typeof and type judgment in JS (with code)
This time I will bring you typeof and type judgment in JS (with code). What are the precautions for typeof and type judgment in JS? The following is a practical case. Let’s take a look. .
typeof
ECMAScript has 5 primitive types, namely Undefined, Null, Boolean, Number and String. We all know that you can use the typeofoperator to find the type of a variable, but when is used to reference a type variable, it will only return object, which means that typeof can only Correctly identify basic type value variables.
var a = "abc"; console.log(typeof a); // "string" var b = 123; console.log(typeof b); // "number" var c = true; console.log(typeof c); // "boolean" var d = null; console.log(typeof d); // "object" var f = undefined; console.log(typeof f); // "undefined" var g; console.log(typeof g); // "undefined" console.log(typeof x); // "undefined"
var a = function() { }; console.log(typeof a); // "function" var b = [1,2,3]; console.log(typeof b); // "object" var c = { }; console.log(typeof c); // "object"
Type judgment
Type judgment generally means judging whether it is an array or an empty object. This is the judgment method I have used or seen every day for this requirement Determine whether it is an array There is an array: var a = [1,2,3,4,5];method one:
toString.call(a); // "[object Array]" method two: a instanceof Array; //true method three: a.constructor == Array; //true The first method is more general, which is the abbreviation of Object.prototype.toString.call(a). The variables judged by instanceof and constructor must be declared on the current page. For example, a page (parent page) has a frame, and the frame refers to a page (child page). An a is declared in the child page and assigned a value. Give a variable to the parent page, then judge the variable, Array == object.constructor will return false;var a = [1,2,3,4,5]; console.log(toString.call(a)); // "[object Array]" console.log(a instanceof Array); //true console.log(a.constructor == Array); //true
method one:
JSON.stringify(obj); // "{}" is converted into a JSON object to determine whether it is an empty braceMethod Two:
if(obj.id){ //IfMethod three:
function isEmptyObject(e) { var t; for (t in e) return !1; return !0 } //trueisEmptyObject(obj); //falseisEmptyObject({ "a":1, "b":2});
The above is the detailed content of typeof and type judgment in JS (with code). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


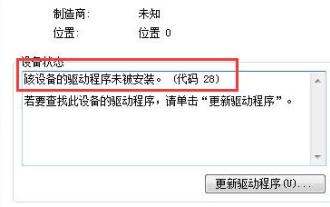
Some users encountered errors when installing the device, prompting error code 28. In fact, this is mainly due to the driver. We only need to solve the problem of win7 driver code 28. Let’s take a look at what should be done. Do it. What to do with win7 driver code 28: First, we need to click on the start menu in the lower left corner of the screen. Then, find and click the "Control Panel" option in the pop-up menu. This option is usually located at or near the bottom of the menu. After clicking, the system will automatically open the control panel interface. In the control panel, we can perform various system settings and management operations. This is the first step in the nostalgia cleaning level, I hope it helps. Then we need to proceed and enter the system and
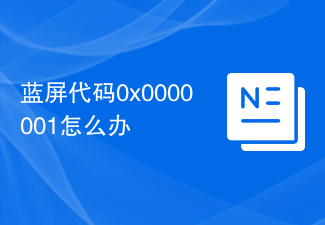
What to do with blue screen code 0x0000001? The blue screen error is a warning mechanism when there is a problem with the computer system or hardware. Code 0x0000001 usually indicates a hardware or driver failure. When users suddenly encounter a blue screen error while using their computer, they may feel panicked and at a loss. Fortunately, most blue screen errors can be troubleshooted and dealt with with a few simple steps. This article will introduce readers to some methods to solve the blue screen error code 0x0000001. First, when encountering a blue screen error, we can try to restart
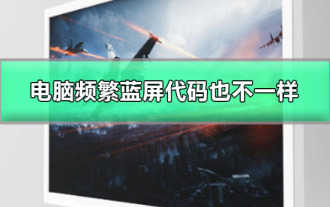
The win10 system is a very excellent high-intelligence system. Its powerful intelligence can bring the best user experience to users. Under normal circumstances, users’ win10 system computers will not have any problems! However, it is inevitable that various faults will occur in excellent computers. Recently, friends have been reporting that their win10 systems have encountered frequent blue screens! Today, the editor will bring you solutions to different codes that cause frequent blue screens in Windows 10 computers. Let’s take a look. Solutions to frequent computer blue screens with different codes each time: causes of various fault codes and solution suggestions 1. Cause of 0×000000116 fault: It should be that the graphics card driver is incompatible. Solution: It is recommended to replace the original manufacturer's driver. 2,
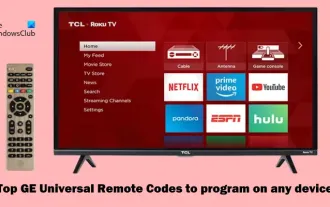
If you need to program any device remotely, this article will help you. We will share the top GE universal remote codes for programming any device. What is a GE remote control? GEUniversalRemote is a remote control that can be used to control multiple devices such as smart TVs, LG, Vizio, Sony, Blu-ray, DVD, DVR, Roku, AppleTV, streaming media players and more. GEUniversal remote controls come in various models with different features and functions. GEUniversalRemote can control up to four devices. Top Universal Remote Codes to Program on Any Device GE remotes come with a set of codes that allow them to work with different devices. you may
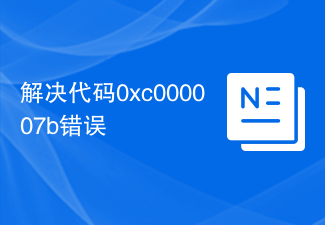
Termination Code 0xc000007b While using your computer, you sometimes encounter various problems and error codes. Among them, the termination code is the most disturbing, especially the termination code 0xc000007b. This code indicates that an application cannot start properly, causing inconvenience to the user. First, let’s understand the meaning of termination code 0xc000007b. This code is a Windows operating system error code that usually occurs when a 32-bit application tries to run on a 64-bit operating system. It means it should
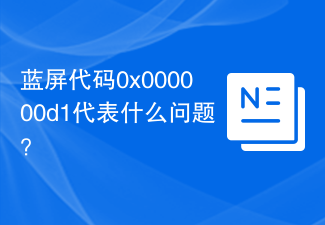
What does the 0x000000d1 blue screen code mean? In recent years, with the popularization of computers and the rapid development of the Internet, the stability and security issues of the operating system have become increasingly prominent. A common problem is blue screen errors, code 0x000000d1 is one of them. A blue screen error, or "Blue Screen of Death," is a condition that occurs when a computer experiences a severe system failure. When the system cannot recover from the error, the Windows operating system displays a blue screen with the error code on the screen. These error codes
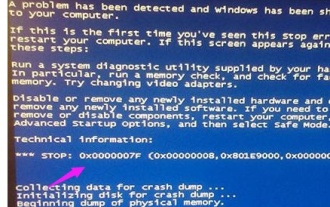
Blue screen is a problem we often encounter when using the system. Depending on the error code, there will be many different reasons and solutions. For example, when we encounter the problem of stop: 0x0000007f, it may be a hardware or software error. Let’s follow the editor to find out the solution. 0x000000c5 blue screen code reason: Answer: The memory, CPU, and graphics card are suddenly overclocked, or the software is running incorrectly. Solution 1: 1. Keep pressing F8 to enter when booting, select safe mode, and press Enter to enter. 2. After entering safe mode, press win+r to open the run window, enter cmd, and press Enter. 3. In the command prompt window, enter "chkdsk /f /r", press Enter, and then press the y key. 4.
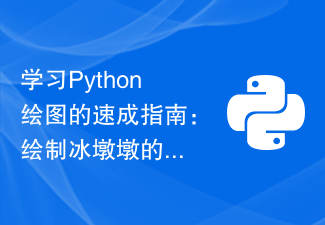
Quickly get started with Python drawing: code example for drawing Bingdundun Python is an easy-to-learn and powerful programming language. By using Python's drawing library, we can easily realize various drawing needs. In this article, we will use Python's drawing library matplotlib to draw a simple graph of ice. Bingdundun is a cute panda who is very popular among children. First, we need to install the matplotlib library. You can do this by running in the terminal
