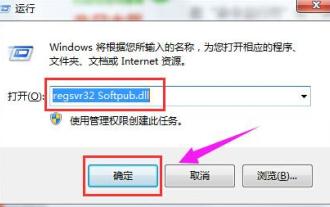
When deleting or decompressing a folder on your computer, sometimes a prompt dialog box "Error 0x80004005: Unspecified Error" will pop up. How should you solve this situation? There are actually many reasons why the error code 0x80004005 is prompted, but most of them are caused by viruses. We can re-register the dll to solve the problem. Below, the editor will explain to you the experience of handling the 0x80004005 error code. Some users are prompted with error code 0X80004005 when using their computers. The 0x80004005 error is mainly caused by the computer not correctly registering certain dynamic link library files, or by a firewall that does not allow HTTPS connections between the computer and the Internet. So how about
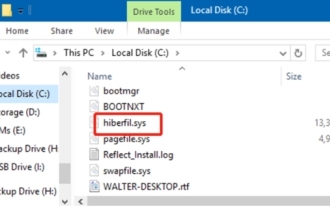
Recently, many netizens have asked the editor, what is the file hiberfil.sys? Can hiberfil.sys take up a lot of C drive space and be deleted? The editor can tell you that the hiberfil.sys file can be deleted. Let’s take a look at the details below. hiberfil.sys is a hidden file in the Windows system and also a system hibernation file. It is usually stored in the root directory of the C drive, and its size is equivalent to the size of the system's installed memory. This file is used when the computer is hibernated and contains the memory data of the current system so that it can be quickly restored to the previous state during recovery. Since its size is equal to the memory capacity, it may take up a larger amount of hard drive space. hiber
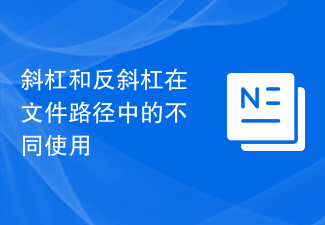
A file path is a string used by the operating system to identify and locate a file or folder. In file paths, there are two common symbols separating paths, namely forward slash (/) and backslash (). These two symbols have different uses and meanings in different operating systems. The forward slash (/) is a commonly used path separator in Unix and Linux systems. On these systems, file paths start from the root directory (/) and are separated by forward slashes between each directory. For example, the path /home/user/Docume
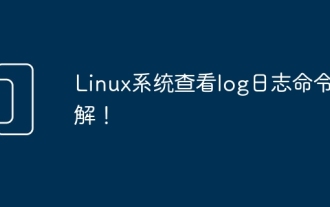
In Linux systems, you can use the following command to view the contents of the log file: tail command: The tail command is used to display the content at the end of the log file. It is a common command to view the latest log information. tail [option] [file name] Commonly used options include: -n: Specify the number of lines to be displayed, the default is 10 lines. -f: Monitor the file content in real time and automatically display the new content when the file is updated. Example: tail-n20logfile.txt#Display the last 20 lines of the logfile.txt file tail-flogfile.txt#Monitor the updated content of the logfile.txt file in real time head command: The head command is used to display the beginning of the log file
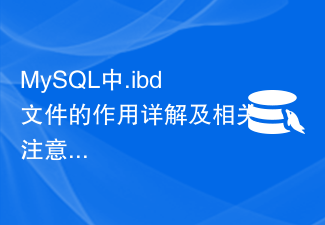
Detailed explanation of the role of .ibd files in MySQL and related precautions MySQL is a popular relational database management system, and the data in the database is stored in different files. Among them, the .ibd file is a data file in the InnoDB storage engine, used to store data and indexes in tables. This article will provide a detailed analysis of the role of the .ibd file in MySQL and provide relevant code examples to help readers better understand. 1. The role of .ibd files: storing data: .ibd files are InnoDB storage
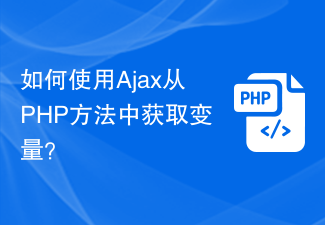
Using Ajax to obtain variables from PHP methods is a common scenario in web development. Through Ajax, the page can be dynamically obtained without refreshing the data. In this article, we will introduce how to use Ajax to get variables from PHP methods, and provide specific code examples. First, we need to write a PHP file to handle the Ajax request and return the required variables. Here is sample code for a simple PHP file getData.php:
