jquery implements carousel chart (with code)
This time I will bring you jquery to implement carousel chart (with code). What are the precautions for jquery to implement carousel chart? The following is a practical case, let’s take a look.
Carousel chart:
I have been exposed to jquery for a while, and today I just used the carousel chart to practice my skills. At the beginning of the blog post, I will introduce an example of simply using jquery to make a carousel chart. In the middle, I will insert some more thoughts about carousel charts. At the end, I will use Javascript method to write a carousel chart. Finally, I will talk about jquery and Javascript. Comparison. The effect of the carousel chart can be viewed by clicking on the following link: http://sandbox.runjs.cn/show/t07kscph
Example of jquery making carousel chart:
HTML part code:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>轮播图</title> <link rel="stylesheet" type="text/css" href="demo.css"/> <script src="../jquery/jquery-2.1.1.min.js" type="text/javascript" charset="utf-8"></script> <script src="demo.js" type="text/javascript" charset="utf-8"></script> </head> <body> <p id="igs"> <a class="ig" href="#"><img src="images/1.jpg"/></a> <a class="ig" href="#"><img src="images/2.jpg"/></a> <a class="ig" href="#"><img src="images/3.jpg"/></a> <a class="ig" href="#"><img src="images/4.jpg"/></a> <a class="ig" href="#"><img src="images/5.jpg"/></a> <p class="btn btn1"><</p> <p class="btn btn2">></p> <ul id="tabs"> <li class="tab">1</li> <li class="tab">2</li> <li class="tab">3</li> <li class="tab">4</li> <li class="tab">5</li> </ul> </p> </body> </html>
css part code:
* { margin: 0; padding: 0; } #igs { margin: 10px auto; width: 700px; height: 320px; position: relative; } .ig { position: absolute; } #tabs { position: absolute; list-style: none; background-color: rgba(255,255,255,.5); left: 300px; bottom: 10px; border-radius: 10px; padding: 5px 0 5px 5px; } .tab{ float: left; text-align: center; line-height: 20px; width: 20px; height: 20px; cursor: pointer; overflow: hidden; margin-right: 4px; border-radius: 100%; background-color: rgb(200,100,150); } .btn{ position: absolute; color: #fff; top: 110px; width: 40px; height: 100px; background-color: rgba(255,255,255,.3); font-size: 40px; font-weight: bold; text-align: center; line-height: 100px; border-radius: 5px; margin: 0 5px; } .btn2{ position: absolute; right: 0px; } .btn:hover{ background-color: rgba(0,0,0,.7); }
js part code:
//定义全局变量和定时器 var i = 0 ; var timer; $(document).ready(function(){ //用jquery方法设置第一张图片显示,其余隐藏 $('.ig').eq(0).show().siblings('.ig').hide(); //调用showTime()函数(轮播函数) showTime(); //当鼠标经过下面的数字时,触发两个事件(鼠标悬停和鼠标离开) $('.tab').hover(function(){ //获取当前i的值,并显示,同时还要清除定时器 i = $(this).index(); Show(); clearInterval(timer); },function(){ // showTime(); }); //鼠标点击左侧的箭头 $('.btn1').click(function(){ clearInterval(timer); if(i == 0){ i = 5;//注意此时i的值 } i--; Show(); showTime(); }); //鼠标点击右侧的箭头 $('.btn2').click(function(){ clearInterval(timer); if(i == 4){ i = -1;//注意此时i的值 } i++; Show(); showTime(); }); }); //创建一个showTime函数 function showTime(){ //定时器 timer = setInterval(function(){ //调用一个Show()函数 Show(); i++; //当图片是最后一张的后面时,设置图片为第一张 if(i==5){ i=0; } },2000); } //创建一个Show函数 function Show(){ //在这里可以用其他jquery的动画 $('.ig').eq(i).fadeIn(300).siblings('.ig').fadeOut(300); //给.tab创建一个新的Class为其添加一个新的样式,并且要在css代码中设置该样式 $('.tab').eq(i).addClass('bg').siblings('.tab').removeClass('bg'); /* * css中添加的代码: * .bg{ background-color: #f00; } * */ }
Completed rendering:
More thoughts on jquery for making carousel pictures
Thought 1: Use the jquery method in the seventh line of code to set the first picture to be displayed and the rest to be hidden. Is there any other way to achieve this?
Idea: Implement it through jquery filters
Code example:
$("#igs a:not( :first-child )").hide();
Extension: If you look at it this way, in the a tag We can omit all the classes in. At the same time, we need to have a deeper understanding of jquery selectors.
Thinking 2: In line 64 of the code, we created a Show function, where we can only see simple effects. Can we make our animation effects more dazzling?
Idea: Use custom animation in jquery to set multiple animation effects
Code example:
//Code tip: You can use fadeIn(), fadeOut (), fadeTo(), animate(), etc. Please refer to relevant information for specific implementation methods
Thinking 3: If we add one or more pictures on the original basis, we have to modify our Code, can we apply this code to more carousel images?
Idea: We set a counter count in front and get the number of pictures through the DOM method
Code example:
var count; $(document).ready(function(){ count= $(".main a").length; /*给动态变化的i备用*/; //。。。代码省略 //鼠标点击左侧的箭头 $('.btn1').click(function(){ clearInterval(timer); if(i == 0){ i = count;//注意此时i的值 } i--; Show(); showTime(); }); //鼠标点击右侧的箭头 $('.btn2').click(function(){ clearInterval(timer); //console.log(count-1); if(i == count-1){ i = -1;//注意此时i的值 } i++; Show(); showTime(); }); });
Use native Javascript method to write a simple wheel Play the picture
Part of the html code:
<p id="container"> <p id="list" style="left: -600px;"> <img src="img/5.jpg" alt="1"/> <img src="img/1.jpg" alt="1"/> <img src="img/2.jpg" alt="2"/> <img src="img/3.jpg" alt="3"/> <img src="img/4.jpg" alt="4"/> <img src="img/5.jpg" alt="5"/> <img src="img/1.jpg" alt="5"/> </p> <p id="buttons"> <span index="1" class="on"></span> <span index="2"></span> <span index="3"></span> <span index="4"></span> <span index="5"></span> </p> <a href="javascript:;" id="prev" class="arrow"><</a> <a href="javascript:;" id="next" class="arrow">></a> </p>
Js part of the code:
<script type="text/javascript"> /* 知识点: */ /* this用法 */ /* DOM事件 */ /* 定时器 */ window.onload = function () { var container = document.getElementById('container'); var list = document.getElementById('list'); var buttons = document.getElementById('buttons').getElementsByTagName('span'); var prev = document.getElementById('prev'); var next = document.getElementById('next'); var index = 1; var timer; function animate(offset) { //获取的是style.left,是相对左边获取距离,所以第一张图后style.left都为负值, //且style.left获取的是字符串,需要用parseInt()取整转化为数字。 var newLeft = parseInt(list.style.left) + offset; list.style.left = newLeft + 'px'; //无限滚动判断 if (newLeft > -600) { list.style.left = -3000 + 'px'; } if (newLeft < -3000) { list.style.left = -600 + 'px'; } } function play() { //重复执行的定时器 timer = setInterval(function () { next.onclick(); }, 2000) } function stop() { clearInterval(timer); } function buttonsShow() { //将之前的小圆点的样式清除 for (var i = 0; i < buttons.length; i++) { if (buttons[i].className == "on") { buttons[i].className = ""; } } //数组从0开始,故index需要-1 buttons[index - 1].className = "on"; } prev.onclick = function () { index -= 1; if (index < 1) { index = 5 } buttonsShow(); animate(600); }; next.onclick = function () { //由于上边定时器的作用,index会一直递增下去,我们只有5个小圆点,所以需要做出判断 index += 1; if (index > 5) { index = 1 } animate(-600); buttonsShow(); }; for (var i = 0; i < buttons.length; i++) { (function (i) { buttons[i].onclick = function () { /* 这里获得鼠标移动到小圆点的位置,用this把index绑定到对象buttons[i]上,去谷歌this的用法 */ /* 由于这里的index是自定义属性,需要用到getAttribute()这个DOM2级方法,去获取自定义index的属性*/ var clickIndex = parseInt(this.getAttribute('index')); var offset = 600 * (index - clickIndex); //这个index是当前图片停留时的index animate(offset); index = clickIndex; //存放鼠标点击后的位置,用于小圆点的正常显示 buttonsShow(); } })(i) } container.onmouseover = stop; container.onmouseout = play; play(); } </script>
Comparison of jquery and Javascript methods
After comparison , we can easily see that the jquery method requires much less code than our Javascript method. In fact, using Javascript directly avoids many problems, such as compatibility issues (this example is not tested, just used for comparison); also, if there are two values of class, separated by spaces, then How should we operate with DOM (idea: use regular expression and array-related methods), so that our code amount will be more; if we want to change the animation effect, we need to modify a lot. , and from the previous introduction, we know that if you want to modify the animation effect, just modify the called animation function directly...
The following words:
This blog post records more of my thinking, among which The specific implementation effects of many methods have not yet been written. Now I am learning jquery while reviewing the Javascript I have learned before. I feel more and more that Javascript is powerful (actually I am weak). There are many things worth studying in depth, and I feel more and more interesting about this thing.
I believe you have mastered the method after reading the case in this article. For more exciting information, please pay attention to other related articles on the php Chinese website!
Recommended reading:
What are the precautions for jQuery version upgrade
Use of $. and $(). in jQuery Detailed explanation
The above is the detailed content of jquery implements carousel chart (with code). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


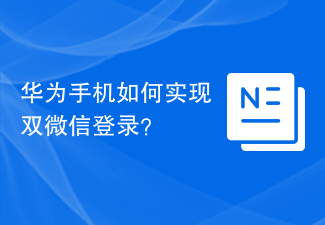
How to implement dual WeChat login on Huawei mobile phones? With the rise of social media, WeChat has become one of the indispensable communication tools in people's daily lives. However, many people may encounter a problem: logging into multiple WeChat accounts at the same time on the same mobile phone. For Huawei mobile phone users, it is not difficult to achieve dual WeChat login. This article will introduce how to achieve dual WeChat login on Huawei mobile phones. First of all, the EMUI system that comes with Huawei mobile phones provides a very convenient function - dual application opening. Through the application dual opening function, users can simultaneously
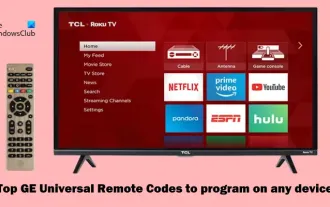
If you need to program any device remotely, this article will help you. We will share the top GE universal remote codes for programming any device. What is a GE remote control? GEUniversalRemote is a remote control that can be used to control multiple devices such as smart TVs, LG, Vizio, Sony, Blu-ray, DVD, DVR, Roku, AppleTV, streaming media players and more. GEUniversal remote controls come in various models with different features and functions. GEUniversalRemote can control up to four devices. Top Universal Remote Codes to Program on Any Device GE remotes come with a set of codes that allow them to work with different devices. you may
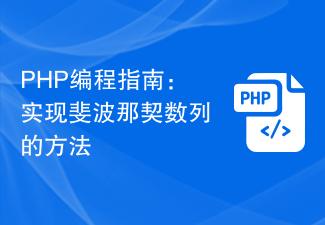
The programming language PHP is a powerful tool for web development, capable of supporting a variety of different programming logics and algorithms. Among them, implementing the Fibonacci sequence is a common and classic programming problem. In this article, we will introduce how to use the PHP programming language to implement the Fibonacci sequence, and attach specific code examples. The Fibonacci sequence is a mathematical sequence defined as follows: the first and second elements of the sequence are 1, and starting from the third element, the value of each element is equal to the sum of the previous two elements. The first few elements of the sequence
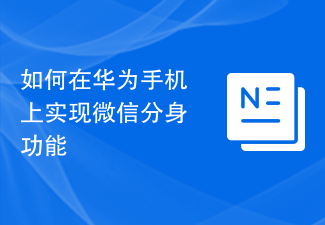
How to implement the WeChat clone function on Huawei mobile phones With the popularity of social software and people's increasing emphasis on privacy and security, the WeChat clone function has gradually become the focus of people's attention. The WeChat clone function can help users log in to multiple WeChat accounts on the same mobile phone at the same time, making it easier to manage and use. It is not difficult to implement the WeChat clone function on Huawei mobile phones. You only need to follow the following steps. Step 1: Make sure that the mobile phone system version and WeChat version meet the requirements. First, make sure that your Huawei mobile phone system version has been updated to the latest version, as well as the WeChat App.
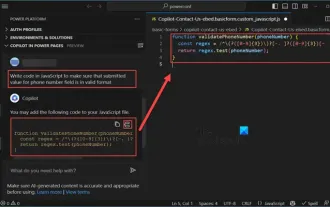
As a programmer, I get excited about tools that simplify the coding experience. With the help of artificial intelligence tools, we can generate demo code and make necessary modifications as per the requirement. The newly introduced Copilot tool in Visual Studio Code allows us to create AI-generated code with natural language chat interactions. By explaining functionality, we can better understand the meaning of existing code. How to use Copilot to generate code? To get started, we first need to get the latest PowerPlatformTools extension. To achieve this, you need to go to the extension page, search for "PowerPlatformTool" and click the Install button
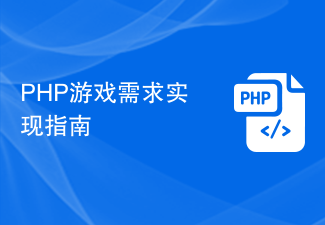
PHP Game Requirements Implementation Guide With the popularity and development of the Internet, the web game market is becoming more and more popular. Many developers hope to use the PHP language to develop their own web games, and implementing game requirements is a key step. This article will introduce how to use PHP language to implement common game requirements and provide specific code examples. 1. Create game characters In web games, game characters are a very important element. We need to define the attributes of the game character, such as name, level, experience value, etc., and provide methods to operate these
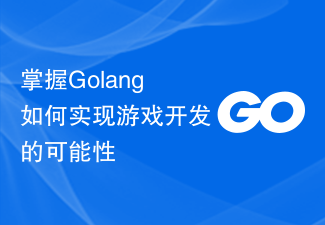
In today's software development field, Golang (Go language), as an efficient, concise and highly concurrency programming language, is increasingly favored by developers. Its rich standard library and efficient concurrency features make it a high-profile choice in the field of game development. This article will explore how to use Golang for game development and demonstrate its powerful possibilities through specific code examples. 1. Golang’s advantages in game development. As a statically typed language, Golang is used in building large-scale game systems.

Working with files in the Linux operating system requires the use of various commands and techniques that enable developers to efficiently create and execute files, code, programs, scripts, and other things. In the Linux environment, files with the extension ".a" have great importance as static libraries. These libraries play an important role in software development, allowing developers to efficiently manage and share common functionality across multiple programs. For effective software development in a Linux environment, it is crucial to understand how to create and run ".a" files. This article will introduce how to comprehensively install and configure the Linux ".a" file. Let's explore the definition, purpose, structure, and methods of creating and executing the Linux ".a" file. What is L
