Explanation of JavaScript related functions
There are some functions that frequently appear in JavaScript learning. This article will explain some of them in detail.
.map
The map method will iterate through each element in the array, process each element according to the callback function, and finally return a new array.
Use the map method to add 3 to each item in oldArray and save them in newArray. oldArray should not be changed.
var oldArray = [1,2,3,4,5];var newArray = oldArray.map(function(val){ return val + 3; }); .reduce
The elements in the array are operated on in sequence from left to right, and the last remaining element is the return value
The cumulative value of the last result (previousVal) and the current value (currentVal).
var array = [4,5,6,7,8];var singleVal = 0;singleVal = array.reduce(function(previousVal, currentVal){ return previousVal + currentVal; }); .filter
The filter method is used to iterate an array and filter out matching elements according to the given conditions.
Use filter to create a new array. The value of the new array is the elements in oldArray whose value is less than 6. The original array is not allowed to be changed. oldArray
var oldArray = [1,2,3,4,5,6,7,8,9,10];var newArray = oldArray.filter(function(val){ return val<6; }); .sort
sort method will change the original array and return the sorted array. sort can pass comparison functions as arguments. The comparison function has a return value. When a is less than b, it returns a negative number; when a is greater than b, it returns a positive number; when they are equal, it returns 0. If no comparison function is passed in, it will convert all values into strings and sort them alphabetically.
Use sort to sort the array from small to large.
var array = [1, 12, 21, 2];array.sort(function(a, b) { return a - b;});result: [1,2,12,21] .reverse
Use reverse to flip the array array. And assigned to newArray.
var array = [1,2,3,4,5,6,7];var newArray = [];newArray = array.reverse(); reverse_result.png .concat
concat The parameter of the method should be an array. The array in the parameter will be concatenated after the original array and returned as a new array.
Use .concat() to splice concatMe behind oldArray and assign it to newArray.
concat_result.png .split
You can use the split method to split a string into an array by a specified delimiter. Pass a parameter to the split method, which will be used as a separator.
Use the split method to split the string string into an array array.
No delimiter.
<script type="text/javascript"> var str="How are you doing today?"; var n=str.split(); document.write(n);</script>输出: How are you doing today?
Separate each character of the string.
H,w are y,u d,ing t,day? .join
join method to convert the array into a string. Each element in it can be connected with the connector you specify. This connector is the parameter you want to pass in.
Use the join method, the connector is ' ' to convert the array joinMe into the string joinedString.
var veggies = ["Celery", "Radish", "Carrot", "Potato"];var salad = veggies.join(" and ");console.log(salad); // "Celery and Radish and Carrot and Potato" .replace
will replace
This article explains some common functions in detail, and more For more related knowledge, please pay attention to the php Chinese website.
Related recommendations:
Explanation on jquery DOM& events
What is the difference between innerText and innerHTML of dom object?
The above is the detailed content of Explanation of JavaScript related functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


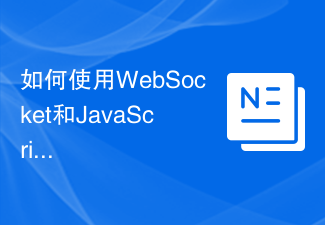
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
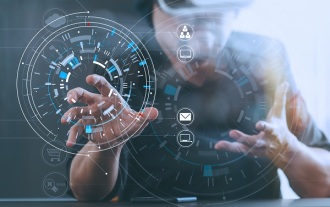
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
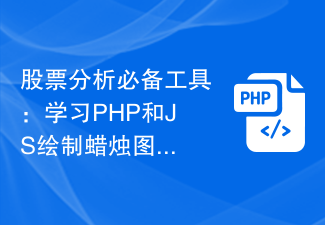
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
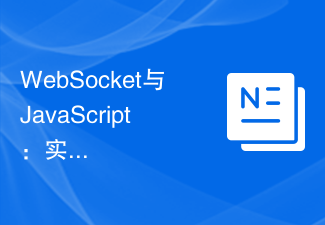
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
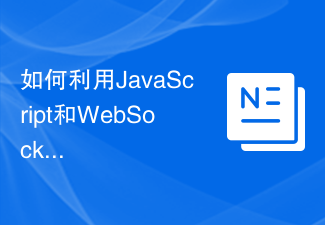
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
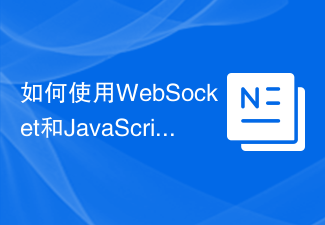
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
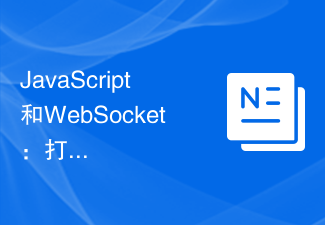
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
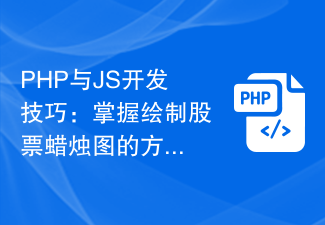
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
