React uses scripting to implement animation
This article will share with you how to use scripts to implement animation. To use scripts to implement animation, we can use the react-motion animation library. It is an excellent animation library and uses scripts to implement animations. (motion means movement), which has certain reference value. Friends in need can refer to
react-motion: https://github.com/chenglou/react-motion
1. Install react-motion animation library
yarn add react-motion//ro npm install react-motion
2. Counter case
This case implements adding the number 0 to 1
1. Import spring and Motion from the react-motion library
spring: Specify how to animate the target value, for example, spring(10, {stiffness: 120, damping: 17})
means "Draw the dynamic value to 10, the spring stiffness is 120, and the damping is 17"
Motion: It is A component that specifically provides animation data. It receives a function as a subcomponent, for example:
< motion > { value => ( ) } </ Motion >
2. Motion component attribute:
defaultStyle : Set the default value before the animation starts
style : Set the animation to the value
import React, {Component} from 'react'; import {spring ,Motion} from 'react-motion'; export default class Main extends Component { render() { return ( <p style={styles.content}> {/*由0 过渡到 1 ; stiffness是阻尼*/} <Motion defaultStyle={{x: 0}} style={{x: spring(1,{stiffness: 20})}}> { value => <p> {value.x} </p> } </Motion> </p> ) } }/*样式*/const styles = { content: { width: '400px', height: '500px', backgroundColor: 'skyblue', margin: '0 auto', }, }
The effect of execution:
2. Animation case for changing transparency and width
Through the above case, we can know that the Motion group is dedicated to providing animation data
. In fact, it does not participate in the drawing of the interface, The drawing process of the interface is completed through subcomponents
. Let's do an animation case of changing transparency and width
1. Import spring and Motion
2 from the
library. The value of value.x
transitions from 0 to 1
. You can modify the transparency and width of p through the animation data class provided by Motion
3.${value.x}
Added backticks
on both sides. This is the string template syntax in es6. ${} can be understood as the effect of interpolator
import React, {Component} from 'react'; import {spring ,Motion} from 'react-motion'; export default class Main extends Component { render() { return ( <p style={styles.content}> {/*由0 过渡到 1 ; stiffness是阻尼*/} ...... {/*改变p的宽度和透明度*/} <Motion defaultStyle={{x: 0}} style={{x: spring(1,{stiffness: 20})}}> { value => <p style={ Object.assign({},styles.pStyle,{ opacity:`${value.x}`, width:`${50+value.x*100}px` })}> 默认 </p> } </Motion> </p> ) } }/*样式*/const styles = { content: { .... }, pStyle: { width: '50px', height: '50px', backgroundColor: 'green', margin: '3px 0', color:'white' }, }
refreshing the interface to 0.2:
The result after execution:
3.TransitionMotion execution loading and unloading animation case
The previously learned TransitionGroup
animation library provides loading and unloading animations of execution components. In fact, react-motion
also provides this function. To use this function, you need to use a new API: TransitionMotion
component, which can help you perform component loading and unloading animations. .
1. Import TransitionMotion, spring and presets from the
react-motion library
spring : Specify how to animate the target value presets : Preset the values of stiffness and damping 2. When adding a component: The state is transitioned by the state defined in willEnter() To the state defined in styles
willEnter(){ return {width: 0, height: 0};} // 由willEnter()中width: 0, height: 0状态过渡到下面styles中width:200, height: 50状态 // spring() 函数为目标值设置动画 // presets.wobbly 等价于 {stiffness: 180, damping: 12} styles={this.state.items.map(item => ({ key: item.key, style: {width: spring(item.w,presets.wobbly), height: spring(50,presets.wobbly)}, }))}
styles={this.state.items.map(item => ({ key: item.key, style: {width: spring(item.w,presets.wobbly), height: spring(50,presets.wobbly)}, }))} // 由styles中width:200, height: 50状态过渡到下面willEnter()中width: 0, height: 0 状态 // presets.wobbly 等价于 {stiffness: 180, damping: 12} //下面的 spring(0,presets.wobbly) 设置目标值动画 willLeave() { return {width: spring(0,presets.wobbly), height: spring(0,presets.wobbly)};}
Complete code of the case:
import React, {Component} from 'react'; import {TransitionMotion,spring , presets} from 'react-motion'; export default class Main extends Component { constructor(props) { super(props); /*定义一个数组:目标状态*/ this.state={ items: [{key: 'a', w: 200},{key: 'b', w: 200}], } } /*装载组件的状态( 进入/添加组件 )*/ willEnter(){ return {width: 0, height: 0}; } /*御载组件的状态( 离开/删除组件 )*/ willLeave() { return {width: spring(0,presets.wobbly), height: spring(0,presets.wobbly)}; } render() { return ( <p style={styles.content}> <TransitionMotion willEnter={this.willEnter} willLeave={this.willLeave} styles={this.state.items.map(item => ({ key: item.key, style: {width: spring(item.w,presets.wobbly), height: spring(50,presets.wobbly)}, }))}> {interpolatedStyles => <p> {interpolatedStyles.map(config => { return <p key={config.key} style={{...config.style, border: '1px solid'}} > {config.key} : {config.style.height} </p> })} </p> } </TransitionMotion> <button onClick={()=>this.startAnimation(0)}>Add C</button> <button onClick={()=>this.startAnimation(1)}>remove C</button> </p> ) } startAnimation(index){ // add if(index==0){ this.setState({ items: [{key: 'a', w: 200},{key: 'b', w: 200},{key: 'c', w: 200}], }) // remove }else{ this.setState({ items: [{key: 'a', w: 200},{key: 'b', w: 200}], }) } } }/*样式*/const styles = { content: { width: '400px', height: '500px', backgroundColor: 'skyblue', margin: '0 auto', }, }
Use React to implement page transition animation
ReactJS learning series courses (React animation use)
The above is the detailed content of React uses scripting to implement animation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


![Animation not working in PowerPoint [Fixed]](https://img.php.cn/upload/article/000/887/227/170831232982910.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
Are you trying to create a presentation but can't add animation? If animations are not working in PowerPoint on your Windows PC, then this article will help you. This is a common problem that many people complain about. For example, animations may stop working during presentations in Microsoft Teams or during screen recordings. In this guide, we will explore various troubleshooting techniques to help you fix animations not working in PowerPoint on Windows. Why aren't my PowerPoint animations working? We have noticed that some possible reasons that may cause the animation in PowerPoint not working issue on Windows are as follows: Due to personal
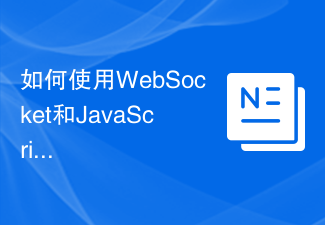
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
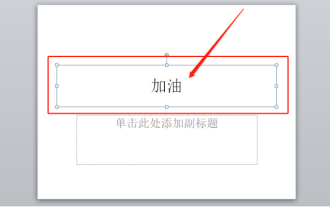
We often use ppt in our daily work, so are you familiar with every operating function in ppt? For example: How to set animation effects in ppt, how to set switching effects, and what is the effect duration of each animation? Can each slide play automatically, enter and then exit the ppt animation, etc. In this issue, I will first share with you the specific steps of entering and then exiting the ppt animation. It is below. Friends, come and take a look. Look! 1. First, we open ppt on the computer, click outside the text box to select the text box (as shown in the red circle in the figure below). 2. Then, click [Animation] in the menu bar and select the [Erase] effect (as shown in the red circle in the figure). 3. Next, click [
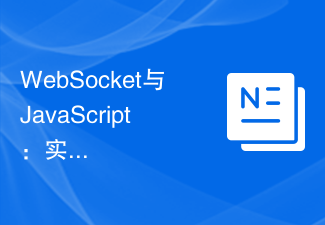
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
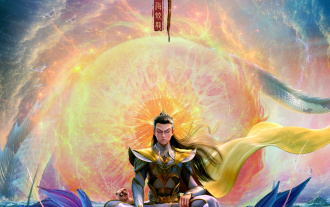
This website reported on January 26 that the domestic 3D animated film "Er Lang Shen: The Deep Sea Dragon" released a set of latest stills and officially announced that it will be released on July 13. It is understood that "Er Lang Shen: The Deep Sea Dragon" is produced by Mihuxing (Beijing) Animation Co., Ltd., Horgos Zhonghe Qiancheng Film Co., Ltd., Zhejiang Hengdian Film Co., Ltd., Zhejiang Gongying Film Co., Ltd., Chengdu The animated film produced by Tianhuo Technology Co., Ltd. and Huawen Image (Beijing) Film Co., Ltd. and directed by Wang Jun was originally scheduled to be released in mainland China on July 22, 2022. Synopsis of the plot of this site: After the Battle of the Conferred Gods, Jiang Ziya took the "Conferred Gods List" to divide the gods, and then the Conferred Gods List was sealed by the Heavenly Court under the deep sea of Kyushu Secret Realm. In fact, in addition to conferring divine positions, there are also many powerful evil spirits sealed in the Conferred Gods List.
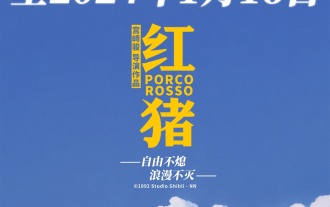
According to news from this site, Miyazaki Hayao's animated film "Porco Rosso" has announced that it will extend the release date to January 16, 2024. This site previously reported that "Porco Rosso" has been launched in the National Art Federation Special Line Cinema on November 17, with a cumulative box office of over 2,000 10,000, with a Douban score of 8.6, and 85.8% of 4 and 5 star reviews. "Porco Rosso" was produced by Studio Ghibli and directed by Hayao Miyazaki. Moriyama Moriyama, Tokiko Kato, Otsuka Akio, Okamura Akemi and others participated in the dubbing. It was originally released in Japan in 1992. The film is adapted from Hayao Miyazaki's comic book "The Age of Airships" and tells the story of the Italian Air Force's ace pilot Pollock Rosen who was magically turned into a pig. After that, he became a bounty hunter, fighting against air robbers and protecting those around him. Plot synopsis: Rosen is a soldier in World War I
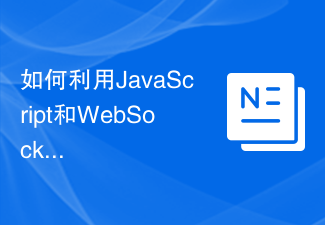
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
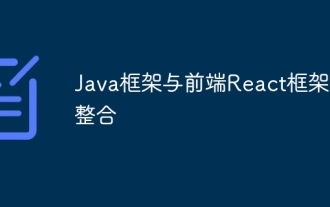
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
