Use React to render components to specified DOM nodes
This time I will bring you the precautions of using React to render components to the specified DOM node and using React to render the component to the specified DOM node. The following is a practical case. , let’s take a look.
As we all know, one of the advantages of React is that its API is particularly simple. By returning the basic structure of a component through the render method, just like a simple function, you can get a reusable react component. But sometimes there are still some restrictions, especially in its API, which cannot control the DOM node that the component should be rendered to, which makes it difficult to control some elastic layer components. The problem will arise when the parent element is set to <a href="http://www.php.cn/wiki/923.html" target="_blank">overflow</a>:hidden
.
For example, it looks like this:
The effect we actually expect is this:
Fortunately, there is a fairly elegant, although not obvious, way to get around this problem. The first react function we learned is the render method, and its function signature is like this:
ReactComponent render( ReactElement element, DOMElement container, [function callback] )
Normally we use this method to render the entire application into a DOM node. The good news is that the method doesn't stop there. We can use the ReactDom.render
method in one component to render another component into a specified DOM element. As a component's render method, it must be pure (for example: it cannot change state or interact with the DOM). So we need to call the ReactDom.render
method in componentDidUpdate or componentDidMount.
In addition, we need to ensure that when the parent element is unloaded, the modified component will also be unloaded.
After sorting it out, we get the following component:
import React,{Component} from 'react'; import ReactDom from 'react-dom'; export default class RenderInBody extends Component{ constructor(p){ super(); } componentDidMount(){//新建一个p标签并塞进body this.popup = document.createElement("p"); document.body.appendChild(this.popup); this._renderLayer(); } componentDidUpdate() { this._renderLayer(); } componentWillUnmount(){//在组件卸载的时候,保证弹层也被卸载掉 ReactDom.unmountComponentAtNode(this.popup); document.body.removeChild(this.popup); } _renderLayer(){//将弹层渲染到body下的p标签 ReactDom.render(this.props.children, this.popup); } render(){ return null; } }
To sum up:
Manually insert a p tag into the body during componentDidMount, and then use ReactDom.render to render the component to the p tag
When we want to render a component directly to the body, we only need to wrap a layer of RenderInBody around the component.
export default class Dialog extends Component{ render(){ return { <RenderInBody>i am a dialog render to body</RenderInBody> } } }
Translator added:
By transforming the above components, we can render and unload the components to the specified dom node, and add position control, as follows:
//此组件用于在body内渲染弹层 import React,{Component} from 'react' import ReactDom from 'react-dom'; export default class RenderInBody extends Component{ constructor(p){ super(p); } componentDidMount(){ /** popupInfo={ rootDom:***,//接收弹层组件的DOM节点,如document.body left:***,//相对位置 top:***//位置信息 } */ let {popupInfo} = this.props; this.popup = document.createElement('p'); this.rootDom = popupInfo.rootDom; this.rootDom.appendChild(this.popup); //we can setAttribute of the p only in this way this.popup.style.position='absolute'; this.popup.style.left=popupInfo.left+'px'; this.popup.style.top=popupInfo.top+'px'; this._renderLayer() } componentDidUpdate() { this._renderLayer(); } componentWillUnmount(){ this.rootDom.removeChild(this.popup); } _renderLayer(){ ReactDom.render(this.props.children, this.popup); } render(){ return null; } }
Note: Position acquisition and root node judgment function
export default (dom,classFilters)=> { let left = dom.offsetLeft, top = dom.offsetTop + dom.scrollTop, current = dom.offsetParent, rootDom = accessBodyElement(dom);//默认是body while (current !=null ) { left += current.offsetLeft; top += current.offsetTop; current = current.offsetParent; if (current && current.matches(classFilters)) { rootDom = current; break; } } return { left: left, top: top ,rootDom:rootDom}; } /*** 1. dom:为响应弹层的dom节点,或者到该dom的位置后,可以做位置的微调,让弹层位置更佳合适 * 2. classFilters:需要接收弹层组件的DOM节点的筛选类名 /
I believe you have mastered the method after reading the case in this article. For more exciting information, please pay attention to other related articles on the PHP Chinese website!
Recommended reading:
JS implementation of images being dragged on web pages
What is the life cycle of React Native components?
The above is the detailed content of Use React to render components to specified DOM nodes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
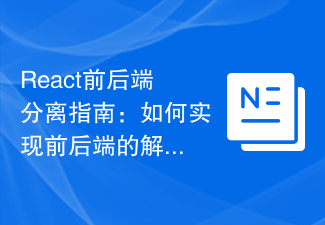
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
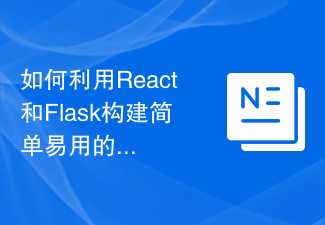
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
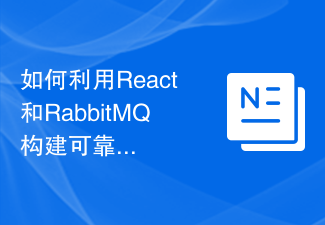
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
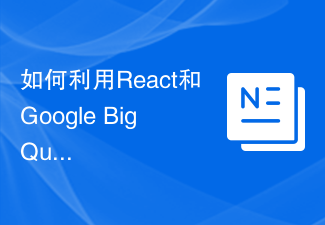
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building
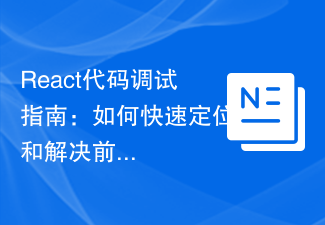
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
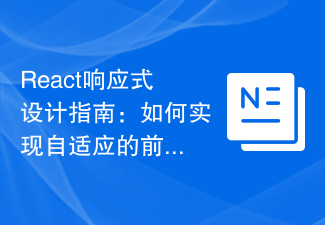
React Responsive Design Guide: How to Achieve Adaptive Front-end Layout Effects With the popularity of mobile devices and the increasing user demand for multi-screen experiences, responsive design has become one of the important considerations in modern front-end development. React, as one of the most popular front-end frameworks at present, provides a wealth of tools and components to help developers achieve adaptive layout effects. This article will share some guidelines and tips on implementing responsive design using React, and provide specific code examples for reference. Fle using React
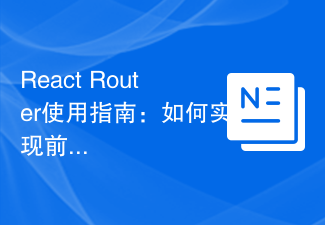
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
