What steps are required to implement JWT user authentication in Koa?
This time I will bring you the steps required for Koa to implement JWT user authentication, and what are the precautions for Koa to implement JWT user authentication. The following is a practical case, let's take a look.
1. Prerequisite knowledge
Token-based authentication
Koajs Chinese Documentation
Koa Framework Tutorial
2. Environment
Microsoft Visual Studio 2017 Integrated Development Environment
Node .js v8.9.4JavascriptRunning environment
##3. Start working and improve step by step
1. Create a basic static resource server and infrastructure The following is the basic code to implement a static server and a handler when token verification is abnormal. Below we will gradually add registration, login, and information functions under this basic code.const path = require('path'); // 用于处理目录路径 const Koa = require('koa'); // web开发框架 const serve = require('koa-static'); // 静态资源处理 const route = require('koa-route'); // 路由中间件 const jwt = require('jsonwebtoken'); // 用于签发、解析`token` const jwtKoa = require('koa-jwt'); // 用于路由权限控制 const koaBody = require('koa-body'); // 用于查询字符串解析到`ctx.request.query` const app = new Koa(); const website = { scheme: 'http', host: 'localhost', port: 1337, join: function () { return `${this.scheme}://${this.host}:${this.port}` } } /* jwt密钥 */ const secret = 'secret'; /* 当token验证异常时候的处理,如token过期、token错误 */ app.use((ctx, next) => { return next().catch((err) => { if (err.status === 401) { ctx.status = 401; ctx.body = { ok: false, msg: err.originalError ? err.originalError.message : err.message } } else { throw err; } }); }); /* 查询字符串解析到`ctx.request.query` */ app.use(koaBody()); /* 路由权限控制 */ // 待办事项…… /* POST /api/register 注册 */ // 待办事项…… /* GET /api/login 登录 */ // 待办事项…… /* GET /api/info 信息 */ // 待办事项…… /* 静态资源处理 */ app.use(serve(path.join(dirname, 'static'))); /* 监听服务器端口 */ app.listen(website.port, () => { console.log(`${website.join()} 服务器已经启动!`); });
Login interface, other resources do not require authentication, and the information interface requires authentication.
/* 路由权限控制 */ app.use(jwtKoa({ secret: secret }).unless({ // 设置login、register接口,可以不需要认证访问 path: [ /^\/api\/login/, /^\/api\/register/, /^((?!\/api).)*$/ // 设置除了私有接口外的其它资源,可以不需要认证访问 ] }));
/* POST /api/register 注册 */ app.use(route.post('/api/register', async (ctx, next) => { const body = ctx.request.body; /* * body = { * user : '御焱', * password : '123456' * } */ // 判断 body.user 和 body.password 格式是否正确 // 待办事项…… // 判断用户是否已经注册 // 待办事项…… // 保存到新用户到数据库中 // 待办事项…… // 是否注册成功 let 是否注册成功 = true; if (是否注册成功) { // 返回一个注册成功的JOSN数据给前端 return ctx.body = { ok: true, msg: '注册成功', token: getToken({ user: body.user, password: body.password }) } } else { // 返回一个注册失败的JOSN数据给前端 return ctx.body = { ok: false, msg: '注册失败' } } })); /* 获取一个期限为4小时的token */ function getToken(payload = {}) { return jwt.sign(payload, secret, { expiresIn: '4h' }); }
/* GET /api/login 登录 */ app.use(route.get('/api/login', async (ctx, next) => { const query = ctx.request.query; /* * query = { * user : '御焱', * password : '123456' * } */ // 判断 query.user 和 query.password 格式是否正确 // 待办事项…… // 判断是否已经注册 // 待办事项…… // 判断姓名、学号是否正确 // 待办事项…… return ctx.body = { ok: true, msg: '登录成功', token: getToken({ user: query.user, password: query.password }) } }));
/* GET /api/info 信息 */ app.use(route.get('/api/info', async (ctx, next) => { // 前端访问时会附带token在请求头 payload = getJWTPayload(ctx.headers.authorization) /* * payload = { * user : "御焱", * iat : 1524042454, * exp : 1524056854 * } */ // 根据 payload.user 查询该用户在数据库中的信息 // 待办事项…… const info = { name: '御焱', age: 10, sex: '男' } let 获取信息成功 = true; if (获取信息成功) { return ctx.body = { ok: true, msg: '获取信息成功', data: info } } else { return ctx.body = { ok: false, msg: '获取信息失败' } } })); /* 通过token获取JWT的payload部分 */ function getJWTPayload(token) { // 验证并解析JWT return jwt.verify(token.split(' ')[1], secret); }
Detailed explanation of the steps to develop mpvue framework with Vue.js
jquery fullpage plug-in to add header and tail copyright Related
#Detailed explanation of the sass configuration steps in vue
The above is the detailed content of What steps are required to implement JWT user authentication in Koa?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


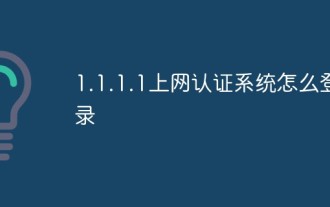
1.1.1.1 Login method for the Internet authentication system: 1. Search for the campus network wireless signal and connect; 2. Open the browser and select "Self-Service" on the pop-up authentication interface; 3. Enter the user name and initial password to log in; 4. Complete Personal information and set a strong password.
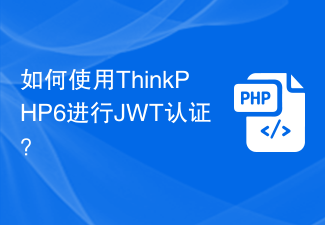
JWT (JSONWebToken) is a lightweight authentication and authorization mechanism that uses JSON objects as security tokens to securely transmit user identity information between multiple systems. ThinkPHP6 is an efficient and flexible MVC framework based on PHP language. It provides many useful tools and functions, including JWT authentication mechanism. In this article, we will introduce how to use ThinkPHP6 for JWT authentication to ensure the security and reliability of web applications
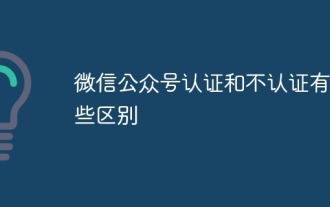
The difference between WeChat public account authentication and non-authentication lies in the authentication logo, function permissions, push frequency, interface permissions and user trust. Detailed introduction: 1. Certification logo. Certified public accounts will obtain the official certification logo, which is the blue V logo. This logo can increase the credibility and authority of the public account and make it easier for users to identify the real official public account; 2. Function permissions. Certified public accounts have more functions and permissions than uncertified public accounts. For example, certified public accounts can apply to activate the WeChat payment function to achieve online payment and commercial operations, etc.
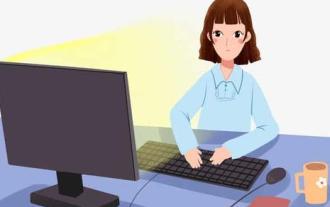
What games can be played with i34150 with 1G independent graphics? Can it play small games such as LoL? GTX750 and GTX750TI are very suitable graphics card choices. If you just play some small games or not play games, it is recommended to use the i34150 integrated graphics card. Generally speaking, the price difference between graphics cards and processors is not very big, so it is important to choose a reasonable combination. If you need 2G of video memory, it is recommended to choose GTX750TI; if you only need 1G of video memory, just choose GTX750. GTX750TI can be seen as an enhanced version of GTX750, with overclocking capabilities. Which graphics card can be paired with i34150 depends on your needs. If you plan to play stand-alone games, it is recommended that you consider changing the graphics card. you can choose

KC certification is to enable consumers to more clearly understand the certification mark marked on the products they purchase. It is a national unified certification mark that is used to reduce the various certification fees borne by product manufacturers. The Korea Institute of Technical Standards (KATS) announced on August 20, 2008 that it would implement KC certification from July 2009 to December 2010.
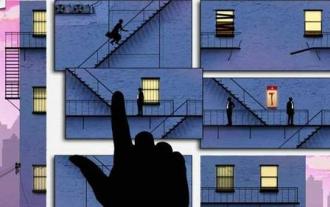
What configurations are needed to use CAD smoothly? To use CAD software smoothly, you need to meet the following configuration requirements: Processor requirements: In order to run "Word Play Flowers" smoothly, you need to be equipped with at least one Intel Corei5 or AMD Ryzen5 or above processor. Of course, if you choose a higher-performance processor, you'll be able to get faster processing speeds and better performance. Memory is a very important component in the computer. It has a direct impact on the performance and user experience of the computer. Generally speaking, we recommend at least 8GB of memory, which can meet the needs of most daily use. However, for better performance and smoother usage experience, it is recommended to choose a memory configuration of 16GB or above. This ensures that the
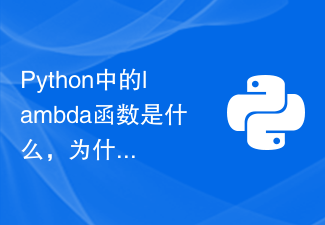
In this article, we will learn about the lambda function in Python and why we need it, and see some practical examples of lambda functions. What is lambda function in Python? A Lambda function is often called an "anonymous function" and is the same as a normal Python function, except that it can be defined without a name. The >def keyword is used to define ordinary functions, while the lambda keyword is used to define anonymous functions. However, they are limited to single-line expressions. They, like regular functions, can accept multiple arguments. Syntax lambdaarguments:expression This function accepts any number of inputs, but only evaluates and returns an expression. Lamb
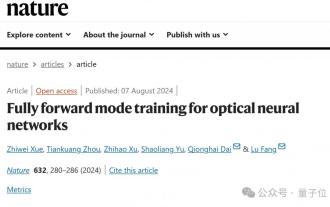
Using light to train neural networks, Tsinghua University results were recently published in Nature! What should I do if I cannot apply the backpropagation algorithm? They proposed a Fully Forward Mode (FFM) training method that directly performs the training process in the physical optical system, overcoming the limitations of traditional digital computer simulations. To put it simply, it used to be necessary to model the physical system in detail and then simulate these models on a computer to train the network. The FFM method eliminates the modeling process and allows the system to directly use experimental data for learning and optimization. This also means that training no longer needs to check each layer from back to front (backpropagation), but can directly update the parameters of the network from front to back. To use an analogy, like a puzzle, backpropagation
