Detailed explanation of the use of classes in js and typescript
This time I will bring you a detailed explanation of the use of classes in js and typescript. What are the precautions for using classes in js and typescript. The following is a practical case, let's take a look.
Preface
For a front-end developer, class is rarely used because in JavaScript Most of them are functional programming. When you raise your hand, there is a function, and there is almost no trace of class or new. Therefore Design Pattern is also a shortcoming of most front-end developers.
In the process of learning Angular recently, I discovered that it uses a lot of classes. I have to admire it. Angular is indeed an excellent framework that deserves in-depth study.
This article will briefly introduce classes in JavaScript and TypeScript.
Before introducing class, some basic concepts should be introduced first.
1. Static members
Members of the class itself can be inherited, but instances cannot be accessed. They are generally found in tool classes, such as the most common $.ajax in the jQuery era, ajax is $ The static method is easy to use, and there is no need to get a new instance through new or function call.
2. Private members
Members within a class generally cannot be inherited and can only be used internally. Instances cannot be accessed. They are a bit like variables inside a closure, but they are still certain. The difference is that currently JavaScript cannot directly define private members and can only assist in implementation through other methods.
3. Getter/setter
Accessor properties. When we access or modify the properties of an instance, we can intercept these two operations through the accessor properties to do some For other things, Vue uses this API to track data changes.
4. Instance members
refers to the members of the instance created by new, which can be inherited. This feature also enables code reuse.
5. Abstract class, abstract method
Abstract class refers to a class that cannot be instantiated. Calling it through the new keyword will report an error. It is generally designed as a parent class.
Abstract method only provides the name, parameters and return value of the method. It is not responsible for implementation. The specific implementation is completed by the subclass. If a subclass inherits from an abstract class, then the subclass must implement the parent class. All abstract methods, otherwise an error will be reported.
Neither of these two concepts can be directly implemented in JavaScript, but they can be easily implemented in TypeScript or other Object-oriented languages. In addition, this feature is also an important means to achieve polymorphism.
Case introduction
In order to better introduce classes, this article will use three classes as examples, namely Person, Chinese, and American . You can quickly know from the words: Person is the parent class (base class), Chinese and American are subclasses (derived classes).
Person has three attributes: name, age, gender, sayHello method and fullName accessor attribute. At the same time, Person also has some static members and private members. Since it is too difficult to think of examples, let’s use foo, bar, x, y, z instead.
As subclasses, Chinese and American inherit the instance members and static members of Person. At the same time, they also have some methods and attributes of their own:
Chinese has the kungfu attribute and can practice martial arts.
American has twitter and can also sendTwitter.
Next we will use JavaScript and TypeScript to implement this case.
Class in JavaScript
Class in JavaScript should be discussed separately. Two keywords, class and extends, are provided in ES6. Although They are just syntactic sugar, and the bottom layer still uses prototype to implement inheritance, but it cannot be denied that this writing method does make the code clearer and easier to read.
Class in ES6
class Person { // #x = '私有属性x'; // static x = '静态属性x'; // name; // age; // gender; // 上面的写法还在提案中,并没有成为正式标准,不过变化的可能性已经不大了。 // 顺便吐槽一下,用 # 表示私有成员,真的是很无语. /** * Person的静态方法,可以被子类继承 * 可以通过 this 访问静态成员 */ static foo() { console.log(`类 ${this.name} 有一个 ${this.x}`); } constructor(name, age, gender) { this.name = name; this.age = age; this.gender = gender; } /** * 数据存储器,可以访问实例成员,子类的实例可以继承 * 以通过 this 访问实例成员 */ get fullName() { const suffix = this.gender === '男' ? '先生' : '女士'; return this.name + suffix; } set fullName(value) { console.log(`你已改名为 ${value} `); } /** * Person的实例方法,可以被子类的实例继承 * 可以通过 this 访问实例成员 */ sayHello() { console.log(`你好我是 ${this.fullName} ,我 ${this.age} 岁了`); } } Person.x = '静态属性x';
class Chinese extends Person { static bar() { console.log(`类 ${this.name} 的父类是 ${super.name}`); super.foo(); } constructor(name, age, gender, kungfu) { super(name, age, gender); this.kungfu = kungfu; } martial() { console.log(`${this.name} 正在修炼 ${this.kungfu} `); } }
class American extends Person { // static y = '静态属性y'; static bar() { console.log(`类 ${this.name} 有自己的 ${this.y} ,还继承了父类 ${super.name} 的 ${super.x}`); } constructor(name, age, gender, twitter) { super(name, age, gender); this.twitter = twitter; } sendTwitter(msg) { console.log(`${this.name} : `); console.log(` ${msg}`); } }
American.y = '静态属性y'; Person.x; // 静态属性x Person.foo(); // 类 Person 有一个 静态属性x Chinese.x; // 静态属性x Chinese.foo(); // 类 Chinese 有一个 静态属性x Chinese.bar(); // 类 Chinese 的父类是 Person American.x; // 静态属性x American.y; // '静态属性y American.foo(); // 类 American 有一个 静态属性x American.bar(); // 类 American 有自己的 静态属性y ,还继承了父类 Person 的 静态属性x const p = new Person('Lucy', 20, '女'); const c = new Chinese('韩梅梅', 18, '女', '咏春拳'); const a = new American('特朗普', 72, '男', 'Donald J. Trump'); c.sayHello(); // 你好我是 韩梅梅女士 ,我 18 岁了 c.martial(); // 韩梅梅 正在修炼 咏春拳 a.sayHello(); // 你好我是 特朗普先生 ,我 72 岁了 a.sendTwitter('推特治国'); // 特朗普 : 推特治国
The inheritance of class
before ES6 is essentially to create the subclass first Instance object this,
然后再将父类的方法添加到 this 上面 Parent.apply(this) 。
ES6 的继承机制完全不同,实质是先创造父类的实例对象 this,所以必须先调用 super 方法,
然后再用子类的构造函数修改this。
为了实现继承,我们需要先实现一个 extendsClass 函数,它的作用是让子类继承父类的静态成员和实例成员。
function extendsClass(parent, child) { // 防止子类和父类相同名称的成员被父类覆盖 var flag = false; // 继承静态成员 for (var k in parent) { flag = k in child; if (!flag) { child[k] = parent[k]; } } // 继承父类prototype上的成员 // 用一个新的构造函数切断父类和子类之间的数据共享 var F = function () { } F.prototype = parent.prototype; var o = new F(); for (var k in o) { flag = k in child.prototype; if (!flag) { child.prototype[k] = o[k]; } } }
function Person(name, age, gender) { this.name = name; this.age = age; this.gender = this.gender; // 如果将 getter/setter 写在 prototype 会获取不到 Object.defineProperty(this, 'fullName', { get: function () { var suffix = this.gender === '男' ? '先生' : '女士'; return this.name + suffix; }, set: function () { console.log('你已改名为 ' + value + ' '); }, }); } Person.x = '静态属性x'; Person.foo = function () { console.log('类 ' + this.name + ' 有一个 ' + this.x); } Person.prototype = { constructor: Person, // get fullName() { }, // set fullName(value) { }, sayHello: function () { console.log('你好我是 ' + this.fullName + ' ,我 ' + this.age + ' 了'); }, };
function Chinese(name, age, gender, kungfu) { // 用call改变this指向,实现继承父类的实例属性 Person.call(this, name, age, gender); this.kungfu = kungfu; } Chinese.bar = function () { console.log('类 ' + this.name + ' 的父类是 ' + Person.name); Person.foo(); } Chinese.prototype = { constructor: Chinese, martial: function () { console.log(this.name + ' 正在修炼 ' + this.kungfu + ' '); } }; extendsClass(Person, Chinese);
function American(name, age, gender, twitter) { Person.call(this, name, age, gender); this.twitter = twitter; } American.y = '静态属性y'; American.bar = function () { console.log('类 ' + this.name + ' 有自己的 ' + this.y + ' ,还继承了父类 ' + Person.name + ' 的 ' + Person.x); } American.prototype = { constructor: American, sendTwitter: function (msg) { console.log(this.name + ' : '); console.log(' ' + msg); } }; extendsClass(Person, American);
TypeScript 中的 class
讲完了 JavaScript 中的类,还是没有用到 抽象类,抽象方法,私有方法这三个概念,由于 JavaScript 语言的局限性,想要实现这三种概念是很困难的,但是在 TypeScript 可以轻松的实现这一特性。
首先我们稍微修改一下例子中的描述,Person 是抽象类,因为一个正常的人肯定是有国籍的,Person 的 sayHello 方法是抽象方法,因为每个国家打招呼的方式不一样。另外一个人的性别是只能读取,不能修改的,且是确定的是,不是男生就是女生,所以还要借助一下枚举。
enum Gender { female = 0, male = 1 };
abstract class Person { private x: string = '私有属性x,子类和实例都无法访问'; protected y: string = '私有属性y,子类可以访问,实例无法访问'; name: string; public age: number; public readonly gender: Gender; // 用关键字 readonly 表明这是一个只读属性 public static x: string = '静态属性x'; public static foo() { console.log(`类 ${this.name} 有一个 ${this.x}`); } constructor(name: string, age: number, gender: Gender) { this.name = name; this.age = age; this.gender = gender; } get fullName(): string { const suffix = this.gender === 1 ? '先生' : '女士'; return this.name + suffix; } set FullName(value: string) { console.log(`你已改名为 ${value} `); } // 抽象方法,具体实现交由子类完成 abstract sayHello(): void; }
class Chinese extends Person { public kungfu: string; public static bar() { console.log(`类 ${this.name} 的父类是 ${super.name}`); super.foo(); } public constructor(name: string, age: number, gender: Gender, kungfu: string) { super(name, age, gender); this.kungfu = kungfu; } public sayHello(): void { console.log(`你好我是 ${this.fullName} ,我 ${this.age} 岁了`); } public martial() { console.log(`${this.name} 正在修炼 ${this.kungfu} `); } }
class American extends Person { static y = '静态属性y'; public static bar() { console.log(`类 ${this.name} 有自己的 ${this.y} ,还继承了父类 ${super.name} 的 ${super.x}`); } public twitter: string; public constructor(name: string, age: number, gender: Gender, twitter: string) { super(name, age, gender); this.twitter = twitter; } public sayHello(): void { console.log(`Hello, I am ${this.fullName} , I'm ${this.age} years old`); } public sendTwitter(msg: string): void { console.log(`${this.name} : `); console.log(` ${msg}`); } }
Person.x; // 静态属性x Person.foo(); // 类 Person 有一个 静态属性x Chinese.x; // 静态属性x Chinese.foo(); // 类 Chinese 有一个 静态属性x Chinese.bar(); // 类 Chinese 的父类是 Person American.x; // 静态属性x American.y; // '静态属性y American.foo(); // 类 American 有一个 静态属性x American.bar(); // 类 American 有自己的 静态属性y ,还继承了父类 Person 的 静态属性x const c: Chinese = new Chinese('韩梅梅', 18, Gender.female, '咏春拳'); const a: American = new American('特朗普', 72, Gender.male, 'Donald J. Trump'); c.sayHello(); // 你好我是 韩梅梅女士 ,我 18 岁了 c.martial(); // 韩梅梅 正在修炼 咏春拳 a.sayHello(); // Hello, I am 特朗普先生 , I'm 72 years old a.sendTwitter('推特治国'); // 特朗普 : 推特治国
相信看了本文案例你已经掌握了方法,更多精彩请关注php中文网其它相关文章!
推荐阅读:
The above is the detailed content of Detailed explanation of the use of classes in js and typescript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
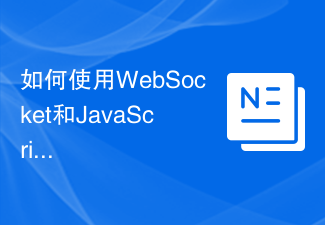
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
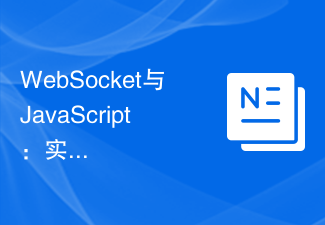
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
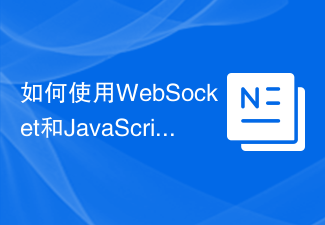
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
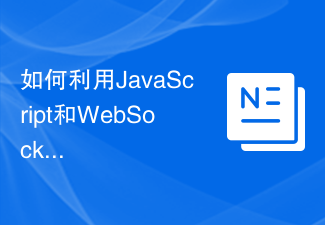
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
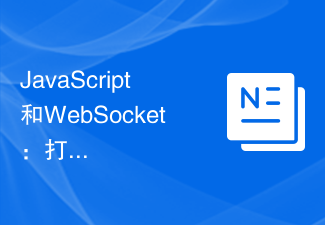
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
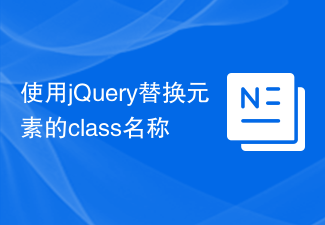
jQuery is a classic JavaScript library that is widely used in web development. It simplifies operations such as handling events, manipulating DOM elements, and performing animations on web pages. When using jQuery, you often encounter situations where you need to replace the class name of an element. This article will introduce some practical methods and specific code examples. 1. Use the removeClass() and addClass() methods jQuery provides the removeClass() method for deletion
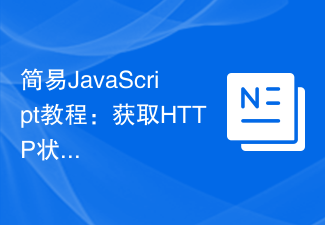
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
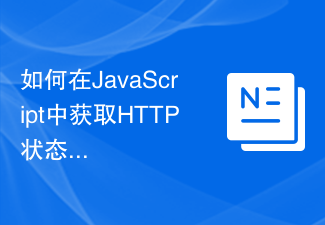
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
