How to insert multiple pieces of data through PHP MySQL
It is often seen inserting data into the database on the website. This article explains how to insert multiple pieces of data into the database.
Use MySQLi and PDO to insert multiple pieces of data into MySQL
mysqli_multi_query() function can be used to execute multiple SQL statements.
The following example adds three new records to the "MyGuests" table:
Example (MySQLi - Object Oriented)
<?php$servername = "localhost";$username = "username";$password = "password";$dbname = "myDB"; // 创建链接$conn = new mysqli($servername, $username, $password, $dbname);// 检查链接if ($conn->connect_error) { die("连接失败: " . $conn->connect_error);} $sql = "INSERT INTO MyGuests (firstname, lastname, email) VALUES ('John', 'Doe', 'john@example.com');";$sql .= "INSERT INTO MyGuests (firstname, lastname, email) VALUES ('Mary', 'Moe', 'mary@example.com');";$sql .= "INSERT INTO MyGuests (firstname, lastname, email) VALUES ('Julie', 'Dooley', 'julie@example.com')"; if ($conn->multi_query($sql) === TRUE) { echo "新记录插入成功";} else { echo "Error: " . $sql . "<br>" . $conn->error;} $conn->close();?>
Please note that each SQL statement must be separated by a semicolon.
Example (MySQLi - Procedure-oriented)
<?php$servername = "localhost";$username = "username";$password = "password";$dbname = "myDB"; // 创建链接$conn = mysqli_connect($servername, $username, $password, $dbname);// 检查链接if (!$conn) { die("连接失败: " . mysqli_connect_error());} $sql = "INSERT INTO MyGuests (firstname, lastname, email) VALUES ('John', 'Doe', 'john@example.com');";$sql .= "INSERT INTO MyGuests (firstname, lastname, email) VALUES ('Mary', 'Moe', 'mary@example.com');";$sql .= "INSERT INTO MyGuests (firstname, lastname, email) VALUES ('Julie', 'Dooley', 'julie@example.com')"; if (mysqli_multi_query($conn, $sql)) { echo "新记录插入成功";} else { echo "Error: " . $sql . "<br>" . mysqli_error($conn);} mysqli_close($conn);?>
Example (PDO)
<?php$servername = "localhost";$username = "username";$password = "password";$dbname = "myDBPDO"; try { $conn = new PDO("mysql:host=$servername;dbname=$dbname", $username, $password); // set the PDO error mode to exception $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // 开始事务 $conn->beginTransaction(); // SQL 语句 $conn->exec("INSERT INTO MyGuests (firstname, lastname, email) VALUES ('John', 'Doe', 'john@example.com')"); $conn->exec("INSERT INTO MyGuests (firstname, lastname, email) VALUES ('Mary', 'Moe', 'mary@example.com')"); $conn->exec("INSERT INTO MyGuests (firstname, lastname, email) VALUES ('Julie', 'Dooley', 'julie@example.com')"); // 提交事务 $conn->commit(); echo "新记录插入成功";}catch(PDOException $e){ // 如果执行失败回滚 $conn->rollback(); echo $sql . "<br>" . $e->getMessage();} $conn = null;?>
Using prepared statements
mysqli extension provides a second way to use for insert statements.
We can prepare statements and bind parameters.
mysql extension can send statements or queries to mysql database without data. You can nematically associate or "bind" variables.
Example (MySQLi uses prepared statements)
<?php$servername = "localhost";$username = "username";$password = "password";$dbname = "myDB"; // 创建连接$conn = new mysqli($servername, $username, $password, $dbname);// 检测连接if ($conn->connect_error) { die("连接失败: " . $conn->connect_error);} else { $sql = "INSERT INTO MyGuests(firstname, lastname, email) VALUES(?, ?, ?)"; // 为 mysqli_stmt_prepare() 初始化 statement 对象 $stmt = mysqli_stmt_init($conn); //预处理语句 if (mysqli_stmt_prepare($stmt, $sql)) { // 绑定参数 mysqli_stmt_bind_param($stmt, 'sss', $firstname, $lastname, $email); // 设置参数并执行 $firstname = 'John'; $lastname = 'Doe'; $email = 'john@example.com'; mysqli_stmt_execute($stmt); $firstname = 'Mary'; $lastname = 'Moe'; $email = 'mary@example.com'; mysqli_stmt_execute($stmt); $firstname = 'Julie'; $lastname = 'Dooley'; $email = 'julie@example.com'; mysqli_stmt_execute($stmt); }}?
We can see that modularization is used to solve the problem in the above example. We can achieve easier reading and management by creating code blocks.
Pay attention to the binding of parameters. Let's take a look at the code in mysqli_stmt_bind_param():
mysqli_stmt_bind_param($stmt, 'sss', $firstname, $lastname, $email);
This function binds parameters Query and pass parameters to the database. The second parameter is "sss" . The following list shows the parameter types. The s character tells mysql that the parameter is a string.
can be the following four parameters:
i - integer
d - double precision floating point number
s - string
b - Boolean value
Each parameter must specify a type to ensure data security. Type judgment can reduce the risk of SQL injection vulnerabilities.
This article explains in detail how to insert multiple pieces of data through php. For more learning materials, please pay attention to the php Chinese website.
Related recommendations:
How to insert data through PHP MySQL
How to create a MySQL table through PHP
How to create a database through PHP MySQL
The above is the detailed content of How to insert multiple pieces of data through PHP MySQL. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


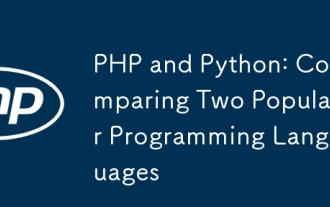
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
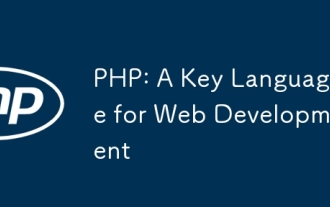
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
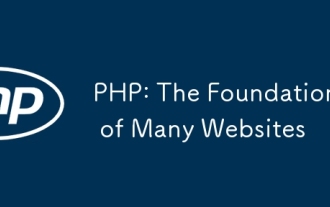
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
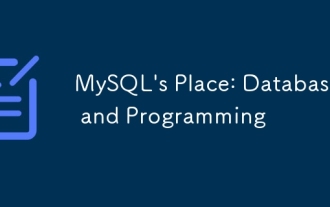
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
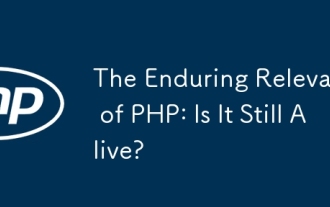
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
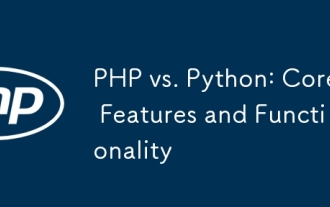
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
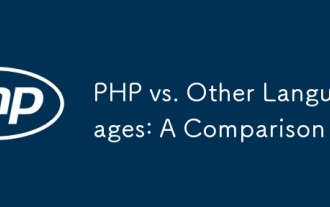
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
