


Detailed interpretation of JavaScript arrow function generator Date JSON
Now I will bring you a brief analysis of JavaScript arrow function generator Date JSON. Let me share it with you now and give it as a reference for everyone.
The ES6 standard adds a new function: Arrow Function.
x => x *x 上面的箭头相当于: function (x){ return x*x; }
Arrow functions are equivalent to anonymous functions and simplify function definition. One like the one above contains only one expression,
even { ... } and return are omitted. There is also a method that can contain multiple statements. In this case, { ... } and return cannot be omitted:
x =>{ if(x > 0){ return x * x; }else{ return -x *x; } }
If there is not one parameter, it needs to be enclosed in brackets ():
// 两个参数 (x,y) => x*x + y *y // 无参数; () =>3.14 // 可变参数 (x,y,...rest) =>{ var i, sum = x +y; for(i=0;i<rest.length;i++){ sum += rest[i]; } return sum; }
this
Now, the arrow function completely fixes the pointing of this. This always points to the lexical scope, which is the outer caller obj:
var obj = { birth:1990, getAge:function(){ var b = this.birth; // 1990 var fn = () => new Date().getFullYear() - this.birth; // this指向obj 对象。 return fn(); } } obj.getAge(); // 25
If arrow functions are used, the previous hack writing method;
var that = this;
is no longer needed.
Since this has been bound in the arrow function according to whether it is scoped or not, when calling the arrow function with call() or apply(), this cannot be
bound, that is The first parameter passed in is ignored.
var obj = { birth:1990, getAge:function(year){ var b = this.burth; // 1990 var fn = (y) =>y-this.birth; // this.birth 仍是1990 return fn.call({birth:2000},year); } }; obj.getAge(2015); // 25
generator
generator (generator) is a new data type introduced by the ES6 standard. A generator looks like a function, but can return multiple times.
function* foo(x){ yield x +1; yieldx + 2; return x +3; }
The difference between generator and function is that generator is defined by function* (note the extra * sign), and, in addition to the return statement, you can also use yield to return multiple times.
The function can only return once, so Tourmaline returns an Array. However, if it is replaced with a generator, it can return one number at a time and multiple times.
function* fib(max){ var t, a = 0, b=1, n=1; while (n < max){ yield a; t = a +b; a = b; b = t; n++; } return a; }
Try calling directly:
fib(5); // fib {[[GeneratorStatus]]: "suspended", [[GeneratorReceiver]]: Window}
Directly calling a generator is different from calling a function. fib(5) only creates a generator object and has not yet executed it.
There are two methods for calling the generator object. One is to continuously call the next() method of the generator object:
var f = fib(5); f.next(); // {value: 0, done: false} f.next(); // {value: 1, done: false} f.next(); // {value: 1, done: false} f.next(); // {value: 2, done: false} f.next(); // {value: 3, done: true}
Date
In JavaScript, Date objects are used to represent dates and times.
To get the current system time, use:
var now = new Date(); now; //// Wed Jun 24 2015 19:49:22 GMT+0800 (CST) now.getFullYear(); //2015,年份 now.getMonth(); // 5,月份,注意月份范围为0~11,5表示六月 now.getDate();// 24 ,表示24 号 now.getHours(); // 3,表示星期三 now.getMinutes(); // 19 ,24小时制 now.getSeconds(); // 22,秒 now.getMilliseconds(); //875 毫秒 now.getTime(); // 1435146562875, 以number形式表示的时间戳 如果要创建一个执行日期和时间的Date对象,可以用: var d = new Date(2015,5,19,20,15,30,123); d;// Fri Jun 19 2015 20:15:30 GMT+0800 (CST)
JSON
JSON is the abbreviation of JavaScript Object Notation, which is A data exchange format.
In JSON, there are only a few data types:
1, number: It is exactly the same as JavaScript’s number;
2, boolean: It is JavaScript’s true or false;
3, String: It is the String of JavaScript;
4, null: It is the null of JavaScript;
5, array: It is the Array representation of JavaScript—— [];
6,object: It is the {...} representation of JavaScript.
SON also stipulates that the character set must be UTF-8, so there is no problem if it represents multiple languages. For unified parsing, JSON strings must use double quotes "", and Object keys must also use double quotes "".
Serialization
var guagua = { name: '小明', age: 14, gender: true, height: 1.65, grade: null, 'middle-school': '\"W3C\" Middle School', skills: ['JavaScript', 'Java', 'Python', 'Lisp'] }; JSON.stringify(xiaoming); // '{"name":"小明","age":14,"gender":true,"height":1.65,"grade":null," middle-school":"\"W3C\" Middle School","skills": ["JavaScript","Java","Python","Lisp"]}'
Result:
{ "name": "小明", "age": 14, "gender": true, "height": 1.65, "grade": null, "middle-school": "\"W3C\" Middle School", "skills": [ "JavaScript", "Java", "Python", "Lisp" ] }
The second parameter is used to control how to filter the key value of the object, if we only want to output the specified Attributes can be passed into Array:
JSON.stringify(xiaoming, ['name', 'skills'], ' ');
Result:
{ "name": "guagua", "skills": [ "JavaScript", "Java", "Python", "Lisp" ] }
Also You can pass in a function, so that each key-value pair of the object will be processed by the function first:
function convert(key, value) { if (typeof value === 'string') { return value.toUpperCase(); } return value; } JSON.stringify(guagua, convert, ' ');
The above code changes all attribute values to uppercase:
{ "name": "guagua", "age": 14, "gender": true, "height": 1.65, "grade": null, "middle-school": "\"W3C\" MIDDLE SCHOOL", "skills": [ "JAVASCRIPT", "JAVA", "PYTHON", "LISP" ] }
If we still want to To precisely control how to serialize Xiaoming, you can define a toJSON() method for xiaoming, which directly returns the data that JSON should serialize:
Deserialization
Get To a string in JSON format, we directly use JSON.parse() to turn it into a JavaScript object:
JSON.parse('[1,2,3,true]'); //[1,2,3,true] JSON.parse('{"name":"瓜瓜","age":14}'); // Object{name:'瓜瓜',age:14} JSON.parse('true'); // true JSON.parse('123.45'):// 123.45 JSON.parse()还可以接收一个函数,用来转换解析出的属性: JSON.parse('{"name":"guagua","age":14}',function(key,value){ //把number * 2 if(key ==='name'){ return value + '同学' } return value; }) ; // Object{name: '瓜瓜同学',age: 14}
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
JavaScript output display content (basic tutorial)
The above is the detailed content of Detailed interpretation of JavaScript arrow function generator Date JSON. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
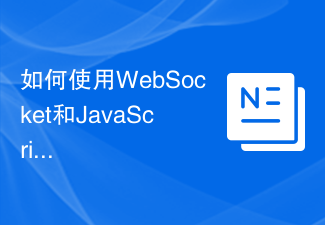
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
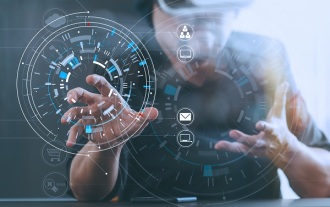
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
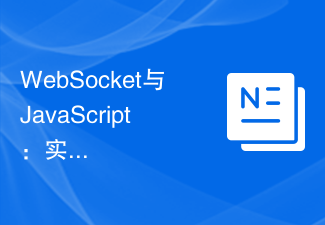
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
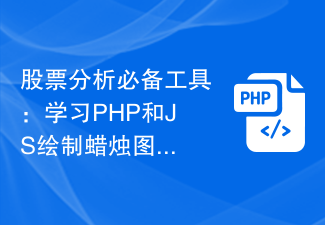
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
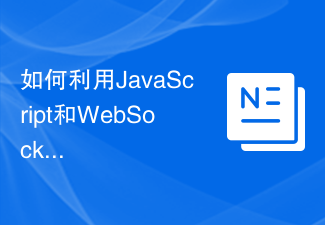
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
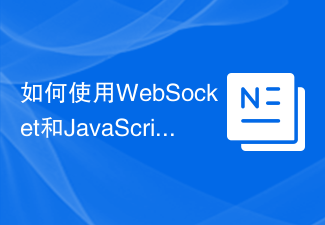
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
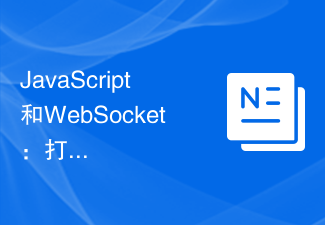
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
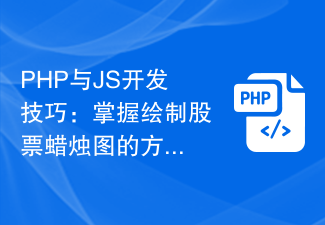
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
