JS basics-Math array Date
This article explains the basic math array and date related knowledge of js.
1.Math
1. Write a function that returns a random integer from min to max, including min but excluding max
//不包含最大数 function getNum(min,max){ //包含最小值,随机最小值与最大值之间的整数且不包含最大值 console.log(min+Math.floor(Math.random()*(max-min))) } getNum(10,15)
2. Write a function, Returns a random integer between min and max, including min and max
//Also includes the maximum number
function getNum2(min,max){ //包含最小值,随机最小值与最大值之间的整数且包含最大值 console.log(min+Math.floor(Math.random()*(max+1-min))) } getNum2(10,15)
3. Write a function to generate a random number of length n String, the value range of string characters includes 0 to 9, a to z, A to Z.
function getRandStr(len){ //补全函数 var str1='' var str = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'; for(var i=0;i<len;i++){ var ss=parseInt(Math.random()*str.length); str1 += str[ss]; } return str1 } var str = getRandStr(10); // 例:0a3iJiRZap console.log(str);
4. Write a function to generate a random IP address. A legal IP address is 0.0.0.0~255.255.255.255
function getRandIP(){ //补全 var ip='' for (var i=0; i<4; i++){ var str=Math.floor(Math.random()*256); ip += str; // 3个点是4个数 if( i<3 ){ ip += "."; } } return ip; }var ip = getRandIP()console.log(ip) // 例:10.234.121.45
5. Write a function to generate a random color character String, the legal color is #000000~ #ffffff
function getRandColor(){ var str = "0123456789abcdef"; var strlen = str.length; var colorstr = "#"; for(var i = 0; i < 6; i++){ var index = Math.floor(Math.random()*strlen); colorstr += str[strlen]; } return colorstr } var color = getRandColor() console.log(color) // 例:#3e2f1b
2. Array
1. What are the functions of push, pop, shift, unshift, join, and splice in the array method? Use the splice function to implement the push, pop, shift, and unshift methods respectively.
push(), pop(), unshift(), and shift() are both operations on the array and will change the array itself. length and content.
The difference is that push() and pop() add and delete from the tail of the array, while unshift() and shift() add and delete from the head of the array.
The splice() method adds/removes items to/from the array and returns the removed item. This method mutates the original array.
The join() method is used to put all elements in the array into a string. Elements are separated by the specified delimiter.
//splice函数分别实现push、pop、shift、unshift方法//例子: var arr = [1,2,3,4,5] //push arr.splice(arr.length,0,6); //位置,要删除元素的个数,要添加到数组的元素 console.log(arr);//[1,2,3,4,5,6] console.log(arr.length) //pop arr.splice(arr.length-1,1) console.log(arr);//[1,2,3,4,5] //shift arr.splice(0,1); console.log(arr);//[2,3,4,5] //unshift arr.splice(0,0,1); console.log(arr);//[1,2,3,4,5]
2. Write a function to operate the array. Each item in the array becomes the original square and operate on the original array.
function squareArr(arr){ for(var i = 0; i < arr.length; i++ ){ arr[i] *= arr[i] } return arr; } var arr = [2, 4, 6] squareArr(arr) console.log(arr) // [4, 16, 36]
3. Write a function to operate the array and return a New array, the new array only contains positive numbers, the original array remains unchanged
function filterPositive(arr){ var newArr = []; for(var i = 0; i < arr.length; i++){ if(typeof(arr[i]) === "number" && arr[i] > 0){ newArr.push(arr[i]); } } return newArr; } var arr = [3, -1, 2, '饥人谷', true] var newArr = filterPositive(arr) console.log(newArr) //[3, 2]
3.Date
1. Write a function getChIntv to get the interval from the current time to the specified date
function getChIntv(s) { //时间=指定时间-当前时间 var time = Math.abs(Date.parse(s) - Date.now()); //天数=时间/天的毫秒 var days = parseInt(time/(1000*60*60*24)); //几小时=时间%天的毫秒/一小时毫秒 var hours = parseInt((time%(1000*60*60*24))/(1000*60*60)); //几分钟=时间%天的毫秒/一小时毫秒/一分钟毫秒 var minutes = parseInt(((time%(1000*60*60*24))%(1000*60*60))/(1000*60)); //几秒=时间%天的毫秒/一小时毫秒/一分钟毫秒/一秒毫秒 var seconds = parseInt(((time%(1000*60*60*24))%(1000*60*60))%(1000*60)/1000); return days + '天' + hours + '小时' + minutes + '分钟' + seconds+ '秒' } var str = getChIntv('2017-7-01'); console.log(str);
2. Change the hh-mm-dd format digital date to Chinese date
function getChsDate(s) { var arr = ['零','一','二','三','四','五','六','七','八','九','十','十一','十二','十三' , '十四','十五','十六','十七','十八','十九','二十','二十一','二十二','二十三','二十四', '二十五','二十六','二十七','二十八','二十九','三十','三十一'] //字符串拆成数组 var time = s.split('-'); // 输出字符串就行 var year = time[0]; var month = time[1]; var day = time[2]; var newYear = arr[year[0]]+arr[year[1]]+arr[year[2]]; var newMonth = arr[parseInt(month)]; var newDay = arr[parseInt(day)]; return newYear+"年"+newMonth+"月"+newDay+"日"; } var str = getChsDate('2017-06-30'); console.log(str); // 二零一七年六月三十日
3. Write a function whose parameters are the string format of the milliseconds of the time object, and the return value is a string. Assume that the parameter is the time object milliseconds t, and the following strings are returned according to the time of t:
// Just now (t is less than 1 minute interval from the current time)
// 3 minutes ago (t greater than or equal to 1 minute, less than 1 hour from the current time)
// 8 hours ago (t greater than or equal to 1 hour from the current time, less than 24 hours)
// 3 days ago (t greater than or equal to 24 hours from the current time) hours, less than 30 days)
// 2 months ago (t is greater than or equal to 30 days from the current time and less than 12 months)
// 8 years ago (t is greater than or equal to 12 months from the current time)
// Code:
function friendlyDate(time){ var msec=new Date() // getTime()返回距 1970 年 1 月 1 日之间的毫秒数 var nowTime=msec.getTime() var sec=(nowTime-time)/1000 if(sec<60){ return '刚刚' }else if(sec>=60&&sec<3600){ return '三分钟前' }else if(sec>=3600&&sec<8*3600){ return '8小时前' }else if(sec>=24*3600&&sec<24*3600*30){ return '3天前' }else if(sec>=24*3600*30&&sec<24*3600*30*12){ return '2个月前' }else if(sec>=24*3600*30*12){ return '8年前' } } var str = friendlyDate( '1484286699422' ) var str2 = friendlyDate('1483941245793') console.log(str) //2个月前 console.log(str2) //2个月前
This article explains the basic knowledge of js. For more knowledge, please pay attention to the php Chinese website.
Related recommendations:
Related knowledge points about front-end cross-domain summary
Common cross-domain solutions for front-end (all)
The above is the detailed content of JS basics-Math array Date. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


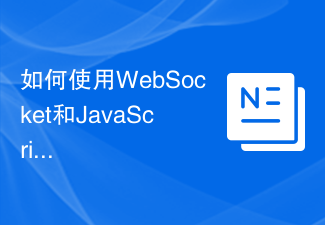
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
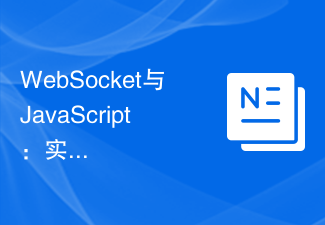
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
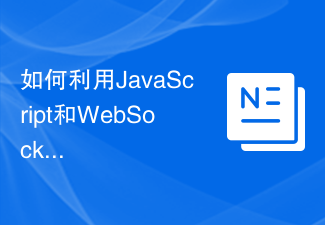
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
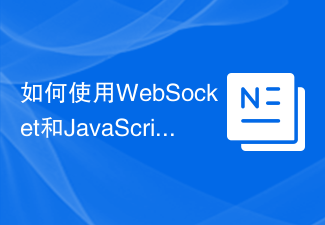
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
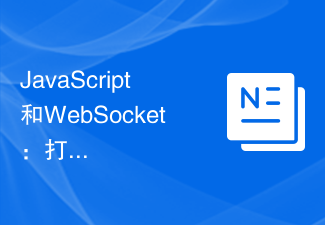
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
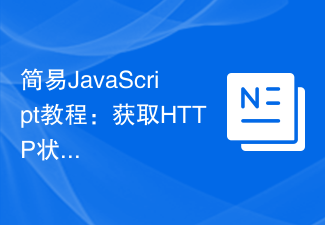
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
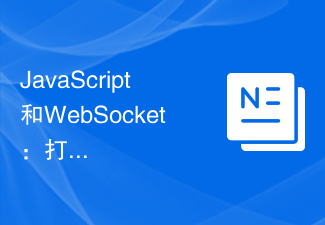
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
